PowerShell objects are instances of classes that encapsulate data and behavior, allowing users to manipulate complex data structures and perform actions efficiently.
Here's a simple code snippet demonstrating how to create and display a PowerShell object:
$person = New-Object PSObject -Property @{ Name = 'John'; Age = 30 }
$person
What are Objects in PowerShell?
Understanding the Concept of Objects
In PowerShell, objects are a fundamental building block that represent data in a structured way. Unlike simple data types, objects can have both properties and methods. Properties are attributes associated with the object, while methods are actions that can be performed on the object. To simplify, you can think of properties as "characteristics" of the object, and methods as "actions" the object can take.
The critical distinction is that, while a value is just a single piece of data, an object is a collection of data and functionality. For instance, consider a car: its properties may include color and make, while its methods could include actions like "start" or "stop."
Types of Objects in PowerShell
PowerShell supports different types of objects:
-
Built-in Objects: These include core data types such as strings, numbers, arrays, and hashtables. They're ready-made objects that you encounter frequently when using PowerShell commands.
-
Custom Objects: These allow you to define your structure. Custom objects can be useful, especially when you want to represent complex data in a clear format. You can create custom objects using `New-Object` or create a `[PSCustomObject]` which is even simpler.
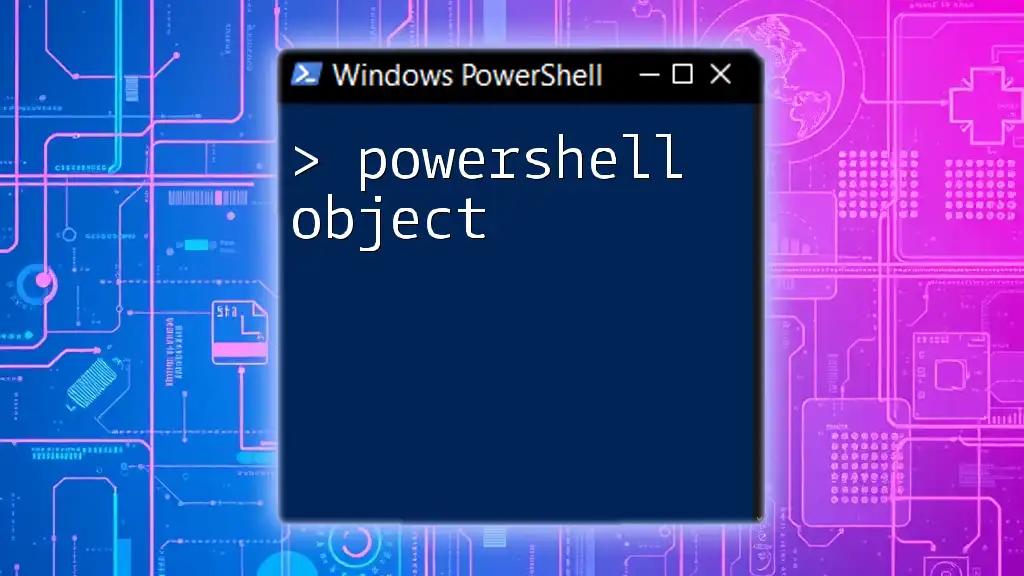
The Anatomy of a PowerShell Object
Properties of PowerShell Objects
Properties define the data held within an object. You can retrieve them to understand what information the object carries.
To access properties of an object, you can use the dot notation. For instance, if you retrieve a process object, you may want to access its name and ID. Here's how you can do that:
$process = Get-Process | Select-Object -First 1
$process.Name # Accessing the property 'Name'
$process.Id # Accessing the property 'Id'
Methods of PowerShell Objects
Methods determine what functions can be executed on an object. They often modify object properties or perform specific tasks.
For example, when generating random numbers, you might create a random number object and then call its `Next()` method like this:
$randomNumber = New-Object System.Random
$randomNumber.Next(1, 100) # Calling the method 'Next'
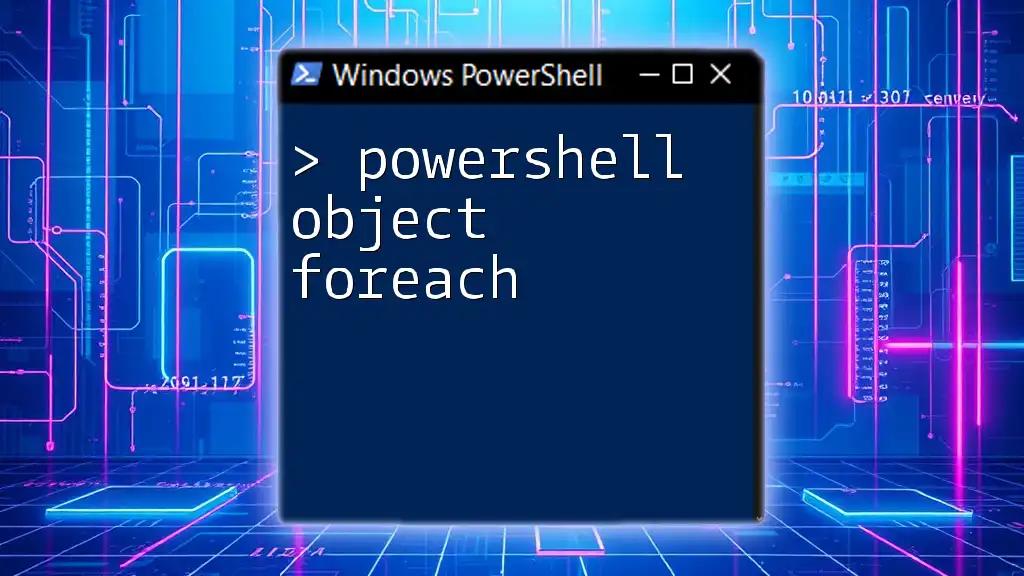
Working with PowerShell Objects
Retrieving Objects from Cmdlets
PowerShell is heavily reliant on cmdlets that return objects. Each cmdlet often produces a collection of objects that can be manipulated in the pipeline. This feature allows you to chain commands together seamlessly to process data.
