PowerShell errors occur when a command fails to execute properly, often due to issues such as syntax errors or invalid parameters.
Get-Content "C:\nonexistentfile.txt"
What is PowerShell?
PowerShell is a powerful scripting language and automation framework developed by Microsoft. It allows system administrators and users to automate tasks, manage configurations, and work with systems through a command-line interface. A key feature of PowerShell is its robustness, which includes an extensive set of cmdlets (commands) designed to manage data and systems efficiently.
The ability to handle errors effectively is crucial when writing PowerShell scripts. Proper error handling not only helps to identify issues quickly but also ensures that scripts can fail gracefully, providing useful feedback to users.
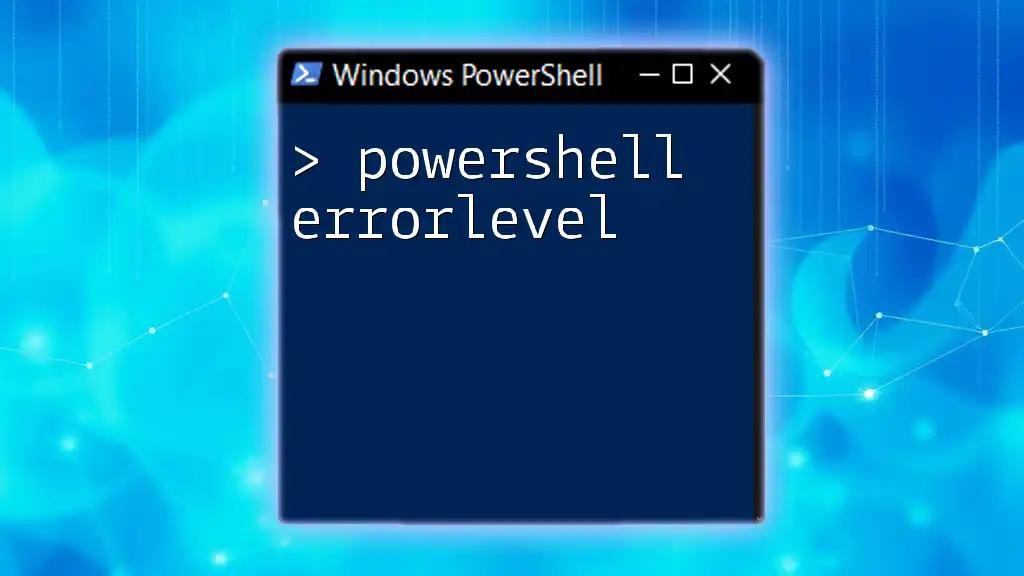
Understanding Errors in PowerShell
Errors in PowerShell signify that something has gone wrong during the execution of a command or script. Being able to identify and respond to these errors is essential for creating reliable and efficient scripts.
Types of Errors
Syntax Errors
A syntax error occurs when the code does not conform to the rules of the PowerShell language. This means that PowerShell cannot interpret the command correctly. Common causes of syntax errors include:
- Missing quotation marks
- Incorrect use of parentheses or braces
- Typos in command names or parameters
Example of a Syntax Error
Get-Content "C:\path\to\file.txt
In the above example, the missing closing quotation mark results in a syntax error, preventing PowerShell from executing the command.
Runtime Errors
Runtime errors occur during the execution of a command, typically due to the state of the system or invalid operations. These errors can be caused by:
- Incorrect file paths
- Permissions issues
- Missing resources
Example of a Runtime Error
Get-Content "C:\path\to\nonexistentfile.txt"
In this instance, attempting to retrieve the content of a nonexistent file leads to a runtime error. PowerShell returns an error message indicating that the specified file could not be found.
Logical Errors
Logical errors are tricky as they don’t cause a script to fail outright; instead, the script runs without any errors but produces incorrect results. This is typically due to flawed logic or incorrect assumptions in the code.
Example of a Logical Error
$sum = 0
foreach ($number in 1..5) {
$sum += $number * 2
}
Write-Output "The sum is $sum" # Expected 30, but it is actually 20
In the example above, the expected output should be 30, but due to the logic used in the script, the actual output is 20. This can be particularly challenging to identify and debug.

Handling Errors in PowerShell
Error handling is essential for robust PowerShell scripts. Using error handling constructs allows you to gracefully manage and respond to potential issues.
