The `Get-ADObject` cmdlet in PowerShell is used to retrieve Active Directory objects, allowing administrators to query and manipulate AD data efficiently.
Here’s a code snippet to demonstrate its usage:
Get-ADObject -Filter * -Property Name | Select-Object Name
This command fetches all Active Directory objects and displays their names.
Understanding Active Directory Objects
What is Active Directory?
Active Directory (AD) is a directory service developed by Microsoft. It plays a vital role in managing networks and user accounts in Windows environments. AD stores information about members of the domain, including users, groups, computers, and other resources. This centralized management makes it easier to secure resources and facilitate user authentication and authorization.
Types of Active Directory Objects
There are several types of objects that can be managed within Active Directory:
-
Users: These are accounts representing individual users. Each user account includes various attributes such as username, password, and roles, which define the user’s permissions on the network.
-
Groups: Groups are collections of user accounts that can be managed as a single unit. There are two main types of groups:
- Security Groups: Used to assign permissions to resources.
- Distribution Groups: Primarily used for email distribution lists and not for security permissions.
-
Computers: Each computer in the domain is represented as an object in AD, which is essential for network management and security protocols.
-
Organizational Units (OUs): OUs are containers that help organize users, groups, and computers into a hierarchical structure, making it easier to manage policies and permissions.
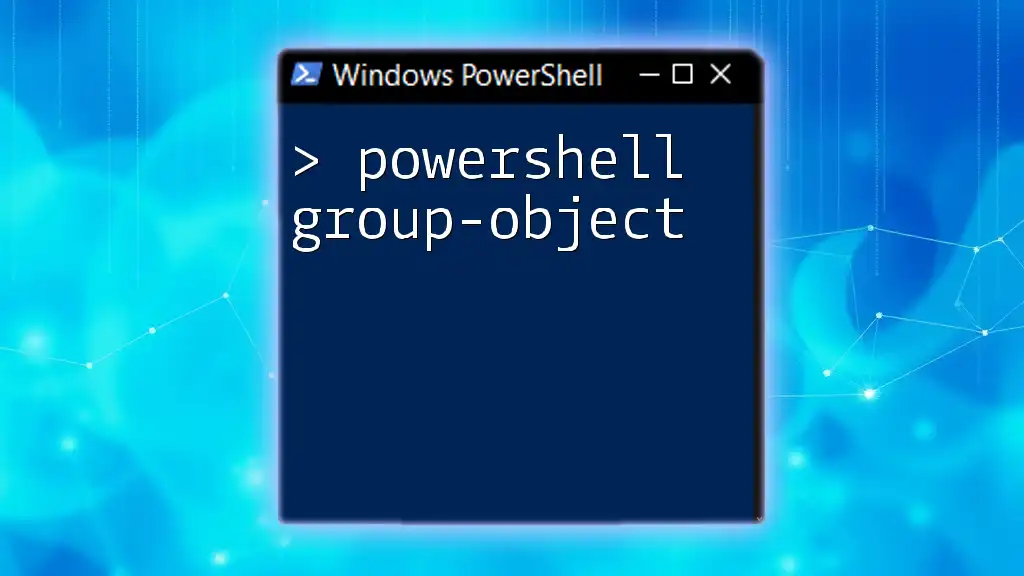
What is Get-ADObject?
Definition and Purpose
`Get-ADObject` is a powerful cmdlet in PowerShell that retrieves objects from Active Directory. It allows administrators to query and operate on a wider range of AD objects compared to more specialized cmdlets like `Get-ADUser` or `Get-ADGroup`. By utilizing this cmdlet, users can access information about resources, making it essential for anyone working in systems administration.
Syntax of Get-ADObject
The basic syntax for `Get-ADObject` is as follows:
Get-ADObject [-Identity] <ADObject> [-Properties <String[]>] [-Filter <String>]
- Identity: Identifies the AD object being retrieved.
- Properties: Specifies which properties of the object to return.
- Filter: Retrieves objects based on specific criteria.
Understanding the correct usage of parameters is crucial for effectively employing `Get-ADObject` in real-world scenarios.
