The PowerShell System Object is a foundational type in .NET that provides a wide range of methods and properties, enabling users to manipulate and interact with various data types and objects in PowerShell scripts.
$myObject = New-Object PSObject -Property @{ Name = "John Doe"; Age = 30 }
$myObject | Format-List
What is a System Object in PowerShell?
The System.Object is the fundamental building block of all objects in PowerShell. It represents the most general type in the .NET framework, serving as a parent class from which all other types are derived. Understanding System.Object is crucial because PowerShell primarily operates on objects rather than plain text. This object-oriented approach allows for powerful data manipulation and script design.

The Base Class of All Objects
As the ancestor of all .NET types, System.Object encapsulates essential functionalities that allow every object in PowerShell to inherit its methods and properties. Being an object-oriented scripting language, PowerShell emphasizes the use of these objects, ensuring a consistent and versatile scripting experience.
Characteristics of System.Object
- Inheritable: All objects can derive properties and methods from System.Object.
- Standard Methods: Includes fundamental methods like `ToString()`, `Equals()`, and `GetHashCode()` which most objects utilize.
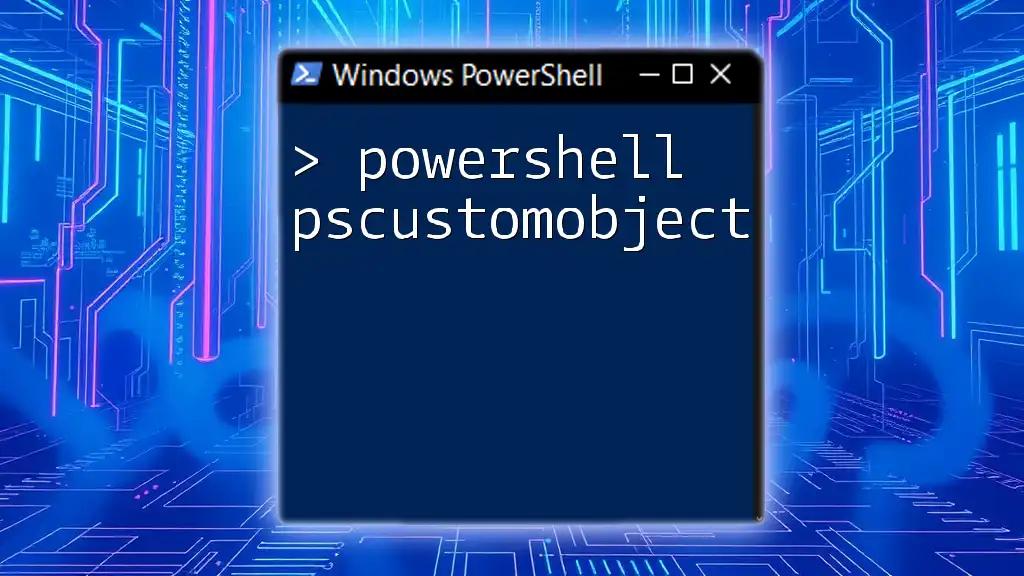
Properties and Methods of System.Object
PowerShell provides several built-in properties and methods within the System.Object class:
-
ToString(): Converts an object to its string representation, making it easier to read or display.
Example:
$stringRepresentation = $myObject.ToString()
-
Equals(): Checks if the current instance is equal to another object, allowing for robust comparison operations.
Example:
if ($myObject.Equals($anotherObject)) { Write-Host "Objects are equal." }
-
GetHashCode(): Generates a hash code for the object, useful in scenarios involving hash tables and unique identification.

