In PowerShell, you can listen on a specific port using the `New-Object` cmdlet combined with `System.Net.Sockets.TcpListener` to create a TCP listener.
$listener = New-Object System.Net.Sockets.TcpListener(8080); $listener.Start(); Write-Host 'Listening on port 8080...'
Understanding Ports
What is a Port?
In network communications, a port serves as a communication endpoint. Think of a port as a specific channel through which data can flow. Ports can be classified into two main types: TCP (Transmission Control Protocol) and UDP (User Datagram Protocol).
- TCP ports are connection-oriented, ensuring that data is reliably delivered in the correct sequence.
- UDP ports, on the other hand, are connectionless and may not guarantee delivery or order, making them suitable for applications where speed is crucial, such as streaming.
Common Use Cases for Listening on Ports
Listening on a port using PowerShell can be utilized in numerous contexts:
- Remote Management: Administrators can set up listeners for managing servers and services remotely.
- Service Hosting: Developers can host applications directly on a port for client connections.
- Network Diagnostics: Technicians can create listeners to monitor incoming traffic for troubleshooting purposes.
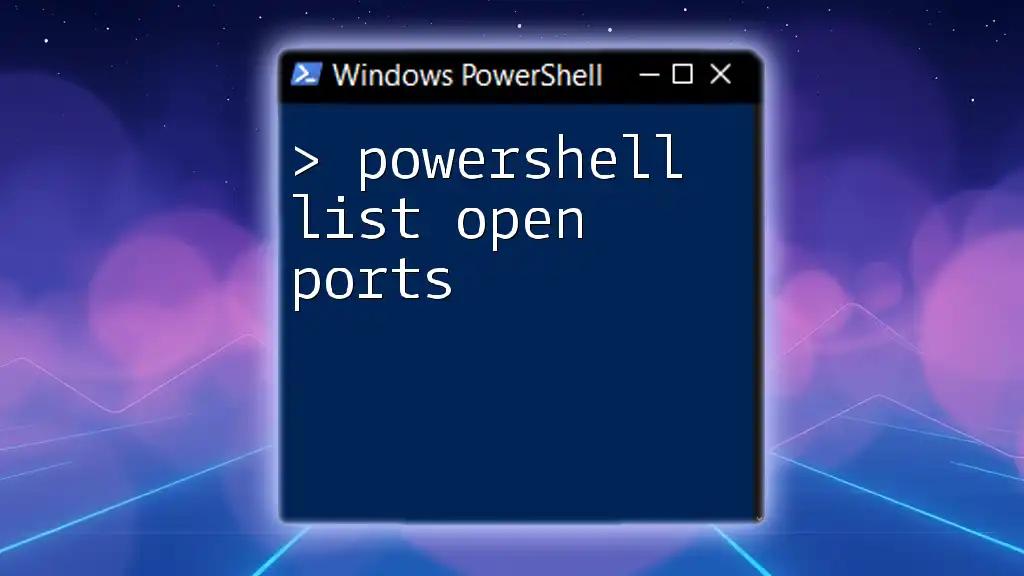
Setting Up PowerShell to Listen on a Port
Prerequisites
Before you dive into creating a PowerShell listener, ensure you meet the following conditions:
- You have PowerShell installed on your system, preferably version 5.1 or later.
- Necessary permissions to execute scripts and modify network settings.
- Familiarity with the .NET Framework, as PowerShell leverages it for socket programming.
The Basic Command Structure
The foundational command structure for PowerShell listening on a port revolves around the `TcpListener` class. The syntax can be simplified as follows:
Start-Listener -Port <PortNumber>
This command sets up a listener that awaits incoming connections on the designated port.
