The `Select-Object` cmdlet in PowerShell allows you to choose specific columns from an object or pipeline output, enabling you to view only the information you need.
Here’s a code snippet to illustrate how to select specific columns:
Get-Process | Select-Object Name, Id, CPU
Understanding PowerShell Output Objects
PowerShell operates primarily on objects rather than plain text. When you execute a command in PowerShell, it usually returns objects that hold data in a structured way—comprising properties and values. This is crucial when you want to manipulate and extract specific data efficiently. Each object may encapsulate several columns (properties), allowing you to focus on just what you need.
For instance, consider the following command that retrieves a list of processes running on your system:
Get-Process
This command outputs a series of objects, each representing a process, with properties such as `Name`, `Id`, `CPU`, and `WorkingSet`. By understanding these objects, you can efficiently select specific columns for more focused data processing.
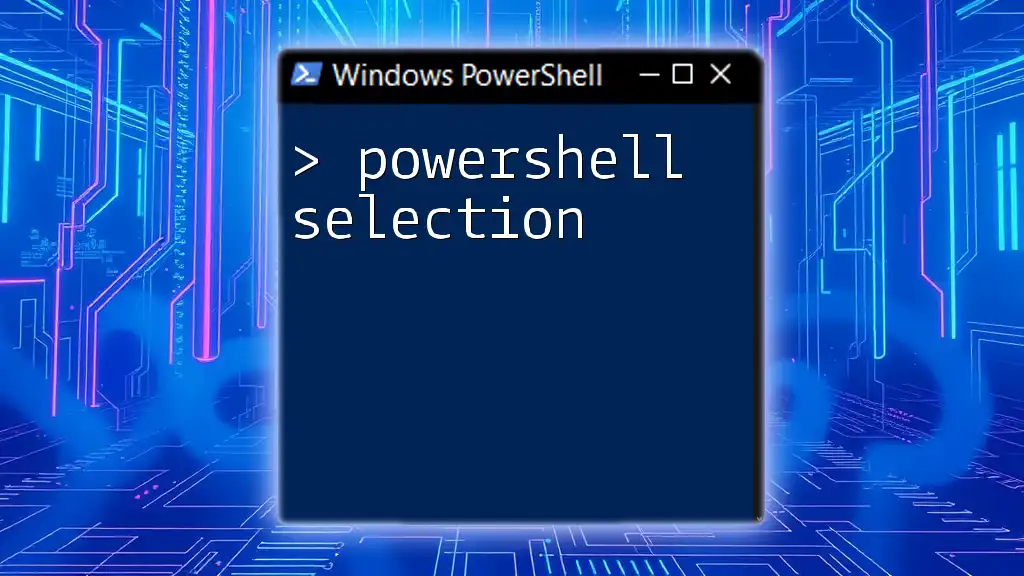
The Select-Object Cmdlet Explained
The `Select-Object` cmdlet is a powerful tool in PowerShell used to pick specific properties from objects. The syntax is straightforward:
Select-Object [-Property] <String[]>
Here, `-Property` indicates the properties (or columns) you want to select. By using this cmdlet, you can streamline your output to include only the relevant data, minimizing clutter and improving readability.
Basic Column Selection
To select specific properties from the output, you can specify them by name. For example, if you want to view just the names and IDs of each running process, you can use:
Get-Process | Select-Object Name, Id
In this command, the output will show only the `Name` and `Id` columns, allowing you to focus on those specific details without any unnecessary information.
Selecting Columns with Expressions
The flexibility of PowerShell becomes even more apparent when you use calculated properties. You can select properties and rename them on the fly. For instance, if you wish to change the `Id` property to `ProcessID`, you can do so with a calculated property syntax:
Get-Process | Select-Object Name, @{Name='ProcessID'; Expression={$_.Id}}
In this example, you create a new column `ProcessID` while retaining the `Name`, thus enhancing data readability.
