The `LastIndexOf` method in PowerShell is used to find the position of the last occurrence of a specified substring within a string, returning -1 if the substring is not found.
Here’s a code snippet demonstrating its usage:
$myString = "Hello, World! Welcome to the World of PowerShell."
$lastIndex = $myString.LastIndexOf("World")
Write-Host "The last index of 'World' is: $lastIndex"
Understanding String Methods in PowerShell
What are String Methods?
String methods are built-in functions in PowerShell designed to manipulate and interact with text data. These methods allow you to perform various operations such as searching, replacing, and formatting strings easily. Common string manipulations include concatenation, substring extraction, and searches for specific content within a string.
Role of `LastIndexOf` in String Manipulation
The `LastIndexOf` method specifically locates the last occurrence of a specified substring within a string. This method is valuable when you need to retrieve the position of the last match, which can be especially useful for tasks such as parsing data or analyzing text files where context matters.

Syntax of the `LastIndexOf` Method
Basic Syntax
The basic syntax of the `LastIndexOf` method in PowerShell is as follows:
[int] LastIndexOf(string $value, [int] $startIndex=-1, [int] $count=-1, [CultureInfo] $culture=null)
This function takes multiple parameters that allow you to customize the search behavior.
Parameters Explained
- $value: This parameter represents the substring you want to locate within the source string.
- $startIndex: It is the index to start searching from. By default, it is set to -1, which means you search the entire string.
- $count: This number determines how many characters to examine during the search. The default value of -1 means it will search the entire string.
- $culture: This optional parameter allows you to specify culture-specific rules for string comparison, enabling local sensitivity in matching strings.
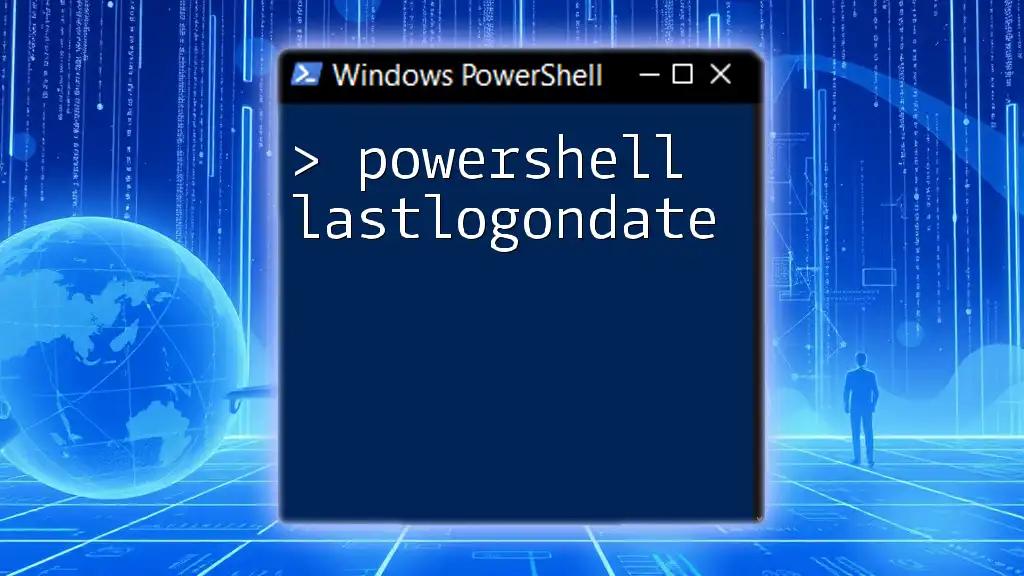
How to Use `LastIndexOf` in PowerShell
Basic Example
Using `LastIndexOf` is straightforward. Here’s a simple example to illustrate its usage:
$string = "PowerShell is powerful, and PowerShell is versatile."
$lastIndexOfPowerShell = $string.LastIndexOf("PowerShell")
In this example, `$lastIndexOfPowerShell` will contain the index of the last occurrence of the substring "PowerShell". The result will be 37, as it appears last in the string.
