PowerShell concatenation allows you to combine multiple strings into a single string using the `+` operator or the `-join` operator for arrays.
Here's a quick example of string concatenation using both methods:
# Using + operator
$greeting = "Hello, " + "World!"
Write-Host $greeting
# Using -join operator
$array = "Hello", "World!"
$joinedGreeting = $array -join ", "
Write-Host $joinedGreeting
Understanding String Concatenation in PowerShell
What is String Concatenation?
String concatenation is the process of joining two or more strings together to form a single string. This is a fundamental operation in programming, as it allows developers to build dynamic text content. In the context of PowerShell, string concatenation is essential for creating informative messages, dynamic file paths, or any other scenario where text assembly is required.
Why Use PowerShell for String Concatenation?
PowerShell is particularly suited for string manipulation due to its versatility and integrated cmdlets. With its rich set of features, PowerShell allows for easy and efficient concatenation, making it a favored tool among system administrators and developers. PowerShell's ability to handle objects, including strings, also enhances its concatenation capabilities.
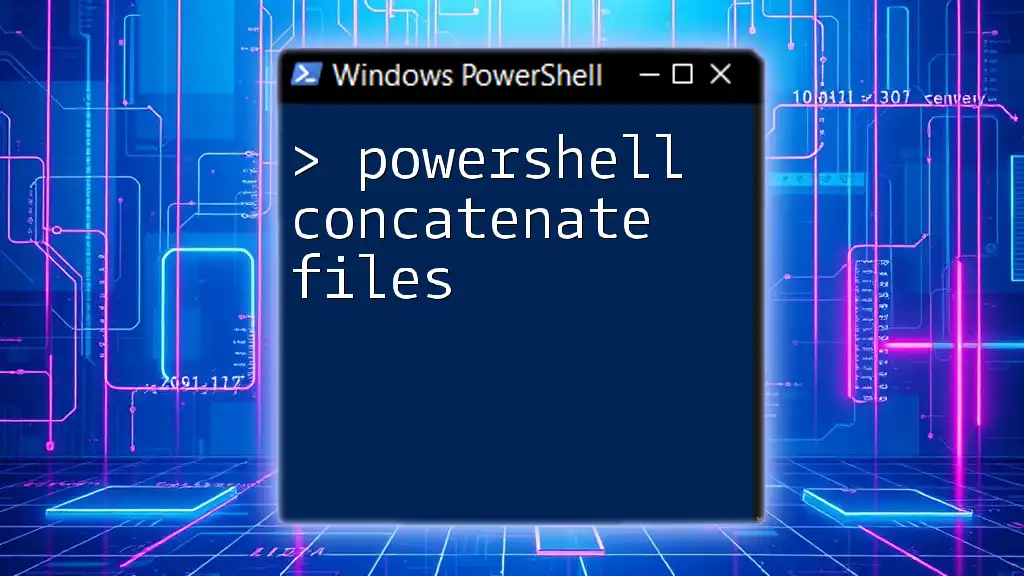
Employing PowerShell to Concatenate Strings
Basic String Concatenation Techniques
Using the `+` Operator
The simplest way to concatenate strings in PowerShell is by utilizing the `+` operator. This straightforward method enables you to join strings directly.
For example, consider the following code snippet that combines first and last names:
$firstName = "John"
$lastName = "Doe"
$fullName = $firstName + " " + $lastName
In this example, the `+` operator combines the variable `$firstName`, a space `" "`, and `$lastName` to create the full name "John Doe".
Utilizing the `-f` Operator for Formatting
PowerShell also provides the `-f` operator for string formatting. This method is beneficial for inserting multiple variables into a formatted string.
Here’s an example that employs the `-f` operator:
$name = "Alice"
$greeting = "Hello, {0}" -f $name
In this case, `{0}` serves as a placeholder for the variable `$name`, resulting in "Hello, Alice". This approach is particularly useful when dealing with multiple variables and complex strings.