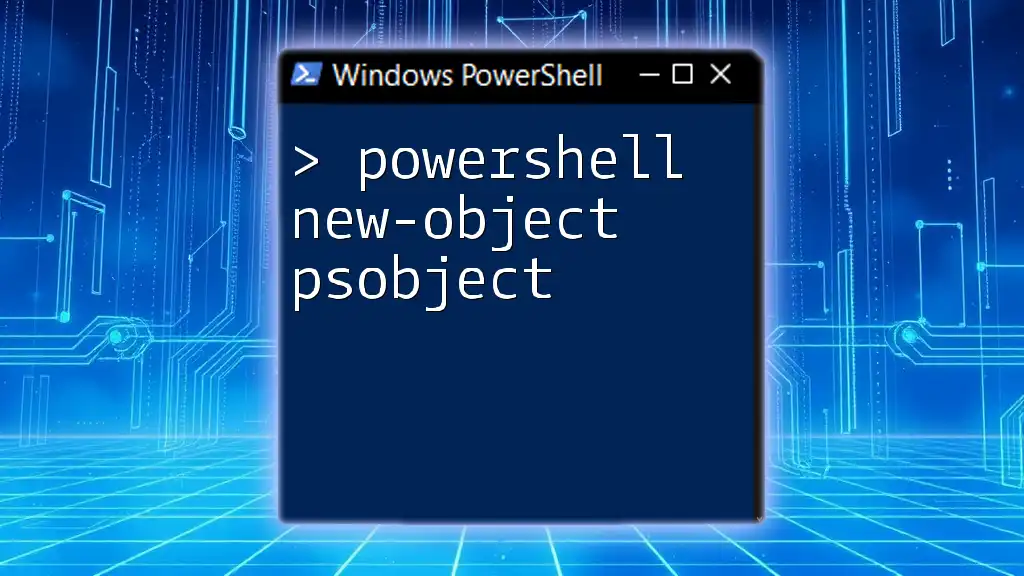
Using Get-ADObject in Practice
Retrieving All AD Objects
To retrieve all Active Directory objects, simply use:
Get-ADObject -Filter *
This command will return every object in the Active Directory, displaying a variety of properties such as names and distinguished names for each object.
Filtering Results
Using the Filter Parameter
The `-Filter` parameter allows administrators to narrow down their search for specific objects. An example filtering for user accounts that include "Admin" in their name would look like this:
Get-ADObject -Filter {Name -like "*Admin*"}
This command retrieves only those objects whose names match the specified criteria, greatly reducing the output and improving efficiency.
Using the LDAP Filter Syntax
The `-LDAPFilter` parameter can be leveraged to apply advanced LDAP filtering. For example, to specifically find all user objects, you can use:
Get-ADObject -LDAPFilter "(objectClass=user)"
This example demonstrates the power of LDAP queries to fine-tune the search results by leveraging the structure of the directory.
Selecting Specific Properties
It's often beneficial to retrieve only the properties you need. The `-Properties` parameter helps in achieving this. For instance:
Get-ADObject -Filter * -Properties Name, ObjectClass, DistinguishedName
In this command, only the specified properties of all objects would be returned, thus streamlining the data you must process.
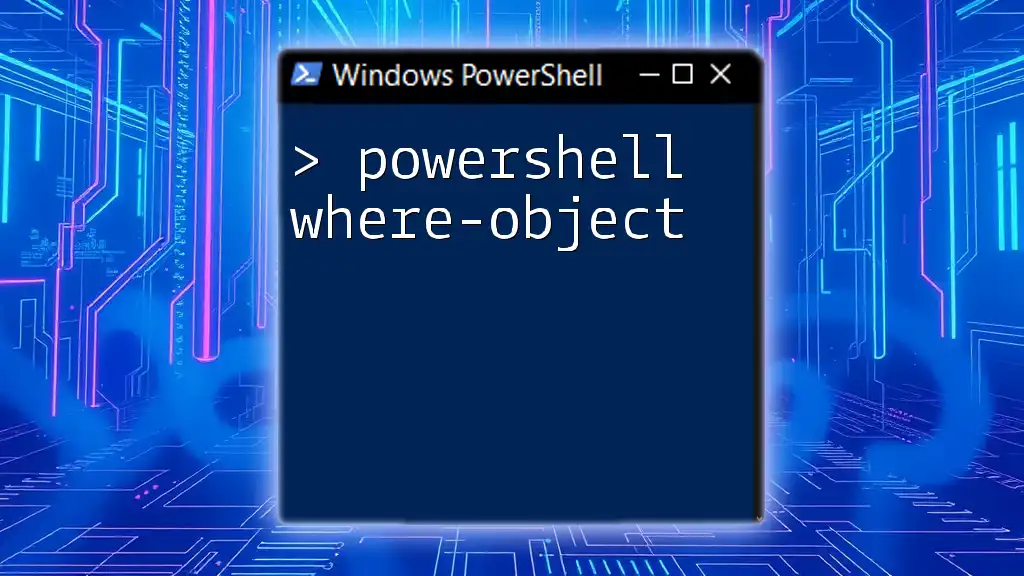
Advanced Usage of Get-ADObject
Utilizing Get-ADObject with PowerShell Pipelines
PowerShell's pipeline feature allows for combining multiple cmdlets to create more intricate commands. For example, this command retrieves all Active Directory objects and filters to display only user accounts:
Get-ADObject -Filter * | Where-Object { $_.ObjectClass -eq "user" }
This command illustrates the elasticity of PowerShell, enabling complex operations with straightforward syntax.
