To change the current directory in PowerShell, you can use the `Set-Location` cmdlet or its alias `cd` followed by the path you want to navigate to.
Set-Location 'C:\Path\To\Your\Directory'
# or using the alias
cd 'C:\Path\To\Your\Directory'
Understanding PowerShell Directories
What is a Directory in PowerShell?
In PowerShell, a directory refers to a folder within the file system hierarchy of your operating system. It's a container used to organize files and other directories, and PowerShell provides commands to navigate and manipulate these directories. Understanding how to manage directories is fundamental for effective scripting and automation.
Default PowerShell Directory
When you launch PowerShell, it defaults to a specific directory based on your user profile, usually something like `C:\Users\<YourUsername>` on Windows. This directory serves as your starting point for all commands and scripts you execute. Knowing your starting point can save time as you navigate through the file system.
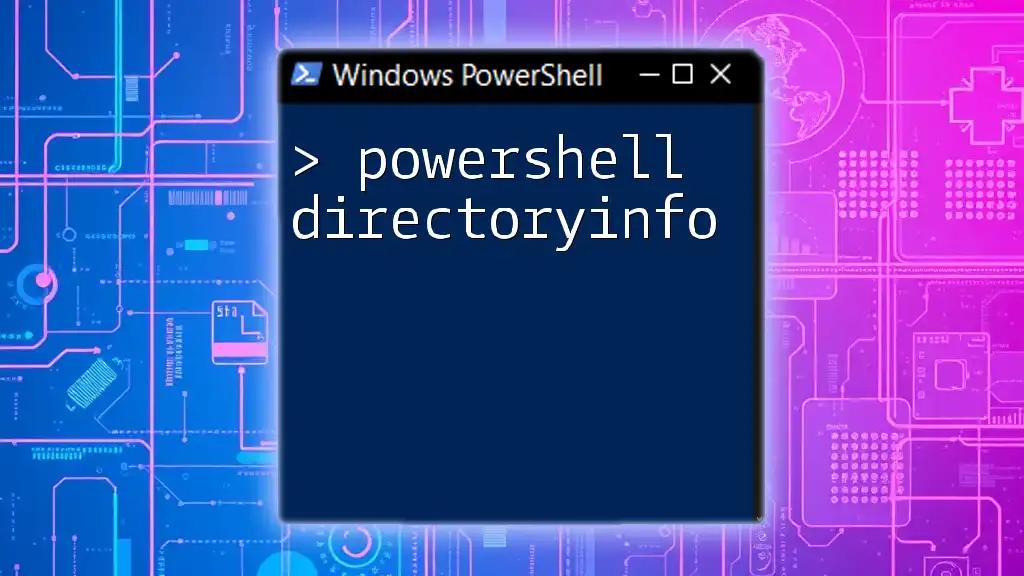
How to Change Directory in PowerShell
Using the `Set-Location` Cmdlet
The primary cmdlet for changing directories in PowerShell is `Set-Location`. This cmdlet is straightforward, allowing you to point PowerShell to a new directory. The syntax is simple:
Set-Location -Path "C:\Your\Target\Directory"
This command directs PowerShell to the specified directory. If the directory exists, your current working directory will change to that location.
Using the Alias `cd`
For convenience, PowerShell includes the alias `cd`, which works the same way as `Set-Location`. This makes it easier for users familiar with other command-line interfaces to navigate.
Here’s how you can use `cd`:
cd "C:\Your\Target\Directory"
This command achieves the same result and can help speed up your workflow.

Navigating with Ease
Using Relative Paths
PowerShell supports relative paths, which allow you to change directories without specifying the full file path every time. For example, if your current directory is `C:\Users\YourUsername`, and you want to go up one level, you can simply use:
cd ..
The `..` indicates the parent directory, making it easy to navigate back when needed. To change into a subdirectory from your current location, like entering a folder named "Projects", you can do the following:
cd Projects
This command saves you from typing the full absolute path.
Using Absolute Paths
In scenarios where you need to specify the complete folder location, absolute paths are the way to go. An absolute path starts from the root of the drive and specifies every part of the directory structure leading to your target location.
For example, if you want to change your directory to your documents folder, you can use:
Set-Location -Path "C:\Users\YourUsername\Documents"
Using absolute paths is particularly useful in scripts, where you want to ensure that the correct directory is always accessed, regardless of the current working directory.
