The `DirectoryInfo` class in PowerShell provides a way to create, manipulate, and retrieve information about directories and subdirectories in the file system.
# Example: Create a DirectoryInfo object and list its files
$dirInfo = New-Object System.IO.DirectoryInfo 'C:\MyFolder'
$dirInfo.GetFiles() | ForEach-Object { Write-Host $_.Name }
Understanding DirectoryInfo
What is DirectoryInfo?
In PowerShell, DirectoryInfo is a vital class provided by the .NET framework that allows users to work easily with directories (folders). It represents a directory in the file system and is instrumental in performing operations such as creation, deletion, and modification of directories. Unlike traditional commands, DirectoryInfo offers a more object-oriented approach, making it easier to manage directories programmatically.
Syntax of DirectoryInfo
To initiate a DirectoryInfo object, you can use the following basic syntax:
$directoryInfo = New-Object System.IO.DirectoryInfo("C:\Path\To\Directory")
This line of code creates a new instance of the DirectoryInfo class, allowing you to interact with the specified directory. You can replace the path with any valid directory path on your system, enabling seamless access to its properties and methods.
Key Properties of DirectoryInfo
The DirectoryInfo class comes equipped with several properties that provide crucial information about the directory:
-
FullName: This property returns the complete path of the directory. For example:
$directoryInfo.FullName
-
Name: Returns just the name of the directory without the entire path. This is useful for logging or displaying directory names.
-
CreationTime: Retrieves the date and time when the directory was created. This can help track when directories were established for organizational purposes.
-
LastAccessTime: Provides the last accessed date and time of the directory, which is essential for monitoring usage.
-
LastWriteTime: Retrieves the last date and time the directory was modified, helping users keep track of changes.
-
Attributes: This property lists the attributes of the directory, such as ReadOnly, Hidden, and others, thus allowing quick assessments of directory status.
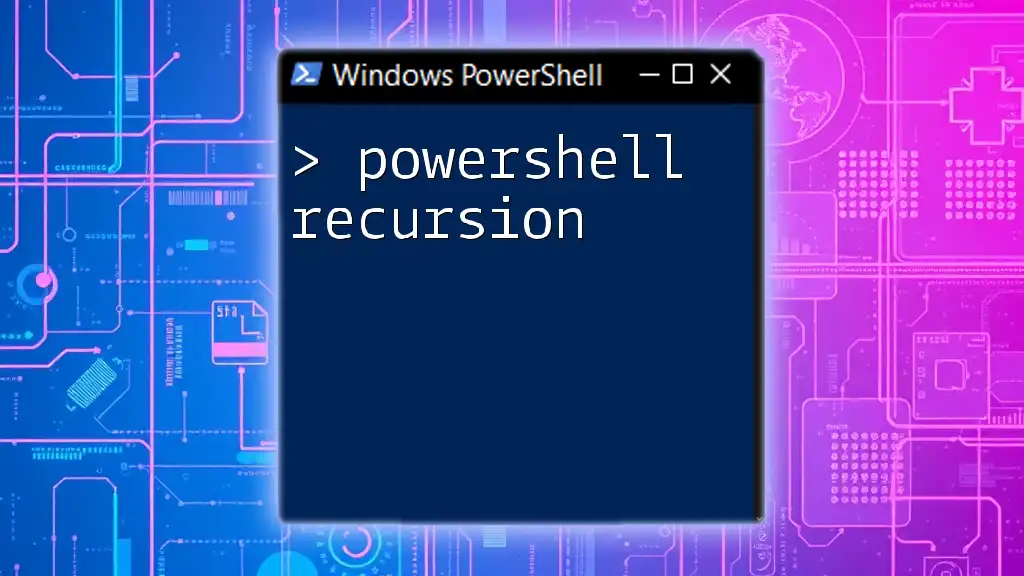
Common Methods of DirectoryInfo
Getting Subdirectories
One powerful feature of the DirectoryInfo class is its ability to easily retrieve subdirectories. This is done using the `GetDirectories()` method:
$subDirs = $directoryInfo.GetDirectories()
This method returns an array of DirectoryInfo objects, representing all subdirectories within the specified directory.
Accessing Files
Similarly, to access files in the directory, you can use the `GetFiles()` method:
$files = $directoryInfo.GetFiles()
This retrieves an array of files stored in the directory, enabling further manipulations such as filtering based on file types.
