Unlock the power of automation and efficiency with these essential PowerShell tricks to streamline your scripting experience.
# Display a greeting message
Write-Host 'Hello, World!'
Getting Started with PowerShell
What is PowerShell?
PowerShell is a powerful command-line shell and scripting language designed for system administration and automation. It allows users to automate tasks and manage configuration across a wide range of platforms. Unlike the traditional command prompt, PowerShell is built on the .NET framework, enabling users to access a rich set of features and objects that make scripting more intuitive.
Setting Up Your PowerShell Environment
To access PowerShell, you can open it easily on any operating system:
- Windows: Type "PowerShell" in the Start menu or search bar.
- macOS: Use the Terminal and install PowerShell via Homebrew with the command `brew install --cask powershell`.
- Linux: Install it directly from the package manager of your distribution or download from the PowerShell GitHub repository.
Customizing your PowerShell profile enhances usability and productivity. You can create a profile script that executes automatically every time you launch PowerShell. Here’s a basic setup to define custom aliases and enable some useful modules:
# Create a new profile if it doesn't exist
if (!(Test-Path -Path $PROFILE)) {
New-Item -ItemType File -Path $PROFILE -Force
}
# Open the profile in a text editor
notepad $PROFILE
Once in the profile, you can add commands like setting aliases or importing modules.
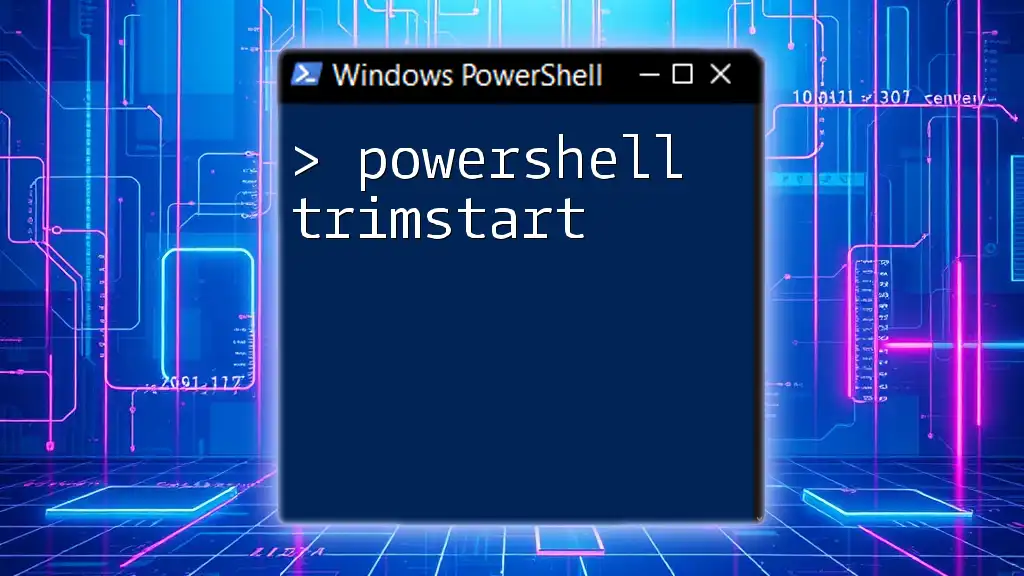
Essential PowerShell Tricks
Command-Line Editing Shortcuts
Using editing shortcuts can significantly speed up your workflow. Ctrl+R allows you to reverse search through your command history, making it easy to find previously executed commands without typing them out again. Other useful keyboard shortcuts:
- Ctrl+A: Move the cursor to the beginning of the line.
- Ctrl+E: Move the cursor to the end of the line.
- Arrow keys: Navigate through your command history conveniently.
Command Aliases: Simplifying Your Workflow
Aliases in PowerShell serve as shortcuts for cmdlets, allowing you to type less and work faster. For instance, rather than typing `Get-ChildItem`, you can simply use `gci`. Here are a few common aliases:
- `ls` for `Get-ChildItem`
- `cd` for `Set-Location`
- `cls` for `Clear-Host`
Creating your own aliases is straightforward. Use the `Set-Alias` cmdlet to define a new alias. For example:
Set-Alias -Name list -Value Get-ChildItem
This command lets you type `list` instead of `Get-ChildItem` for quick directory listings.
