PowerShell's `SecureString` is a type of string that stores sensitive information such as passwords in an encrypted format, providing enhanced security compared to regular strings.
Here’s a code snippet showcasing its usage:
$secureString = ConvertTo-SecureString "MyP@ssw0rd" -AsPlainText -Force
Write-Host "The secure string has been created."
What is SecureString?
SecureString is a special data type in PowerShell designed to securely handle sensitive information, such as passwords and personal data. It implements encryption to protect the data in memory, ensuring that it is not exposed to potential vulnerabilities or memory-based attacks. Unlike standard strings, which store plain text, SecureString maintains the confidentiality of sensitive information, making it essential for secure scripting.
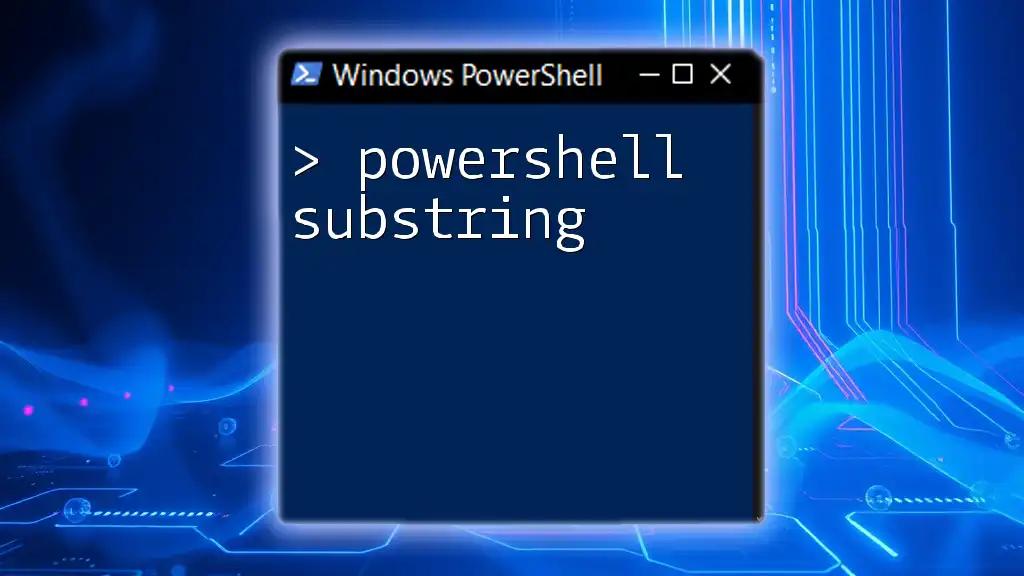
Why Use SecureString in PowerShell?
Using SecureString is pivotal in any script dealing with sensitive information because of its inherent security features.
- Protection Against Memory-Based Attacks: SecureString helps mitigate risks related to memory scraping techniques, where attackers attempt to extract sensitive information residing in the application's memory.
- Reduced Risk of Exposing Sensitive Information: By utilizing SecureString, developers can prevent accidental exposure of passwords during script execution or logging, ensuring that sensitive data remains confidential.
