The `-f` operator in PowerShell is used for formatting strings by inserting values into placeholders within the string, providing a simple way to construct dynamic strings.
$name = "Alice"
$greeting = "Hello, {0}!" -f $name
Write-Host $greeting
Introduction to PowerShell String Formatting
PowerShell is a powerful scripting language primarily used for task automation and configuration management. One of the essential features of PowerShell is string formatting, allowing users to create dynamic and customizable messages that enhance the clarity and effectiveness of scripts. Among its various functionalities, the `-f` operator stands out as a straightforward yet potent tool for string interpolation.
Understanding the Basic Syntax of the `-f` Operator
The fundamental syntax of the `-f` operator is straightforward:
"String {0} {1}" -f value1, value2
Within the string, placeholders indicated by `{}` are used to specify where the formatted values will be injected. Each placeholder is indexed, starting from 0 for the first value, 1 for the second value, and so on.
Code Example
To illustrate its usage, consider the following snippet:
$template = "Hello, {0}!"
$name = "World"
$greeting = $template -f $name
Write-Output $greeting # Outputs: Hello, World!
In this example, the string is constructed dynamically based on the value assigned to `$name`, demonstrating the power of dynamic string generation.

Practical Applications of the `-f` Operator
Dynamic String Creation
One of the primary applications of the `-f` operator is to create dynamic messages. This functionality is particularly useful in scripts that communicate status updates, error messages, or general information.
Code Example
Consider a scenario where we want to notify the user when an action has been completed:
$user = "Alice"
$action = "logged in"
$message = "User {0} has {1}." -f $user, $action
Write-Output $message # Outputs: User Alice has logged in.
In this case, the message clearly indicates who performed what action, showcasing the practical benefits of string formatting.
Formatting Dates and Numbers
The `-f` operator can also be leveraged to format dates and numbers, ensuring that output is both readable and meaningful.
Formatting Dates for Better Readability
For example, we can present the current date in a specific format:
$date = Get-Date
$formattedDate = "Today's date is: {0:yyyy-MM-dd}" -f $date
Write-Output $formattedDate # Outputs: Today's date is: yyyy-MM-dd formatted date.
This not only makes the output more legible but also emphasizes the utility of `-f` in various contexts.
Rounding Numbers
Another common use is formatting numerical values. Using the `-f` operator, the following code rounds a number to two decimal places:
$pi = 3.14159
$formattedPi = "Pi rounded to 2 decimal places is: {0:F2}" -f $pi
Write-Output $formattedPi # Outputs: Pi rounded to 2 decimal places is: 3.14
The `{0:F2}` format specifier tells PowerShell to represent the number as a float with two decimal points, further illustrating the versatility of string formatting.
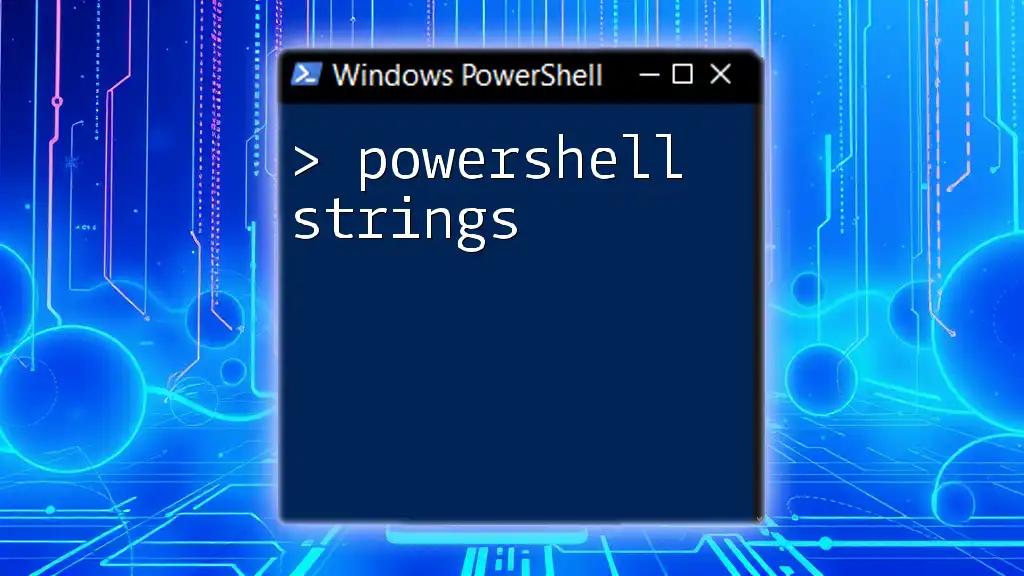
Combining the `-f` Operator with Other PowerShell Features
Integrating with Loops
The `-f` operator shines in conjunction with loops, allowing for the generation of multiple dynamic strings in a single run.