Finding Occurrences from a Specific Index
You can also control the search starting point by using the `$startIndex` parameter. This is particularly useful when you want to exclude certain parts of the string from the search. For example:
$lastIndexPowerShellFrom15 = $string.LastIndexOf("PowerShell", 15)
In this case, the search will only consider the substring up to index 15. The value returned will be -1, indicating that "PowerShell" does not appear within the specified range.
Limiting the Search with Count
The `$count` parameter allows you to limit the number of characters that `LastIndexOf` examines. This can be highly useful in situations where you want to limit the scope of your search:
$lastIndexLimited = $string.LastIndexOf("PowerShell", 40, 20)
This snippet will search for "PowerShell" starting from index 40 and examining only 20 characters. Depending on the string's content, it helps to pinpoint occurrences even more precisely.
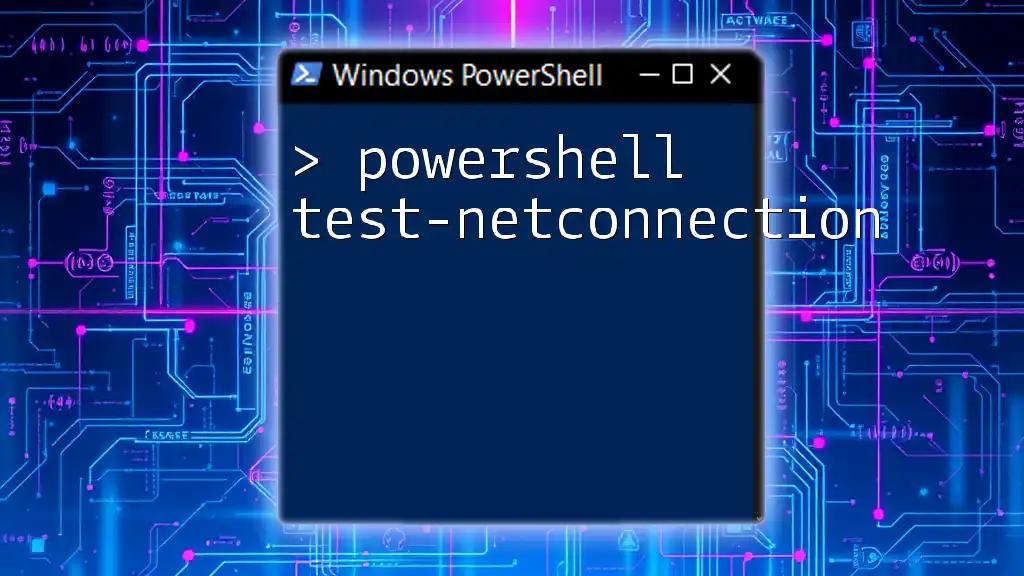
Handling NotFound Cases
Interpreting Return Values
When you use `LastIndexOf`, it’s important to understand its return values. If the specified substring is not found, the method returns -1. To effectively manage these situations in your scripts, you can implement a conditional structure to handle these scenarios gracefully:
if ($lastIndexOfPowerShell -eq -1) {
"Substring not found."
}
This check ensures that your script can handle cases where the substring does not exist without causing errors or unexpected behavior.