Creating a New Directory
Creating a new subdirectory is straightforward with the DirectoryInfo class. Use the `CreateSubdirectory(name)` method to establish a new folder:
$newDir = $directoryInfo.CreateSubdirectory("NewSubDirectory")
This creates a new subdirectory named "NewSubDirectory" in the current directory you are interacting with.
Deleting a Directory
The `Delete()` method allows you to remove an existing directory. Use caution with this method, as it will permanently delete the directory:
$directoryInfo.Delete()
It's essential to ensure that the directory is empty or flag it to remove non-empty directories as well beforehand, as this could lead to unintentional data loss.
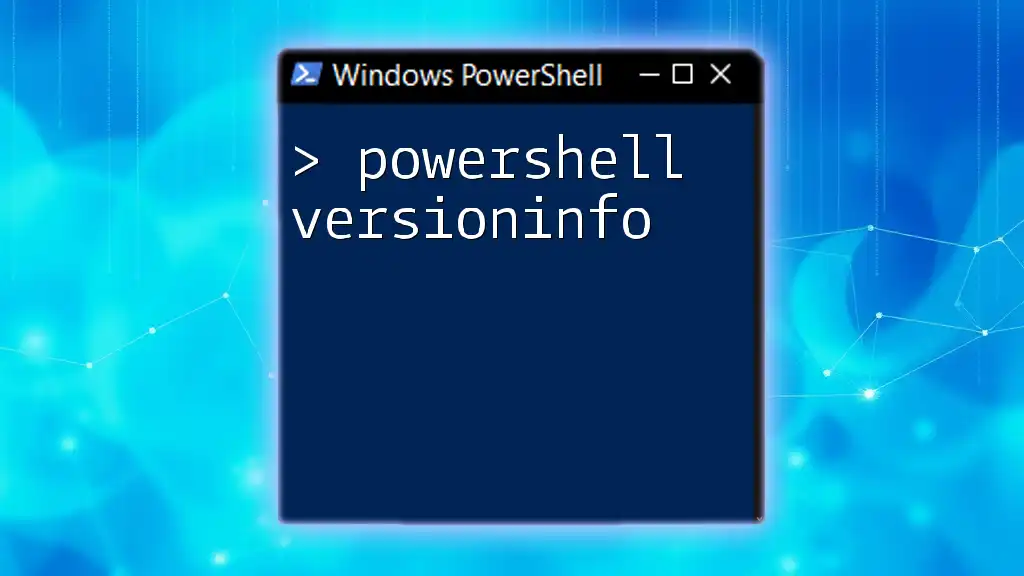
Advanced Usage of DirectoryInfo
Filtering Files and Directories
You can refine your file and directory retrieval using wildcards in the `GetFiles()` and `GetDirectories()` methods. For example, to fetch only text files, you can use:
$txtFiles = $directoryInfo.GetFiles("*.txt")
This command limits the returned files to those with a `.txt` extension, enhancing efficiency when dealing with large numbers of files.
Checking for Existence
You can verify whether a directory exists using the `Exists` property. This is essential for ensuring operations do not fail due to nonexistent paths:
if ($directoryInfo.Exists) {
"Directory exists."
} else {
"Directory does not exist."
}
This simple check can help build more robust scripts by avoiding unnecessary errors.
Modifying Attributes
Manipulating directory attributes is another powerful feature of the DirectoryInfo class. For instance, to set a directory as Hidden, you can modify the `Attributes` property:
$directoryInfo.Attributes = [System.IO.FileAttributes]::Hidden
This effectively makes the directory invisible in typical file explorations, which can be useful for keeping certain directories discreet.