Common cmdlets include `Get-Service` and `Get-Process`, which return service and process objects respectively. Understanding how to work with these returned objects is crucial for effective scripting.
Templating with Custom Objects
Creating Custom Objects
Creating custom objects makes it significantly easier to manage structured datasets. You can quickly define a new object using either `New-Object` or the more straightforward `[PSCustomObject]`. Here’s an example:
$customObject = [PSCustomObject]@{
Name = "John Doe"
Age = 30
}
Adding Properties to Custom Objects
Once you've created a custom object, you can easily add or modify its properties. This flexibility allows for dynamic data structures aligned with your needs.
$customObject.City = "New York" # Adding a property

Working with Arrays of Objects
Creating Arrays of Objects
PowerShell allows you to manage collections of objects effortlessly. By creating an array of custom objects, you can handle multiple data entries in a structured way. Here’s how to set up an array of custom objects:
$arrayOfObjects = @()
$arrayOfObjects += [PSCustomObject]@{ Name = "Alice"; Age = 25 }
$arrayOfObjects += [PSCustomObject]@{ Name = "Bob"; Age = 30 }
Iterating Through Arrays of Objects
The ability to iterate through arrays of objects allows you to perform operations on each entry. A common use case is to print the properties of each object.
foreach ($person in $arrayOfObjects) {
Write-Host "Name: $($person.Name), Age: $($person.Age)"
}

Filtering and Sorting Objects
Using Where-Object for Filtering
The `Where-Object` cmdlet is a powerful tool for filtering collections based on specified criteria. This allows you to narrow down results efficiently. For instance, if you wanted to filter adults from the previous array, you could do the following:
$adults = $arrayOfObjects | Where-Object { $_.Age -ge 18 }
Sorting Objects with Sort-Object
After filtering, you might want to sort the objects to make it easier to read or analyze the data. Using `Sort-Object`, you can sort by any property. Here’s how it can be done:
$sortedArray = $arrayOfObjects | Sort-Object Age

Commonly Used PowerShell Objects
File and Directory Objects
When working with the file system, `Get-ChildItem` is commonly used to retrieve file and directory objects. Understanding these objects helps manage files programmatically.
Network Objects
PowerShell also provides cmdlets that return network-related objects such as `Get-NetIPAddress`, which returns details about IP configurations. Working with these objects is vital for network management tasks.
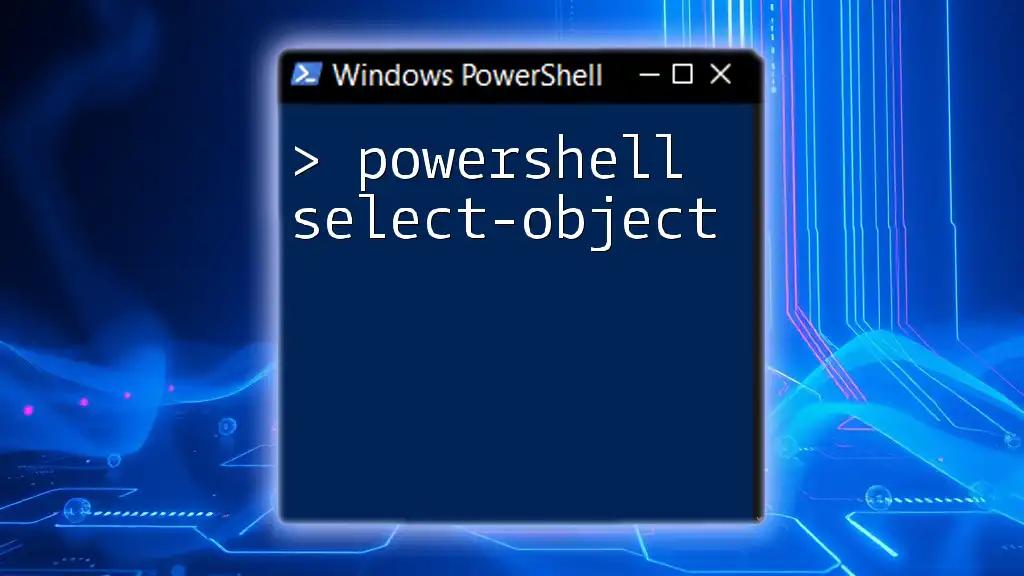
Best Practices for Using PowerShell Objects
Naming Conventions
Using clear and consistent naming conventions for object properties and methods is crucial. Consistency leads to improved readability and maintainability of your scripts.
