The `IndexOf` method in PowerShell is used to return the zero-based index of the first occurrence of a specified string within another string, or -1 if the string is not found.
Here’s a code snippet demonstrating its usage:
$myString = "Hello, World!"
$index = $myString.IndexOf("World")
Write-Host "The index of 'World' is: $index"
Understanding the Basics of Strings in PowerShell
What is a String in PowerShell?
In PowerShell, a string is a sequence of characters enclosed in single or double quotes. Strings can contain letters, numbers, symbols, and whitespace, making them versatile for various programming tasks. Understanding how to manipulate strings is foundational in PowerShell scripting since most data interactions involve strings.
Here's an example of a basic string declaration:
$greeting = "Hello, PowerShell!"
String Methods in PowerShell
PowerShell provides a rich set of string methods that allow you to modify, search, and manipulate strings efficiently. Among these methods, the `IndexOf` method stands out as a crucial tool for locating a substring within a string.
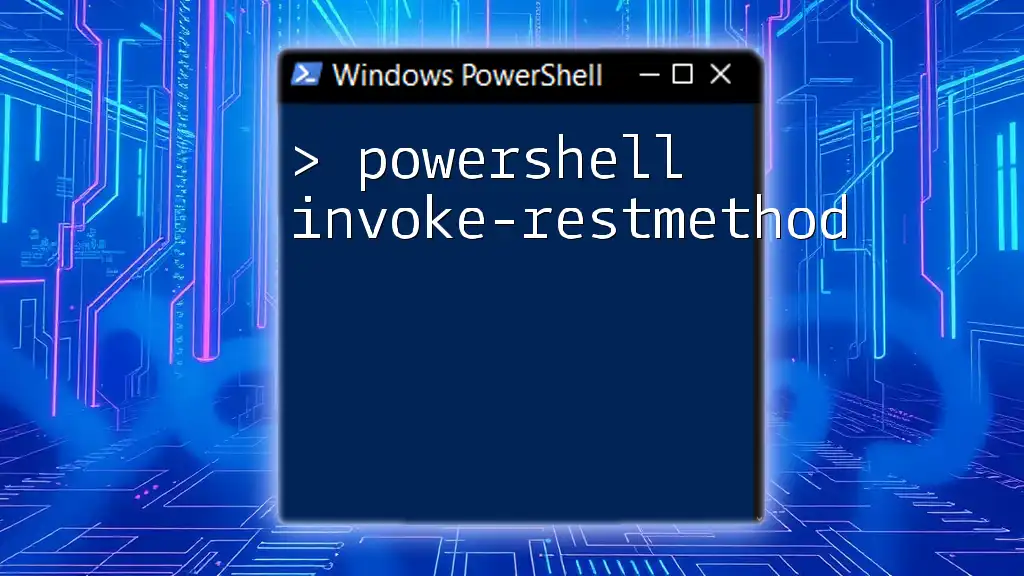
The PowerShell `IndexOf` Method
What is the `IndexOf` Method?
The `IndexOf` method in PowerShell is used to find the position of a specified substring within a string. It returns the zero-based index of the first occurrence of the substring or -1 if the substring is not found. This functionality is essential for various scripting operations, such as data validation and parsing.
Syntax of the `IndexOf` Method
The basic syntax of the `IndexOf` method is straightforward:
$index = $string.IndexOf($substring)
- `$string` is the original string you are searching within.
- `$substring` is the string you are trying to locate.
Return Value of `IndexOf`
The return value of the `IndexOf` method is significant. If the substring is found, you receive its index position; if not, it returns -1. Understanding these return values can help you handle scenarios where the substring may or may not be present.
