In PowerShell, you can instruct the script to continue executing even if an error occurs by using the `$ErrorActionPreference` variable set to `'Continue'`.
Here’s a code snippet illustrating this:
$ErrorActionPreference = 'Continue'
Get-Item 'C:\NonExistentFile.txt' # This will not stop the script execution
Write-Host 'Continuing execution despite error...'
Understanding Error Handling in PowerShell
Types of Errors
In PowerShell, errors can be classified mainly into two types:
Terminating Errors are severe issues that cause the script to stop executing entirely. For example, if you attempt to access a file that doesn’t exist using `Get-Content`, PowerShell will generate a terminating error that interrupts the flow of your script.
Non-terminating Errors, on the other hand, allow the script to continue running despite the error. This type of error might occur when you try to access a property of a nonexistent object. PowerShell will generate an error message, but the script continues execution. Understanding these distinctions is crucial for effective error handling.
Error Action Preferences
The behavior of error handling in PowerShell can be adjusted using the `$ErrorActionPreference` variable. By default, this variable is set to `Continue`, which means that non-terminating errors will be reported and allow the script to continue running.
Changing this variable to `Stop` will change the behavior, making all errors terminate the script execution. For example, setting `$ErrorActionPreference = "Stop"` forces PowerShell to treat all errors as terminating errors, significantly affecting how scripts run and handle exceptions.
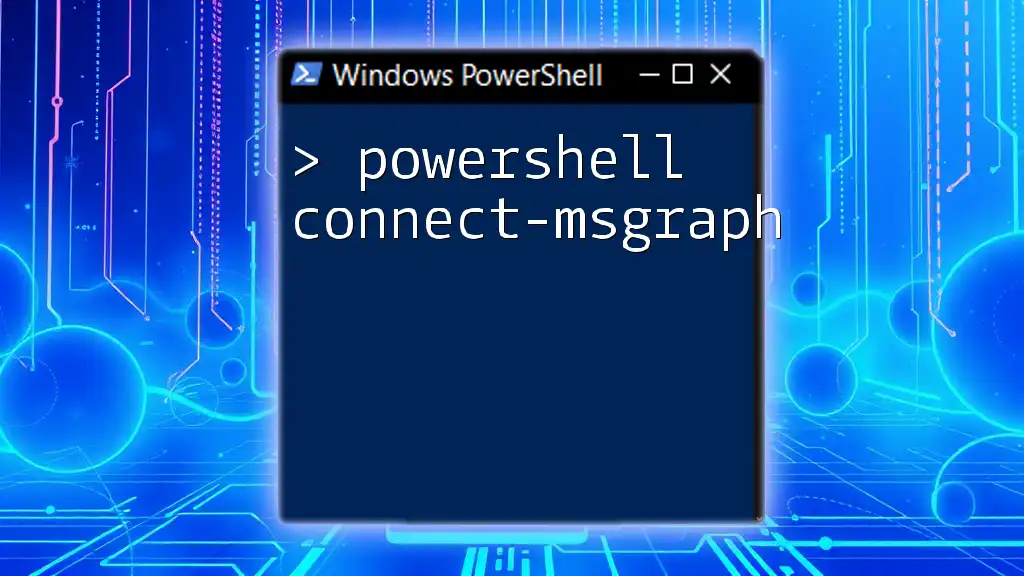
The `-ErrorAction` Parameter
Overview of `-ErrorAction`
The `-ErrorAction` parameter provides a way to adjust error handling for individual Cmdlets within your script. This parameter allows you to specify how PowerShell should react to errors encountered during execution.
Here are some common values for `-ErrorAction`:
- Continue: The default behavior where PowerShell displays an error message but continues executing the script.
- SilentlyContinue: The command fails without displaying an error message, and the script continues.
- Stop: The script terminates execution immediately upon encountering the error.