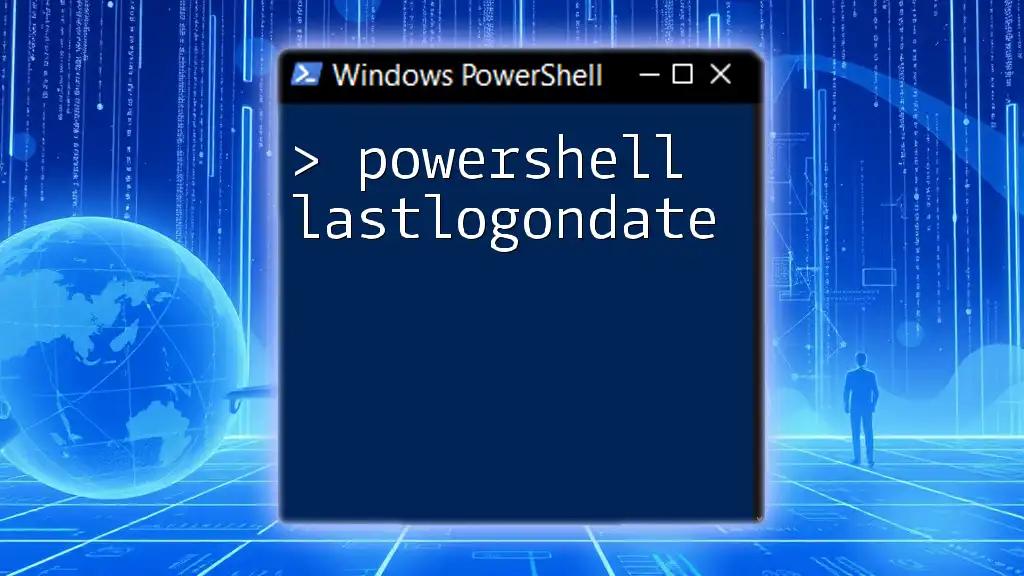
Implementing a Simple PowerShell Listener
Creating a TCP Listener
To create a basic TCP listener in PowerShell, you can utilize the following code snippet. This example listens on port 8080:
$listener = [System.Net.Sockets.TcpListener]::new(8080)
$listener.Start()
- Line 1: This line instantiates a new TcpListener object on port 8080.
- Line 2: Calling the `Start()` method begins listening for incoming connection requests on the specified port.
Accepting Connections
Once your listener is set up, the next step is to accept connections from clients. Use the following code to accomplish this:
$client = $listener.AcceptTcpClient()
$stream = $client.GetStream()
- AcceptTcpClient() creates a new TcpClient object representing the incoming connection, which you assign to the variable `$client`.
- GetStream() enables you to work with the network stream for communication (reading data, writing data, etc.).

Listening with Advanced Features
Handling Multiple Connections
In real-world applications, you may need to handle multiple clients simultaneously. You can achieve this by employing threads for asynchronous connections. Below is an example of how to manage multiple connections:
while ($true) {
$client = $listener.AcceptTcpClient()
Start-Job -ScriptBlock {
# Handling client in a separate job
# Add your client handling logic here
}
}
- The while loop continues to listen indefinitely for new clients.
- Each time a new client connects, `Start-Job` initiates a new background process to manage that client's connection.
Logging Incoming Connections
For auditing and troubleshooting, you might want to log incoming connections. To do this, utilize the code snippet below:
$logFile = "connection_log.txt"
Add-Content $logFile ("Connection from " + $client.Client.RemoteEndPoint.ToString())
- This example creates a simple log entry with the client's endpoint information, which can help identify who connected at what time.
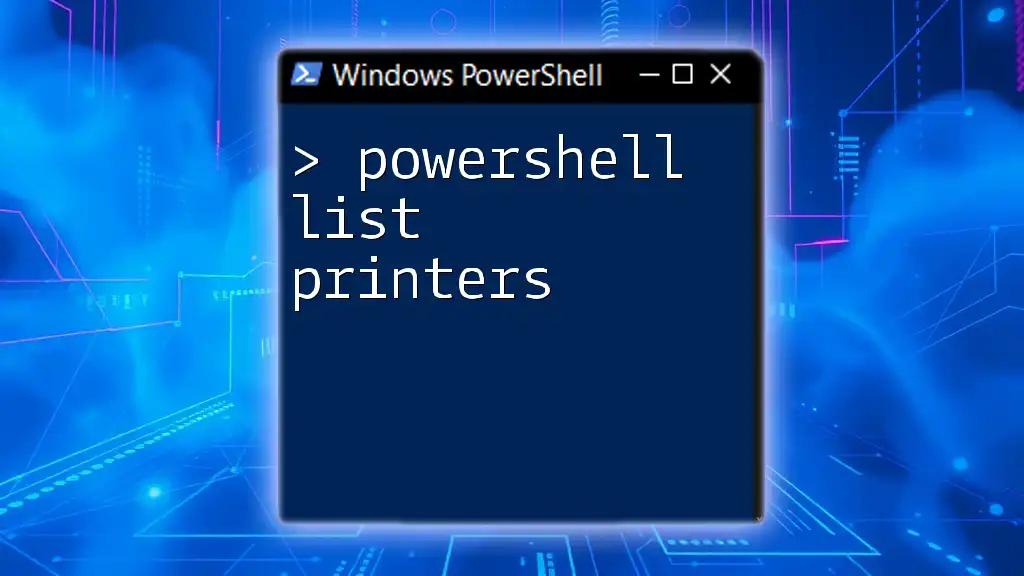
Security Considerations
Understanding Firewall and Network Settings
When allowing your PowerShell listener to accept connections, it's crucial to understand how firewalls and network settings function. Ensure that the firewall allows traffic on the specified port (e.g., port 8080). This often requires configuring exceptions for the PowerShell application.
