In PowerShell, the `Get-ChildItem` cmdlet is commonly used to retrieve a list of items in a specified location, such as files and directories.
Here’s a code snippet to demonstrate how to list all files and folders in the current directory:
Get-ChildItem
Understanding Lists in PowerShell
What is a List?
In programming, a list is a collection of elements that can store various data types. Lists provide developers with a flexible way to work with groups of data. In PowerShell, lists can enhance your scripting capabilities through a structured way to manage sequences of items.
Types of Lists in PowerShell
PowerShell provides different types of lists that cater to various needs. The primary types are:
Arrays: These are fixed-size collections of elements. Once you create an array, the size cannot be changed. Arrays allow you to store multiple items in a single variable.
Example of creating an array:
$myArray = @(1, 2, 3, 4, 5)
ArrayLists: Unlike arrays, ArrayLists are dynamic. They can grow or shrink in size, making them particularly useful when the number of elements isn't known ahead of time.
Example of using ArrayLists:
[System.Collections.ArrayList]$myArrayList = New-Object System.Collections.ArrayList
$myArrayList.Add(1) | Out-Null
$myArrayList.Add(2) | Out-Null
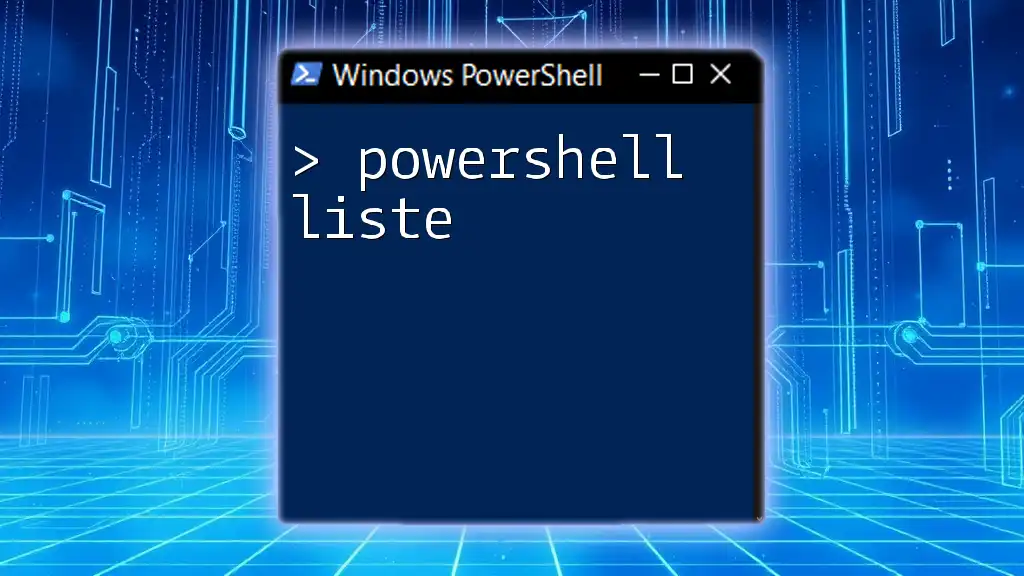
Working with Lists in PowerShell
Creating Lists
Creating lists in PowerShell is straightforward. You can initialize arrays and ArrayLists using simple syntax.
Example of creating an array of fruits:
$fruits = @('Apple', 'Banana', 'Cherry')
For an ArrayList, you can initialize it like this:
$numbers = New-Object System.Collections.ArrayList
$numbers.Add(10) | Out-Null
$numbers.Add(20) | Out-Null
Accessing List Items
Accessing elements from lists can be accomplished using index numbers. Indexes in PowerShell are zero-based; therefore, the first element in a list is at index 0.
Example showcasing index-based access:
$firstFruit = $fruits[0] # Outputs 'Apple'
You can retrieve multiple items or even slice the list, depending on your needs.
Adding and Removing Items
Adding Items
Adding items to lists is simple. With arrays, you can use the `+=` operator to append new items. However, this creates a new array each time, which can lead to performance issues in larger datasets.
Example of adding an item to an array:
$fruits += 'Date' # This re-creates the array to include 'Date'
For ArrayLists, use the `Add` method:
$myArrayList.Add('Elderberry') | Out-Null # Efficiently adds 'Elderberry'
Removing Items
To remove items efficiently, you can filter the array (which creates a new array) or utilize the `Remove` method for ArrayLists.
Example demonstrating removal from an array:
$fruits = $fruits | Where-Object {$_ -ne 'Banana'} # Removes 'Banana'
For ArrayLists, removing specific items can be done as follows:
$myArrayList.Remove(20) # Removes the first occurrence of '20'
Iterating Through Lists
Iterating through list items is essential for data manipulation. You can use loops to process each item.
Example using `foreach`:
foreach ($fruit in $fruits) {
Write-Output $fruit
}
This will output each fruit in the `$fruits` list. Other loops, such as `for`, can be similarly used, offering flexibility based on your preferences.