How to Use `-ErrorAction`
You can use the `-ErrorAction` parameter directly in your Cmdlet to demonstrate its functionality. For example:
Get-Content "nonexistentfile.txt" -ErrorAction Continue
In this case, PowerShell tries to fetch the contents of "nonexistentfile.txt". Instead of stopping the script, it will display an error message and continue executing the remaining commands.
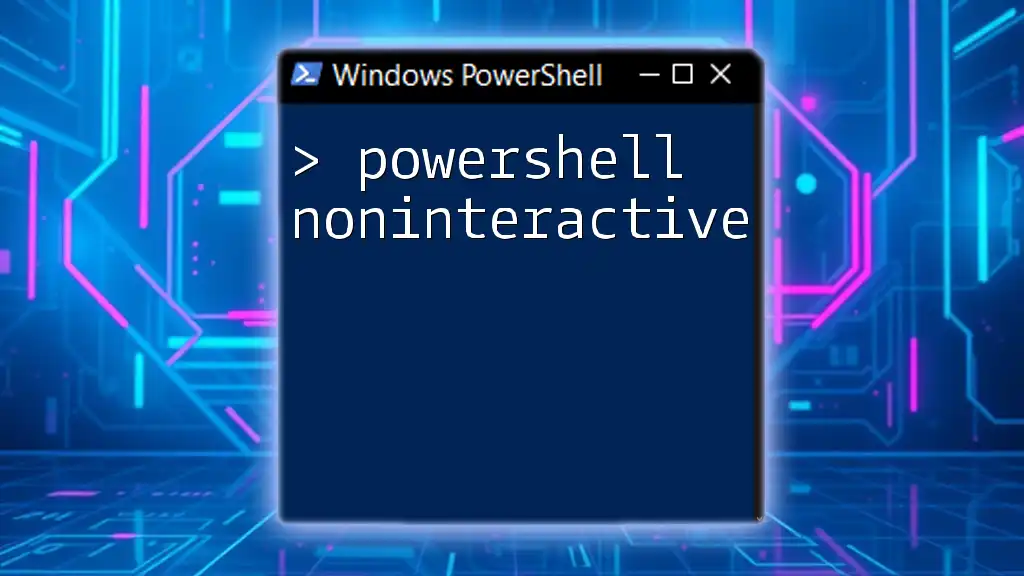
Implementing `Try`, `Catch`, and `Finally` Blocks
Introduction to Exception Handling
PowerShell provides structured error handling through `Try`, `Catch`, and `Finally` blocks. This is particularly useful for managing errors in a way that maintains control over the execution flow, allowing you to handle unexpected situations gracefully.
Using `Try` with `Continue on Error`
Inserting the `Try` block into your script allows you to define a section of code to test for errors. If an error occurs, you can handle it in the `Catch` block. Here's an example:
try {
Get-Content "file1.txt" -ErrorAction Stop
} catch {
Write-Host "An error occurred: $_"
} finally {
Write-Host "Execution continues"
}
In this example, PowerShell attempts to read "file1.txt". If it fails (assuming the file does not exist), control passes to the `Catch` block, where the error is captured and displayed. Regardless of whether an error occurs, the `Finally` block always executes, confirming that the script can continue.
The Role of `Finally`
The `Finally` block is particularly important for cleanup operations that should always occur, regardless of whether an error was encountered. For instance, if you allocate resources or open connections, using `Finally` ensures they get released properly, avoiding leaks or unresolved states.