Pipelining Commands
Piping is one of the most powerful features in PowerShell, allowing you to pass the output of one command directly into another command. This creates a seamless flow of data and enhances command efficiency. For example, retrieving the top 10 processes by CPU usage can be done with:
Get-Process | Sort-Object CPU -Descending | Select-Object -First 10
In this example, `Get-Process` retrieves all running processes, `Sort-Object` orders them by CPU usage, and `Select-Object` limits the output to the top 10 processes.
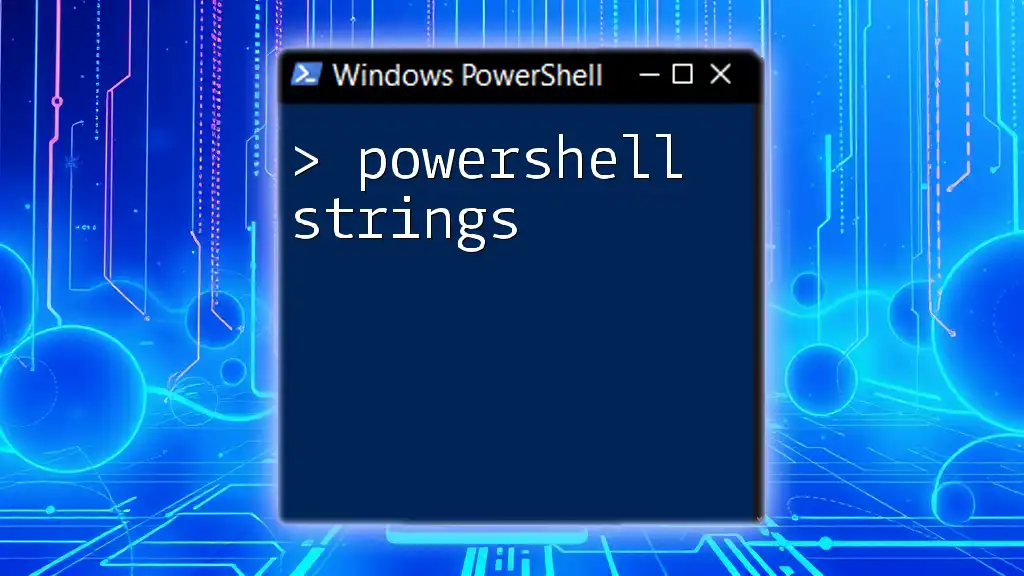
Advanced PowerShell Tricks
Using Cmdlets and Functions
Understanding Cmdlets
Cmdlets are specialized .NET classes that perform specific functions within PowerShell. They follow a Verb-Noun format, which makes it easy to understand what they do. For example, `Get-Service` retrieves information about services on your system. Each cmdlet comes with a built-in help feature, accessible with:
Get-Help Get-Service -Full
Creating Custom Functions
Custom functions in PowerShell allow you to encapsulate frequently used commands into reusable units. Let’s create a simple function to list directory contents:
function Get-DirList {
param([string]$path)
Get-ChildItem -Path $path
}
You can invoke this function like so:
Get-DirList -path "C:\Users"
This makes it easier to retrieve directory listings without repeatedly typing the full `Get-ChildItem` command.
Error Handling Techniques in PowerShell
Effective error handling is critical when writing scripts. PowerShell’s `Try`, `Catch`, and `Finally` blocks enable you to manage errors gracefully. Here’s a simple example of how to handle an error when attempting to read a nonexistent file:
Try {
Get-Content "nonexistentfile.txt"
} Catch {
Write-Host "An error occurred: $_"
}
This captures the error and outputs a message without crashing the script, allowing for smoother execution in production environments.
Leveraging PowerShell Modules
PowerShell modules are packages that contain cmdlets, functions, variables, and other elements. They can greatly extend the capabilities of your scripting environment. To find and import modules from the PowerShell Gallery, you can use the `Find-Module` and `Install-Module` cmdlets. For example, to install the Azure module for managing cloud resources:
Install-Module -Name Az -AllowClobber -Scope CurrentUser
This command installs the Azure module, allowing you to leverage its functionalities for cloud management right from PowerShell.