Creating Custom Objects in PowerShell
PowerShell provides two primary ways to create custom objects: using the New-Object cmdlet and leveraging PSCustomObject.
Using the New-Object Cmdlet
The `New-Object` cmdlet creates a new instance of an object. This method allows for versatile object creation with specified properties.
Example:
$myObject = New-Object PSObject -Property @{
Name = "Example"
Age = 25
}
Here, a new System.Object is created with properties `Name` and `Age`.
Using PSCustomObject
PSCustomObject is a more optimized way to create objects, providing clearer syntax and better performance. This method is recommended for most scenarios.
Example:
$myCustomObject = [PSCustomObject]@{
Name = "Example"
Age = 25
}
Using PSCustomObject allows you to work with named properties directly and is particularly useful for creating structured data.
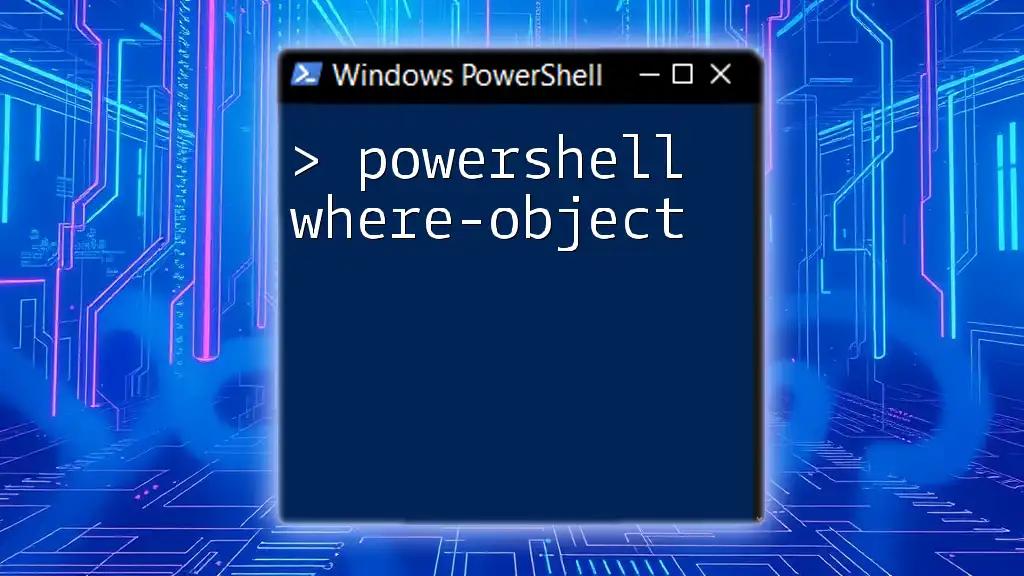
Practical Examples of System.Object
Working with System.Objects involves accessing their properties and invoking their methods.
Accessing Properties of System.Object
You can easily access properties set during object creation:
Example:
$myObject.Name
This retrieves the value of the `Name` property from the `$myObject` instance.
Using Methods of System.Object
Methods such as `ToString()` are invaluable when you need to view or log object data.
Example:
$customString = $myCustomObject.ToString()
Write-Host "Custom Object: $customString"
This utilizes the `ToString()` method to convert the object into a legible string format.
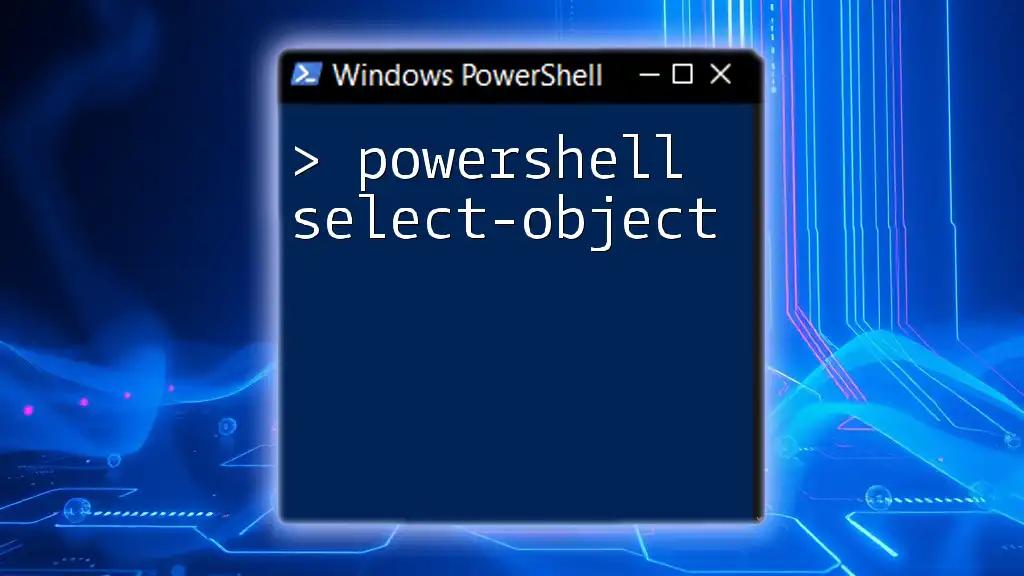
Working with Collections of System.Objects
In many cases, you’ll need to work with multiple objects collectively.
Creating and Managing Arrays of Objects
You can create arrays to hold multiple System.Objects, allowing for complex data handling.
Example:
$arrayOfObjects = @($myObject, $myCustomObject)
Once you have an array, you can iterate through its elements.
Example:
foreach ($obj in $arrayOfObjects) {
Write-Output $obj.Name
}
This loop will print the `Name` property of each object in the array.

Leveraging System.Object in Function Creation
Functions are essential for encapsulating reusable code. You can create functions that utilize System.Objects effectively.
Defining Functions that Return System.Objects
Creating a function that returns a custom System.Object is straightforward and enhances code organization.
Example:
function Create-User {
param (
[string]$Name,
[int]$Age
)
return [PSCustomObject]@{ Name = $Name; Age = $Age }
}
With this function, you can create user objects simply by calling `Create-User` with desired parameters.

