In PowerShell, the `-contains` operator checks whether a collection contains a specific object, allowing for easy membership testing.
$array = 1, 2, 3, 4, 5
if ($array -contains 3) {
Write-Host 'The array contains the number 3!'
}
Understanding PowerShell Objects
Definition of Objects
In PowerShell, objects are instances of classes that encapsulate data and functionality. They can represent a variety of items within the system, such as processes, files, services, and more. For example, when you retrieve a list of running services, the output is an array of service objects, each containing properties like `Name`, `Status`, and `DisplayName`.
Properties and Methods of Objects
Objects in PowerShell have two main attributes:
-
Properties: These store data about the object. For instance, a file object has properties such as `FullName`, `Length`, and `CreationTime`.
-
Methods: These are functions that allow you to manipulate the object or perform actions. A file object has methods like `CopyTo`, `MoveTo`, and `Delete`.
Here’s an example of creating an object and accessing its properties:
$fileObject = Get-Item "C:\example.txt"
Write-Host "The file name is: $($fileObject.Name)"
Write-Host "The file size is: $($fileObject.Length) bytes"
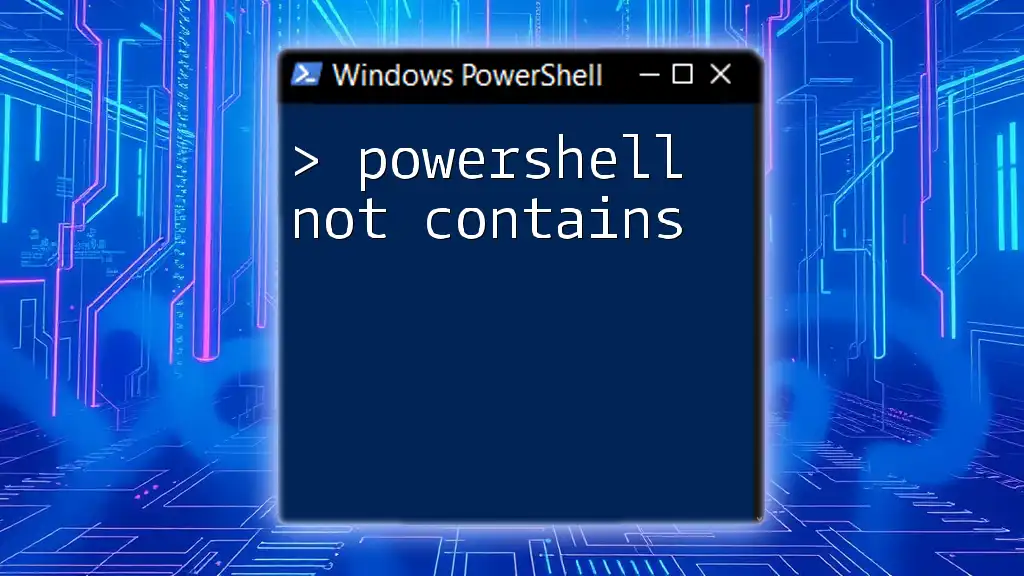
The "Contains" Concept in PowerShell
What Does "Contains" Mean?
The term "contains" in PowerShell can refer to different functionalities depending on the context. It often relates to checking if an object includes specific values or attributes.
For example, you may want to see if an array contains a specific element, if a string includes a substring, or whether a hash table has a specific key or value.
Using `Contains` in PowerShell
Working with Arrays
The `-contains` operator is used to check if an array contains a specific value. It is case-insensitive and can handle any data type.
Here’s an example:
$array = @('apple', 'banana', 'cherry')
if ($array -contains 'banana') {
Write-Host "The array contains banana."
} else {
Write-Host "The array does not contain banana."
}
In this example, the output will confirm that the array does indeed contain "banana."
Working with Strings
For strings, the comparisons are typically performed using the `-like` or `-match` operators. The `-like` operator checks for a wildcard pattern match, while `-match` uses regular expressions.
For example, using `-like`:
$string = "Hello, PowerShell!"
if ($string -like "*PowerShell*") {
Write-Host "The string contains 'PowerShell'."
}
Alternatively, using `-match`:
if ($string -match "PowerShell") {
Write-Host "The string contains 'PowerShell' (matched with regex)."
}
Working with Hash Tables
To determine if a hash table contains a specific key or value, you can check directly for keys using the `ContainsKey` method or `.Keys` property.
Example code snippet:
$hashTable = @{ "Name"="John"; "Age"=30; "City"="New York" }
if ($hashTable.ContainsKey("Age")) {
Write-Host "The hash table contains the key 'Age'."
}
This method is particularly useful for configuration scripts, ensuring that necessary parameters are defined.