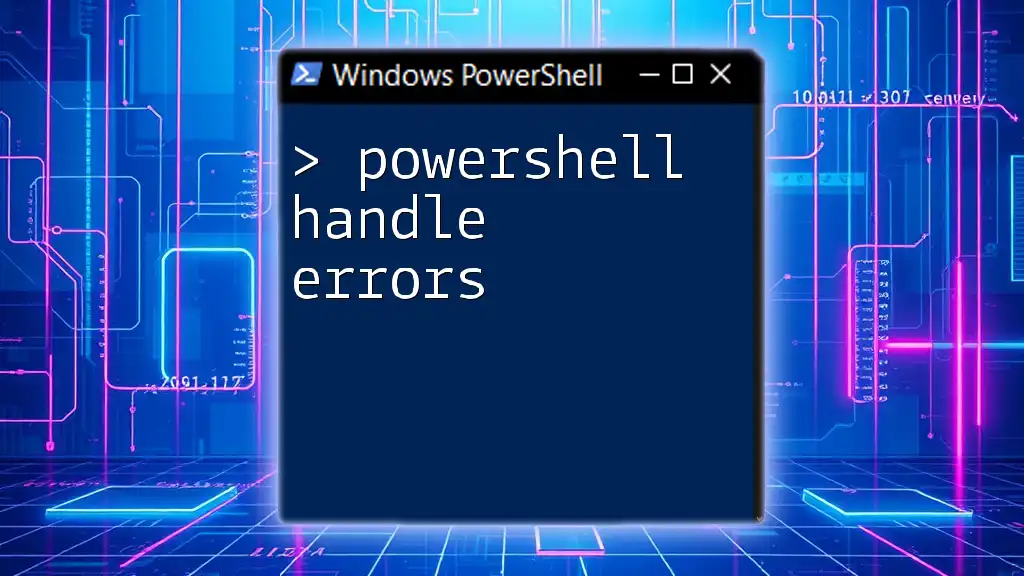
Customizing Error Handling: Using `$Error` Array
What is the `$Error` Variable?
The `$Error` variable in PowerShell is an automatic variable that holds an array of error objects. Each object represents the error that occurred during the session, allowing you to control and log errors more effectively.
Examples of Using `$Error`
You can leverage the `$Error` variable to capture and respond to errors without terminating your script. Consider this example:
Get-Content "wrongfile.txt" -ErrorAction SilentlyContinue
if ($Error.Count -gt 0) {
Write-Host "Errors occurred, but script continues."
}
In this case, the attempt to read "wrongfile.txt" fails, but because we set `-ErrorAction` to `SilentlyContinue`, no error message is displayed. Instead, we check the `$Error` array to determine if any errors were raised and take action accordingly. This approach gives you control over the output and allows your script to remain user-friendly and resilient.
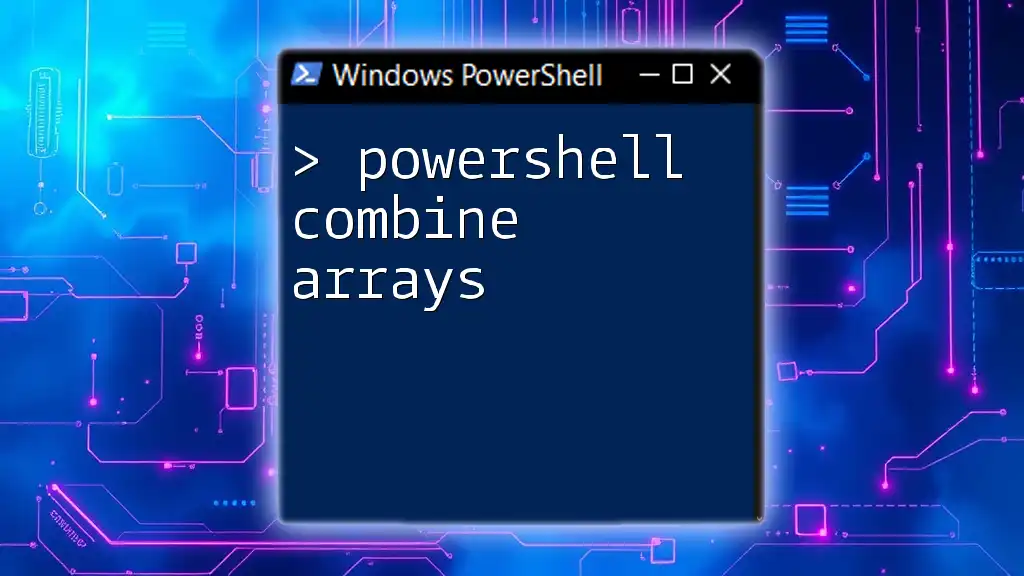
Best Practices for Error Handling in PowerShell
Logging Errors
One of the best practices in error handling is logging errors to aid in diagnosis and troubleshooting. Logging helps you understand when and where errors occur, especially in complex scripts that automate critical tasks. For instance, you can log errors like this:
try {
# Your command here
} catch {
Add-Content -Path "error.log" -Value $_
}
This approach captures errors and appends them to "error.log," creating a record that you can review later to identify issues.
