The `msiexec` command in PowerShell is a utility used to install, modify, or perform operations related to Windows Installer packages (.msi files) directly from the command line.
msiexec /i "C:\Path\To\YourInstaller.msi" /quiet /norestart
Understanding msiexec Command-line Options
What is msiexec.exe?
The msiexec executable is a component of the Windows Installer, responsible for the installation, modification, and uninstallation of Windows Installer packages (.msi files). Its role is critical as it processes the installation instructions embedded in an MSI file. When you invoke msiexec, you can perform operations such as installing new software, repairing existing installations, or removing software from your system.
Common msiexec command-line options
Understanding the command-line options available with msiexec is essential for effective usage. Here are some of the most commonly used options:
-
/i: This option is used to install an MSI package. When specified, it tells msiexec that it should install the program contained within the MSi file.
-
/x: This option uninstalls an MSI package. Like the install function, it manages the removal of previously installed applications.
-
/qn: This flag runs the installation in silent mode with no user interface. This option is particularly useful for deployments requiring automation, eliminating any prompts that would hinder automation scripts.
-
/l: This option enables logging and captures installation details. It is invaluable for troubleshooting installation issues by providing a detailed log of the installation process.
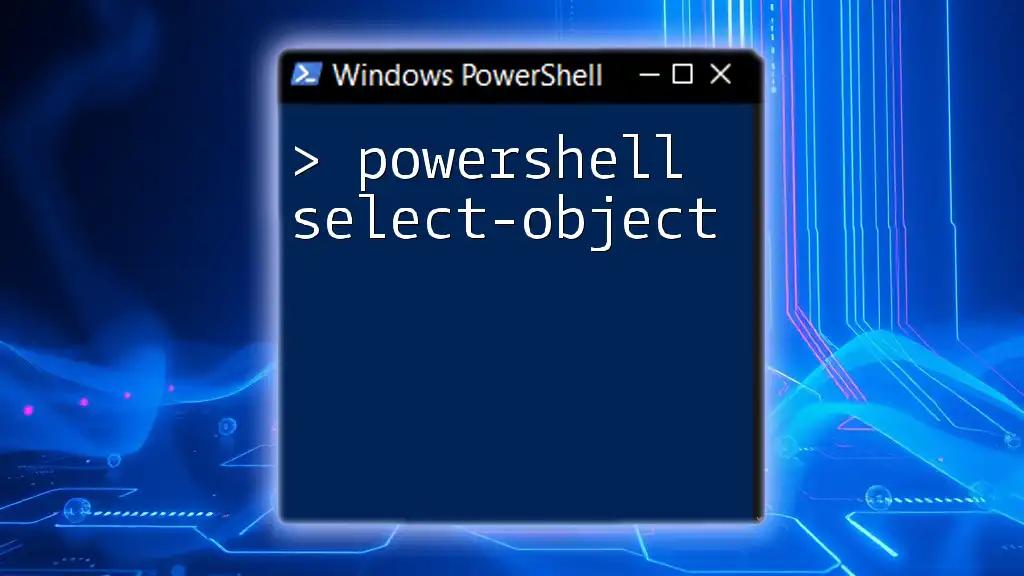
Prerequisites for Using msiexec in PowerShell
Required Permissions
Before running msiexec commands in PowerShell, ensure you have the necessary administrative rights. Many operations that write to the system, such as installations or uninstalls, require elevated permissions to avoid issues and ensure system integrity.
PowerShell Environment Set-Up
To utilize msiexec via PowerShell, you first need to make sure that PowerShell is installed and accessible on your Windows system. You can check this by typing `powershell` into the Windows search bar or running `Get-Host` in your command line. Confirm that you’re operating on a version of PowerShell that is compatible with Windows Installer features.