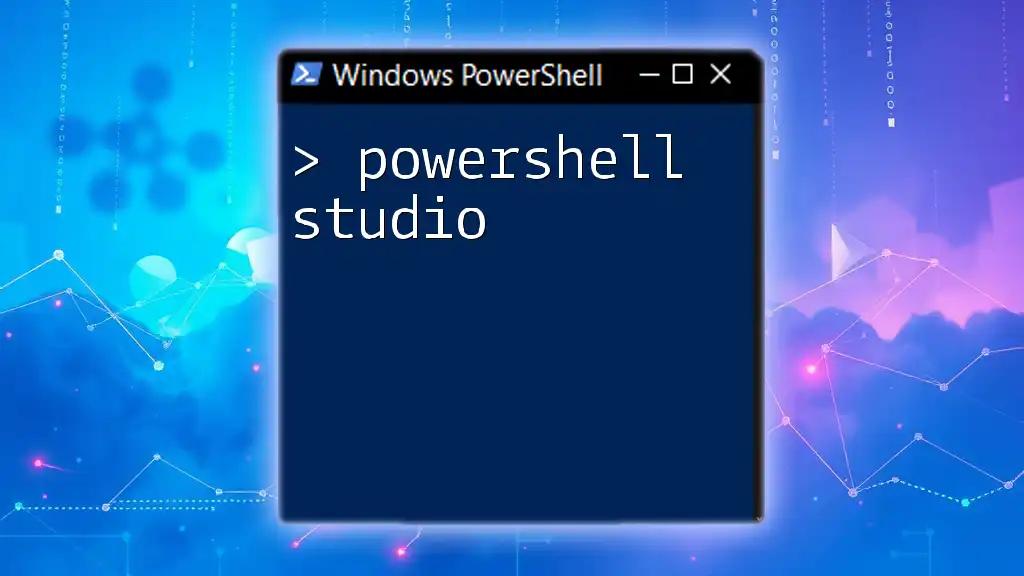
Practical Applications of `LastIndexOf`
Data Parsing Scenarios
`LastIndexOf` is particularly useful in data parsing scenarios, especially when working with file paths, configuration files, or log files. For example, suppose you need to extract the file extension from a path:
$filePath = "C:\Documents\reports\sales_report_2023.xlsx"
$lastDotIndex = $filePath.LastIndexOf(".")
$fileExtension = $filePath.Substring($lastDotIndex)
In this code, `LastIndexOf` finds the position of the last period (.) in the file path, allowing you to extract the extension correctly.
User Input Validation
You can also utilize `LastIndexOf` to validate user input effectively. If you want to ensure that users provide input containing a specific term, you can do something like this:
$inputString = Read-Host "Enter a string to search"
$lastIndexCheck = $inputString.LastIndexOf("check")
if ($lastIndexCheck -ne -1) {
"The substring 'check' was found at index $lastIndexCheck."
} else {
"The substring 'check' was not found."
}
This code snippet checks if the term "check" is present in the user’s input, helping you provide immediate feedback.

Comparisons with Other Methods
`IndexOf` vs. `LastIndexOf`
While both `IndexOf` and `LastIndexOf` are used to find substrings, they have distinct differences. `IndexOf` finds the first occurrence of a substring, which can be useful for the initial match detection, while `LastIndexOf` targets the last occurrence, making it ideal for scenarios where the string may contain multiple instances.
Use cases for each method:
- Use `IndexOf` when you only need the first match's position, for instance, validating that a warning message has been logged.
- Use `LastIndexOf` when you need the context of the last match, such as parsing the last component of a file path or checking when an event last occurred.
When to Use Each Method
Understanding the context is crucial when choosing between `IndexOf` and `LastIndexOf`. Always ask:
- Do I need the first position or the last?
- What is the surrounding content I need to account for?