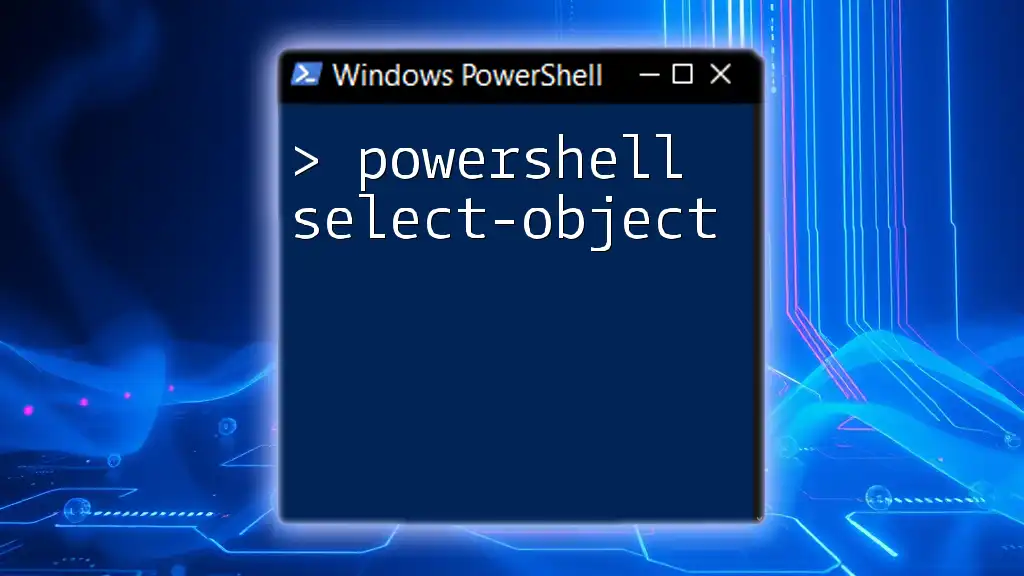
Using the Pipeline with Select-Object
One of PowerShell’s strengths lies in its pipeline capabilities, allowing you to chain commands smoothly. The `Select-Object` cmdlet works effectively within this pipeline. For example, if you want to filter running services and show only their names and display names, you could run:
Get-Service | Where-Object Status -eq 'Running' | Select-Object Name, DisplayName
In this command, you first filter the services based on their status (`Running`) and then select only the `Name` and `DisplayName` properties. This powerful combination ensures your output is both relevant and concise.
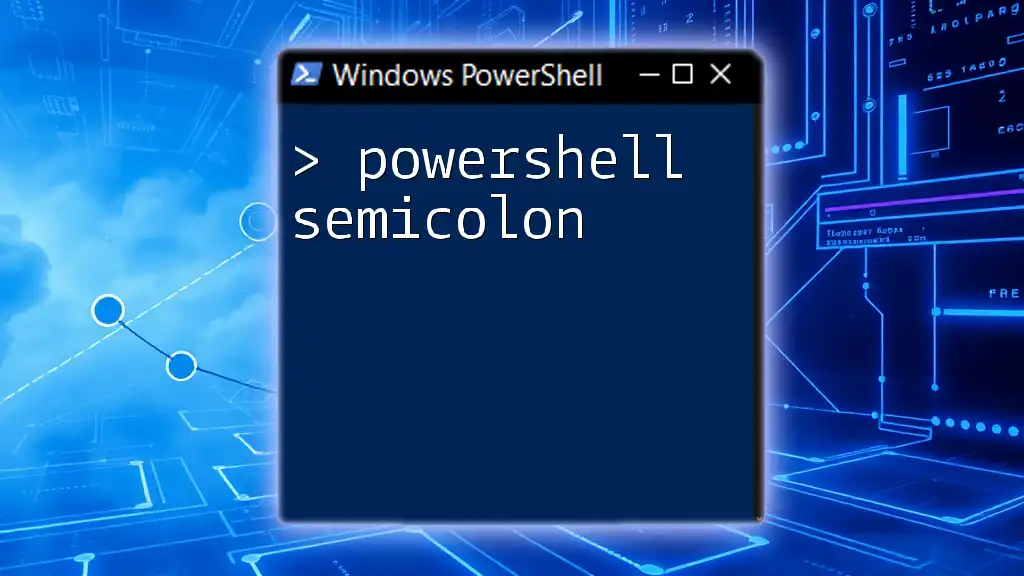
Filtering Columns with Where-Object
Sometimes, you may need to filter out data before selecting the relevant columns. The `Where-Object` cmdlet can help refine your dataset by applying conditions. For example, to get processes that consume more than 100 CPU cycles and select their names and CPU usage, the command would look like this:
Get-Process | Where-Object CPU -gt 100 | Select-Object Name, CPU
With this command, only the processes consuming over 100 CPU cycles are selected, which you can then analyze without distraction from less relevant data.
