The `Group-Object` cmdlet in PowerShell is used to group objects based on a specified property, enabling easier analysis and aggregation of data.
Here’s a simple code snippet to demonstrate its usage:
Get-Process | Group-Object -Property ProcessName
What is PowerShell Group-Object?
The `Group-Object` cmdlet in PowerShell is a powerful tool for organizing and categorizing data. Its primary function is to group the objects that are passed to it based on specified properties. This cmdlet is particularly useful in data analysis and reporting since it allows you to quickly identify patterns and trends.
For example, if you have a list of processes running on a machine, you can group them by their names, making it easier to see how many instances of each process are running.
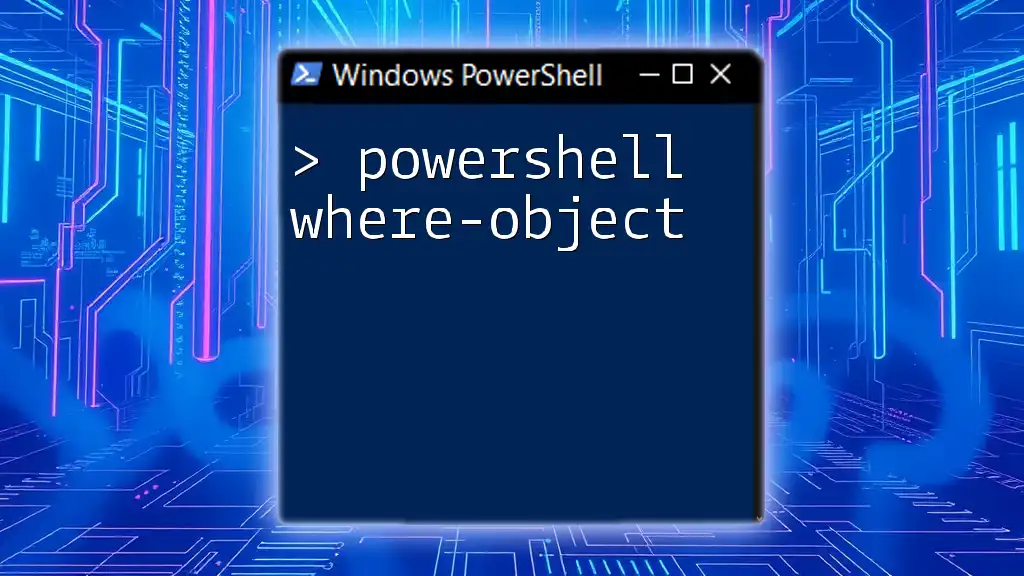
How to Use Group-Object in PowerShell
Basic Syntax
To effectively use `Group-Object`, it's important to understand its syntax. Here is the core structure:
Group-Object [-Property] <String[]> [-AsHashTable] [-NoElement] [<CommonParameters>]
- `-Property`: Specifies the property to group by.
- `-AsHashTable`: Returns the results as a hashtable.
- `-NoElement`: Excludes the grouped objects from the output.
Example: Basic Grouping
To group a list of processes by their name, you would use the cmdlet as follows:
Get-Process | Group-Object -Property ProcessName
In this example, `Get-Process` retrieves all the current processes running on the machine, and `Group-Object` organizes them by the `ProcessName`. The output will display distinct process names along with a count of how many instances are running for each name.