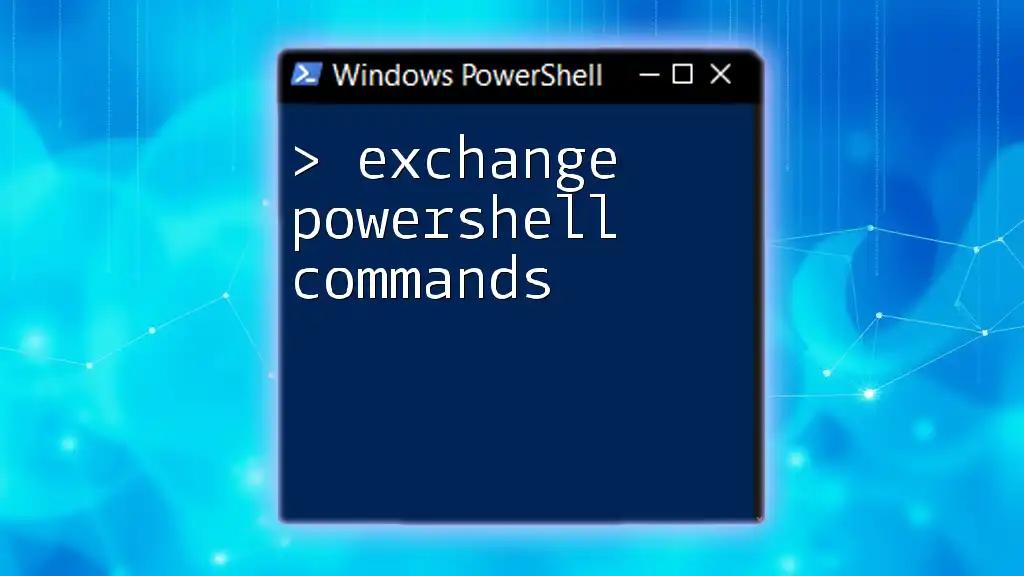
Check Your Current Directory
Get the Current Directory
If you're ever unsure where you are in your directory structure, you can check your current location using the `Get-Location` cmdlet. This command will display your present working directory:
Get-Location
It's a simple yet valuable tool for confirming your context, especially before executing commands that depend on your current directory.
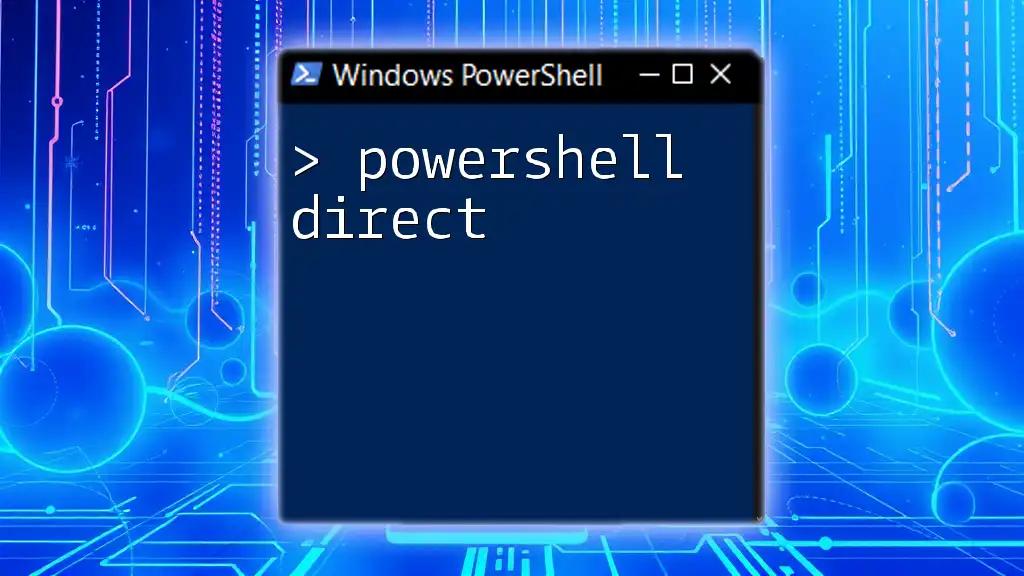
Change Directory with PowerShell Script
Creating a Simple PowerShell Script
Creating a PowerShell script that changes directories can streamline your workflow, especially if you often switch between the same directories. Here's an example script that accepts a target directory as a parameter:
# ChangeDirectory.ps1
param (
[string]$TargetPath
)
Set-Location -Path $TargetPath
This script allows you to run it and specify any directory as an argument, facilitating quick changes without retyping commands.
How to Run the Script
To execute the script, you would typically navigate to its location and run it using:
.\ChangeDirectory.ps1 "C:\Your\Target\Directory"
After running the script, PowerShell will change to the specified directory.
Error Handling in the Script
To enhance your script’s robustness, it's essential to include error handling. This will prevent your script from failing unexpectedly if an invalid directory is supplied. Here's how you might implement error handling:
try {
Set-Location -Path $TargetPath -ErrorAction Stop
} catch {
Write-Host "Failed to change directory: $_"
}
This snippet catches any errors that occur during the directory change and outputs a friendly message, making it easier to troubleshoot what went wrong.
