The `New-Object` cmdlet in PowerShell is used to create a new instance of a .NET object, and `PSObject` is commonly utilized to create a custom object that can hold various properties and values.
Here's a code snippet demonstrating its use:
$customObject = New-Object PSObject -Property @{ Name = 'John Doe'; Age = 30; Occupation = 'Developer' }
Understanding PowerShell Objects
What is an Object?
In programming, an object is a collection of related data and functionalities. This encapsulation allows for better data management and representation within scripts and applications. PowerShell leverages the .NET framework, meaning that the objects you work with in PowerShell are essentially .NET objects, providing a robust structure to handle various data types.
The Role of PSObject in PowerShell
PSObject is a special type of object designed to hold multiple properties and methods in a way that makes scripting intuitive and flexible. Unlike standard .NET objects, a PSObject can easily be manipulated and extended at runtime.
The benefits of using PSObject include:
- Dynamic Properties: You can add and change properties on the fly, catering to different data scenarios.
- Integrated with PowerShell Pipeline: PSObject integrates seamlessly with PowerShell’s pipeline, allowing for easy manipulation of data.
- Customizable Output: You can define how the object should be displayed, making it easier to present in a user-friendly manner.
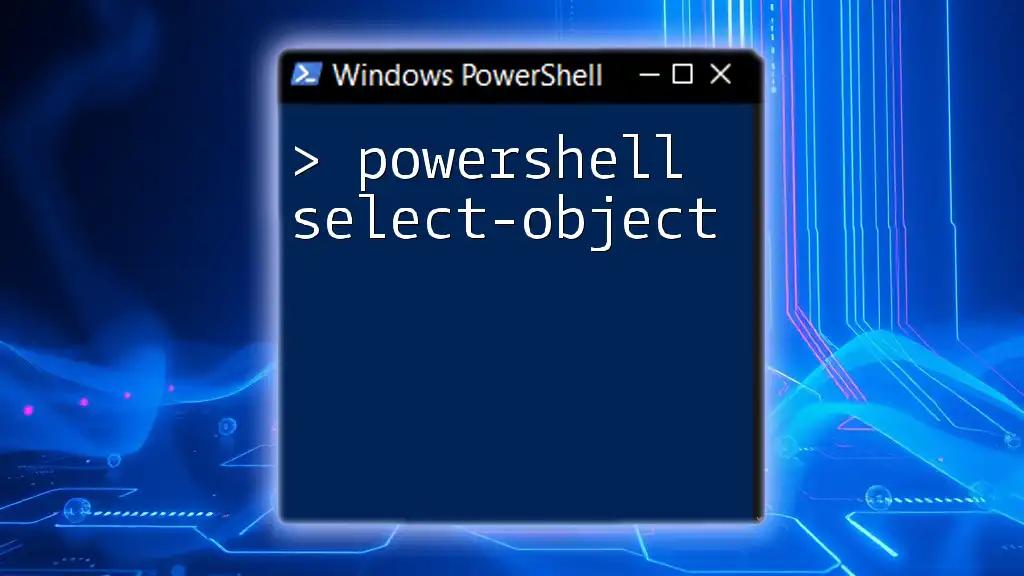
Using New-Object with PSObject
Overview of the New-Object Cmdlet
New-Object is a PowerShell cmdlet that builds new objects from .NET classes. The syntax for the `New-Object` cmdlet is straightforward:
New-Object -TypeName <TypeName> [-ArgumentList <Arguments>]
This allows you to create objects not just from built-in types but also from user-defined types, providing substantial flexibility when scripting.
Creating PSObject with New-Object
To specifically create a PSObject, you can use the `-TypeName PSObject` along with the `-Property` parameter. The following example demonstrates the creation of a simple PSObject:
$person = New-Object PSObject -Property @{
Name = "John Doe";
Age = 30;
Occupation = "Engineer"
}
In this example, we create a `PSObject` called `$person` with three properties: `Name`, `Age`, and `Occupation`.
Adding Properties to a PSObject
How to Add Properties Dynamically
You can extend an existing PSObject by adding properties dynamically using the `Add-Member` cmdlet. Here’s how to add a property to the `$person` object after its initial creation:
$person | Add-Member -MemberType NoteProperty -Name Address -Value "123 Main St"
This command dynamically adds an `Address` property to the `$person` object, demonstrating the flexibility that PSObject provides.
Modifying Existing Properties
Updating properties in PSObject is straightforward. For instance, if you want to modify the `Age` property, you can do so like this:
$person.Age = 31
This command changes the `Age` property from `30` to `31`, showcasing how easy it is to manage properties.