Code Example
Imagine a list of users logging into a system:
$users = @("Alice", "Bob", "Charlie")
foreach ($user in $users) {
$message = "Welcome, {0}!" -f $user
Write-Output $message
}
This loop iterates through each user, creating a personalized welcome message for each, demonstrating how string formatting can be applied at scale.
Utilizing Conditional Statements
By combining the `-f` operator with conditional statements, you can create tailored messages based on certain criteria.
Code Example
For instance, depending on whether a user is an admin or not, you could craft a different message:
$isAdmin = $true
$message = "You are {0} admin." -f ($isAdmin -eq $true ? "an" : "not an")
Write-Output $message # Outputs: You are an admin.
This flexibility enables the creation of more relevant and contextualized outputs within scripts.
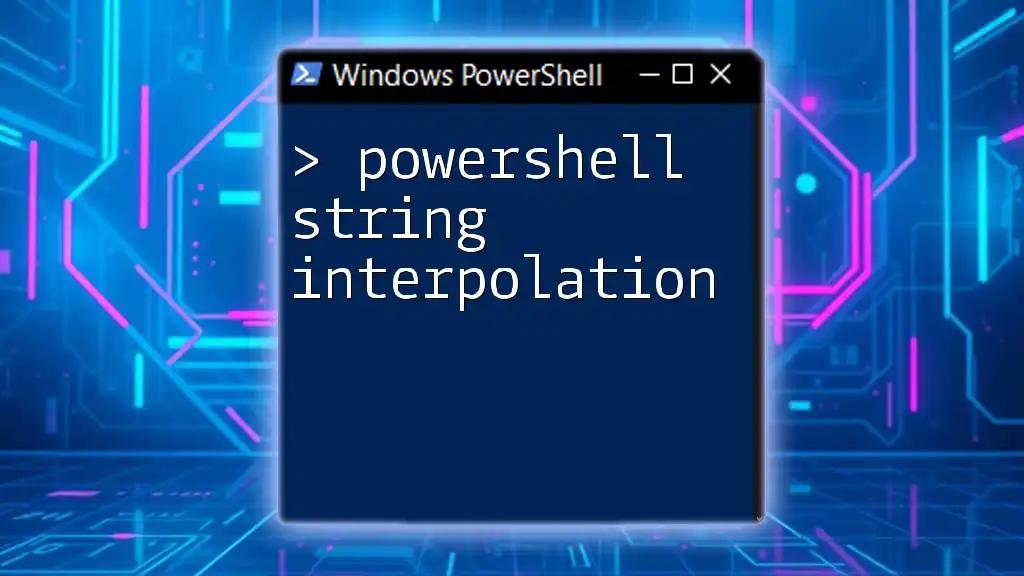
Advanced Usage of the `-f` Operator
Complex Formatting Scenarios
With the `-f` operator, it's easy to manage multiple placeholders effectively. This proves beneficial in constructing messages with various values.
Code Example
You might want to include more information in a single string:
$name = "Alice"
$age = 30
$info = "{0} is {1} years old and plays {2}." -f $name, $age, "tennis"
Write-Output $info # Outputs: Alice is 30 years old and plays tennis.
Here, you see how multiple pieces of information can seamlessly combine into a cohesive message.
Nesting String Formats
For even more complex scenarios, the `-f` operator allows nesting of string formats, adding another layer of versatility.
Code Example
Imagine wanting to include a calculation within a formatted string:
$baseString = "My name is {0} and I am {1}." -f $name, "older than {0}" -f 20
Write-Output $baseString # Outputs: My name is Alice and I am older than 20.
This showcases how you can dynamically construct strings, even while referencing other formatted inputs.

Troubleshooting Common Issues with the `-f` Operator
Despite its usefulness, there are some common pitfalls when using the `-f` operator. Ensuring that placeholders correctly correspond to actual values is paramount.
- Missing Placeholders: If you reference a placeholder that does not exist, PowerShell returns an error.
- Type Mismatches: Ensure that the provided values match the expected types within the format specifiers.
- Common Errors and Solutions: Familiarize yourself with common error messages and their remedies, as these will enhance your debugging capability.

Conclusion
In summary, the PowerShell string `-f` operator serves a vital role in creating dynamic, readable, and contextually relevant messages within scripts. By mastering this operator, you can enhance the efficacy of your PowerShell scripting and provide clearer outputs for users and administrators alike. Engaging with practical examples and real-life scenarios will solidify your understanding and proficiency in using the `-f` operator effectively.

Additional Resources
For those eager to delve deeper into the world of PowerShell, consider exploring the official documentation, recommended books, and online courses dedicated to PowerShell scripting. Engaging in community forums can also provide valuable insights and support as you navigate your PowerShell journey.