The PowerShell `Substring` method allows you to extract a specified number of characters from the right side of a string, starting from a given index.
Here’s a code snippet demonstrating how to use it:
# Define the string
$string = "Hello, World!"
# Extract substring from the right, starting at index 7
$substring = $string.Substring(7, 6)
Write-Host $substring # Output: World!
Understanding Substring in PowerShell
What is a Substring?
A substring is simply a segment of a string. In PowerShell, strings are collections of characters, and each character is indexed starting from zero. For example, in the string "Hello", 'H' is at index 0, 'e' is at index 1, and so forth. Understanding how to manipulate these strings through substrings is a fundamental skill in scripting, especially when processing and extracting data.
The Need for Right Substring Extraction
You might find yourself in situations where you need to extract part of a string—especially from the right. This is useful in many real-world scenarios, such as when handling file names, cleaning user input, or extracting specific data from logs. For instance, extracting the last part of a file path or isolating a date from a timestamp can greatly improve data handling.

The `Substring()` Method in PowerShell
Syntax Overview
The `Substring()` method is a key tool for manipulating strings in PowerShell. Its basic syntax is as follows:
$string.Substring(startIndex, length)
- Parameters Explained:
- `startIndex`: This parameter specifies where to start the substring extraction in the target string.
- `length`: This defines how many characters to include in the substring.
Basic Example of the Substring Method
Here's a simple example to illustrate the use of the `Substring()` method:
$myString = "Hello World"
$result = $myString.Substring(6, 5) # Output will be "World"
Write-Output $result
In this example, we are starting the substring from index 6 and extracting 5 characters, which results in "World".
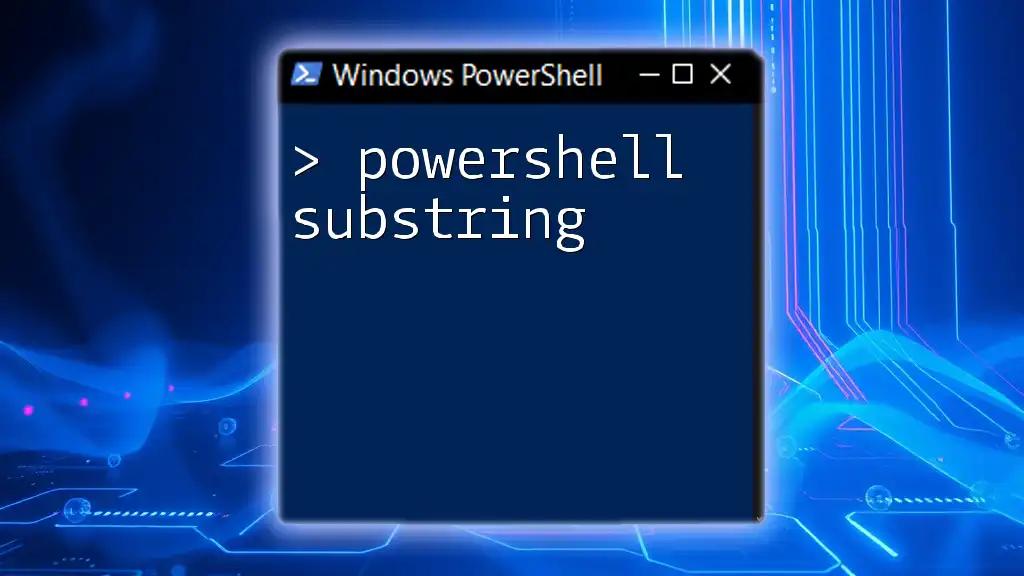
Extracting a Substring from the Right
The Concept of "Right" Substring
When we talk about extracting a substring from the right, we refer to starting our extraction based on the count of characters from the end of the string. To achieve this, it's crucial to calculate the `startIndex` by subtracting the desired substring length from the total length of the string.
Implementing Right Substring Logic
To derive the starting index for a right substring, you can use the following formula:
$startIndex = $string.Length - length
Here's a practical example:
$string = "PowerShell Scripting"
$length = 9
$startIndex = $string.Length - $length
$rightSubstring = $string.Substring($startIndex)
Write-Output $rightSubstring # Output will be "Scripting"
In this case, we want to extract the last 9 characters of the string "PowerShell Scripting". The starting index is calculated as 16 (the total length) minus 9, resulting in the substring "Scripting".
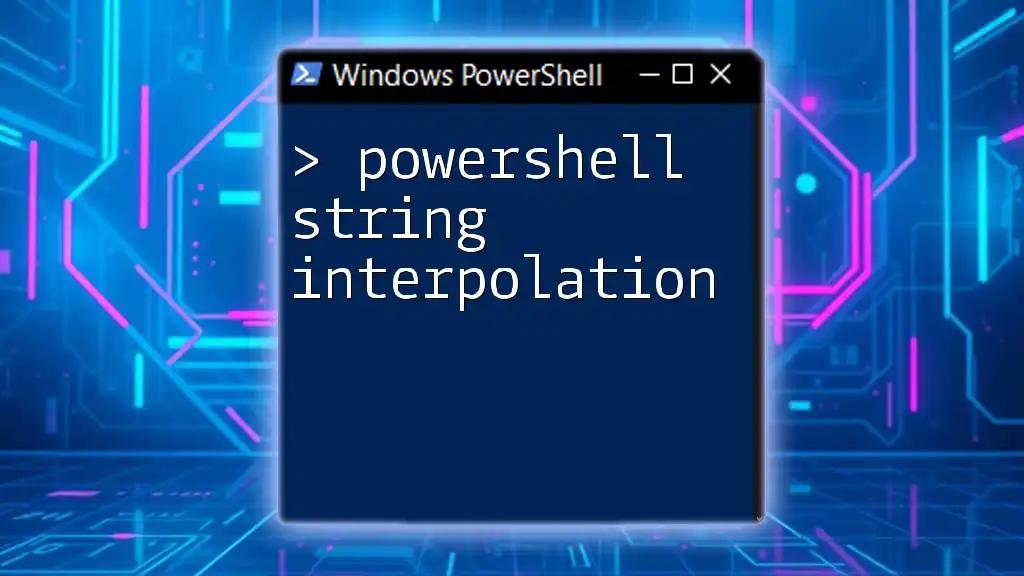
Using PowerShell Right Substring in Real Scenarios
File Name Manipulation
Consider the situation where you want to extract the file extension from a full file path. You can accomplish this efficiently using the right substring method:
$filePath = "C:\Users\JaneDoe\Documents\report.docx"
$extensionLength = 5 # Length of ".docx"
$startIndex = $filePath.Length - $extensionLength
$fileExtension = $filePath.Substring($startIndex)
Write-Output $fileExtension # Output will be ".docx"
In this snippet, we isolate the file extension by calculating the appropriate starting index based on its length, ultimately extracting ".docx".
Data Extraction from Text
Another common scenario involves extracting a specific date from a string of data. Here’s how to achieve this:
$data = "2023-09-20T08:30:00"
$dateLength = 10
$startIndex = $data.Length - $dateLength
$date = $data.Substring($startIndex)
Write-Output $date # Output will be "2023-09-20"
By using the length of the date string, we can accurately retrieve "2023-09-20" from the full timestamp.