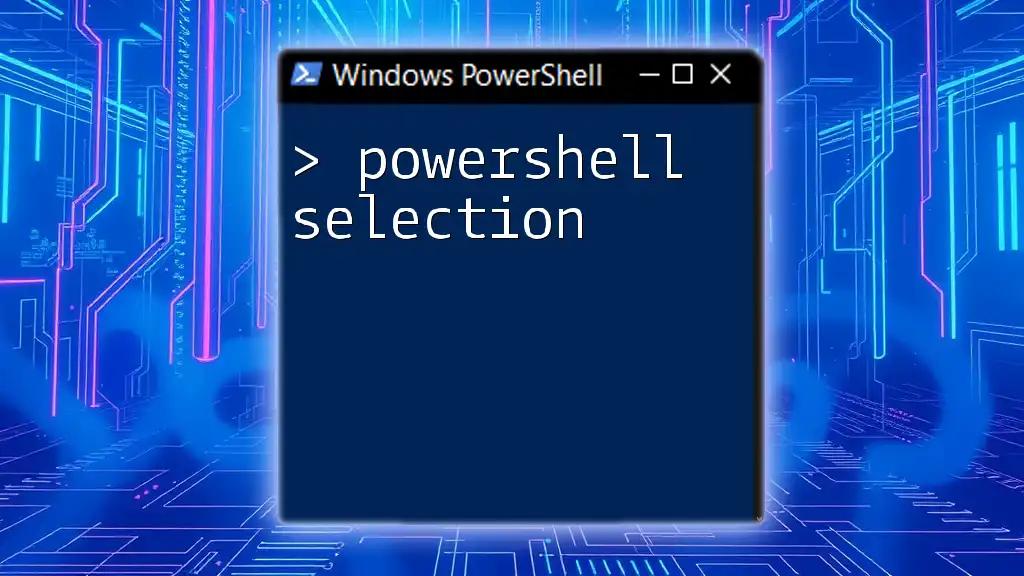
Running msiexec from PowerShell
How to Run an MSI from PowerShell
Running an MSI installer from PowerShell can be accomplished by utilizing the `Start-Process` cmdlet, which starts one or more processes on your computer. Below is a code snippet that demonstrates how to run an MSI using PowerShell:
Start-Process msiexec.exe -ArgumentList '/i', 'path\to\your-file.msi', '/qn' -Wait
In this example:
- Start-Process is the cmdlet used to initiate the installation.
- msiexec.exe specifies the executable for Windows Installer.
- The `-ArgumentList` allows you to pass several options, including:
- `/i` to signify installation.
- `'path\to\your-file.msi'` as the path to the MSI file you want to install.
- `/qn` to perform a silent installation.
- The `-Wait` parameter ensures that the PowerShell script waits for the installation to complete before moving on.
Using PowerShell Run MSI Command
Another straightforward method involves using the `&` operator, which allows you to execute a command. Here's how you can leverage this operator:
& msiexec.exe /i "path\to\your-file.msi" /quiet
In this example:
- The `&` operator is used to explicitly invoke the `msiexec.exe` command.
- `/quiet` is an alias for the `/qn` option, continuing the silent installation without user interaction.
Both methods are effective, and selecting one depends on your scripting style and specific needs.
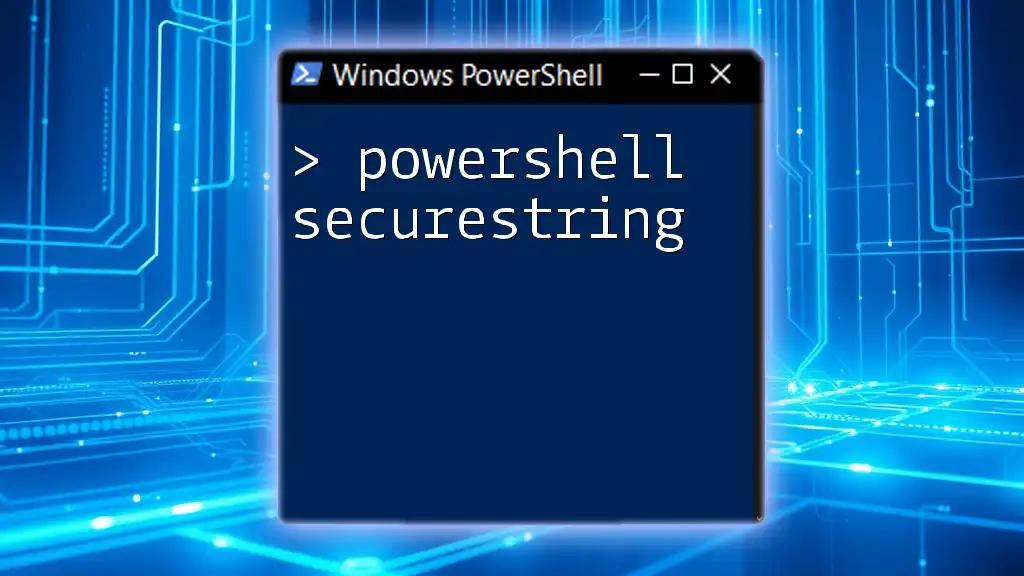
Advanced Features of msiexec in PowerShell
Running MSI Packages with Properties
One powerful aspect of msiexec is the ability to pass properties during installations. This is especially useful for configuring installations based on specific user requirements. Here's a code example that illustrates this:
Start-Process msiexec.exe -ArgumentList '/i', 'path\to\your-file.msi', '/qn', 'PROPERTY_NAME=VALUE' -Wait
Here, `PROPERTY_NAME=VALUE` lets you specify configuration settings for the installation, such as installation directories or feature selections.
