In PowerShell, the `-like` operator is used for pattern matching with wildcard characters, while the `-contains` operator checks for the existence of an exact value within a collection.
Here's a code snippet illustrating both operators:
# Example usage of -like and -contains
$fruits = @('Apple', 'Banana', 'Cherry')
# Using -like to find items matching a pattern
$fruits -like 'A*' # Matches 'Apple'
# Using -contains to check for an exact value
$fruits -contains 'Banana' # Returns True
Understanding PowerShell's Comparison Operators
What are Comparison Operators?
Comparison operators in PowerShell are used to evaluate relationships between values, allowing you to perform logical comparisons. They are essential in scripting as they facilitate decisions and filtering functionality, helping you automate tasks efficiently.
Overview of the `-like` and `-contains` Operators
Two widely used comparison operators in PowerShell are `-like` and `-contains`. While both operators help you perform comparisons, they serve different purposes:
- `-like`: Primarily used for string pattern matching.
- `-contains`: Used to determine whether a specific item exists within a collection, such as an array.
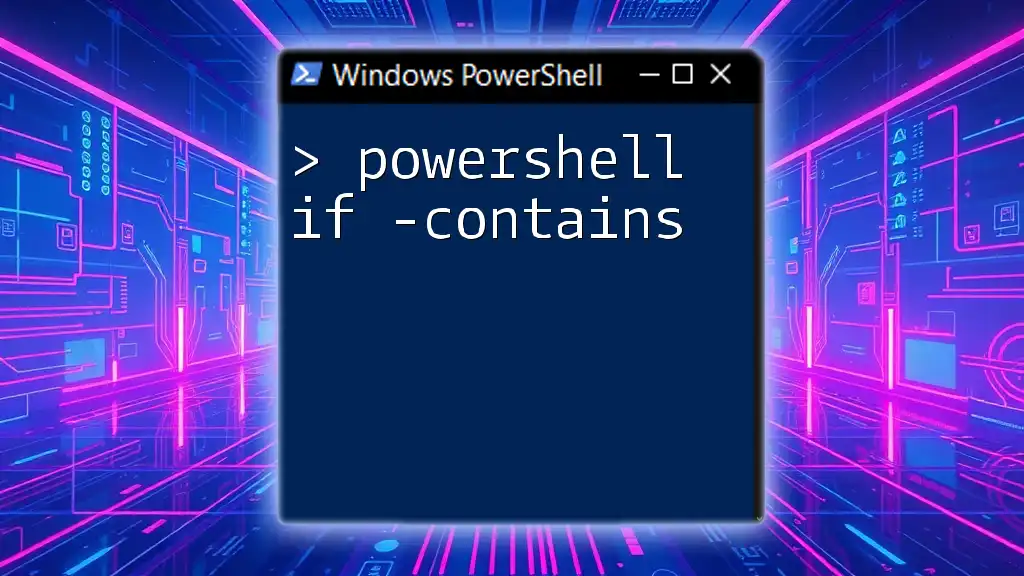
The `-like` Operator
Introduction to `-like`
The `-like` operator allows you to compare strings against a specified pattern. It's commonly used when working with strings that may include wildcards, enabling flexible searches. Its primary function is to check for substring matches within a string.
Syntax and Wildcards
The basic syntax for the `-like` operator is as follows:
<Expression> -like <Pattern>
Wildcards:
- `*` matches zero or more characters.
- `?` matches exactly one character.
Example: Using Wildcards with `-like`
$fruitList = "apple", "banana", "cherry"
$fruitList | Where-Object { $_ -like "b*" }
In this example, the result will be `"banana"` because it matches the pattern that starts with "b."
Common Use Cases for `-like`
Checking Substrings in Strings: One common application of `-like` is to check whether a substring exists within a string.
Filtering Objects: You can also use `-like` in filtering objects returned from cmdlets based on properties.
Example: Filtering Objects by Name
Get-Process | Where-Object { $_.Name -like "*explorer*" }
This command retrieves all processes with names that include "explorer," such as "explorer" and "explorer.exe."
