To count the number of lines in a file using PowerShell, you can use the following command:
(Get-Content "C:\path\to\your\file.txt" | Measure-Object -Line).Lines
This command reads the content of the specified file and counts the lines, providing you with the total line count.
Understanding Line Counting in Files
What is Line Counting?
Line counting refers to the process of determining the total number of lines present in a text file. This operation is fundamental in various data processing and management scenarios, such as log analysis, data validation, and content structuring. Counting lines can help pinpoint issues, assess data completeness, and facilitate reporting.
Different Types of Files
When working with line counting, it’s essential to recognize the types of files you may encounter:
- Text files are plain, human-readable files storing data in lines.
- CSV files are comma-separated values that leverage line counting often for data analysis.
- Log files are generated by applications and can be crucial for tracking errors and system behavior.
File Encoding Considerations
When counting lines, consider the file encoding, as it can affect how content is read and interpreted. For instance, a file encoded in UTF-16 may need special handling to read it correctly. Always ensure that your PowerShell script or commandline is compatible with the file's encoding to avoid miscalculations.
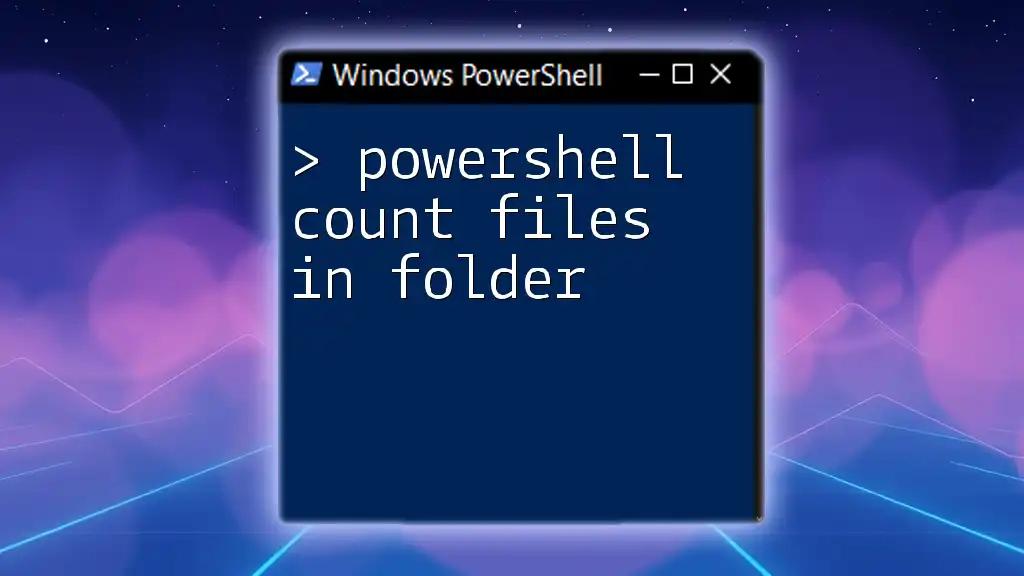
Basic Command to Count Lines
Using `Get-Content`
The simplest way to count lines in a file using PowerShell is through the `Get-Content` cmdlet. The basic syntax for this command is:
Get-Content "filePath" | Measure-Object -Line
Explanation of Components:
- `Get-Content`: This cmdlet is designed to retrieve content from the specified file.
- `Measure-Object -Line`: This portion of the command counts the number of lines retrieved from the file.
Code Snippet Example:
Below is an example of how to use this command effectively:
$lineCount = Get-Content "C:\path\to\your\file.txt" | Measure-Object -Line
Write-Output "Number of lines: $($lineCount.Lines)"
In this snippet, the script retrieves the content of `file.txt`, counts the lines, and then outputs the total.

