The `-ErrorAction Stop` parameter in PowerShell is used to immediately halt execution when an error occurs, allowing for more controlled error handling in scripts. Here's a code snippet demonstrating its use:
Get-Content 'nonexistentfile.txt' -ErrorAction Stop
Understanding ErrorAction Parameter
What is the ErrorAction Parameter?
The `ErrorAction` parameter in PowerShell is a fundamental feature designed to manage error handling during the execution of commands or scripts. When a command fails or encounters an error, `ErrorAction` determines how the script will respond. This parameter helps maintain control over script execution and provides flexibility in handling unexpected situations.
Types of ErrorAction Values
PowerShell recognizes several values for the `ErrorAction` parameter, each influencing how errors are processed:
-
Continue: This is the default behavior that allows the script to continue executing, even when an error occurs. While useful in many scenarios, it can lead to unnoticed failures.
-
Stop: With this value, execution is halted immediately when an error is encountered. This is critical for ensuring the integrity of the script, especially in important or sensitive operations.
-
SilentlyContinue: Using this value suppresses error messages and continues execution. While it can reduce clutter, it may lead to silent failures that can complicate troubleshooting.
-
Inquire: This value prompts the user for input when an error occurs, asking what action should be taken next. It introduces a level of interactivity into the script.
-
Ignore: This value ignores errors completely, which can be beneficial in certain contexts but is generally not recommended as it can lead to lost or corrupted data.
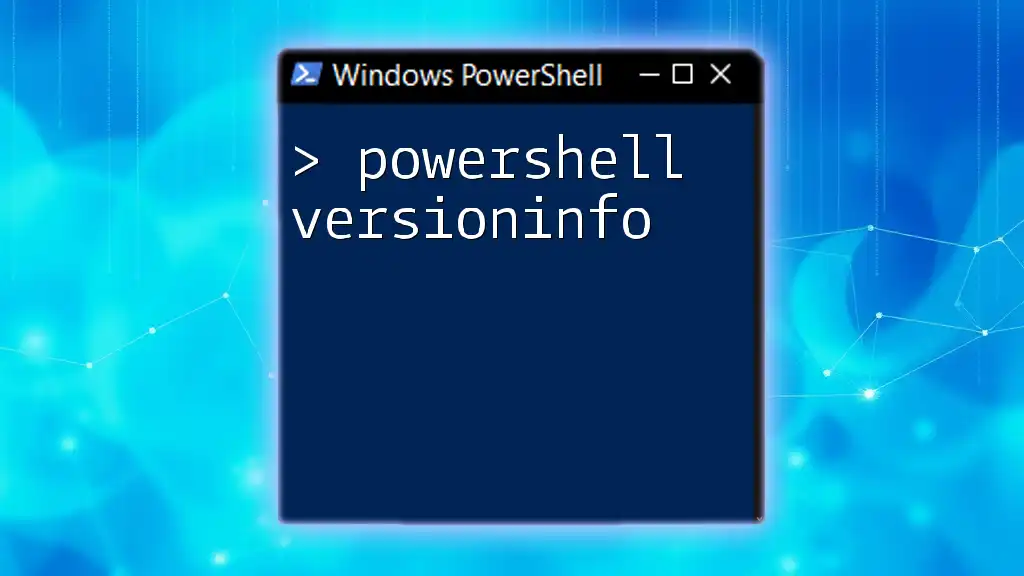
Deep Dive into ErrorAction Stop
What Does ErrorAction Stop Do?
Using `ErrorAction Stop` effectively turns every error into a critical warning, stopping the execution of the current script or command. This functionality ensures that no further actions are taken until the user addresses the error, providing a safeguard for maintaining stability.
When to Use ErrorAction Stop
Utilizing `ErrorAction Stop` is essential when you want your script to halt for any errors that occur. Here are some scenarios where it is particularly useful:
-
In production environments, where script failures may lead to significant consequences, immediate stopping on error can help avoid irreversible issues.
-
During data migration processes, ensuring all operations complete successfully is critical.
-
For scripts that perform system modifications, such as changing configurations or administrative tasks, stopping execution can prevent making changes based on incomplete or erroneous conditions.