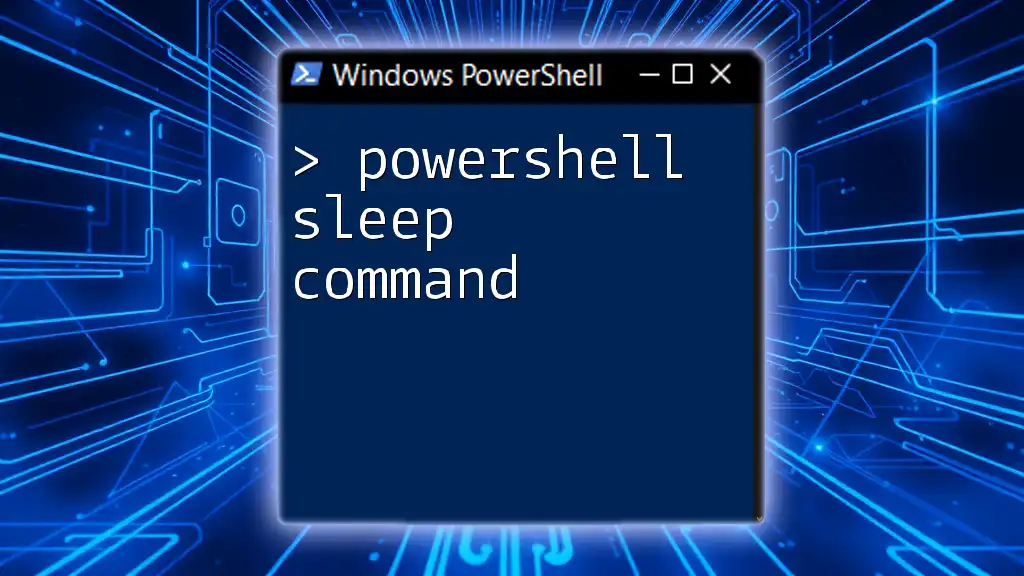
Exporting Selected Columns
PowerShell makes it easy to export selected columns for reporting or further analysis. For example, you can choose specific processes and save their names and IDs into a CSV file as follows:
Get-Process | Select-Object Name, Id | Export-Csv -Path 'processes.csv' -NoTypeInformation
This command creates a CSV file named `processes.csv`, containing just the selected information. Exporting data this way is invaluable for documentation and analysis outside of your PowerShell environment.
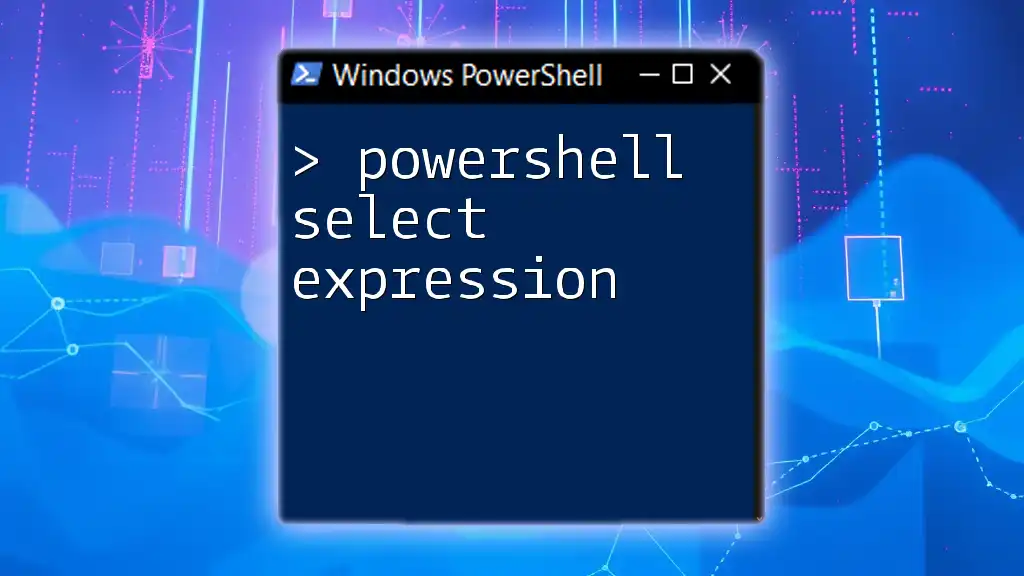
Advanced Usage of Select-Object
To maximize the utility of `Select-Object`, consider leveraging its additional parameters like `-Last`, `-First`, `-Skip`, and `-Unique`. These parameters allow you to control the number of objects being returned or manipulate the output further. For example, to display the last five processes in your list, utilize:
Get-Process | Select-Object -Last 5
This enables you to quickly view the tail end of your data without needing to scroll through the entire output.