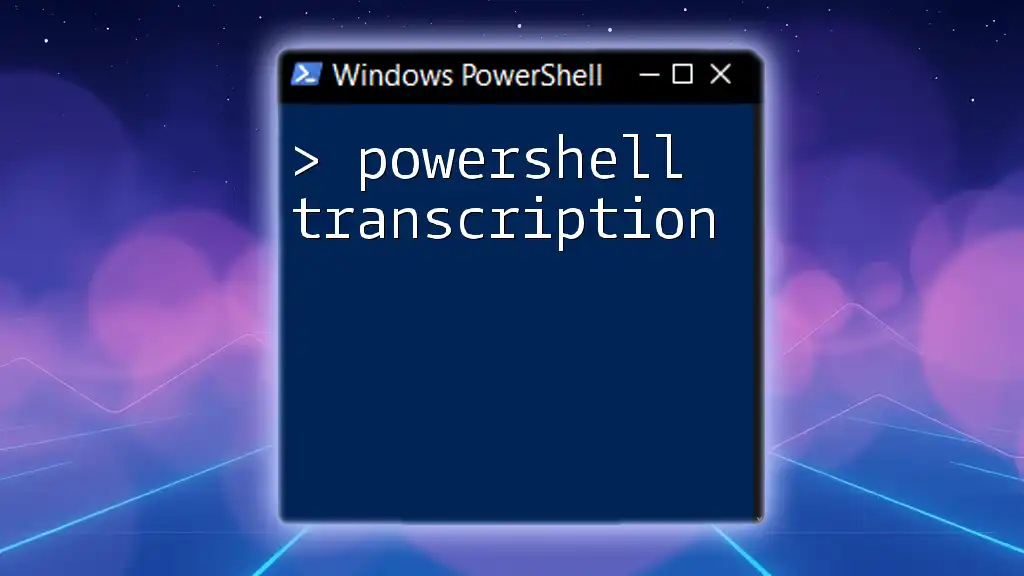
Helpful PowerShell Commands and Snippets
Quick System Information Retrieval
You can quickly retrieve comprehensive system information with the `Get-ComputerInfo` cmdlet. This cmdlet provides details about your system's hardware and software configurations, making it an essential tool for diagnostics and reporting.
Managing Processes with PowerShell
PowerShell enables you to manage processes easily. For example, if you want to stop a running instance of Notepad, you can execute:
Stop-Process -Name "notepad" -Force
This command forcefully halts all instances of Notepad, highlighting how PowerShell can help manage system resources effectively.
Automating Tasks with Scheduled Jobs
PowerShell’s Scheduled Jobs feature enables automation of routine tasks. To create a scheduled job that runs a backup script daily at 2 AM, you can use:
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\Scripts\Backup.ps1"
$trigger = New-ScheduledTaskTrigger -At 2am -Daily
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "DailyBackup"
This snippet not only sets a schedule but also improves time management and ensures tasks are completed without manual intervention.