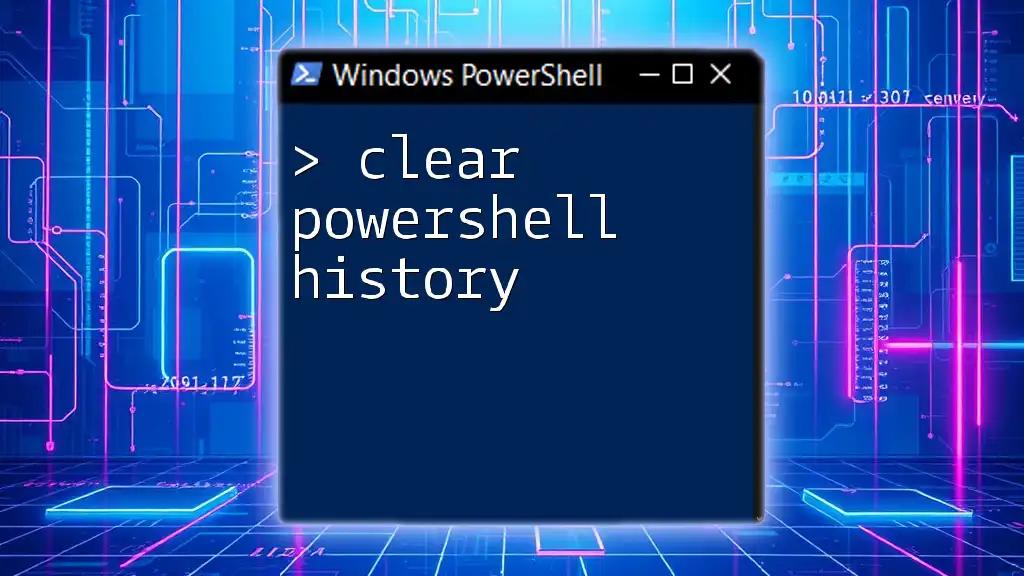
Best Practices for Changing Directories in PowerShell
Consistency and Readability
Maintaining a consistent approach in your command usage and scripts not only makes your code more readable but also easier to understand and maintain. Stick to using either `Set-Location` or `cd` based on your personal preference and keep it consistent throughout your scripts.
Utilizing Comments
Commenting your scripts can significantly enhance their clarity. A well-commented script allows anyone (including your future self) to understand the purpose of each command or segment of code. For instance:
# This script changes the PowerShell working directory
Set-Location -Path $TargetPath
Comments enable anyone who reads your code to quickly grasp its functionality and intent.
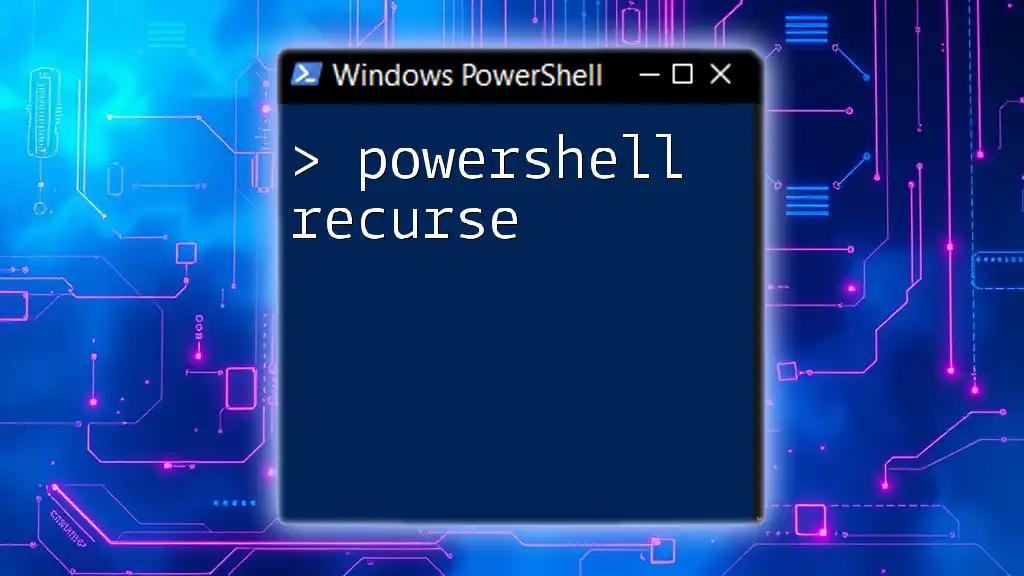
Conclusion
Changing the working directory in PowerShell is a fundamental skill that improves your efficiency when working in the command line. Whether you're using cmdlets like `Set-Location`, aliases like `cd`, or creating scripts for repetitive tasks, mastering these concepts opens up a world of possibilities for file management and automation. Now's the time to practice these commands and scripts to enhance your PowerShell proficiency.