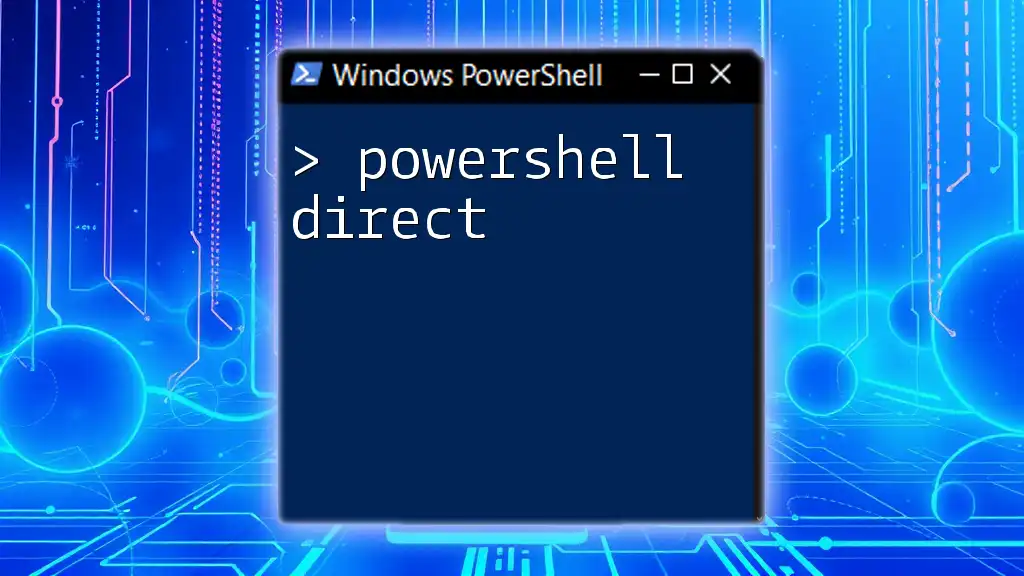
Best Practices for Using DirectoryInfo
Performance Considerations
To enhance performance when working with DirectoryInfo, especially in directories containing a vast number of files or subdirectories, it's essential to filter results efficiently and minimize unnecessary calls. Caching frequently accessed directories can also improve responsiveness.
Error Handling
Proper error handling is critical when working with file systems to prevent unexpected crashes or data loss. Employing Try/Catch blocks can manage potential errors effectively:
try {
# Code that may cause an error
} catch {
# Error handling code
}
This structure allows your script to continue running and gracefully handles any issues that may arise.
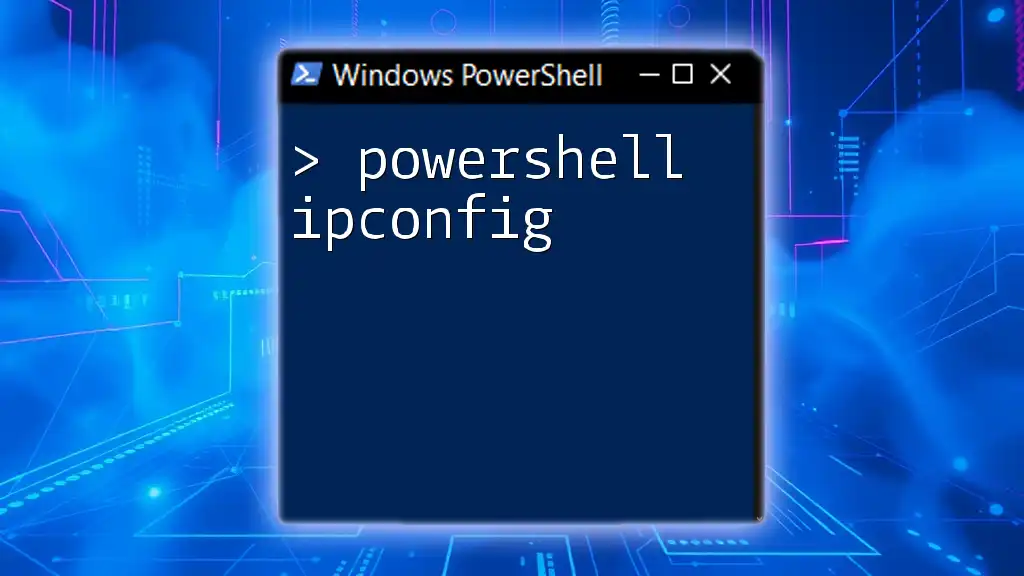
Conclusion
In this guide, you learned about the PowerShell DirectoryInfo class and how it empowers users to manage directories systematically. Through its properties and methods, DirectoryInfo allows for seamless navigation, creation, deletion, and modification of directories, making it a cornerstone for any PowerShell scripting tasks related to file management. By practicing these commands, you will build confidence in using PowerShell effectively, paving the way for mastering even more advanced concepts in this powerful scripting environment.