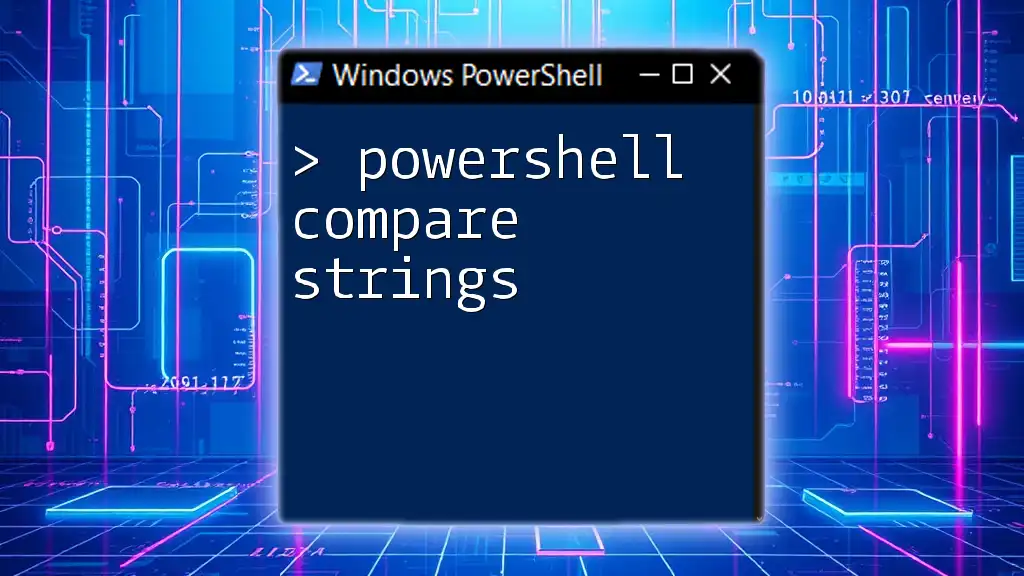
Advanced Techniques for Concatenating Strings
Using the `Join-String` Cmdlet
PowerShell's `Join-String` cmdlet is an excellent method for combining arrays of strings. It allows you to specify a separator for the concatenated result.
The following example illustrates its usage:
$strings = "apples", "bananas", "cherries"
$result = $strings | Join-String -Separator ", "
Here, the `Join-String` cmdlet takes an array of strings and combines them into a single string, separated by a comma and a space, resulting in "apples, bananas, cherries".
Concatenate Strings with Variables
Concatenating strings with variables is common in PowerShell. It simplifies the process of holding information dynamically.
For instance, the following code illustrates how to combine strings and variables:
$item = "book"
$quantity = 2
$message = "You have {0} {1}(s)." -f $quantity, $item
The output here would be "You have 2 books." Utilizing the `-f` operator allows for clean and clear incorporation of variables into the string.
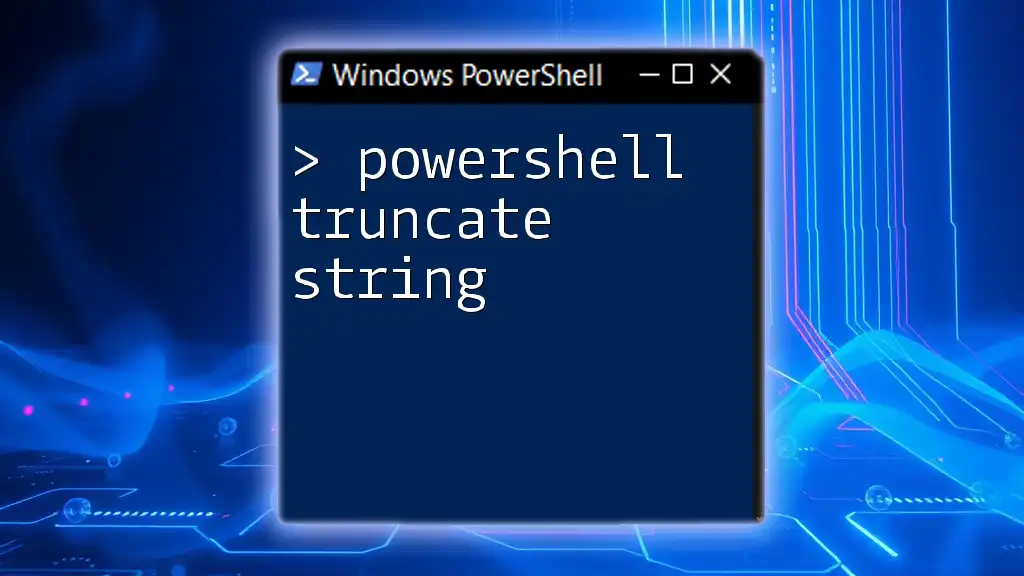
Practical Applications of String Concatenation
Creating Dynamic File Paths
String concatenation can be particularly useful when you need to create dynamic file paths within your scripts. You can easily customize file locations or names depending on user input or script variables.
For example:
$folder = "Documents"
$fileName = "report.txt"
$fullPath = "C:\Users\$env:USERNAME\$folder\$fileName"
This code snippet constructs a path to a file in the current user's Documents folder, dynamically incorporating the username.
Building Complex Strings
You can also build more complex formatted strings with multiple elements. Here's an example:
$username = "user123"
$domain = "example.com"
$email = "$username@$domain"
In this example, the email is constructed by concatenating the `$username` variable with the `$domain`, resulting in "user123@example.com". This method keeps your code clean and efficient.
Concatenating Strings in Loops
PowerShell allows you to concatenate strings within loops, adding elements dynamically. This can be particularly useful when processing collections.
Consider the following example where we iterate through an array of fruits:
$fruits = @("apple", "banana", "cherry")
$fruitList = ""
foreach ($fruit in $fruits) {
$fruitList += "$fruit, "
}
$fruitList = $fruitList.TrimEnd(', ')
This results in the string "apple, banana, cherry". The `TrimEnd` method removes the trailing comma and space, ensuring your final string is tidy.