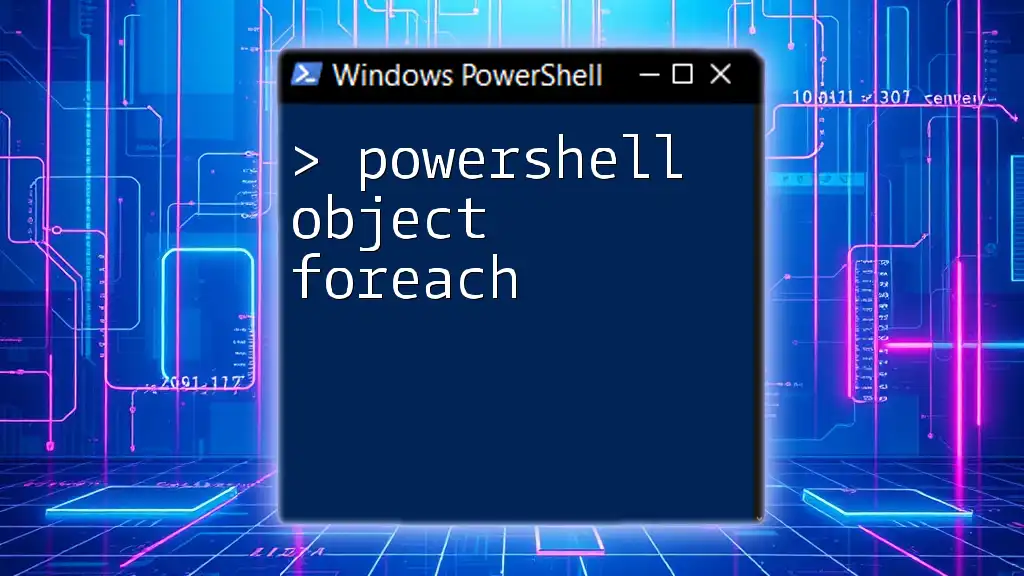
Practical Examples
Example 1: Checking for Membership in a List
When managing user access, you may want to ensure that a user exists in an approved list.
$approvedUsers = @('Alice', 'Bob', 'Charlie')
if ('Bob' -in $approvedUsers) {
Write-Host "'Bob' is an approved user."
} else {
Write-Host "'Bob' is not an approved user."
}
This provides a simple membership check.
Example 2: Searching Within a String
If you're checking logs or output for specific keywords, you could use:
$logEntry = "Error: Disk space critically low on server."
if ($logEntry -like "*Disk space critically low*") {
Write-Host "The log contains an important alert."
}
This alerts you to significant errors or warnings in system logs.
Example 3: Validation in Hash Tables
If you're setting up configurations:
$config = @{
"Server" = "MainServer"
"Timeout" = 30
}
if ($config.ContainsKey("Timeout")) {
Write-Host "Timeout is set in the configuration."
}
Validating the existence of keys in a configuration hash table ensures your script functions correctly.
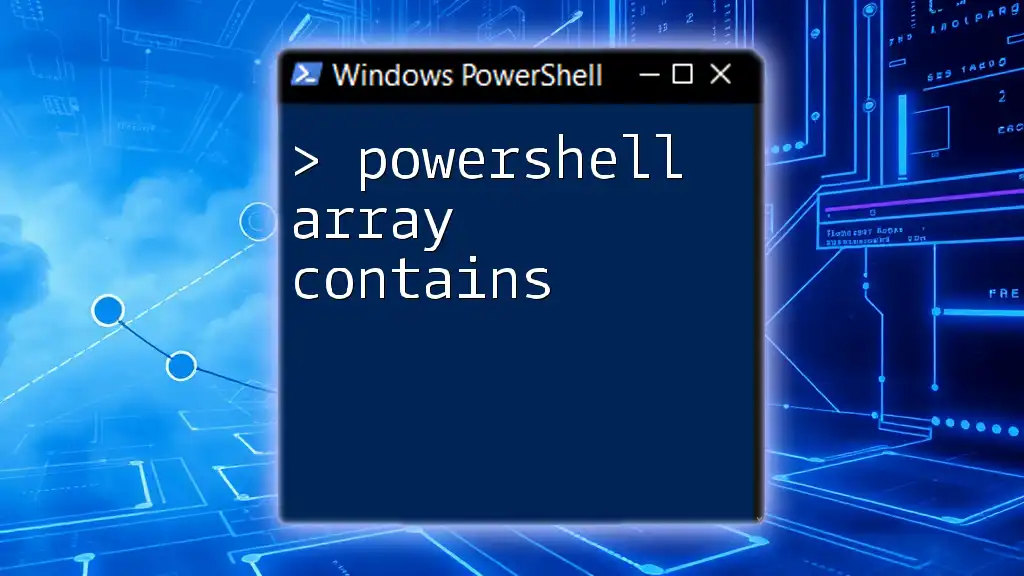
Advanced Techniques
Using `Where-Object` for Complex Queries
You can filter objects in collections based on multiple criteria using the `Where-Object` cmdlet combined with the `-contains`, `-like`, or `-match` operators.
Example of filtering processes based on a name match:
$processes = Get-Process
$filteredProcesses = $processes | Where-Object { $_.Name -like "*Power*" }
foreach ($process in $filteredProcesses) {
Write-Host "Found Power-related process: $($process.Name)"
}
This allows for more dynamic and complex queries based on specific needs.