Combining Get-ADObject with Other Cmdlets
You can further enhance the output by integrating `Get-ADObject` with other cmdlets, such as `Select-Object`. For instance:
Get-ADObject -Filter * | Select-Object Name, ObjectClass | Sort-Object Name
This sequence filters and sorts the output by name, showcasing how you can customize data representation as needed.
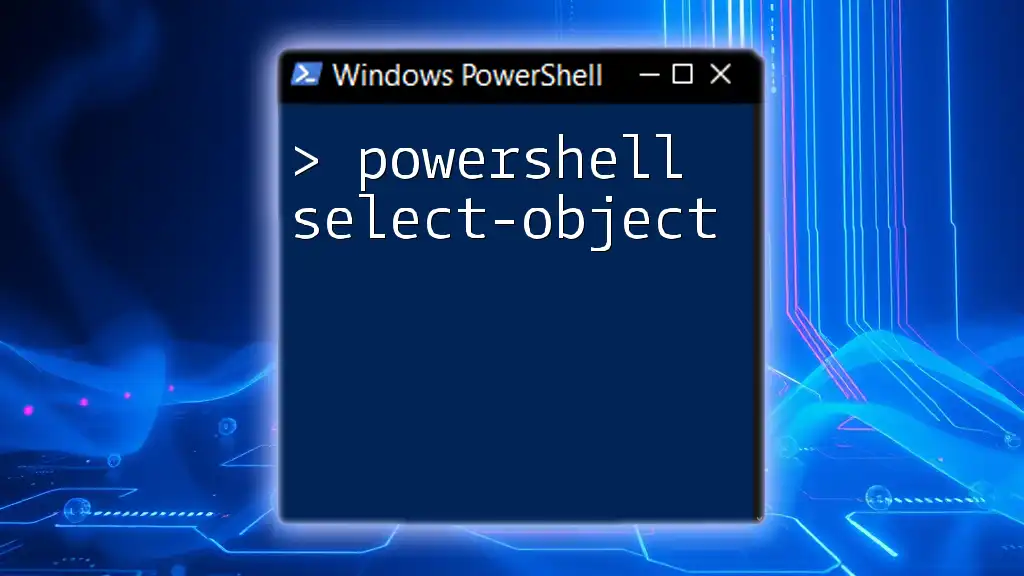
Common Use Cases
Scenario: Bulk User Removal
Sometimes, there may be a need to remove several user accounts simultaneously. Here’s how you can achieve that with `Get-ADObject`:
Get-ADObject -Filter 'Name -like "*olduser*"' | Remove-ADObject
This command identifies user accounts that match "olduser" in their names and removes them. Note: Always be cautious when executing commands that modify AD objects, and consider performing a dry run first to avoid accidental deletions.
Scenario: Reporting Active Directory Counts
If you need to run reports on the number of user accounts, use the following command:
(Get-ADObject -Filter 'ObjectClass=user').count
This provides a straightforward count of all user objects in the directory, aiding in administration and compliance.