Try, Catch, and Finally Blocks
The `try`, `catch`, and `finally` blocks in PowerShell provide a structured way to handle exceptions. This technique allows developers to catch errors and perform specific actions if an error occurs.
Syntax and Usage
try {
# Code that may cause an error
} catch {
# Code that runs if an error occurs
} finally {
# Code that runs regardless of error
}
Example of Try, Catch, and Finally
try {
Get-Content "C:\path\to\nonexistentfile.txt"
} catch {
Write-Host "An error occurred: $_"
} finally {
Write-Host "Execution completed."
}
In this example, if the `Get-Content` command fails, the error message will be displayed, and the `finally` block will execute, indicating the end of the script execution. This structure ensures that you can manage errors effectively while keeping the user informed.
ErrorAction Preference
The `$ErrorActionPreference` variable allows you to control how PowerShell responds to errors. By default, it is set to “Continue”, which means that PowerShell will display the error message and proceed with the execution of subsequent commands.
Usage Example
$ErrorActionPreference = 'Stop'
Get-Content "C:\path\to\nonexistentfile.txt"
By setting `$ErrorActionPreference` to `'Stop'`, the execution will halt upon encountering an error, allowing for important error handling scenarios without proceeding to further commands.
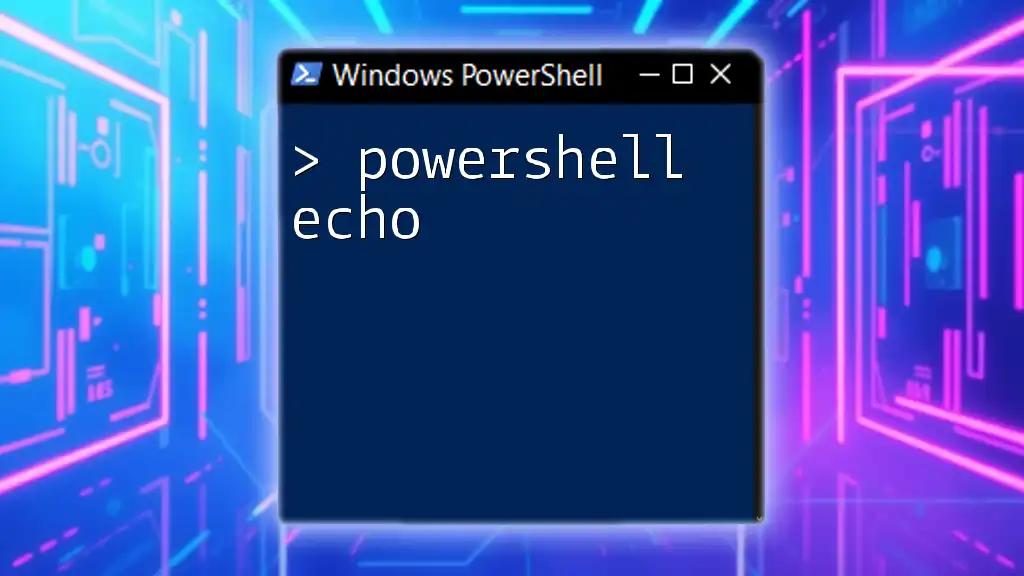
Troubleshooting Common PowerShell Errors
Identifying the Source of the Error
To troubleshoot PowerShell errors effectively, you can utilize the `Get-Error` cmdlet, which helps to identify and display details regarding the most recent error that occurred. This cmdlet provides valuable context that can guide debugging efforts.
Resources for Debugging
PowerShell offers built-in output commands like `Write-Host` and `Write-Output`. Using these commands strategically can significantly improve your ability to log and display messages, thus enhancing script debugging.
Write-Host "Processing file..."
By placing `Write-Host` statements at critical points in your script, you can trace the workflow and monitor execution, helping identify where errors may arise.