Debugging and Working with System.Object
To explore the details of the objects you create, utilize the `Get-Member` cmdlet. This cmdlet reveals the properties and methods available to a given object.
Using Get-Member to Explore Objects
Example:
$myCustomObject | Get-Member
The output will show you all the properties and methods associated with `$myCustomObject`, providing insight into its structure.
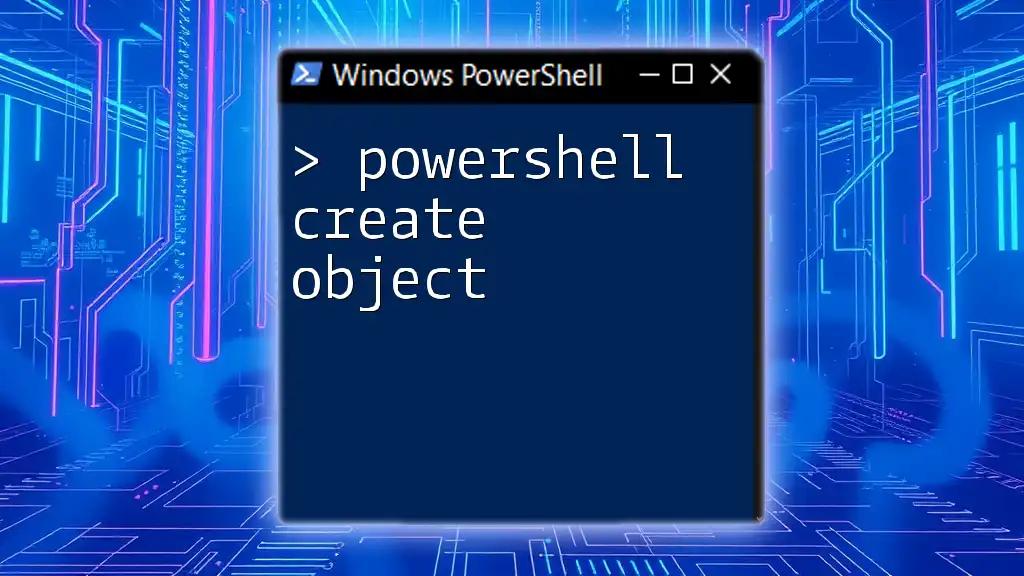
Best Practices When Working with System.Object
Choosing between System.Object and PSCustomObject can dictate your script's performance and clarity.
Choosing Between System.Object and PSCustomObject
While System.Object can be effective, PSCustomObject offers clearer syntax and better performance for custom structures. For general use, prefer PSCustomObject wherever applicable.
Tips for Efficient Object Manipulation
- Organize Properties: Keep property names clear and contextually relevant to maintain readability.
- Ensure Scalability: As your scripts grow, maintaining a coherent object structure is critical.

Conclusion
Understanding the PowerShell System Object is essential for harnessing the full power of PowerShell scripting. From creating and manipulating custom objects to leveraging their properties and methods, mastering System.Object unlocks a new level of scripting capability.
Aim to practice these concepts consistently, as the more comfortable you become with objects, the more efficient and effective your scripts will be. By integrating these methods into your daily tasks, you’ll be well on your way to becoming a PowerShell expert.
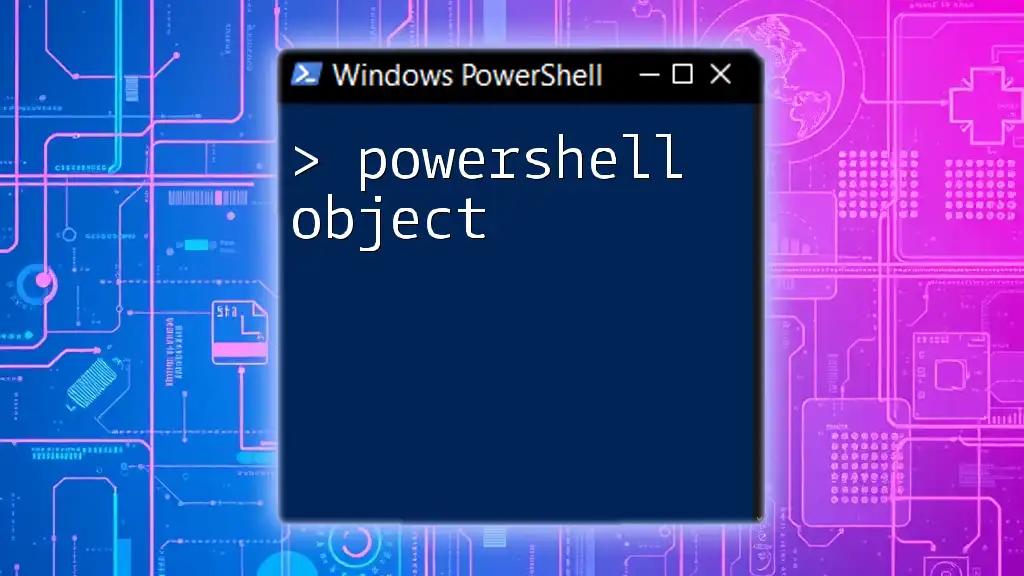
Additional Resources
For further learning, consult the official PowerShell documentation and explore recommended tutorials and learning platforms that focus on object manipulation within PowerShell.
FAQs
Common questions about System.Object often arise during the learning process. Engage in forums and community discussions to clarify concepts, troubleshoot issues, and share your experiences with other learners.
This detailed guide covers the essentials of the PowerShell System Object and provides a structured approach to learning and applying object-oriented principles in your scripts.