Best Practices for Secure Listening
Listening on ports can expose your system to various risks. Here are best practices for securing your PowerShell listener:
- Implement encryption for sensitive data being transmitted.
- Validate all incoming client connections to avoid unauthorized access.
- Regularly review and update your firewall settings to prevent vulnerabilities.
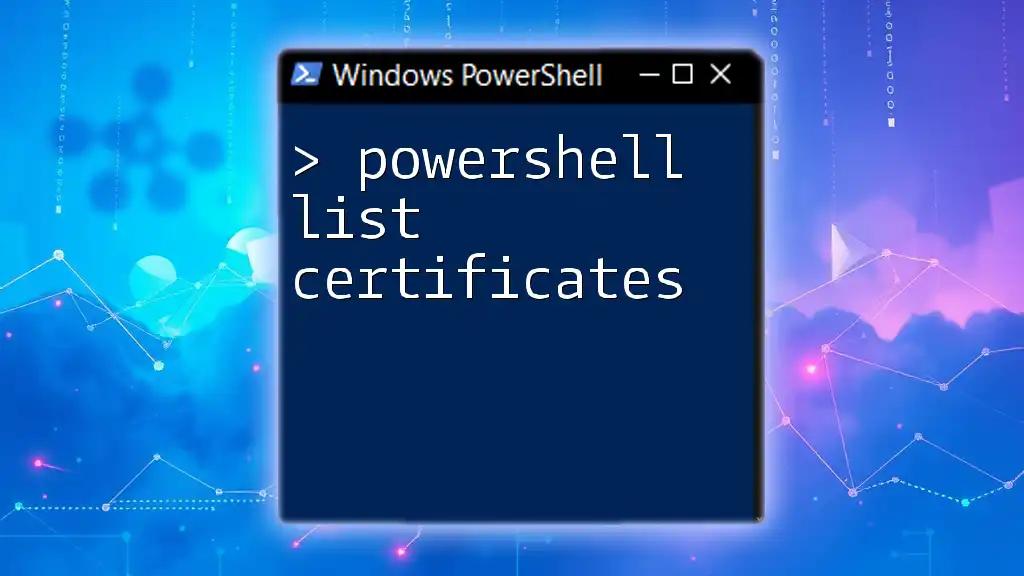
Common Troubleshooting Tips
Port Already in Use
If you encounter an error stating that the port is already in use, try the following:
- Identify the process occupying the port with:
Get-Process -Id (Get-NetTCPConnection -LocalPort 8080).OwningProcess
- You can then terminate the process or choose another port for your listener.
Connection Refused Errors
A connection refused error usually indicates that the listener is not active. Double-check that your listener is started, and the specified port is correct. If using a firewall, verify that it permits incoming connections on the given port.
Listening But Not Receiving Data
If your listener is up but not receiving data, ensure:
- The client is indeed targeting the correct port and IP address.
- Reference the logs you created to see if any connections are being established.

Conclusion
Listening on a port using PowerShell is a powerful feature that opens up numerous possibilities for remote management, service hosting, and network diagnostics. By following the guidelines provided above, you can set up a listener swiftly and securely, while also ensuring that you address common troubleshooting issues to keep your PowerShell listener running smoothly.