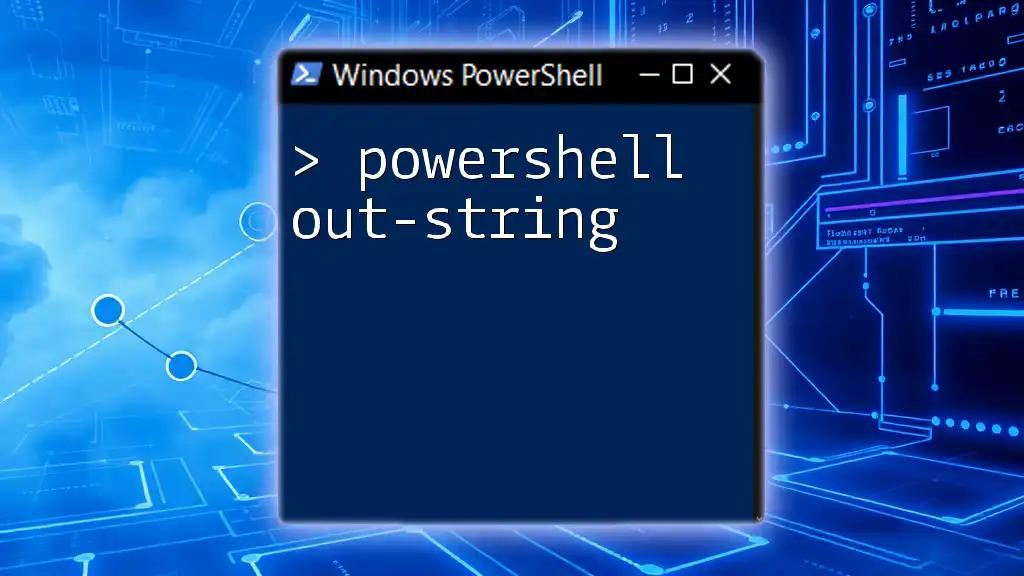
Understanding the Basics of SecureString
Creating a SecureString
Creating a SecureString is straightforward, primarily through PowerShell's built-in cmdlets. To securely prompt for a password, you can use the `Read-Host` cmdlet with the `-AsSecureString` parameter. This practice ensures that any input is masked as it is entered.
Example:
$secureString = Read-Host -AsSecureString "Enter your password"
This command prompts the user to enter a password that is stored as a SecureString variable, preventing it from appearing in plain text.
Converting Plain Text to SecureString
When working with sensitive data in scripts, it is crucial to convert plain text strings to SecureString. This not only helps in maintaining confidentiality but also aligns with best practices for handling sensitive data in scripts.
Keyword focus: powershell convertto securestring
Example:
$plainTextPassword = "MySecretPassword"
$securePassword = ConvertTo-SecureString $plainTextPassword -AsPlainText -Force
In this example, the `ConvertTo-SecureString` cmdlet transforms a plain text password into a SecureString, with the `-AsPlainText` parameter indicating the input type. The `-Force` parameter bypasses any additional confirmation for this operation.

Using ConvertTo-SecureString
What is ConvertTo-SecureString?
`ConvertTo-SecureString` is a powerful cmdlet that converts encrypted strings into SecureString objects. This is essential for securely handling sensitive data such as passwords when scripting.
Different Parameters of ConvertTo-SecureString
-AsPlainText
This parameter is used to specify that the input being processed is a plain text string. However, it should be used judiciously, preferably in scripts where security is not compromised.
-Force
The `-Force` flag is instrumental in bypassing confirmation prompts that might otherwise require user interaction, thus automating scripts more efficiently.
-Key
The `-Key` parameter allows you to specify an encryption key for the SecureString. This is especially useful in scenarios where additional security measures are required, such as when storing sensitive data long-term.
Example: Converting Strings with ConvertTo-SecureString
You can combine these parameters to create a secure password with an encryption key.
$secureStringWithKey = ConvertTo-SecureString "MySecretPassword" -AsPlainText -Force -Key (1..16)
This code generates a SecureString object from the provided plain text password and encrypts it using a defined key.

Working with SecureString in PowerShell
Using SecureString in Cmdlets
SecureString enhances security across many cmdlets that require sensitive information. One prevalent example is `Get-Credential`, which prompts the user for a username and password while utilizing SecureString.
$cred = Get-Credential
This command creates a credential object, wherein the password is maintained securely as a SecureString, thereby enhancing confidentiality.
Converting SecureString Back to Plain Text
In certain situations, it may become necessary to convert SecureString back into plain text. However, this should be performed with utmost caution. A common use case is when integrating with legacy systems that require plain text passwords.
Example:
$plainTextPasswordAgain = [Runtime.InteropServices.Marshal]::PtrToStringAuto([Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureString))
This snippet involves marshaling techniques to retrieve the plain text representation of a SecureString, highlighting the importance of having controlled environments when executing such conversions.