When to Use `Continue on Error`
Understanding when to implement the "continue on error" approach is essential. It’s beneficial in scenarios where the execution of subsequent commands depends on the previous commands not being critical failures. For example, when merging multiple files, one missing file should not halt the overall process, allowing you to report errors later instead.
Conversely, there are situations where halting execution is necessary, such as when dealing with vital data operations or configuration scripts. Weighing these considerations will help you decide effectively.
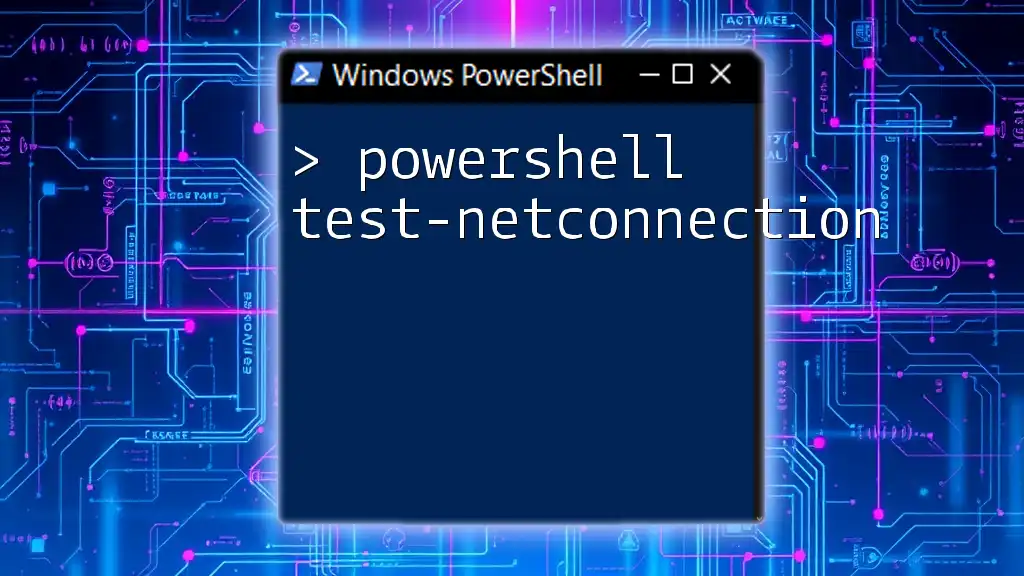
Conclusion
Handling errors effectively is vital for writing robust PowerShell scripts. By understanding the behavior of terminating and non-terminating errors, using `-ErrorAction`, and implementing structured error-handling blocks like `Try`, `Catch`, and `Finally`, you can create cleaner, more reliable scripts. Additionally, customizing error handling using the `$Error` array and following best practices such as logging will elevate your scripting expertise.
Practice these techniques, and you'll undoubtedly become proficient in using PowerShell commands, particularly the `powershell continue on error` strategy that ensures your scripts are resilient and user-friendly. As you explore further, always remember that clear, concise error handling is the hallmark of a competent PowerShell scripter, and it can significantly enhance your scripting capabilities.