Performance Considerations
While objects provide powerful functionality, it’s important to consider performance implications. Heavy use of objects in loops or nesting might slow down script execution. Always aim for efficiency in your data manipulation.
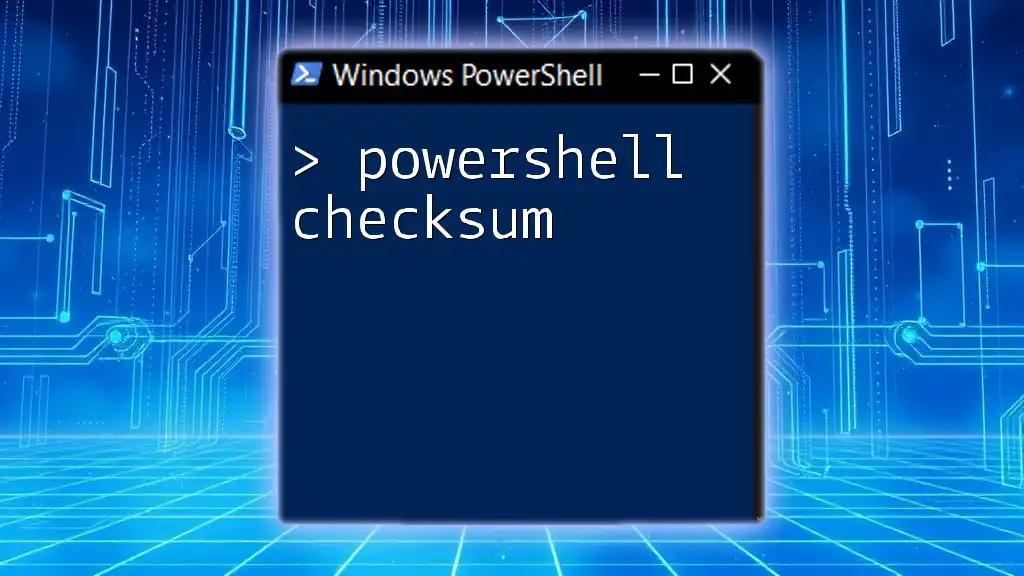
Conclusion
Understanding PowerShell objects is vital for anyone looking to leverage the full capabilities of PowerShell scripting. By mastering objects, properties, methods, and how to manipulate them, you'll greatly enhance your scripting prowess. Practicing and applying these concepts will lead to better, more efficient scripts.
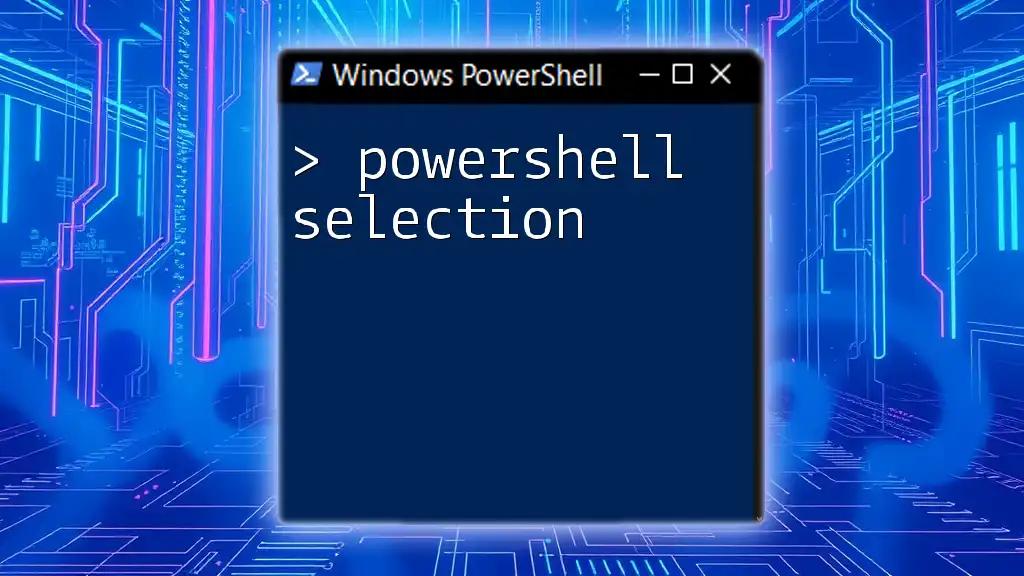
Call to Action
Consider exploring more about PowerShell through our extensive courses, where we dive deeper into scripting techniques and best practices!