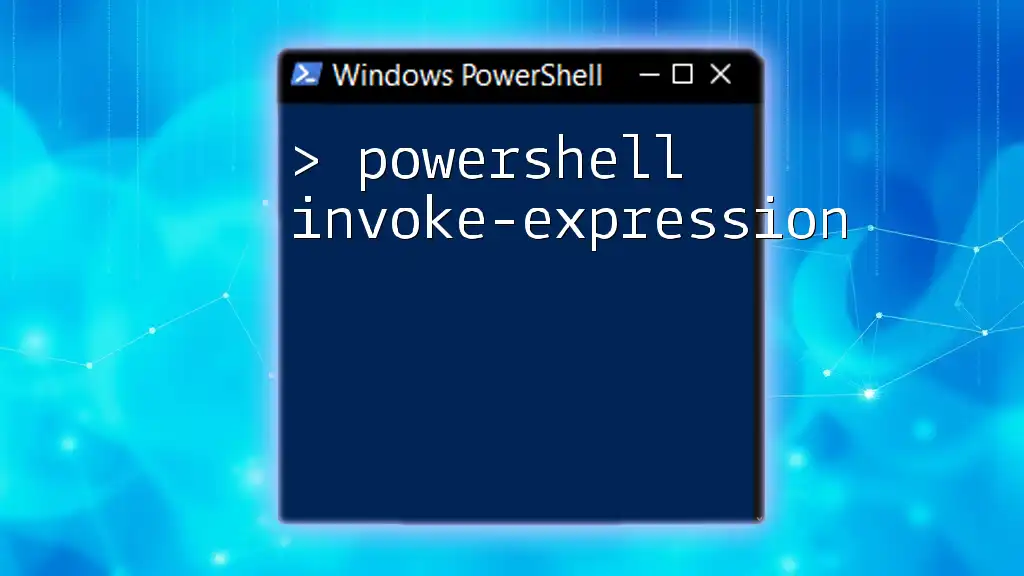
Examples of Using `IndexOf` in PowerShell
Basic Example of `IndexOf`
To demonstrate the `IndexOf` method’s basic functionality, consider this simple code snippet:
$exampleString = "Hello, World!"
$index = $exampleString.IndexOf("World")
Write-Output $index # Output: 7
In this case, the method searches for the substring "World" and finds it starting at index 7. This knowledge allows you to coordinate further actions based on the position of your desired substring.
Case Sensitivity in `IndexOf`
It's crucial to note that PowerShell's `IndexOf` method is case-sensitive by default. This means "world" and "World" are treated as different substrings. For instance, consider this example:
$caseSensitiveExample = "PowerShell is great!"
$indexCaseSensitive = $caseSensitiveExample.IndexOf("powershell")
Write-Output $indexCaseSensitive # Output: -1
Here, the result is -1 because "powershell" (lowercase) is not found in the string, demonstrating how capitalization affects search results.
Using `IndexOf` with Optional Parameters
Exploring Start Index and StringComparison
The `IndexOf` method allows for optional parameters which can customize its behavior. You can provide a start index to begin your search, which is useful when working with repeated occurrences of a substring. Here's a code snippet that illustrates this:
$partialString = "Hello, Hello, Hello!"
$indexFrom = $partialString.IndexOf("Hello", 7)
Write-Output $indexFrom # Output: 13
In this example, the search for "Hello" starts at index 7, leading to the discovery of the substring starting at index 13.
Example of StringComparison
Moreover, you can utilize StringComparison options to specify how the search should be conducted—whether case-sensitive or case-insensitive. Here's an example:
$exampleComparison = "Hello, World!"
$caseInsensitiveIndex = $exampleComparison.IndexOf("WORLD", [System.StringComparison]::CurrentCultureIgnoreCase)
Write-Output $caseInsensitiveIndex # Output: 7
In this case, by indicating CurrentCultureIgnoreCase, the search successfully finds the substring "WORLD" despite the casing, returning the correct index 7.