Advanced SecureString Techniques
Encrypting SecureString for Storage
To securely store SecureStrings, you can convert them into encrypted formats and write them to files for later use. This workflow ensures that sensitive information isn't easily accessible in plain text.
Example:
$secureString | ConvertFrom-SecureString | Out-File "password.txt"
In this code, the SecureString is serialized and written to a file, ensuring that the sensitive information remains protected.
Decrypting SecureString from Storage
To retrieve the SecureString, you can easily read it back from the file and convert it back into a SecureString object.
Example:
$secureStringFromFile = Get-Content "password.txt" | ConvertTo-SecureString
This approach highlights the simplicity of working with SecureString for persistent storage while maintaining data security.
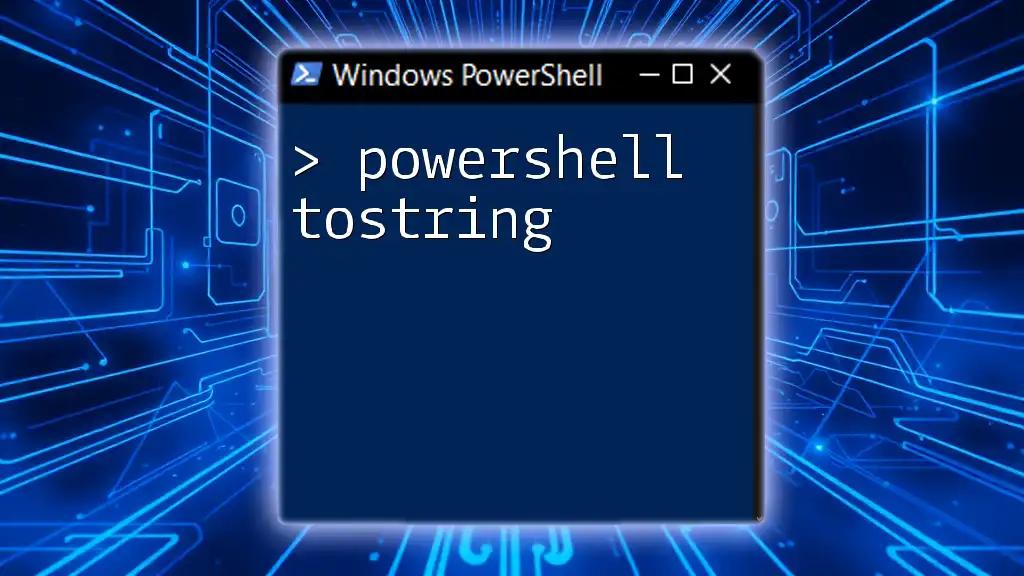
Common Scenarios and Best Practices
When to Use SecureString
You should always consider using SecureString in scenarios where sensitive information is involved, such as:
- Storing passwords and authentication tokens.
- Handling any PII (Personally Identifiable Information).
- Integrating with APIs requiring credentials.
Best Practices for Handling SecureString
- Avoid exposing SecureString unnecessarily: Keep SecureStrings within scope and avoid converting them back to plain text unless absolutely necessary.
- Securely store encryption keys: If you use keys for encryption, ensure they are managed properly and not hard-coded in scripts.
- Follow principle of least privilege: Limit the access to scripts that handle sensitive data.