Filtering Lists
Filtering allows you to create a subset of your list based on specific criteria. PowerShell's `Where-Object` cmdlet is perfect for this.
Example:
$filteredFruits = $fruits | Where-Object {$_ -like 'A*'} # Outputs fruits starting with 'A'
This operation effectively narrows down the list to those elements that meet your filtering conditions.
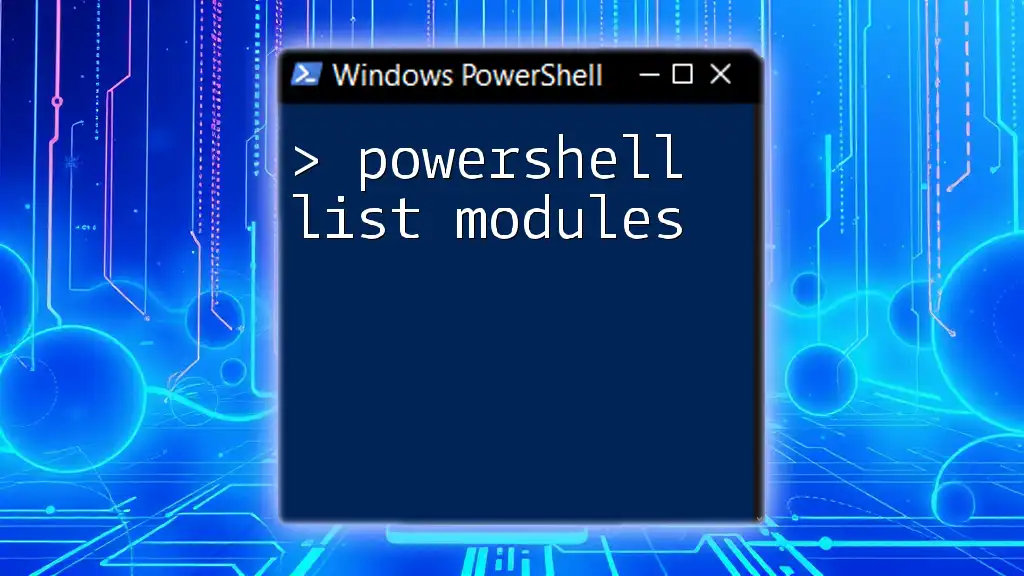
Advanced List Operations
Sorting Lists
Sorting arrays or ArrayLists can be done with the built-in `Sort-Object` cmdlet, which organizes elements in ascending or descending order.
Example of sorting an array:
$sortedFruits = $fruits | Sort-Object
With this, `$sortedFruits` will contain the fruits arranged alphabetically.
Converting and Exporting Lists
In certain situations, you may want to convert lists into other formats, like CSV for easier analysis or sharing.
Example of exporting an array to CSV:
$fruits | Export-Csv -Path "fruits.csv" -NoTypeInformation
This command will create a CSV file containing your list of fruits, making it accessible for spreadsheet software.
Merging Lists
Combining multiple lists can be done seamlessly in PowerShell. This is particularly useful when aggregating data from different sources.
Example of merging arrays:
$moreFruits = @('Fig', 'Grape')
$allFruits = $fruits + $moreFruits # Combines $fruits and $moreFruits
Now, `$allFruits` will contain items from both arrays.
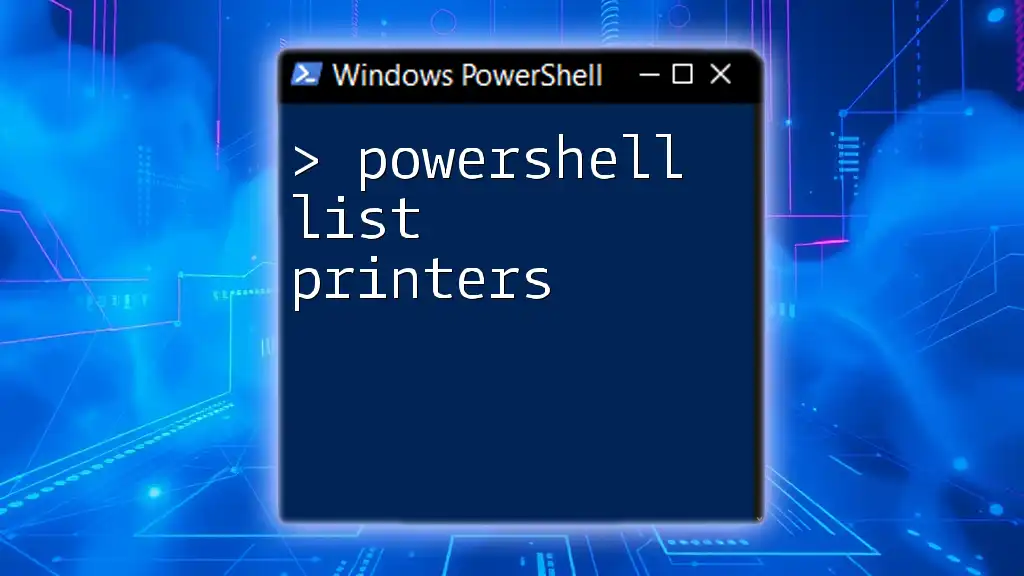
Conclusion
In this guide, we explored the concept of the PowerShell list and its various forms—arrays and ArrayLists. Understanding how to create, access, manipulate, and iterate through lists will significantly boost your efficiency with PowerShell scripts. Remember, lists are not just collections of data; they are powerful tools that elevate your programming capabilities.