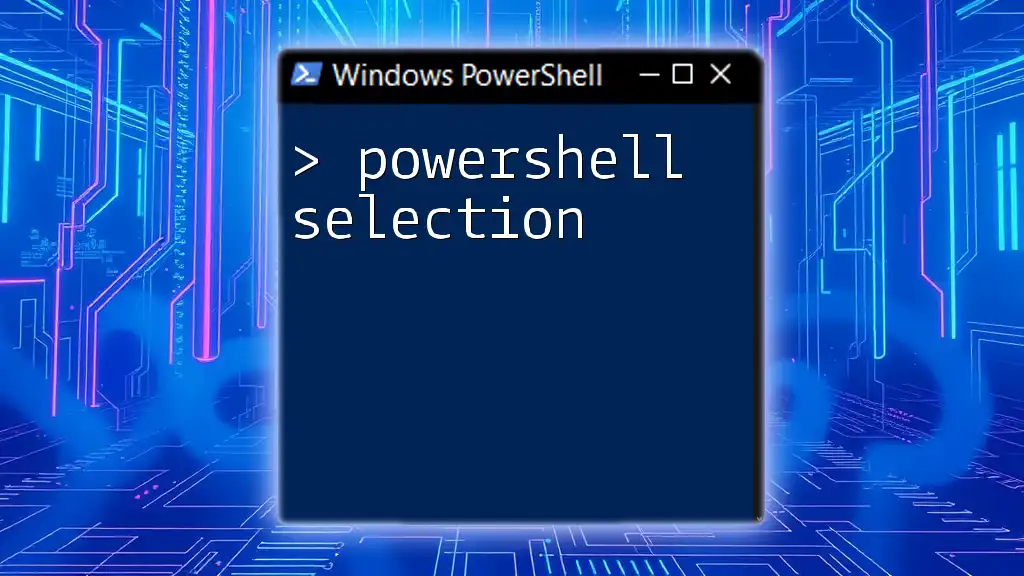
Resources
For further learning, consider exploring:
- Official Documentation on PowerShell and .NET
- Recommended books and online courses on PowerShell scripting
- Community forums and support channels like Stack Overflow for peer assistance
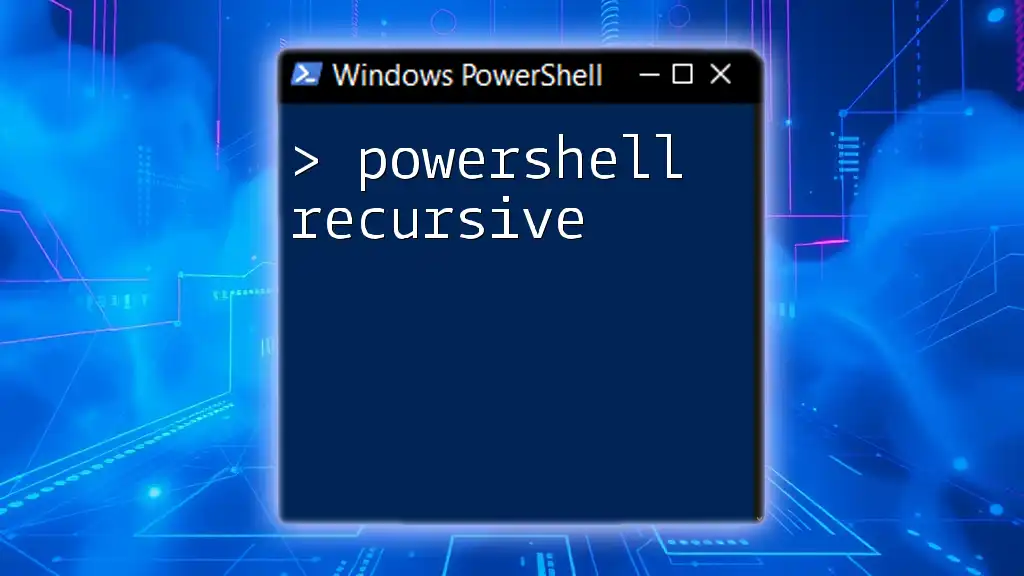
Call to Action
Ready to elevate your PowerShell skills? Sign up for our workshops or classes and unlock the full potential of PowerShell's capabilities. Practice using the DirectoryInfo class with real examples to solidify your understanding, and don’t hesitate to reach out for additional resources or assistance!