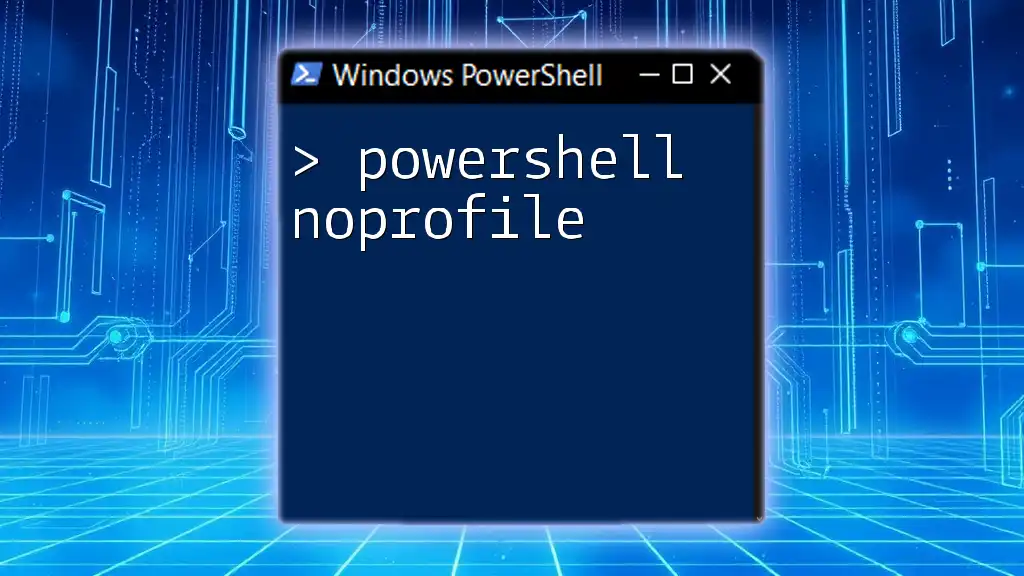
PowerShell Substring and IndexOf
Introduction to PowerShell Substring Method
The Substring method in PowerShell allows you to extract parts of a string based on specified start and length parameters. This can be quite useful when combined with the `IndexOf` method to target specific segments of a string based on the substring's position.
Using `IndexOf` with `Substring`
When you combine `IndexOf` with the `Substring` method, you can extract valuable segments of strings dynamically. For example:
$fullString = "PowerShell is a powerful tool"
$startIndex = $fullString.IndexOf("powerful")
$subString = $fullString.Substring($startIndex, 8)
Write-Output $subString # Output: powerful
In this snippet, after locating "powerful" using `IndexOf`, we then extract it using `Substring`, producing the desired output.
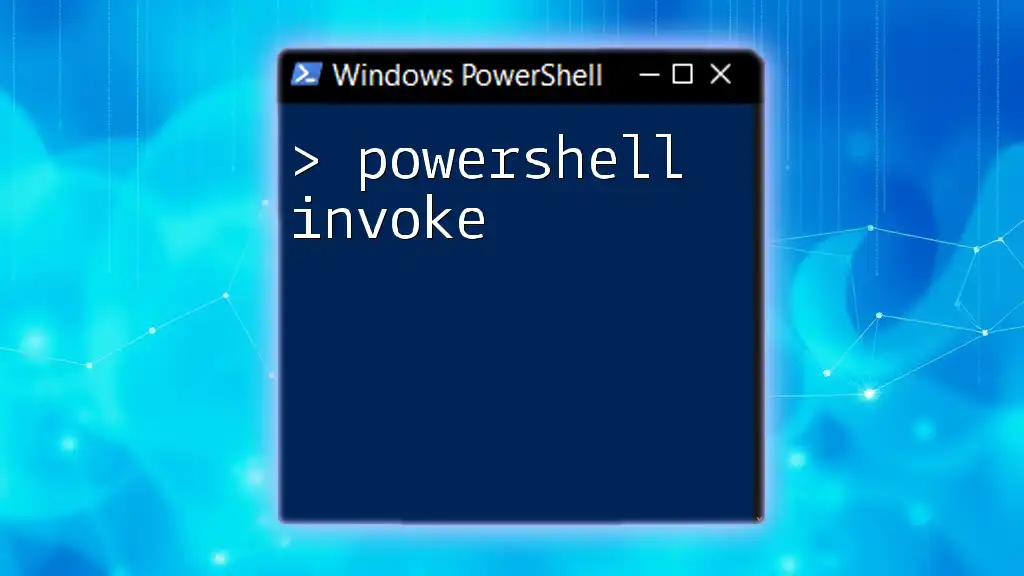
Tips and Best Practices for Using `IndexOf`
Avoiding Common Pitfalls
When using the `IndexOf` method, it's important to remember that not all searches will yield results. Always check for a return value of -1 to avoid unexpected errors in your scripting logic.
Enhancing Performance
For optimal performance, especially when dealing with large strings or many repeated calls to `IndexOf`, consider:
- Minimizing string concatenation, which can require additional memory and processing.
- Caching results of `IndexOf` if you are checking for the same substrings multiple times.