Utilizing LINQ-like Queries with PowerShell
Although PowerShell is not inherently LINQ, you can achieve similar behavior using `Select-Object` with filtering. For example:
$services = Get-Service
$runningServices = $services | Where-Object { $_.Status -eq 'Running' } | Select-Object Name, DisplayName
$runningServices
This captures active services, mimicking the concept of querying in LINQ.
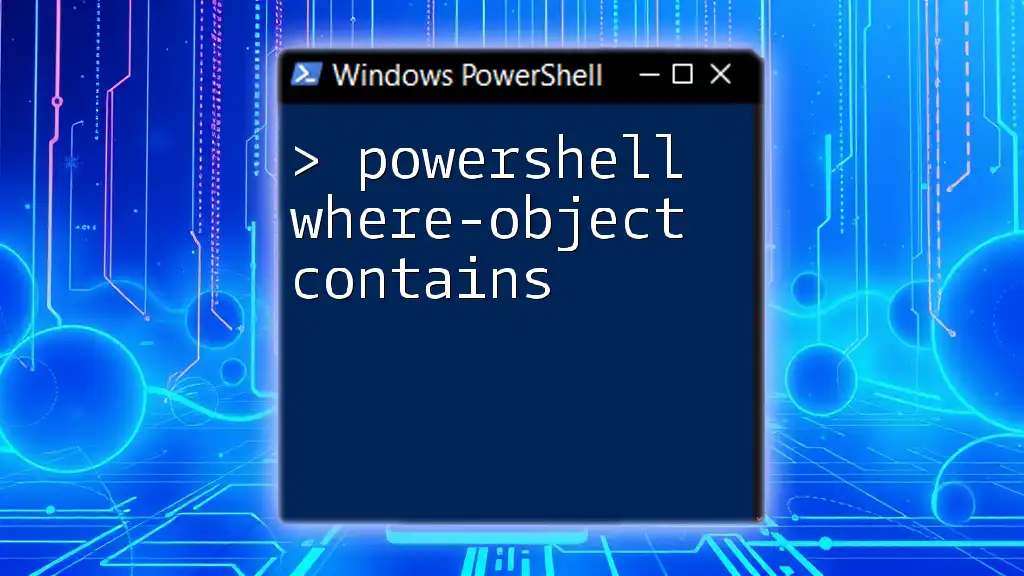
Tips for Effective Use
Best Practices for Checking Object Contents
Understanding when to use `-contains`, `-like`, `-match`, or other methods is crucial. Remember that:
- Use `-contains` for arrays.
- Use `-like` for wildcard searches in strings.
- Use `-match` for regular expression matching.
Common Pitfalls
Common mistakes include:
- Confusing `-eq` and `-contains` when working with collections.
- Forgetting case sensitivity differences in string comparisons.
Correctly identifying these issues will enhance your PowerShell scripting effectiveness.
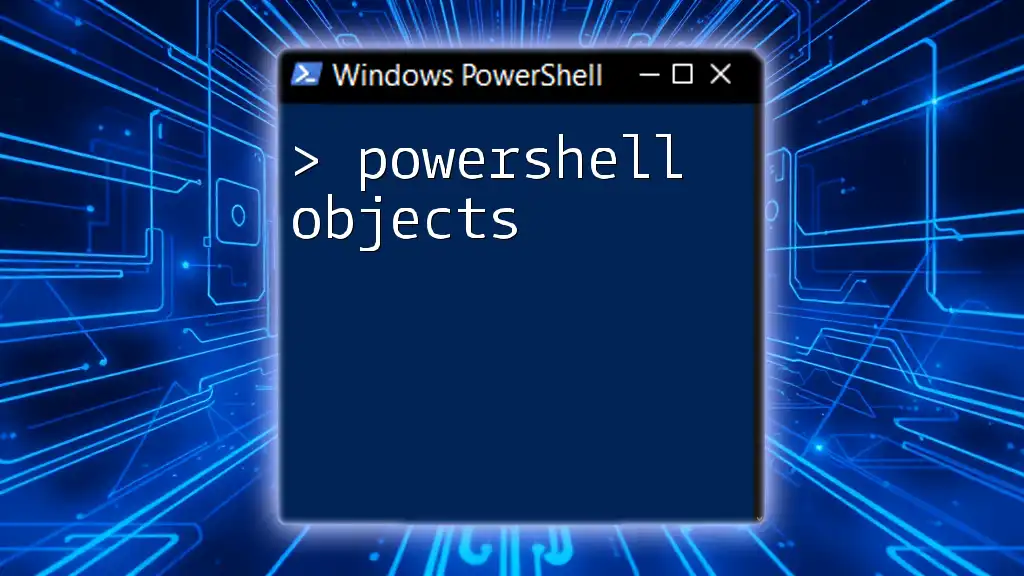
Conclusion
Understanding how to leverage the "PowerShell object contains" concept effectively enables you to manage data and configurations with precision and ease. Mastering these methods will streamline your scripting and increase your productivity.
As you continue your PowerShell journey, don't hesitate to explore various examples and practice different scenarios to deepen your knowledge.