Advanced Techniques for Counting Lines
Using `Select-Object`
You can also leverage `Select-Object` to manipulate outputs further. The command below demonstrates a way to prepare data for counting lines:
Get-Content "filePath" | Select-Object -First 0
By applying `Select-Object`, you can curate the data output before passing it to the `Measure-Object` for line counting.
Optimizing File Reading
Using `-ReadCount`
Optimizing file reading can significantly improve performance, especially with large files. You can implement reading in chunks using the `-ReadCount` parameter.
Example Implementation:
Get-Content "C:\path\to\your\largefile.txt" -ReadCount 0 | Measure-Object -Line
This command reads the entirety of `largefile.txt` at once, optimizing for speed and efficiency while counting the lines.
Counting Lines with Filtering
Sometimes, you may need to count specific lines that match a certain condition. You can accomplish this using the `Where-Object` cmdlet.
How to Filter Specific Lines:
Here's how you can filter lines containing a particular term:
$searchTerm = "error"
$filteredCount = Get-Content "C:\path\to\your\logfile.log" | Where-Object {$_ -like "*$searchTerm*"} | Measure-Object -Line
Write-Output "Number of '$searchTerm' lines: $($filteredCount.Lines)"
In this example, the script counts only those lines that include the word "error," which is useful during log analysis.
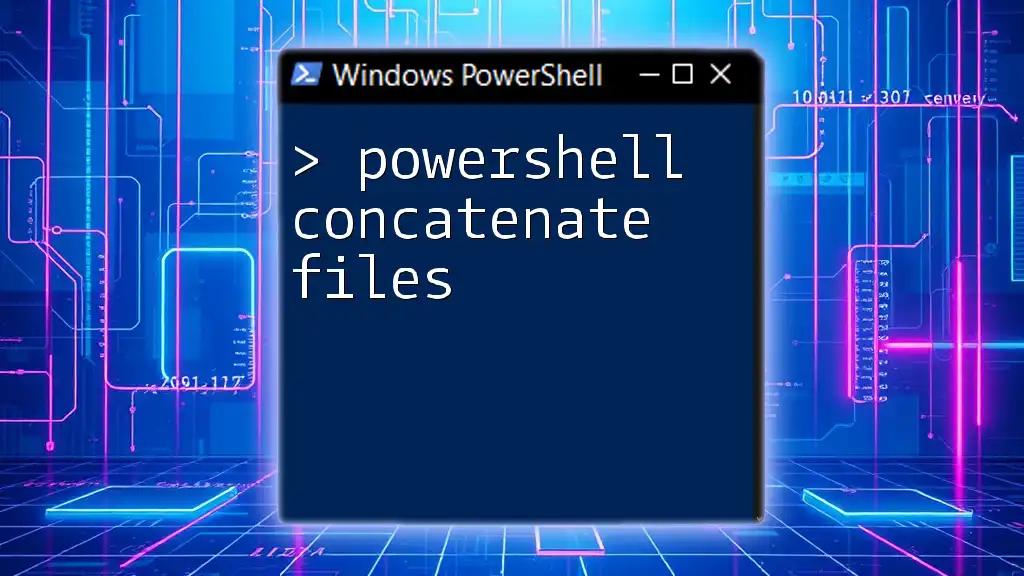
Practical Applications of Line Counting
Log Analysis
One of the most crucial applications of line counting is in log analysis. System logs can serve as an excellent resource for monitoring application health and identifying errors. By counting error lines, you can quickly gauge the severity and frequency of issues, allowing for prompt response and troubleshooting.
Data Validation
Another valuable use case for line counting is data validation. You might need to confirm that a file contains an expected number of records before processing it further. A simple line count can help ensure that the file meets the required standards before it enters your data pipeline.

Potential Issues to Watch Out For
Empty Lines and Comments
When counting lines, be aware of the presence of empty lines and comments. They might skew your results if not addressed properly. You can filter these out by employing a command like:
Get-Content "filePath" | Where-Object {$_ -ne ""}
This command will ignore empty lines and only count lines that contain actual content.
Performance Considerations
While counting lines can be straightforward, there are performance considerations to keep in mind, especially with extremely large files. If the file size is considerable, consider chunking the read process for efficiency. Understanding when to use each method of counting lines is essential for running efficient scripts.

Conclusion
In summary, this guide has walked you through the various methods to efficiently count lines in a file using PowerShell. From the foundational usage of `Get-Content` and `Measure-Object` to advanced filtering techniques, you are now equipped with a toolkit to handle line counting effectively. These skills are vital for tasks such as log analysis and data validation, enabling you to manage and process data with confidence.
Now it's your turn—practice these techniques on your own files and explore the possibilities that PowerShell has to offer! Explore official documentation for commands like `Get-Content`, `Measure-Object`, and seek further tutorials to deepen your knowledge of PowerShell scripting.
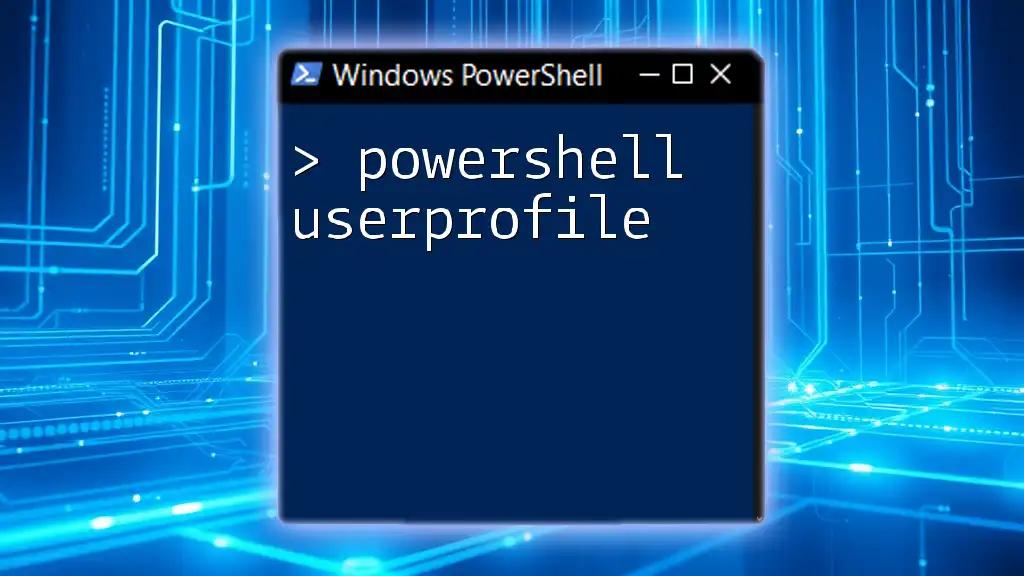
Additional Resources
- Links to Official Documentation: Explore PowerShell’s documentation for more in-depth knowledge.
- Suggested Reading: Additional tutorials and guides on data manipulation techniques and advanced PowerShell scripting methods await your exploration.