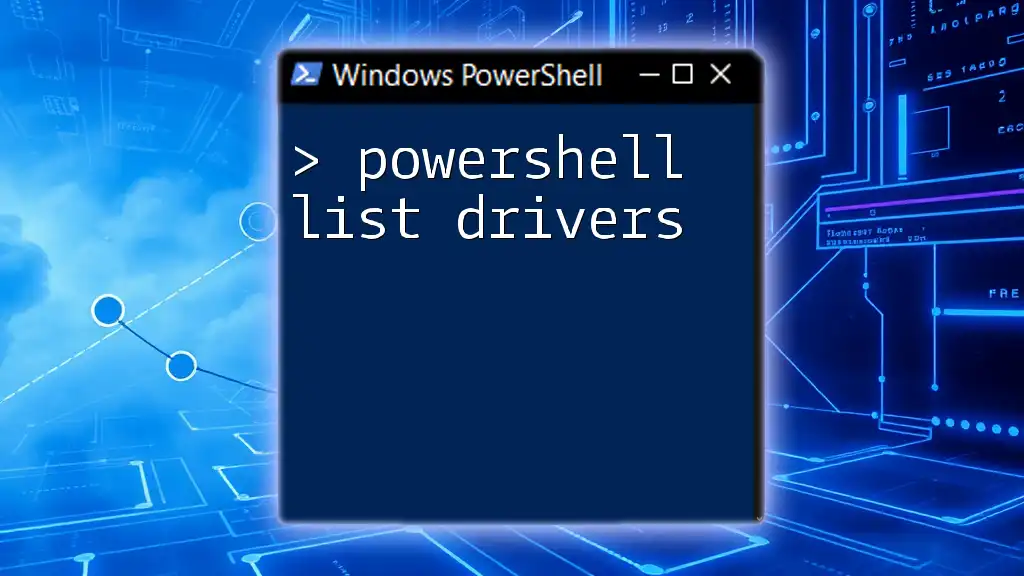
Additional Resources
For those looking to deepen their knowledge, consider exploring renowned books on PowerShell, engaging in online tutorials, or visiting community forums where you can share experiences and learn from others.
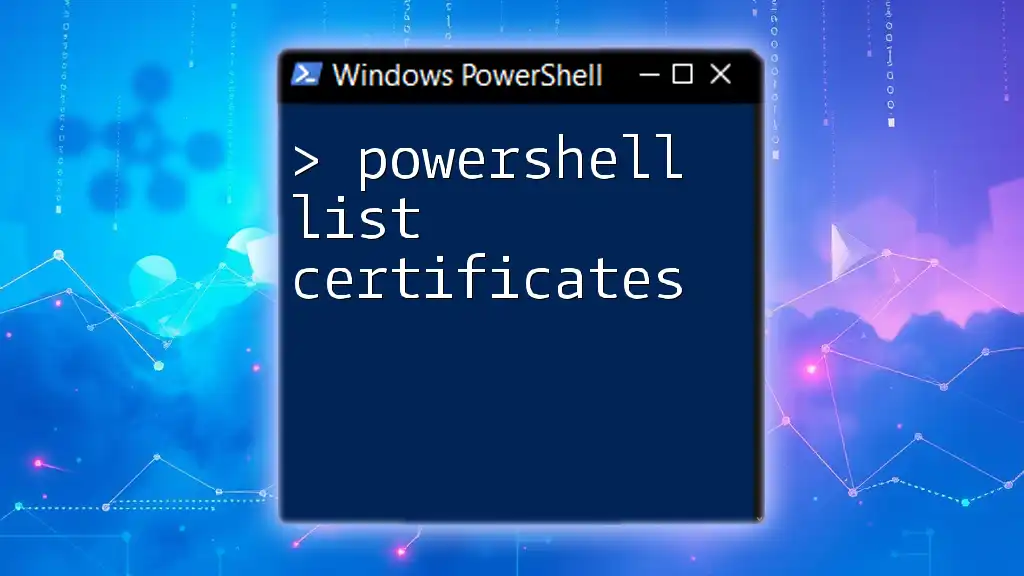
FAQs
What is the difference between an Array and an ArrayList? An array is a fixed-size collection that cannot be altered once created. An ArrayList is dynamic, allowing for easier manipulation of its size.
How can I check if a list is empty? You can check if a list is empty by evaluating its count:
if ($fruits.Count -eq 0) {
Write-Output "The list is empty."
}
Can I create a nested list in PowerShell? Yes, you can create lists within lists. For instance:
$nestedList = @(@('A', 'B'), @('C', 'D'))
This example demonstrates how lists can be structured to accommodate more complex data setups.