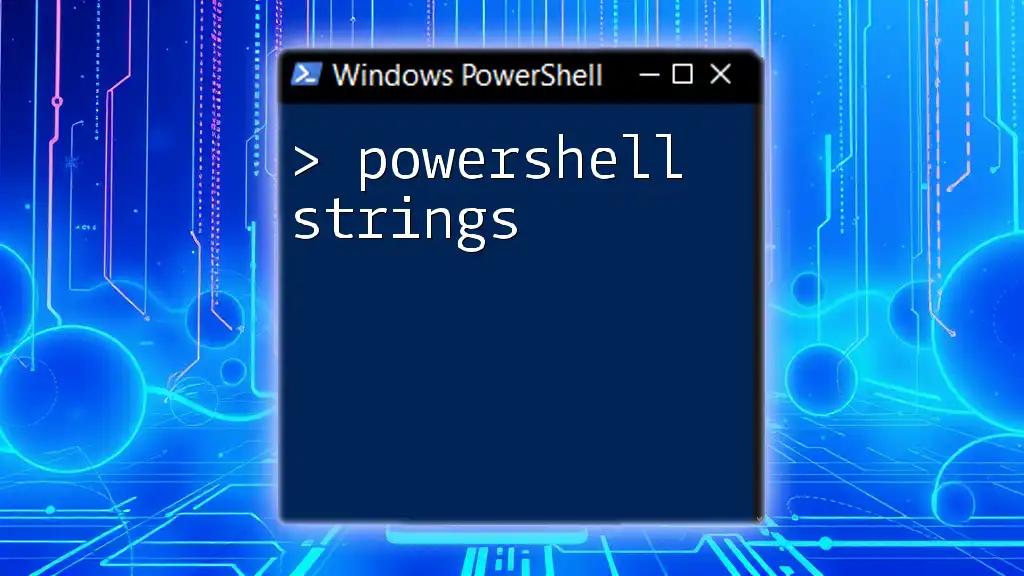
Conclusion
Incorporating PowerShell SecureString into your scripting practices is essential for maintaining the confidentiality of sensitive information. The capabilities of SecureString, combined with the commands like `ConvertTo-SecureString`, provide a robust framework for secure scripting. By following the best practices outlined above, you can ensure that your scripts not only function as intended but also safeguard the sensitive information they handle, ultimately leading to more secure and stable applications.
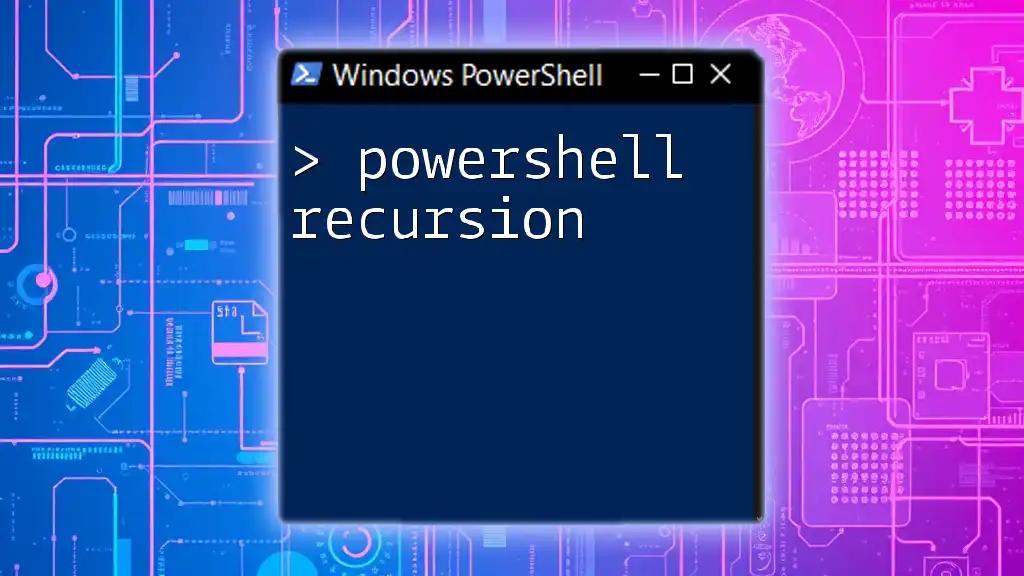
Further Learning Resources
For those looking to expand their knowledge further, consider exploring books, courses, and credible websites focused on PowerShell security practices. Engaging with ongoing education in this domain will enhance your capabilities and keep you updated with best practices in scripting security.