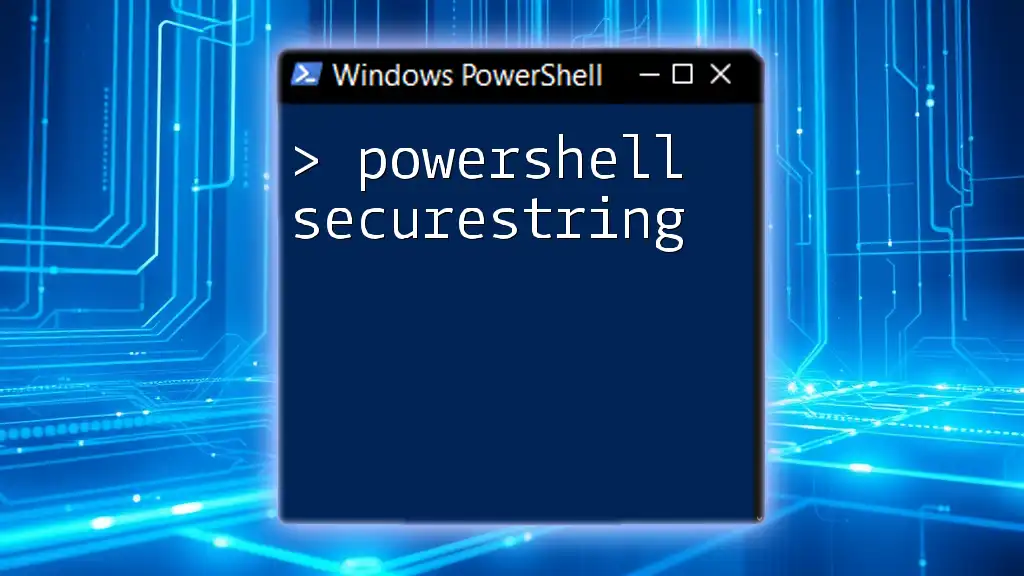
Common Issues and Troubleshooting
Incorrect Results in Concatenation
One common issue when concatenating strings is unexpected results due to trailing spaces or incorrect data types. Always ensure that the data being concatenated is of the string type. Use methods like `Trim()` to handle spaces.
Performance Considerations
While using the `+` operator for concatenation works well for small strings, performance can degrade with extensive concatenation in loops. In such cases, consider using `StringBuilder`, a more efficient choice for manipulating large strings.
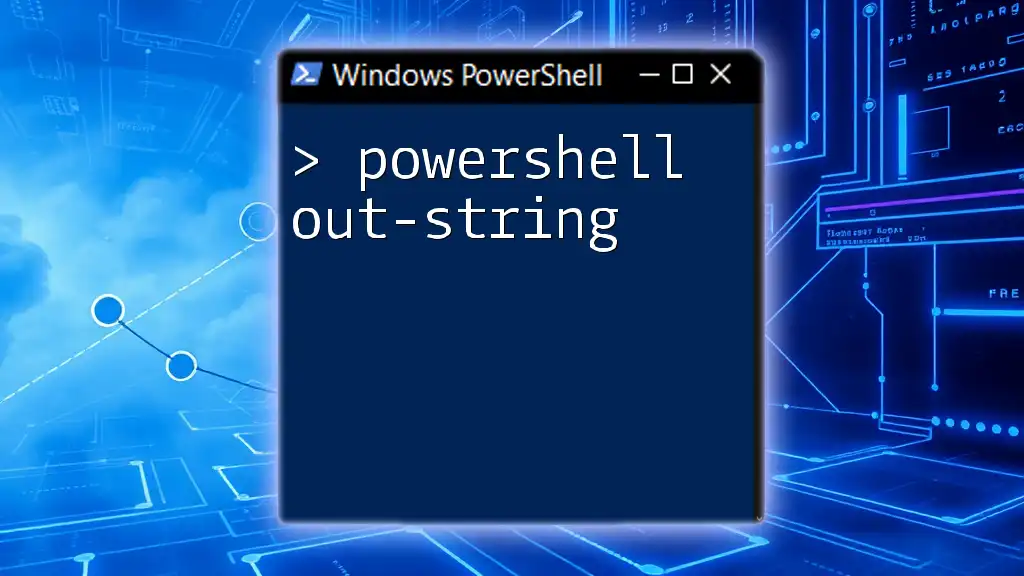
Conclusion
Understanding how to perform string concatenation in PowerShell is crucial for effective script writing and automation tasks. By mastering the different techniques—such as using the `+` operator, the `-f` operator, and cmdlets like `Join-String`—you can enhance your PowerShell scripts and make them more dynamic and user-friendly. The practical applications discussed, from creating file paths to building complex strings, further demonstrate the versatility of PowerShell in handling string operations.
Take the time to practice these techniques, and consider exploring additional resources that can help you deepen your knowledge of PowerShell string manipulation.