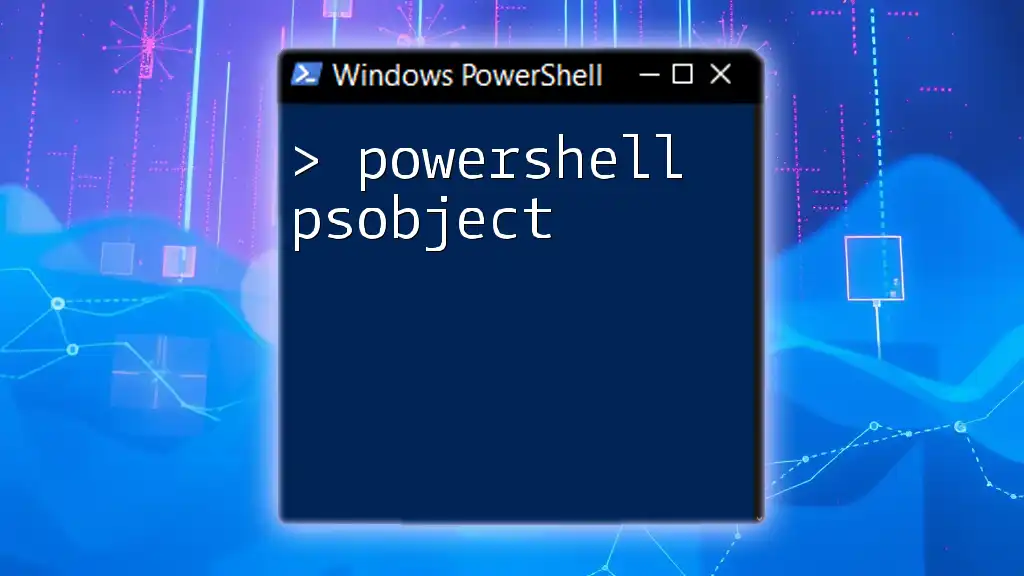
Advanced Grouping Techniques
Group-Object with Multiple Properties
Grouping by multiple properties allows you to get a more fine-tuned view of your data. For instance, if you want to group processes not only by their name but also by the machine they are running on, you can do the following:
Get-Process | Group-Object -Property ProcessName, MachineName
This command will provide output that lists each unique combination of `ProcessName` and `MachineName`, along with the number of running instances. This capability is essential when working in environments with multiple servers or systems.
Utilizing `-AsHashTable` Parameter
The `-AsHashTable` parameter can dramatically change how you work with grouped data. It simplifies the access to grouped items by returning them as a hashtable. Here’s how you can use it:
$groupedProcesses = Get-Process | Group-Object -Property ProcessName -AsHashTable
This command assigns the grouped data to the variable `$groupedProcesses`, allowing you to easily access the count of specific process names by their keys, which can be beneficial in programming scenarios where you need quick access to grouped items without looping through collections.
Using `-NoElement` for Simplified Output
If you're only interested in the count of each group and not the individual items, the `-NoElement` parameter is particularly helpful. Below is an example of how to use it:
Get-Process | Group-Object -Property ProcessName -NoElement
This command will return a table showing each distinct `ProcessName` and its count, without including the original `Process` objects in the output. This is useful for summary reports where detail is not required.
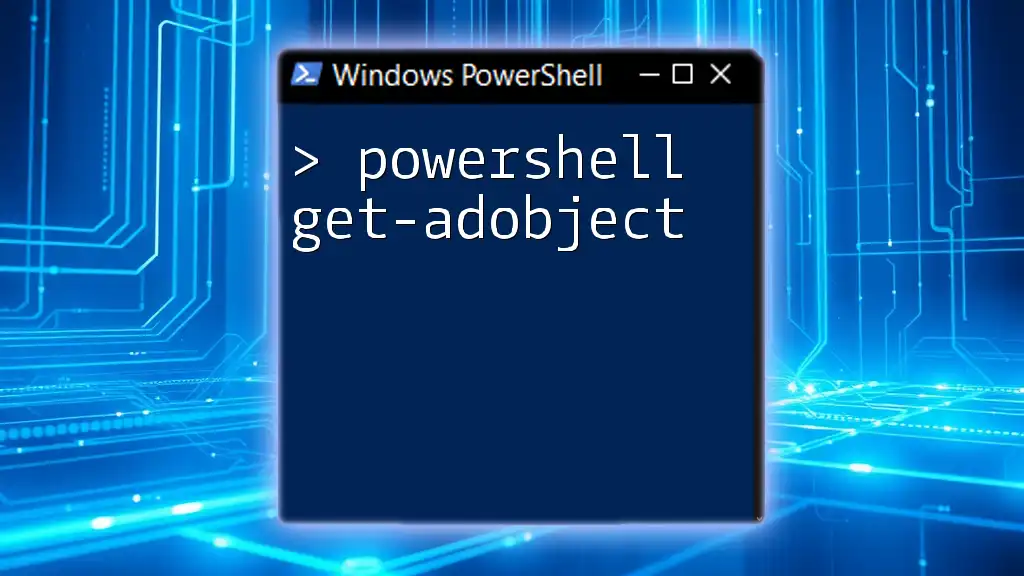
PowerShell Group By in Data Analysis
Real-World Scenarios
The practical applications of `Group-Object` are numerous, especially in fields like IT or system administration. For example, an administrator might want to analyze log files to identify how many times specific errors occur. By grouping log entries by error message, they can quickly ascertain which issues require immediate attention.