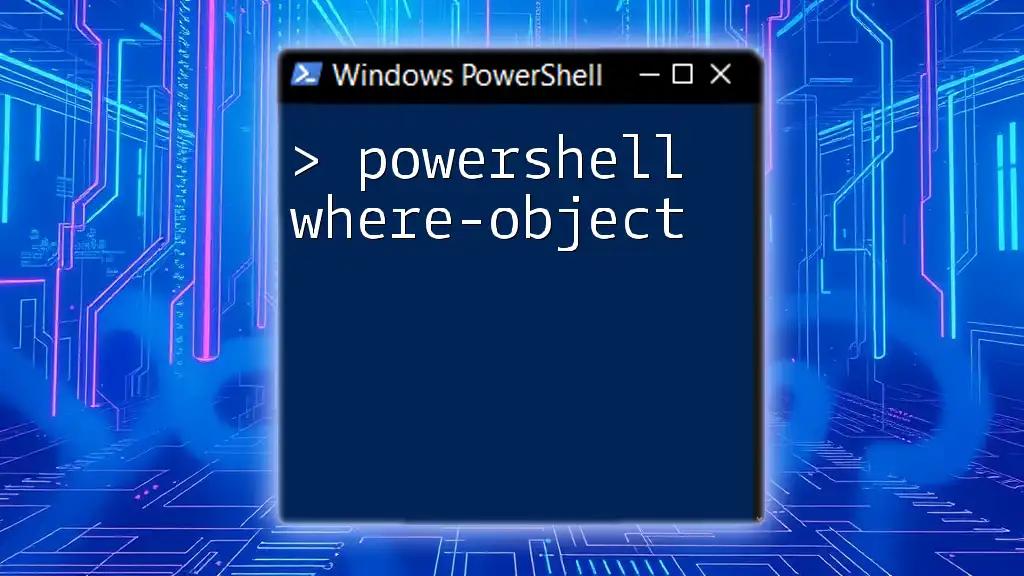
Practical Applications of PSObject
Data Representation
PSObject is frequently used for structured data representation. For example, you can create a collection of PSObjects, like so:
$people = @()
$people += New-Object PSObject -Property @{Name="Alice"; Age=28}
$people += New-Object PSObject -Property @{Name="Bob"; Age=35}
This collection of two PSObjects (`Alice` and `Bob`) can be manipulated collectively, making bulk operations easier.
Exporting PSObject to CSV
One powerful feature of PSObject is that it can be easily exported to various formats. For example, to export a collection of PSObjects to a CSV file, use the `Export-Csv` cmdlet:
$people | Export-Csv -Path "people.csv" -NoTypeInformation
This command saves the `$people` collection into a CSV file named `people.csv`, allowing you to share or analyze the data easily.

Managing PSObject Properties
Enumerating Properties
You might often need to enumerate through the properties of a PSObject. This can be achieved using the following command:
$person.PSObject.Properties
This command outputs all the properties and their current values for the `$person` object, providing a clear view of its structure.
Filtering and Sorting PSObject Collections
PowerShell makes it easy to filter and sort collections of PSObjects. For instance, you can filter the `$people` array to find those older than 30 and sort them by age:
$people | Where-Object { $_.Age -gt 30 } | Sort-Object Age
The above command filters the `$people` collection, retaining only those entries with an `Age` greater than `30` and sorting the results by age.