Customizing Installation
To customize an installation further, you can combine switches and properties that match your specific deployment scenario. Make sure to consult the documentation of each MSI file, as available properties can vary depending on the application being installed.

Logging and Debugging with msiexec and PowerShell
Enabling Logging for Installations
Enabling logging during installations is crucial for diagnosing issues that may arise during the process. You can enable logging by adding the `/l*` parameter as demonstrated below:
Start-Process msiexec.exe -ArgumentList '/i', 'path\to\your-file.msi', '/l*', 'path\to\log.txt' -Wait
In this code:
- `/l*` enables logging for the installation with in-depth details.
- `path\to\log.txt` specifies where to save the log file, which will contain all installation-related data.
Viewing Log Outputs and Troubleshooting Common Issues
Once you have the logs, skim through them for common error codes and messages, which can help identify problems. Some frequent issues include missing dependencies or incorrect file paths, which can be addressed based on insights gained from the log.
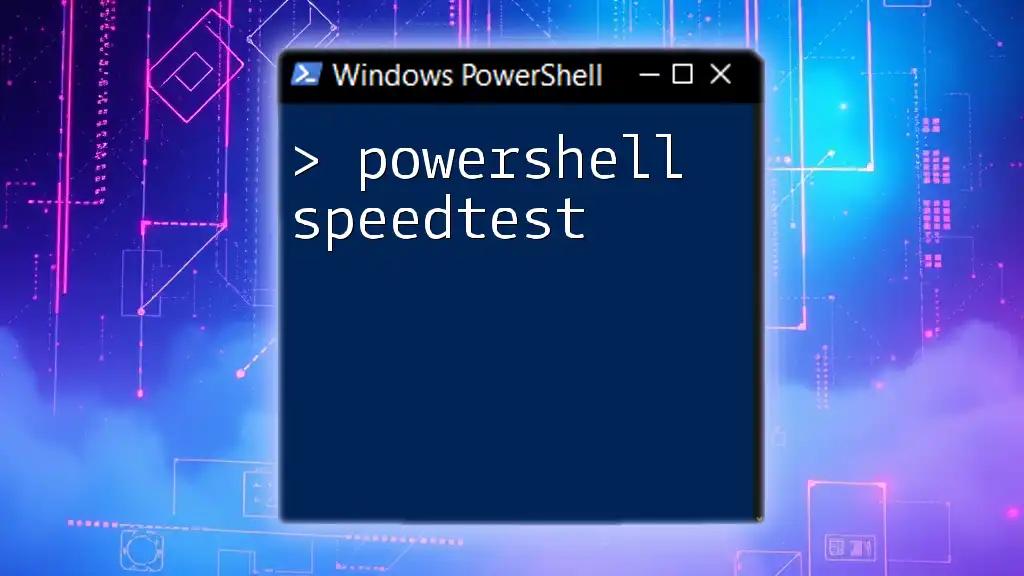
Case Studies: Real-World Scenarios Using PowerShell with msiexec
Automating Software Installation across Multiple Machines
For IT administrators, deploying software across numerous machines can be cumbersome. You can streamline this process using a loop in PowerShell:
foreach ($machine in $machines) {
Invoke-Command -ComputerName $machine -ScriptBlock {
Start-Process msiexec.exe -ArgumentList '/i', 'path\to\your-file.msi', '/qn' -Wait
}
}
In this example:
- The script iterates over a list of machine names stored in the `$machines` variable.
- Invoke-Command runs the installation script remotely on each machine, revolutionizing the deployment process.
Rollback Scenarios: Uninstalling with msiexec
Sometimes, uninstallation is as crucial as installation. Here's how to execute an uninstall command using msiexec:
Start-Process msiexec.exe -ArgumentList '/x', 'GUID_OF_MSI', '/qn' -Wait
In this snippet:
- `/x` signifies that you wish to uninstall an MSI package.
- GUID_OF_MSI represents the unique identifier for the installed MSI package, which facilitates the removal.
You can often retrieve this GUID from the Windows registry or the respective MSI documentation.