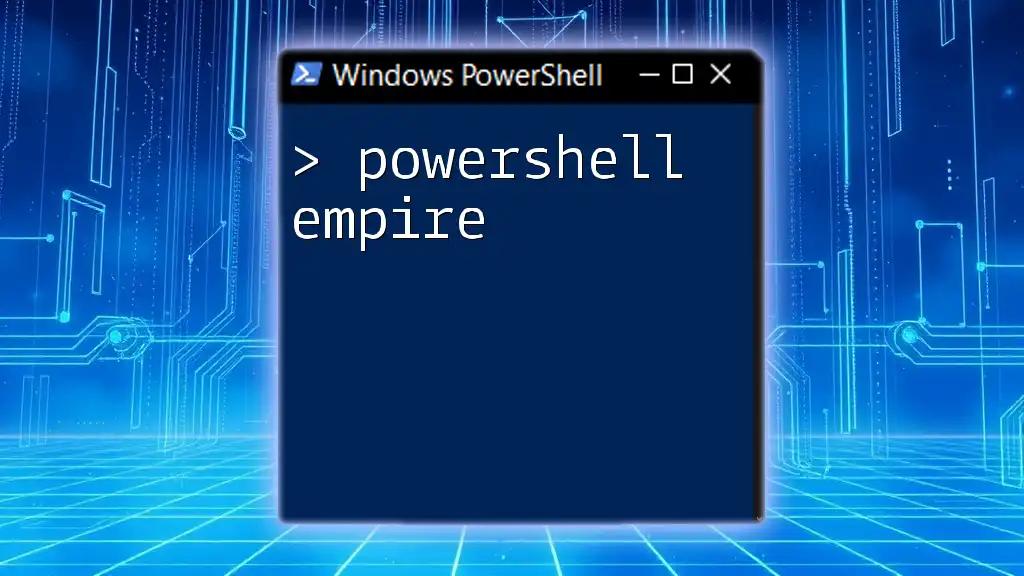
Best Practices for Avoiding Errors
Code Reviews and Testing
Regular code reviews and thorough testing are essential practices to identify potential issues before they escalate. Implementing a robust review process helps catch errors and improve code quality.
Utilizing PowerShell ISE and VS Code
PowerShell Integrated Scripting Environment (ISE) and Visual Studio Code (VS Code) offer advanced debugging features. These tools can help catch errors early during development through syntax highlighting, debugging windows, and integrated help.

Conclusion
In summary, understanding and handling PowerShell errors is fundamental to scripting success. By familiarizing yourself with the various types of errors—syntax, runtime, and logical—you can write cleaner, more effective scripts. Employing structured error handling mechanisms such as `try`, `catch`, and `finally`, along with best practices for troubleshooting, will elevate your scripting skills. Embrace the challenge of learning and experimenting with PowerShell to enhance your automation and system management tasks endlessly.
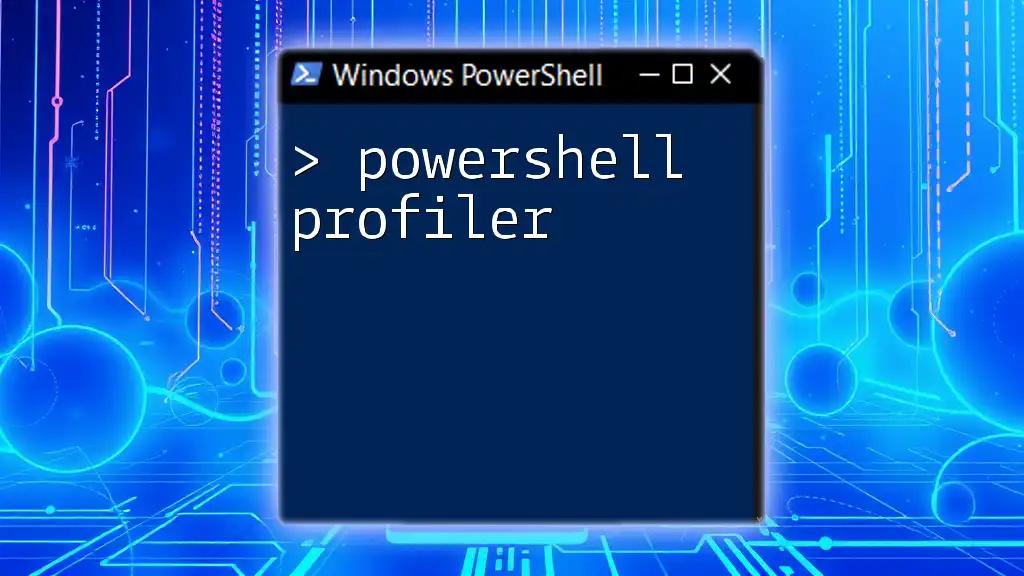
Additional Resources
For further knowledge, consider consulting the official PowerShell documentation, enrolling in relevant courses, or engaging with online forums and communities where PowerShell enthusiasts share tips and insights. These resources will aid you in continuously improving your skills and troubleshooting capabilities in PowerShell.