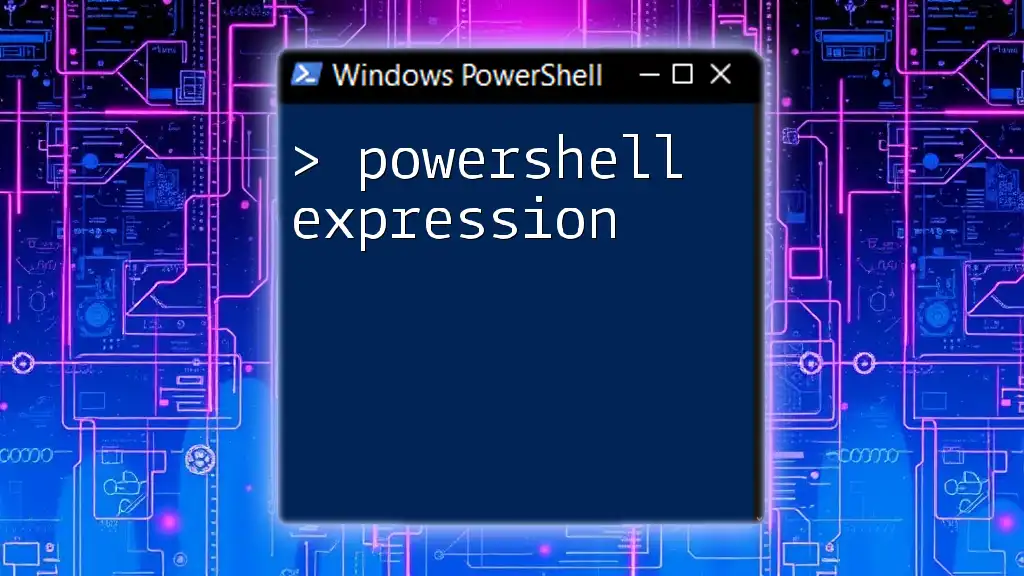
Examples of Using ErrorAction Stop
Basic Example
A straightforward example of using `ErrorAction Stop` is with the `Get-Content` command. If you attempt to read a non-existent file, the command will produce an error:
Get-Content "nonexistentfile.txt" -ErrorAction Stop
This command will halt execution immediately when the error is encountered, providing feedback that the specified file could not be found. This behavior is invaluable for quickly identifying issues.
Practical Scenario: File Operations
Overview
File manipulation is a common task in PowerShell scripts, including operations like copying or renaming files. Using `ErrorAction Stop` during these operations can help maintain data integrity.
Example Code Snippet
Consider the following code, where you want to copy a file:
Copy-Item "source.txt" "destination.txt" -ErrorAction Stop
In this case, if `source.txt` does not exist, the PowerShell execution stops, preventing the creation of an incomplete or incorrect `destination.txt` file. It empowers users to take corrective actions immediately.
Combining ErrorAction with Try-Catch
Syntax Overview
A powerful combination in PowerShell for robust error handling is using `ErrorAction Stop` within a `Try-Catch` block. This offers more granular control over how errors are handled.
Example Code Snippet
Here’s how you can structure your code:
Try {
Get-Content "nonexistentfile.txt" -ErrorAction Stop
} Catch {
Write-Host "An error occurred: $_"
}
In this example, if the `Get-Content` command fails, execution stops, and the `Catch` block captures the error. The `$_` variable within the `Catch` block contains the error message, allowing you to display it or log it for further investigation.