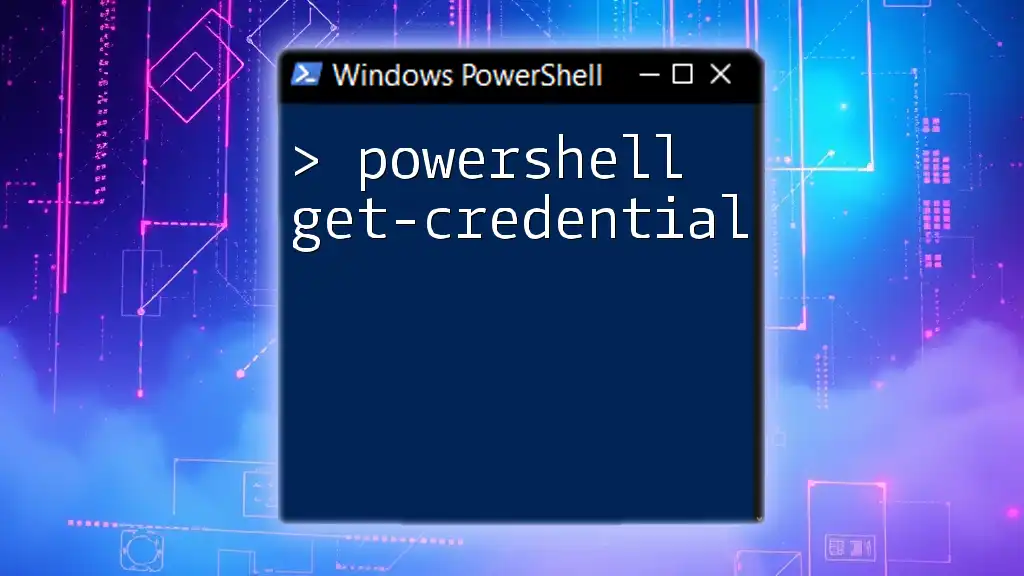
Tips and Best Practices
Ensuring Security and Permissions
When executing commands that affect Active Directory, it is essential to run them with the appropriate permissions to avoid inadvertent changes. Regularly audit and log actions for security and compliance reasons to track changes within your directory.
Performance Considerations
For organizations dealing with large Active Directory environments, performance can be a concern. Utilize the `-ResultSize` parameter to limit the number of retrieved objects. For instance:
Get-ADObject -Filter * -ResultSize 1000
This command fetches only the first 1,000 objects, thereby optimizing performance during large queries.
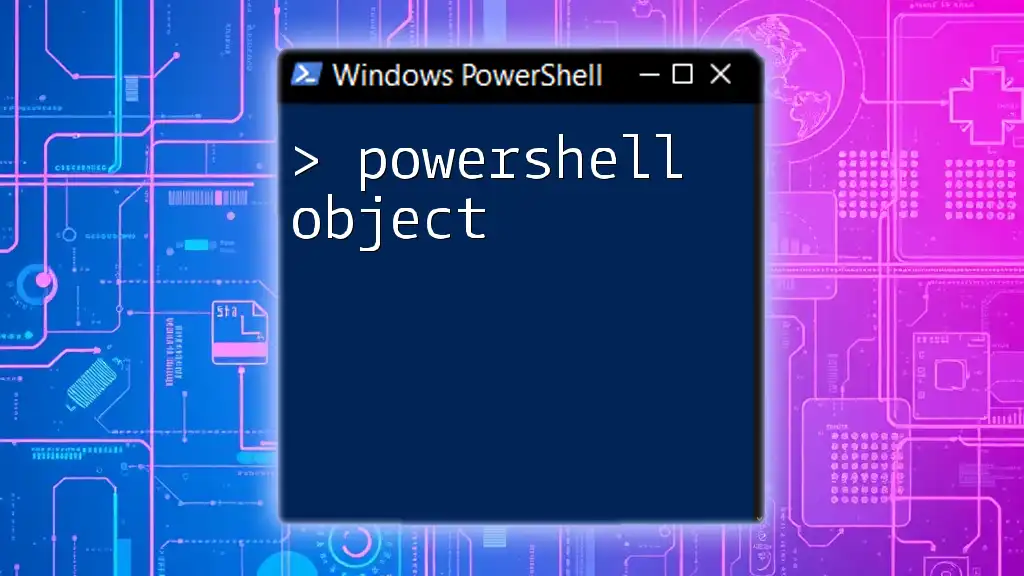
Troubleshooting Common Issues
Common Errors and Their Solutions
Users may encounter errors such as access denials or invalid identity references. Common troubleshooting steps include checking permissions, confirming the validity of object identities, and verifying Active Directory connectivity.
Debugging Tips
If you experience unexpected results or issues, leverage the `-Verbose` option for a more detailed output:
Get-ADObject -Filter * -Verbose
This will provide insights into the underlying processes, making it easier to diagnose issues.