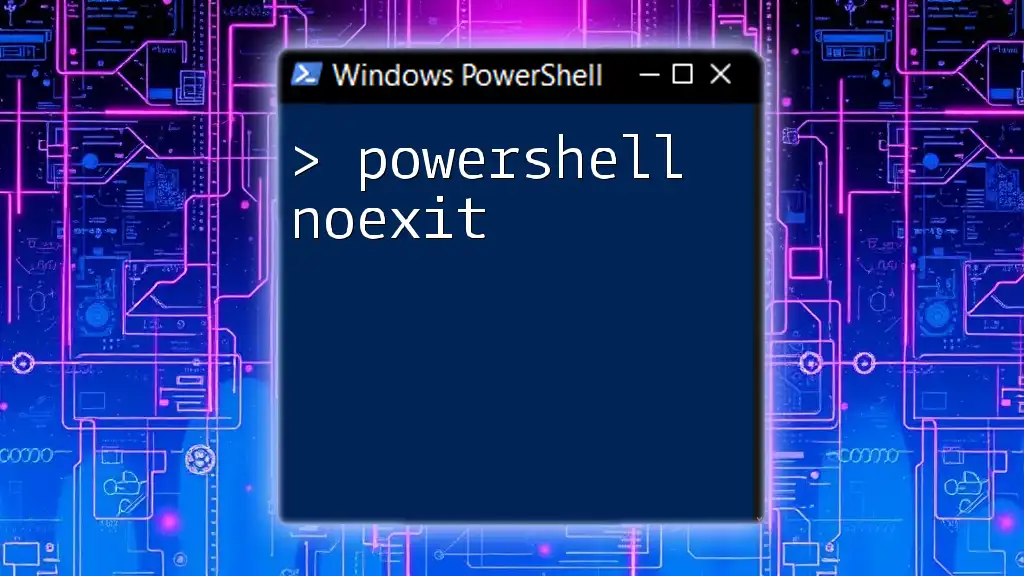
Conclusion
Mastering the `IndexOf` method in PowerShell opens up a world of possibilities for string manipulation. Understanding how to effectively use this method not only enhances your scripting capabilities but also paves the way for more advanced string operations. Practice using `IndexOf` in real-world scenarios to fully grasp its versatility and importance in PowerShell scripting.
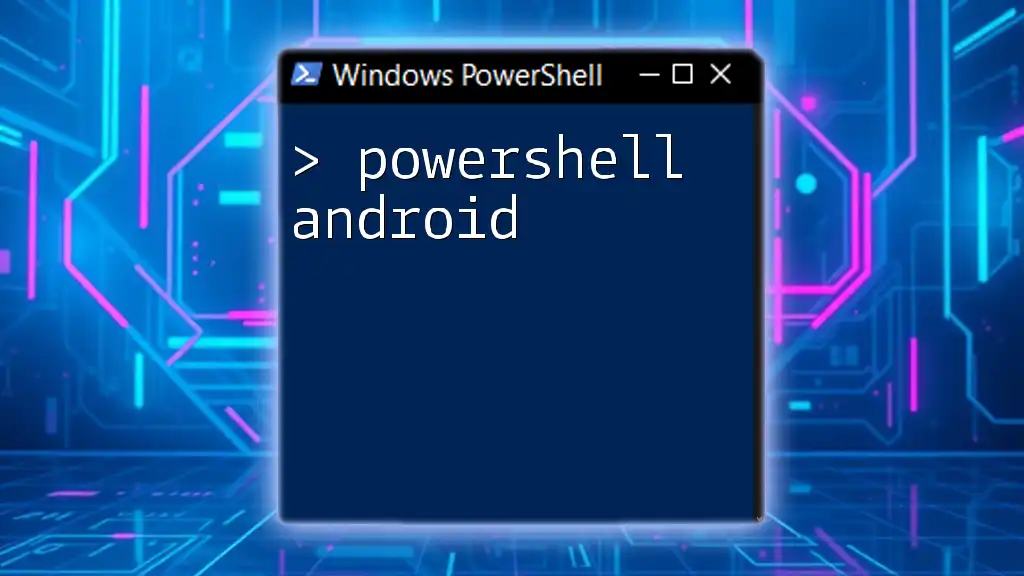
Further Resources
For more in-depth information, consider exploring the official PowerShell documentation or tutorials on string manipulation techniques. These resources can provide additional insights and examples to further enhance your skills.