Visualizing Grouped Data
Visual representation can enhance data analysis significantly. After grouping data, you could leverage various PowerShell functions or external libraries to create visualizations based on the groups you've created. For instance:
$groupedData = Get-Service | Group-Object Status
$groupedData | ForEach-Object { $_.Count }
In this case, you can count the number of services associated with each status (e.g., Running, Stopped) and then create a visual dashboard if needed.
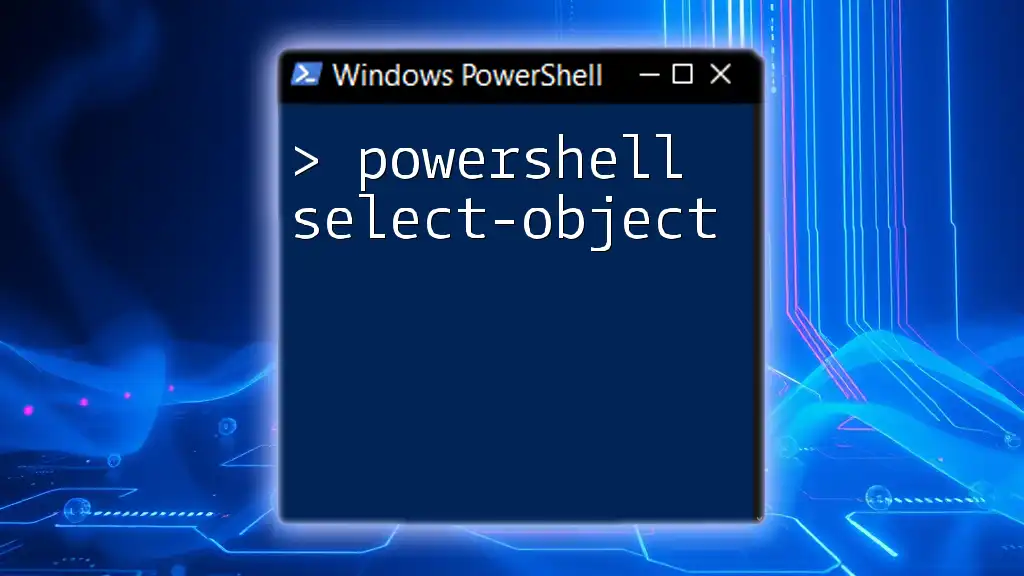
Common Issues and Troubleshooting
Performance Considerations
One caveat to keep in mind when using `Group-Object` is performance. When dealing with large datasets, consider the effect of grouping on performance and avoid excessive usage in tight loops or heavily nested commands.
Comparison with Other Cmdlets
While `Group-Object` is immensely useful, there are scenarios where other cmdlets like `Sort-Object` might be more appropriate. Use `Sort-Object` when you simply want to order objects rather than group them. For instance, if you need to sort processes by memory usage rather than grouping them by name, `Sort-Object` would be the cmdlet of choice.