Working with Arrays and Collections
In addition to standard commands, you can also use `Select-Object` with arrays and collections. For instance, if you have a collection of custom objects, you can select properties with ease:
$customObjectArray = @(
[PSCustomObject]@{ Name="Alpha"; Value=1 },
[PSCustomObject]@{ Name="Bravo"; Value=2 },
[PSCustomObject]@{ Name="Charlie"; Value=3 }
)
$customObjectArray | Select-Object Name
This command selects and displays only the `Name` property from each custom object, demonstrating the cmdlet's versatility across various data types.
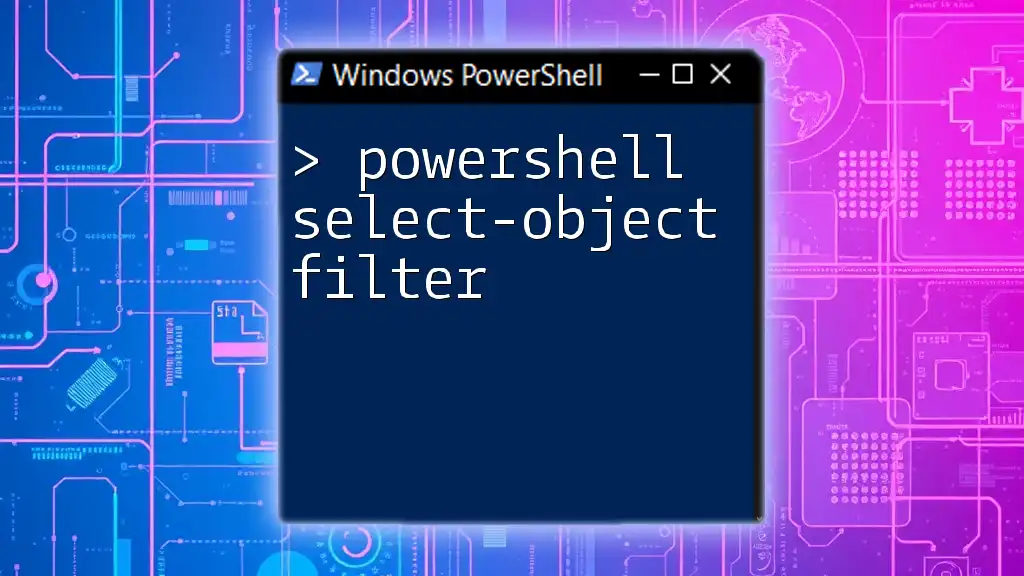
Best Practices for Selecting Columns
When working with `Select-Object`, consider a few best practices to enhance your productivity:
- Be Specific: Only select the columns you need, which boosts performance and clarity.
- Use Calculated Properties Wisely: Utilize them to enhance your output by renaming or applying transformations.
- Combine with Filtering: Always consider using `Where-Object` to refine your dataset before selection to improve output relevance.
Avoid common pitfalls, such as selecting too many columns or forgetting to apply filters, as these can make your results cumbersome and less effective.