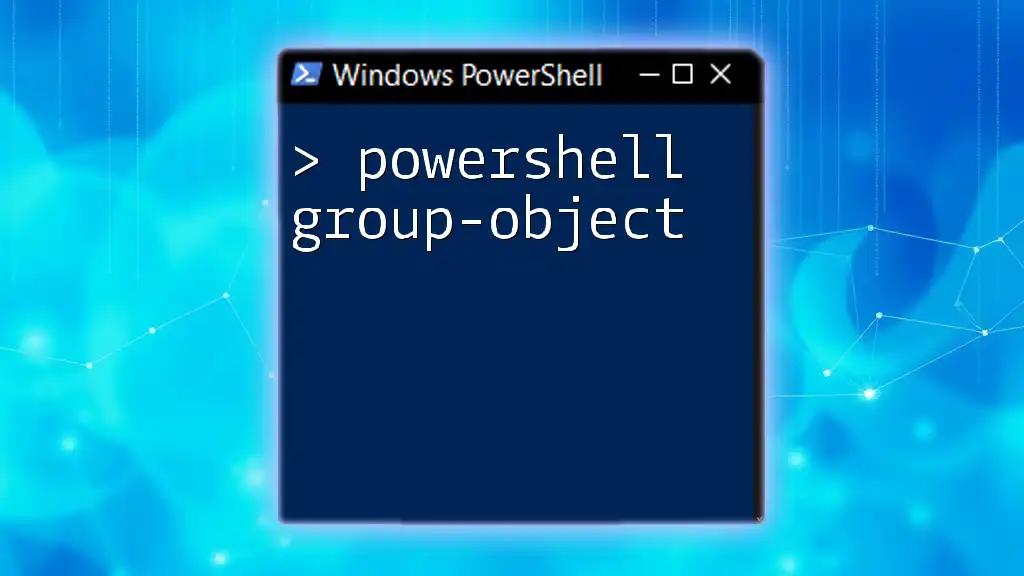
Advanced Techniques with PSObject
Working with Nested PSObjects
In more complex scenarios, you might require nested PSObjects. This is useful when you want to represent related information. Here’s how you can create a nested PSObject:
$course = New-Object PSObject -Property @{
Name = "PowerShell Basics";
Duration = "4 weeks"
}
$student = New-Object PSObject -Property @{
Name = "James";
Course = $course
}
In this example, we create a nested structure where the `$student` only has a reference to the `$course`, allowing for sophisticated data relationships.
Converting PSObject to JSON
To easily share or store data, you might need to convert PSObjects into JSON format. PowerShell makes this process seamless with the `ConvertTo-Json` cmdlet:
$json = $person | ConvertTo-Json
This command converts the `$person` object into a JSON representation, providing an easy way to format and serialize your data.
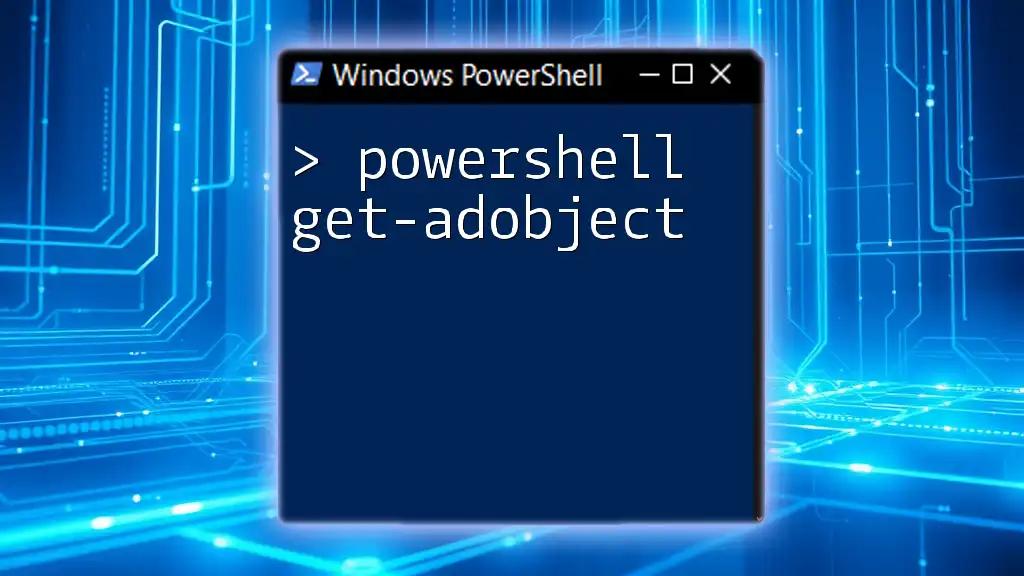
Best Practices for Using PSObject
Performance Considerations
While using PSObjects offers tremendous flexibility, it's essential to be aware of performance implications. Creating a large number of objects or overly complex hierarchies can lead to increased memory usage and slower execution times. It's beneficial to keep object structures as simple as possible while still achieving your goals.
When to Use PSObject
Using PSObject is appropriate in scenarios where you require dynamic fields or need to aggregate data types. They are particularly useful for reporting or when dealing with collections of data. However, be cautious not to use PSObjects in performance-critical applications, where static types might be more efficient.

Conclusion
In summary, the `powershell new-object psobject` command is a powerful tool in the PowerShell toolkit. It allows for the creation of flexible, dynamic objects that can be modified and extended easily to suit various scripting needs. Practicing the techniques outlined will help deepen your understanding and mastery of PowerShell scripting with PSObjects.
By exploring and implementing these concepts, you will enhance your scripting skills and unlock new potential in your PowerShell projects. Whether you're exporting data, creating nested structures, or filtering information, mastering PSObjects will significantly elevate your PowerShell workflow.