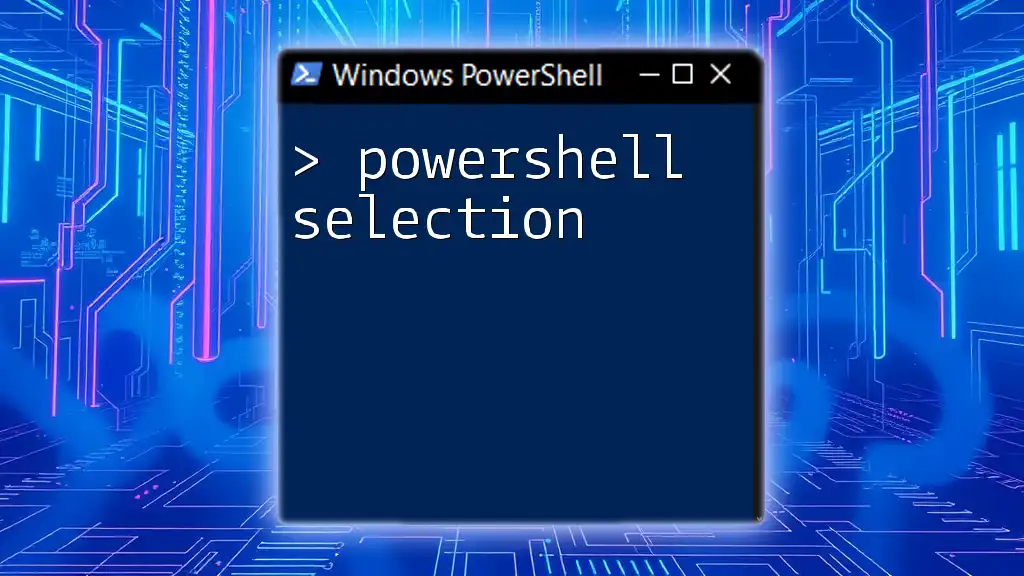
Best Practices for Using ErrorAction Stop
Use in Critical Operations
Utilizing `ErrorAction Stop` is particularly important in critical operations where the outcome greatly impacts your environments, such as scripts used for system configurations or deployments. It acts as a safeguard against unintended actions and ensures that each step completes successfully before proceeding.
Debugging and Testing
During script development, using `Stop` can reveal hidden errors early in the process. It encourages developers to think critically about error management, making debugging more straightforward by highlighting where problems occur.
Documentation and Logging
When using `ErrorAction Stop`, documenting how your script handles errors is essential. Make sure to log errors meaningfully, capturing the circumstances under which they occur. This information can be invaluable for future troubleshooting and maintenance.
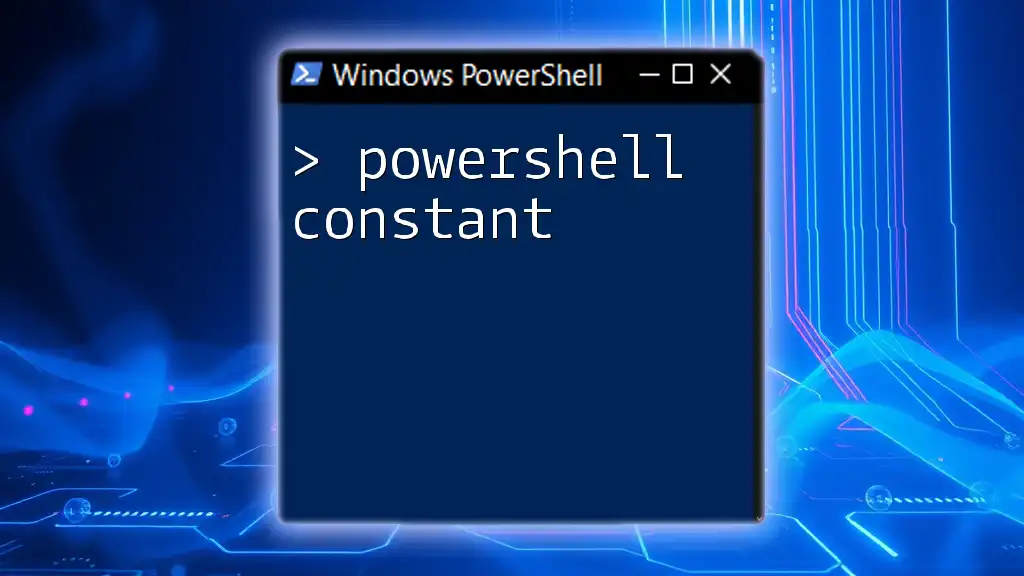
Common Pitfalls to Avoid
Overusing ErrorAction Stop
While `Stop` is a powerful tool, relying on it excessively can lead to frustration. Understand that in some contexts, allowing a script to continue after non-critical errors might be suitable. Assess each situation carefully to decide the appropriate action.
Ignoring Error Messages
One of the largest pitfalls is ignoring the implications of errors. While `SilentlyContinue` might seem convenient, it can lead to debugging nightmares. Instead, embrace error messages as an opportunity to improve your scripts and understand their behavior better.
