In PowerShell, you can check if a string contains a specific substring using the `-like` or `-contains` operators within an `if` statement. Here's a code snippet demonstrating this:
$string = "Hello, World!"
if ($string -like "*World*") {
Write-Host "The string contains 'World'."
}
Understanding PowerShell Strings
What is a String in PowerShell?
In PowerShell, a string is a sequence of characters used to represent text. Strings are essential in scripting for tasks such as output messages, file names, and data manipulation. Understanding how to work with strings allows you to create more dynamic and user-friendly scripts.
How to Create Strings in PowerShell
Creating strings in PowerShell is simple. You can define a string using either single quotes or double quotes. Using single quotes creates a literal string where escape sequences and variables are not evaluated. In contrast, double quotes allow for the evaluation of expressions and variables.
$literalString = 'This is a literal string with no variable $variable'
$evaluatedString = "This is an evaluated string with variable $variable"
In summary, knowing when to use single or double quotes is crucial for effective string manipulation in PowerShell.
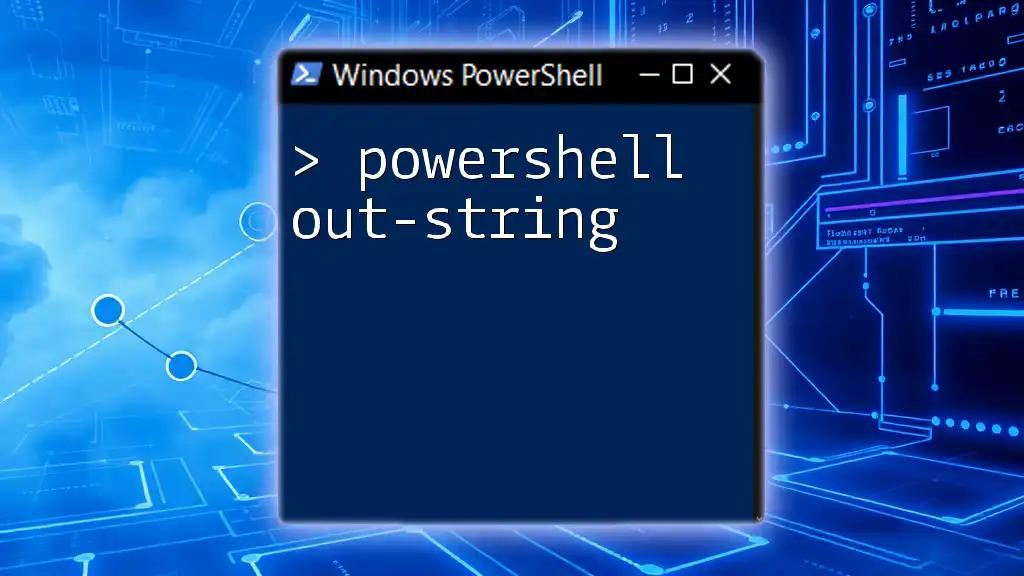
The Concept of "Contains" in PowerShell
What Does "Contains" Mean?
The term "contains" refers to checking whether a string includes a specific substring. This concept is vital in conditional statements, especially when you need to verify the presence of certain text within a string.
Relevant Methods and Operators
Using the `.Contains()` Method
The `.Contains()` method is a straightforward way to check for substrings within a string. It returns True if the substring exists, causing for easy conditional evaluations.
$myString = "Hello, PowerShell!"
$containsSubstring = $myString.Contains("PowerShell")
if ($containsSubstring) {
Write-Host "Substring found!"
}
In this example, the script checks if "PowerShell" is part of `$myString`. If it is, the message "Substring found!" is displayed.
Using the `-like` Operator
The `-like` operator is useful for pattern matching. It uses wildcard characters, making it flexible for more general checks. The asterisk (`*`) represents zero or more characters.
if ($myString -like "*PowerShell*") {
Write-Host "Found PowerShell!"
}
Here, the `-like` operator checks if any part of `$myString` contains "PowerShell" and returns the message accordingly.
Using the `-match` Operator
The `-match` operator utilizes regular expressions for more complex matching scenarios. Unlike `.Contains()` and `-like`, which perform simple checks, `-match` allows for intricate pattern definitions.
if ($myString -match "Power") {
Write-Host "Power found in string!"
}
In this case, the script checks for the presence of "Power" anywhere in `$myString`.