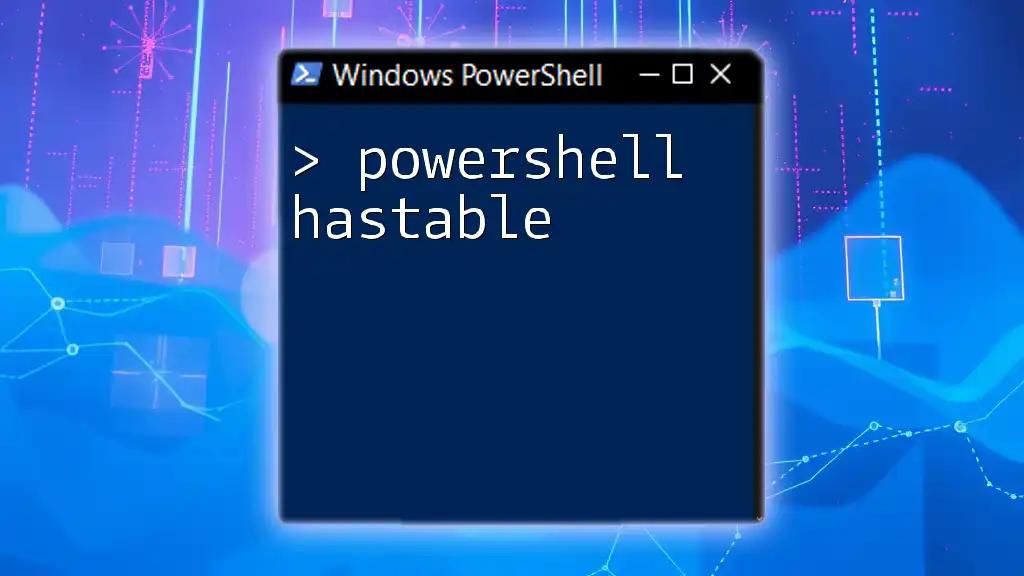
Conclusion
Recap of Key Points
To sum up, the `PowerShell LastIndexOf` method is a powerful tool for string manipulation in PowerShell. It allows developers to find the position of the last occurrence of a substring swiftly. Understanding the syntax, parameters, and practical applications help harness its full potential effectively.
Call to Action
Try implementing `LastIndexOf` in your own PowerShell scripts to enhance your text processing capabilities. Don’t hesitate to share your experiences and any use cases that might add value to the discussion. Join our community for more tutorials and guides on mastering PowerShell commands!
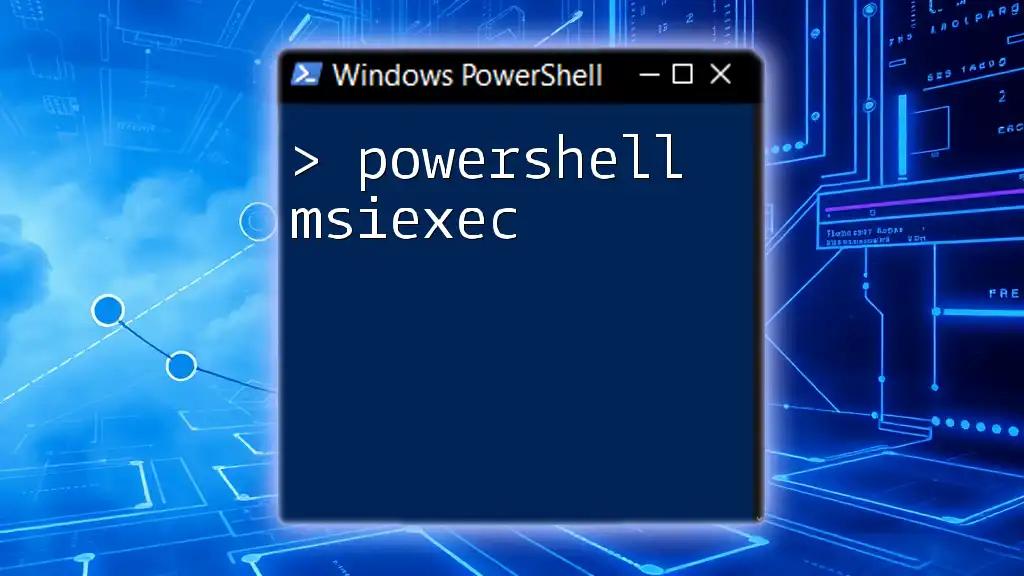
Additional Resources
For more in-depth learning, consider exploring the official PowerShell documentation on string methods and other advanced string manipulations. Books and online courses on PowerShell can also provide insights into best practices and more efficient scripting techniques.

Code Snippets Repository
Feel free to check our GitHub repository for a collection of example scripts showcasing the use of `LastIndexOf` and other useful PowerShell commands!