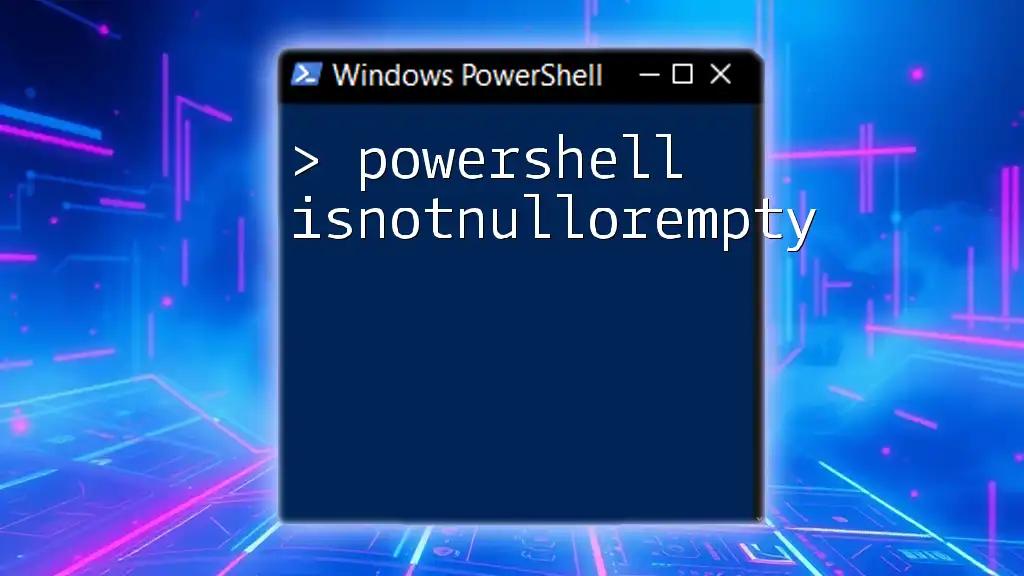
Additional Resources
For further exploration beyond this guide, consider delving into the following resources:
- Documentation on PowerShell networking capabilities.
- Tutorials on advanced socket programming with .NET.
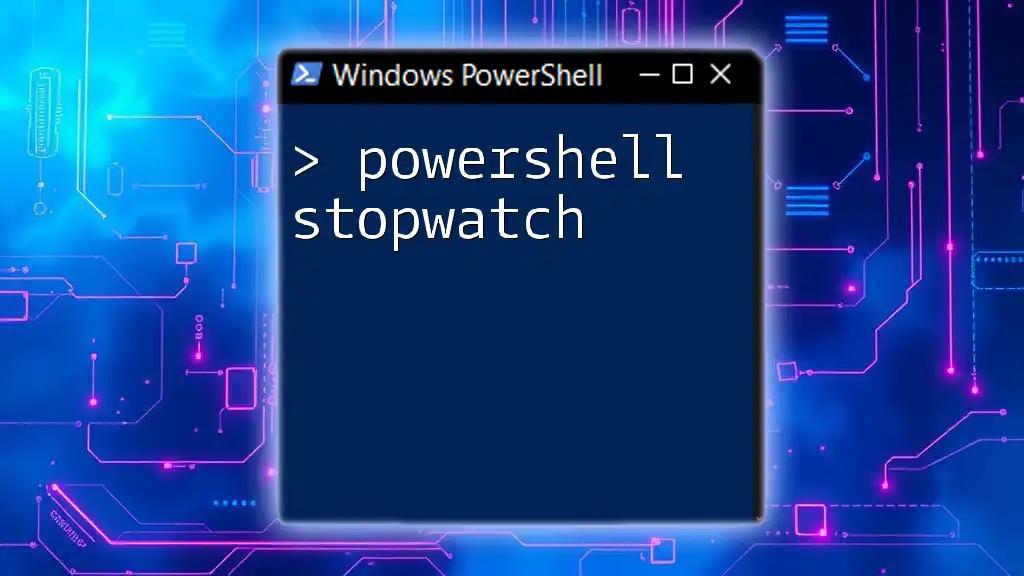
FAQs
What are some common use cases for PowerShell listeners?
PowerShell listeners are often used for remote monitoring, service management, and data collection for diagnostics among other applications.
How can I test if my PowerShell listener is working?
You can use a simple client application or a utility like `telnet` or `netcat` to attempt a connection to the specified port.
Is it safe to leave a PowerShell listener running?
While it can be safe, it's essential to employ proper security practices and monitoring to ensure that unauthorized access does not occur.