Implementing "If Contains" Logic in PowerShell
Basic Syntax of If Statement
An if statement in PowerShell is used to execute code based on the evaluation of a condition. The basic syntax is straightforward:
if (condition) {
# Code to execute if condition is true
}
Here’s how you can use it with the `.Contains()` method:
if ($myString.Contains("Hello")) {
Write-Host "Greeting found!"
}
If "Hello" is part of `$myString`, the script outputs "Greeting found!"
Combining Multiple Conditions
Using Logical Operators
You can enhance control flow by combining multiple conditions using logical operators such as `-and`, `-or`, and `-not`. For example:
if ($myString.Contains("Hello") -and $myString.Contains("PowerShell")) {
Write-Host "Both terms found!"
}
In this script, it checks if both substrings exist, allowing for more complex decision-making.
Using If-Else Statements
If-else statements allow for alternative actions based on evaluation. This structure is beneficial for binary decisions.
if ($myString.Contains("World")) {
Write-Host "World found!"
} else {
Write-Host "World not found!"
}
This example not only checks for "World," but also provides a fallback message if the substring is absent.

Practical Applications of "If Contains"
Use Cases in Scripting
The "if contains" logic is widely applied throughout various scripting scenarios. For instance, developers often use it to filter data based on string content. Whether checking user input, validating data formats, or configuring settings, the ability to check if a string contains specific characters or words is invaluable.
Real World Example: File Processing
Let's consider a practical example where you search through file names. This task demonstrates how your script can handle real-world applications effectively.
$files = Get-ChildItem -Path "C:\Path\To\Files"
foreach ($file in $files) {
if ($file.Name.Contains("Report")) {
Write-Host "Report found: $($file.Name)"
}
}
In this script, every file in the specified directory is checked. If a file name includes "Report," the script outputs the file’s name, showcasing the utility of the "if contains" check in file management.

Best Practices for String Comparison
Performance Considerations
When working with strings, it’s essential to optimize for performance. The choice of method—whether `.Contains()`, `-like`, or `-match`—can affect execution speed depending on the complexity of your checks. For simple substring searches, prefer `.Contains()` for efficiency.
Readability and Maintainability
Crafting clear, understandable scripts enhances readability and maintainability. Using well-named variables, structured code blocks, and comments will help both current and future developers (including yourself) comprehend your script’s purpose and functionality quickly.
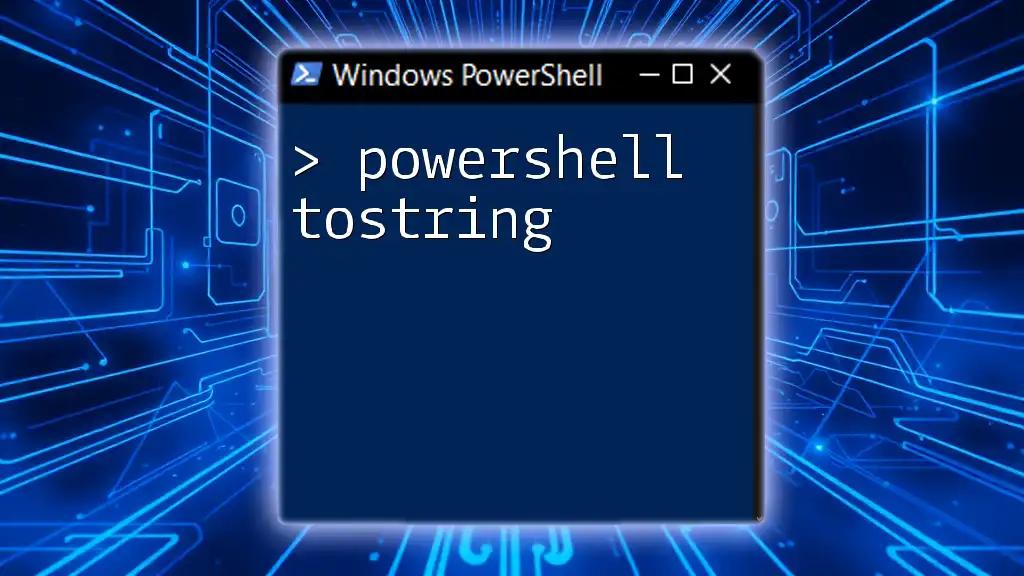
Conclusion
In summary, mastering the concept of "PowerShell if contains string" is crucial for effective scripting. By using methods like `.Contains()`, `-like`, and `-match`, you can enhance your scripts' functionality and add powerful string manipulation capabilities.
Encouragement to practice using these techniques in your scripts will undoubtedly lead to more efficient and effective automation tasks. Exploring further PowerShell topics will elevate your scripting skills, making you a more proficient developer.