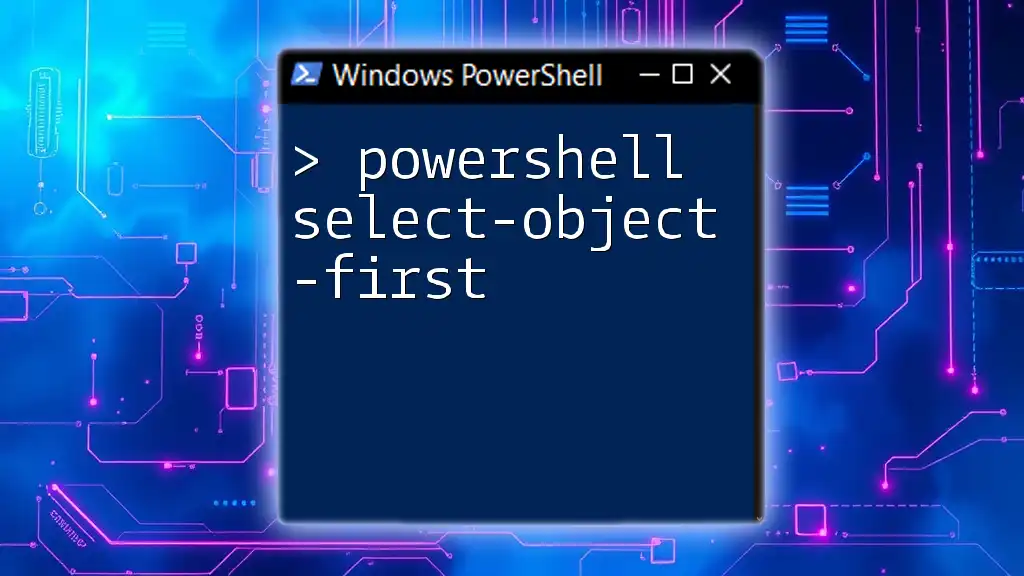
Conclusion
In summary, mastering the `Select-Object` cmdlet in PowerShell is essential for any user interested in managing data efficiently. By understanding how to select specific columns, manipulate output with calculated properties, and effectively use pipelines, you'll elevate your PowerShell skills significantly. Practicing these techniques will not only enhance your scripts but also make your data handling more streamlined and effective.
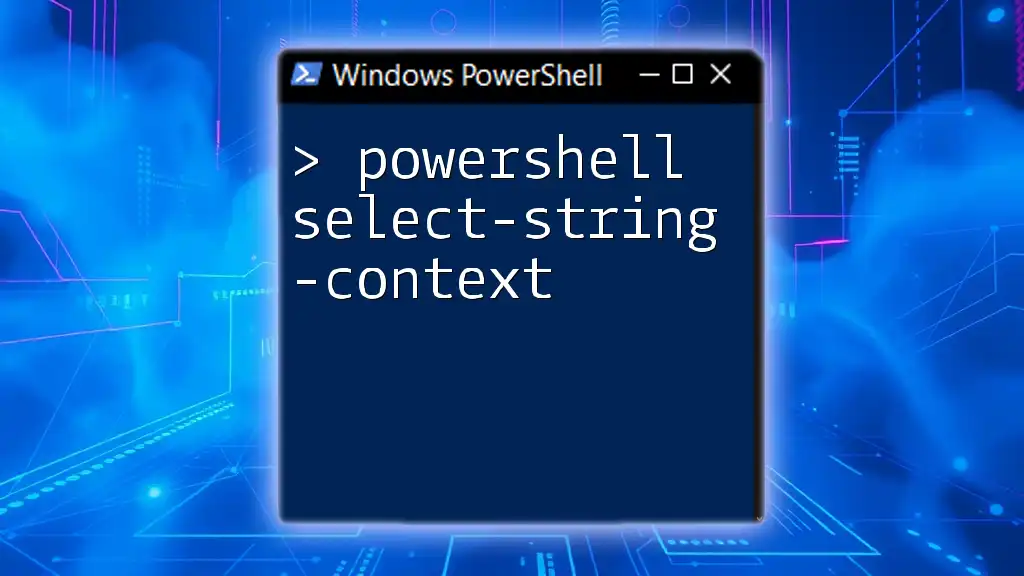
Additional Resources
For those looking to delve deeper into PowerShell, consider exploring the official PowerShell documentation and other tutorials that can provide further insights and advanced techniques.