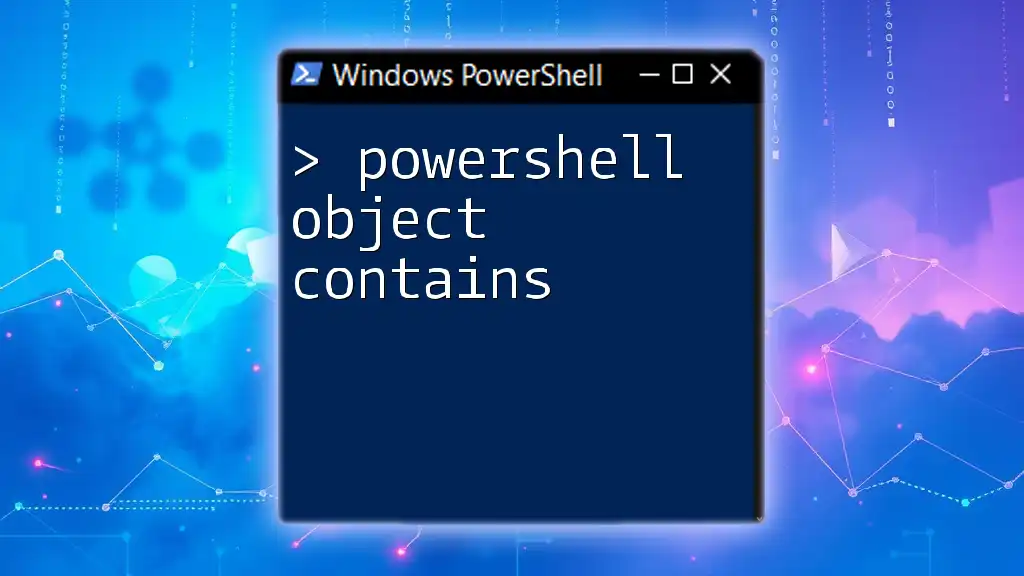
The `-contains` Operator
Introduction to `-contains`
The `-contains` operator is designed to determine if a specific item exists within a collection, such as an array. This operator is straightforward and evaluates to `$true` if the item is found within the collection.
Syntax and Usage
The syntax for the `-contains` operator is as follows:
<Collection> -contains <Item>
Example: Checking for Item Existence
$numbers = 1, 2, 3, 4
$numbers -contains 3
In this instance, the expression evaluates to `$true`, indicating that the value "3" exists within the `$numbers` array.
Common Use Cases for `-contains`
Checking Membership in Arrays: The `-contains` operator is frequently used to validate if certain values exist within an array or collection.
Validating Values in Scripts: It can help in writing scripts that require checks against allowed or predefined datasets.
Example: Validating User Input
$allowedUsers = "Alice", "Bob", "Charlie"
$userInput = "Alice"
If ($allowedUsers -contains $userInput) {
Write-Host "User is allowed."
}
In this example, the script checks if the input user is in the list of allowed users and outputs a message if they are.
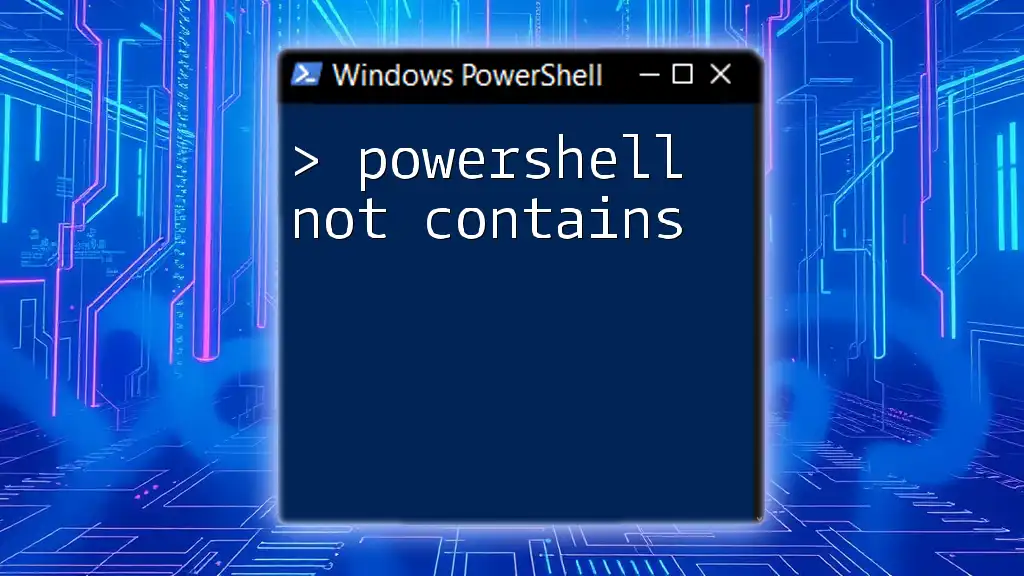
Key Differences Between `-like` and `-contains`
Type of Data Each Operates On
One of the primary differences is that `-like` operates on strings and allows for pattern matching, while `-contains` is used strictly with collections and checks for the existence of a specific item. Knowing which operator to use based on the data type you manipulate is essential for effective scripting.
Use Cases Comparison
Ideal Scenarios for Using `-like`:
- When you need to find patterns or specific text within strings.
- When wildcard searches are necessary to capture variations in data.
Ideal Scenarios for Using `-contains`:
- When you’re working with fixed collections like arrays and need to check membership.
- When you want to validate user inputs against a set of known values.
Examples of Misuse
Incorrect Use of `-like` Instead of `-contains`
# Incorrect
if ($fruitList -like "apple") { }
This code incorrectly uses `-like` to check for an exact match when `-contains` would be more appropriate.
Incorrect Use of `-contains` for Substring Search
# Incorrect
if ($fruitList -contains "app") { }
Here, this statement does not work as intended because `-contains` checks for exact matches, not substrings.