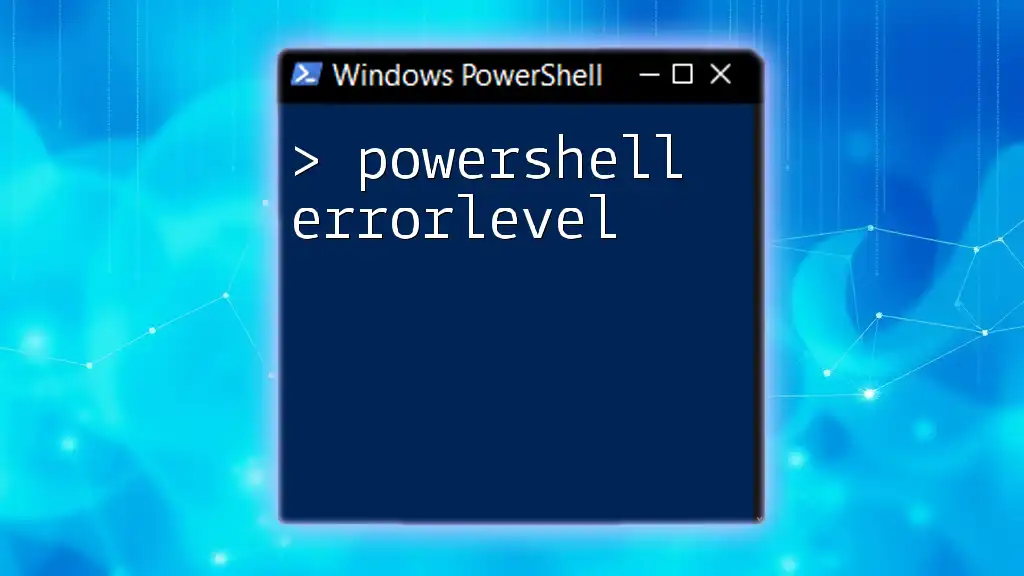
Conclusion
In summary, mastering PowerShell ErrorAction Stop is critical for anyone looking to enhance their scripting skills. By halting execution on errors, you gain control and confidence in your scripts, ensuring your actions produce the intended results. Embrace the practices discussed here, and you will equip yourself with the tools needed for effective PowerShell scripting.
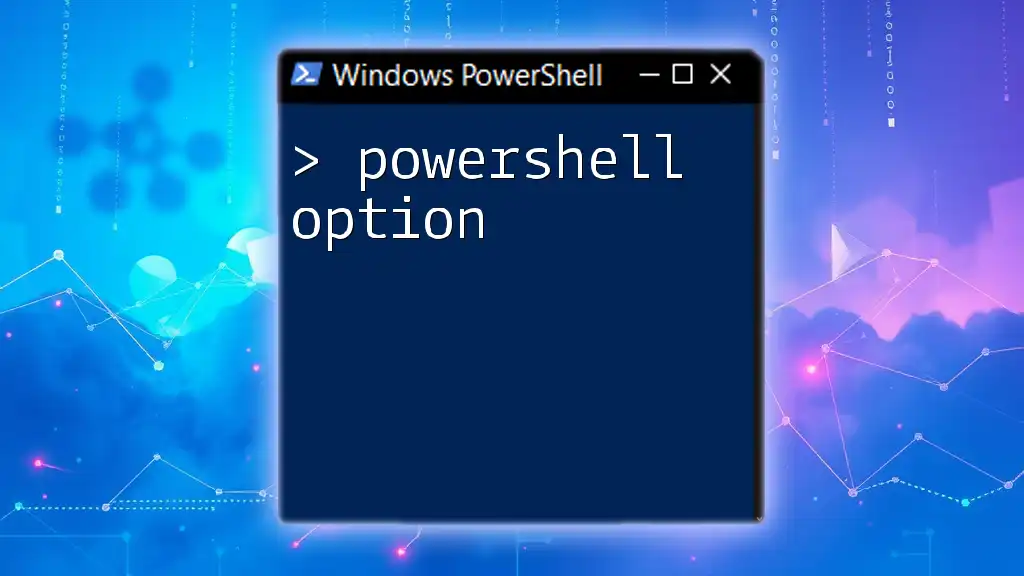
Call to Action
Consider subscribing for more insights into PowerShell and sharing your experiences with `ErrorAction Stop`. Engage in a community of learners dedicated to mastering PowerShell commands efficiently!