Other Methods for Right Substring Extraction
Using `Split()` Method
While the `Substring()` method is powerful, the `Split()` method allows for another approach to achieving similar results. For instance, if you want to extract the last part after a certain character (like a dot in file extensions), you can use:
$filePath = "C:\Users\JaneDoe\Documents\report.docx"
$fileParts = $filePath -split "\."
$fileExtension = $fileParts[-1] # Get the last part after the last dot
Write-Output $fileExtension # Output will be "docx"
Exploring Regular Expressions
Regular expressions (regex) are an advanced technique for string manipulation, offering robust solutions for complex patterns. For extracting rightmost characters, you can use:
$string = "PowerShell Scripting"
$rightSubstring = [regex]::Match($string, ".{9}$").Value
Write-Output $rightSubstring # Output will be "Scripting"
In this example, the regex pattern `.{9}$` matches the last 9 characters of the string, effectively isolating "Scripting".

Conclusion
In this guide, we've explored the concept of PowerShell substring right extraction in depth. From understanding the fundamental `Substring()` method to applying it in real-world scenarios, this article equips you with the knowledge necessary to manipulate strings effectively. Whether you are managing files or processing data, mastering substring extraction enhances your PowerShell scripting capabilities.

Further Reading and Resources
For those eager to continue their PowerShell education, consider exploring the official [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) or engaging with community resources that provide tutorials and insights into effective scripting practices.