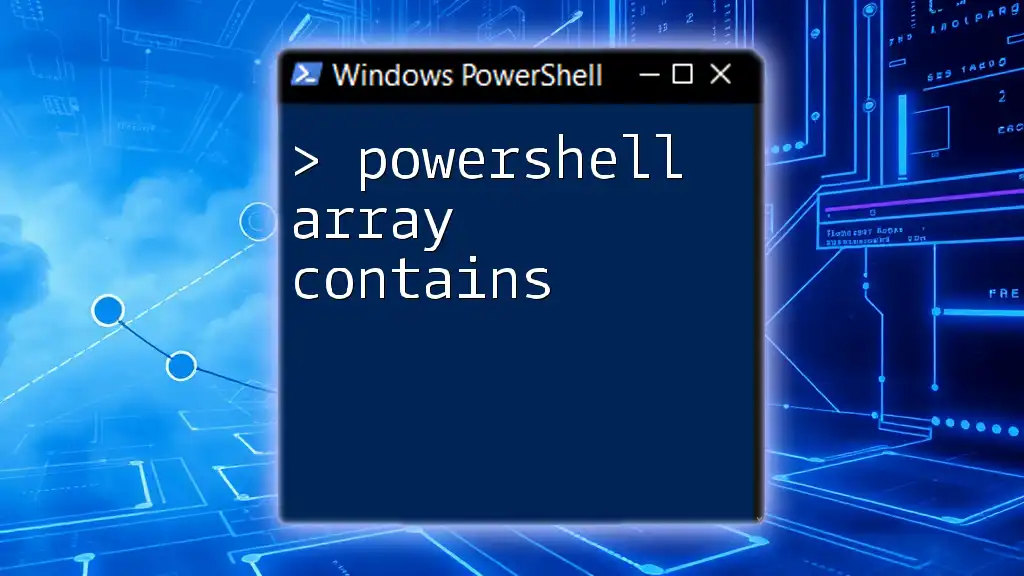
Conclusion
Summary of Comparison Operators
In summary, understanding the differences between `-like` and `-contains` is pivotal when scripting in PowerShell. Using `-like` allows for pattern matching within strings, while `-contains` provides a succinct way to check for an item's existence within a collection.
Practical Tips for Users
-
Choosing the Right Operator: Assess the data type you're working with before selecting an operator. When working with strings, prefer `-like`. When handling arrays or collections, use `-contains`.
-
When to Use Wildcards with `-like`: Employ wildcards strategically to capture broader search results, but be cautious to avoid overmatching.
-
Best Practices for Handling Collections with `-contains`: Always ensure you’re checking for the existence of precise values and not substrings when using `-contains`.
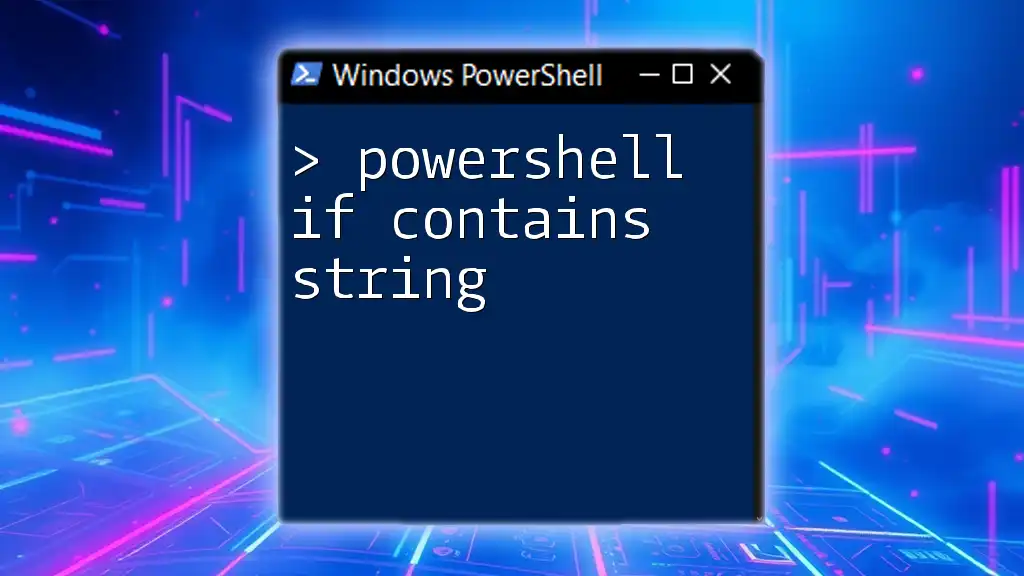
Additional Resources
Further Reading and Tutorials
For more advanced learning, refer to the [official PowerShell documentation](https://learn.microsoft.com/en-us/powershell/scripting/overview?view=powershell-7.2) and consider checking out online tutorials and courses that focus on mastering PowerShell scripting. By deepening your understanding, you can unlock the full power of PowerShell for system administration and automation tasks.