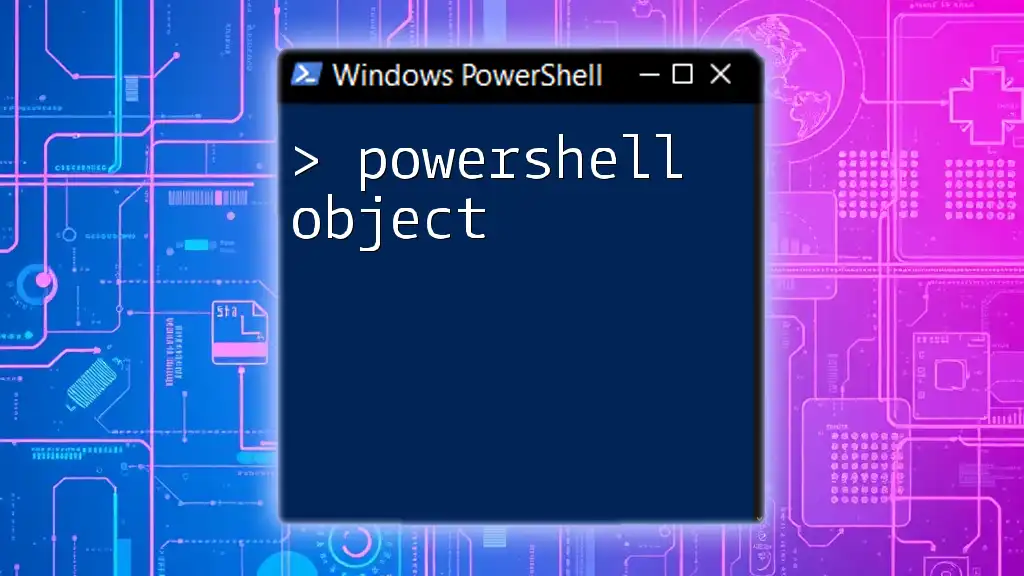
Conclusion
In summary, the `Group-Object` cmdlet is an invaluable tool for anyone looking to organize and analyze data efficiently in PowerShell. By understanding its capabilities and learning how to apply it effectively, you can significantly enhance your data manipulation skills.
Experimenting with various properties, parameters, and real-world scenarios can deepen your understanding and enable you to utilize this cmdlet to its full potential. Join our PowerShell training sessions to explore more advanced topics and enhance your PowerShell proficiency.
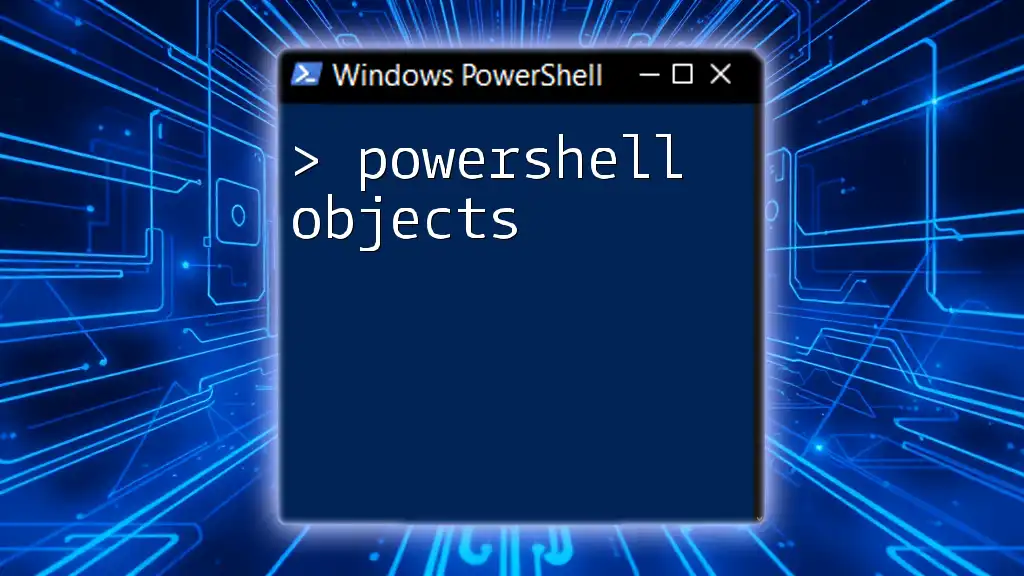
Additional Resources
For further exploration, consider diving into official PowerShell documentation, engaging with community forums, or exploring suggested readings and tutorials. Each resource offers valuable insights into mastering PowerShell and its myriad of functionalities.