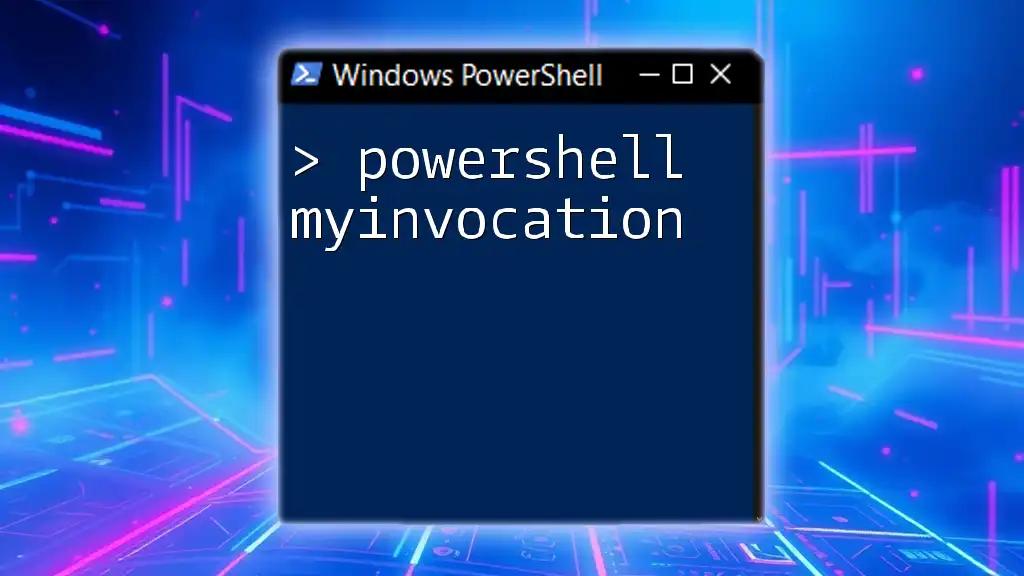
Conclusion
The combination of PowerShell and msiexec provides a robust toolkit for managing Windows installations, configurations, and troubleshooting. By mastering these commands and their nuances, you empower yourself to automate processes, remotely manage software deployments, and diagnose issues effectively.
Consider practicing different commands and configurations to enhance your skills further. Utilizing the advanced capabilities of PowerShell can lead to more efficient IT operations and better overall system management.
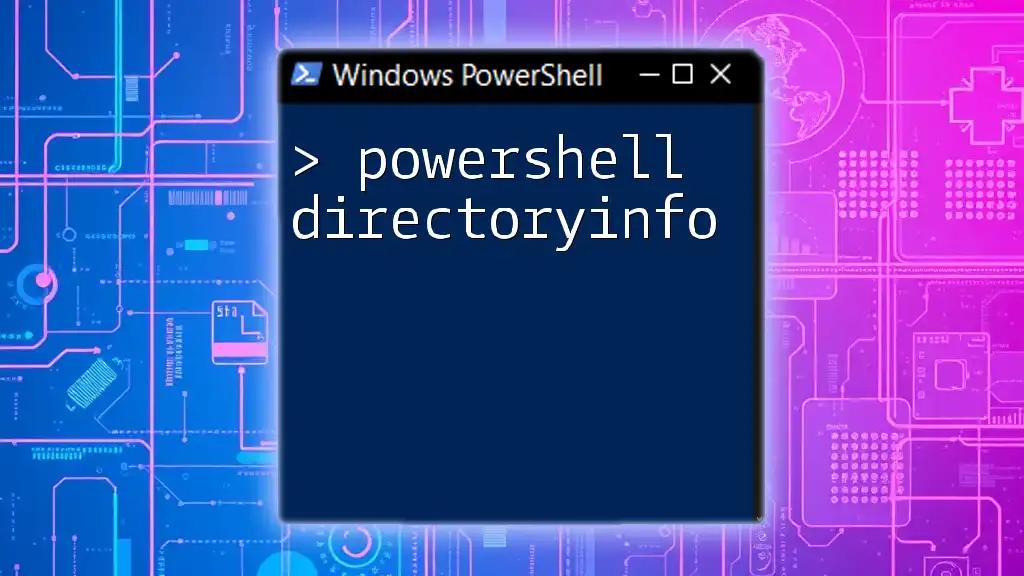
Additional Resources
For further learning, refer to the official documentation for both PowerShell and msiexec. These resources will deepen your understanding and provide up-to-date practices for managing software installations smoothly.

FAQs about PowerShell and msiexec
-
What are the benefits of using PowerShell with msiexec? Leveraging PowerShell alongside msiexec enhances automation, allows for bulk operations, and simplifies script management for installations.
-
Can I install an MSI without admin rights? Typically, administrative rights are required to install software via MSI files. However, certain MSI packages may allow installations without elevation, depending on their design.
-
How do I find the GUID of an installed MSI? The GUID can often be found in the Windows Registry under `HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall` where installed applications are listed.
By exploring these facets of PowerShell msiexec, you are on your way to mastering effective software management in a world of complex and varied applications.