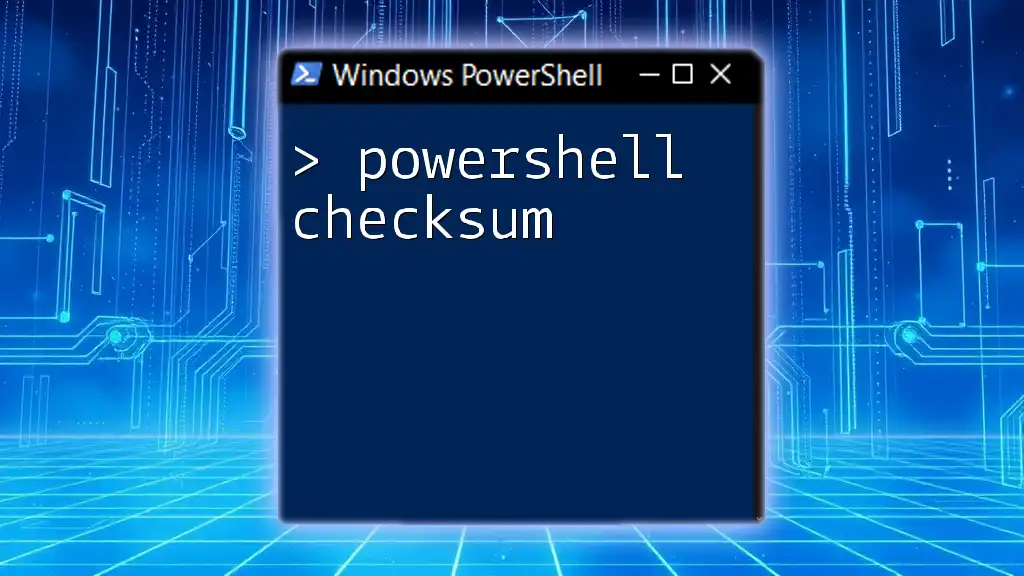
Enhancing PowerShell with GUI Tools
Introduction to GUI Interfaces for PowerShell
While PowerShell’s command-line interface is powerful, GUI tools can make it more accessible, especially for newcomers. Some popular GUI tools include PowerShell ISE, Visual Studio Code, and PowerShell Studio. They provide features like syntax highlighting, debugging tools, and integrated terminal capabilities to enhance productivity.
Creating Windows Forms with PowerShell
You can create simple GUI applications with PowerShell to gather user input or display information. Here’s a basic example of a form to receive user input:
Add-Type -AssemblyName System.Windows.Forms
$form = New-Object System.Windows.Forms.Form
$form.Text = "User Input"
$form.ShowDialog()
This command creates a Windows Form that can be customized with additional controls and events.
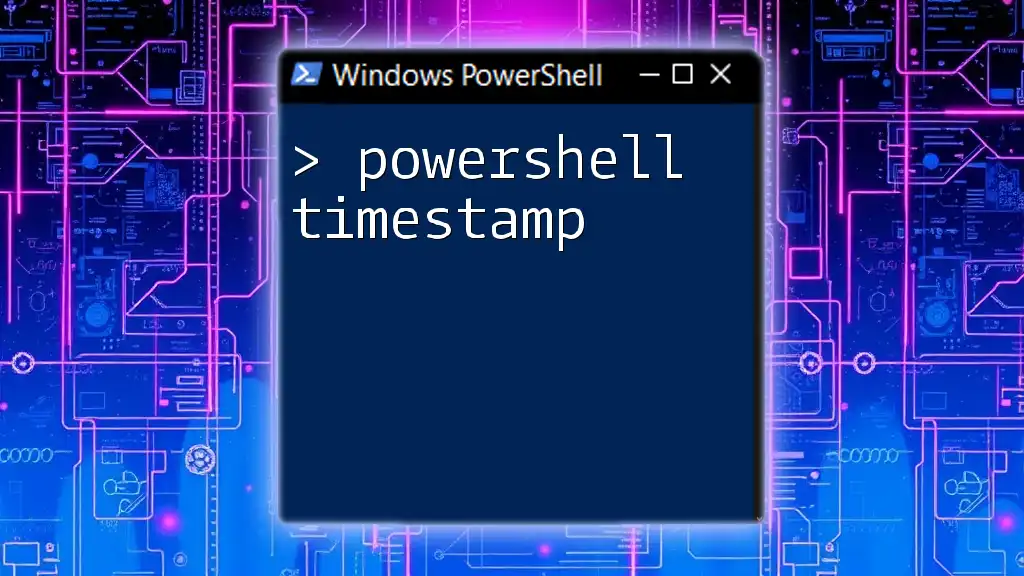
Conclusion
In this guide, you have explored a rich array of PowerShell tricks designed to enhance your efficiency and productivity. From essential command-line editing shortcuts to advanced scripting techniques like error handling and GUI creation, incorporating these tricks into your daily workflow can significantly improve your PowerShell experience. Practice these techniques, expand your knowledge, and consider joining classes or exploring more resources to master PowerShell further.