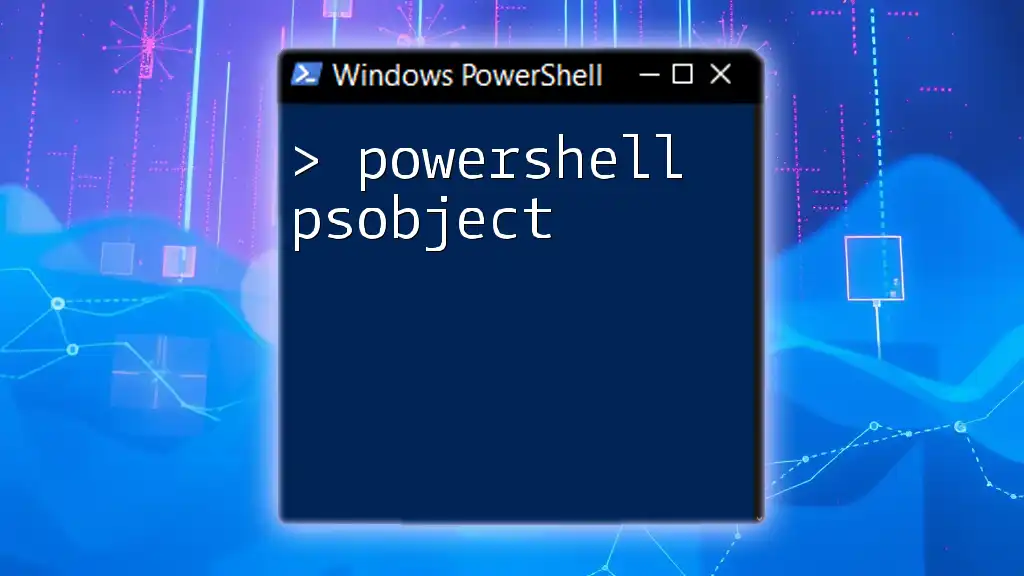
Conclusion
Throughout this guide, we've explored the `Get-ADObject` cmdlet in detail, covering its syntax, use cases, and advanced applications. By understanding how to wield this cmdlet effectively, you can streamline your Active Directory management tasks. As with all PowerShell commands, practice and experimentation will solidify your skills, enabling you to take full advantage of the capabilities offered by the `powershell get-adobject` cmdlet.
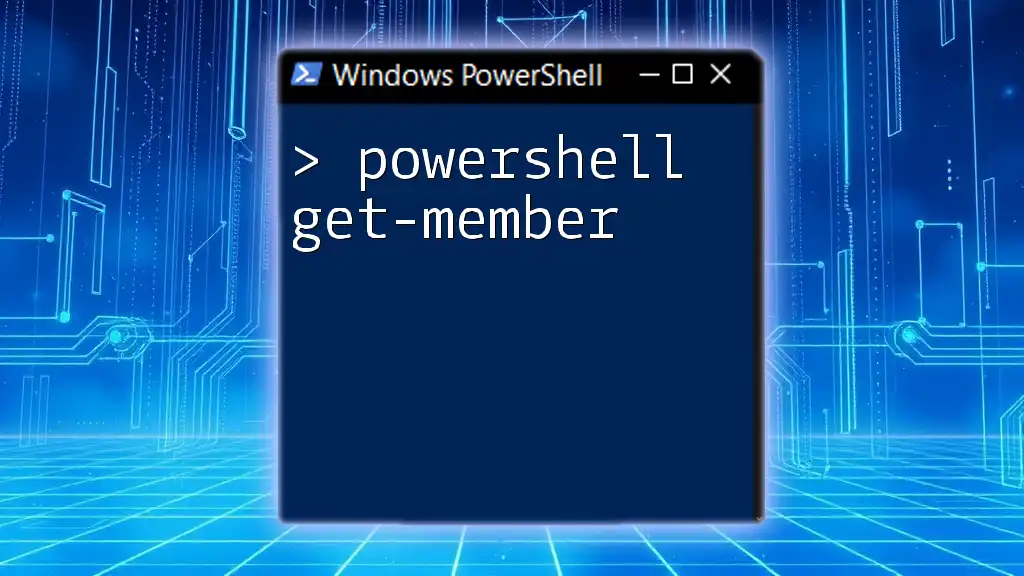
Further Reading
For additional learning, refer to the official Microsoft documentation on [Active Directory Module for Windows PowerShell](https://docs.microsoft.com/en-us/powershell/module/activedirectory/?view=windowsserver2022-ps) and explore books or online resources dedicated to PowerShell and Active Directory management.