The `Microsoft.PowerShell.Commands.WriteErrorException` is utilized in PowerShell to generate an error message, which can provide valuable information during error handling in scripts.
Here’s a code snippet demonstrating its usage:
# Example of using WriteErrorException
try {
# Simulate an error
throw "This is a custom error"
} catch {
# Write the error using WriteErrorException
$ErrorRecord = New-Object System.Management.Automation.ErrorRecord $_ Exception $_.InvocationInfo
Write-Error $ErrorRecord
}
Understanding WriteErrorException
What is an Exception?
An exception in programming is an unexpected event that occurs during the execution of a program which disrupts the normal flow of instructions. In PowerShell, exceptions are commonly used to handle errors gracefully and ensure that your script can respond to various issues that may arise, such as missing files or failed commands.
The common types of exceptions in PowerShell include:
- System.Exception: The base class for all exceptions.
- System.IO.IOException: Occurs during input/output operations, such as file manipulation.
- System.ArgumentException: Triggered when an argument provided to a method is invalid.
The Concept of WriteErrorException
The command `microsoft.powershell.commands.writeerrorexception` is specifically designed for generating errors in PowerShell scripts. It writes an error to the error pipeline, allowing for structured error reporting and management. Understanding when and how to use WriteErrorException is crucial for effective error handling in your scripts.
Using WriteErrorException helps to:
- Signal that a significant error condition has happened.
- Provide developers or users with a clear message indicating what went wrong.
- Ensure that error handling processes can capture and respond appropriately to the errors.
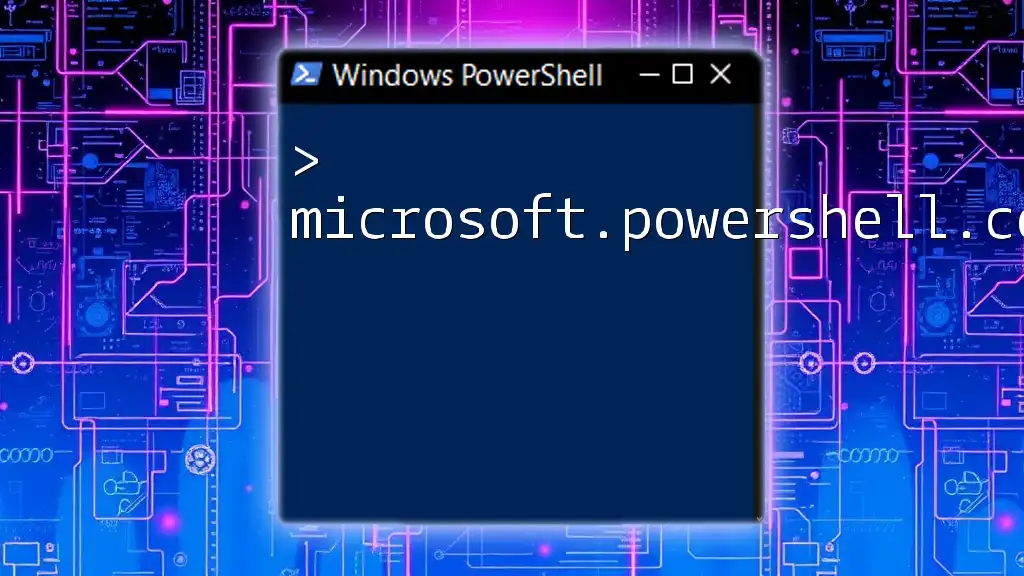
How to Use WriteErrorException Command
Syntax of WriteErrorException
The general syntax for WriteErrorException is as follows:
Write-ErrorException [-Message <String>] [-ErrorCategory <ErrorCategory>] [-ErrorId <String>] [-TargetObject <PSObject>]
Parameters and Their Descriptions
- -Message: The specific error message you want to convey.
- -ErrorCategory: A categorization of the error such as `InvalidOperation`, `NotSpecified`, etc.
- -ErrorId: A unique identifier for the error.
- -TargetObject: The object that caused the error, allowing for more context in the error message.
Practical Examples
Example 1: Basic Usage
In scenarios where you need to indicate a critical issue within your script, using WriteErrorException is straightforward.
Scenario Overview: You want to inform the user that a significant error has occurred.
Write-ErrorException -Message "A critical error has occurred." -ErrorCategory "NotSpecified"
When you run this command, PowerShell outputs the specified error message to the error stream, making it clear that something went wrong.
Example 2: Custom Error Handling
With PowerShell's error handling capabilities, it’s essential to appropriately manage exceptions when they arise. The Try/Catch block in PowerShell allows for this structured handling.
Scenario Overview: An operation within your script may throw an error, and you want to catch and respond appropriately.
Try {
# Some function call that might fail
Throw "An error occurred."
} Catch {
Write-ErrorException -Message $_.Exception.Message -ErrorCategory "OperationStopped"
}
In this example, if the function call fails, the Catch block captures the exception and uses WriteErrorException to report it. The `$_` variable holds the error information, including the message, which provides context to the user.
Example 3: Using WriteError with `ErrorAction`
Sometimes, integrating `ErrorAction` with WriteErrorException can enhance your error reporting, especially when dealing with cmdlets.
Scenario Overview: You want to prevent an interruption when dealing with non-existent files and generate an error message if that occurs.
Get-Item "C:\NonExistentFile.txt" -ErrorAction Stop -ErrorVariable myError
if ($myError) {
Write-ErrorException -Message "Failed to retrieve the item." -ErrorCategory "ObjectNotFound"
}
In this case, if the file does not exist, PowerShell stops execution due to the `-ErrorAction Stop`. The error is captured in the variable `$myError`, which then allows you to provide a custom error message using WriteErrorException.
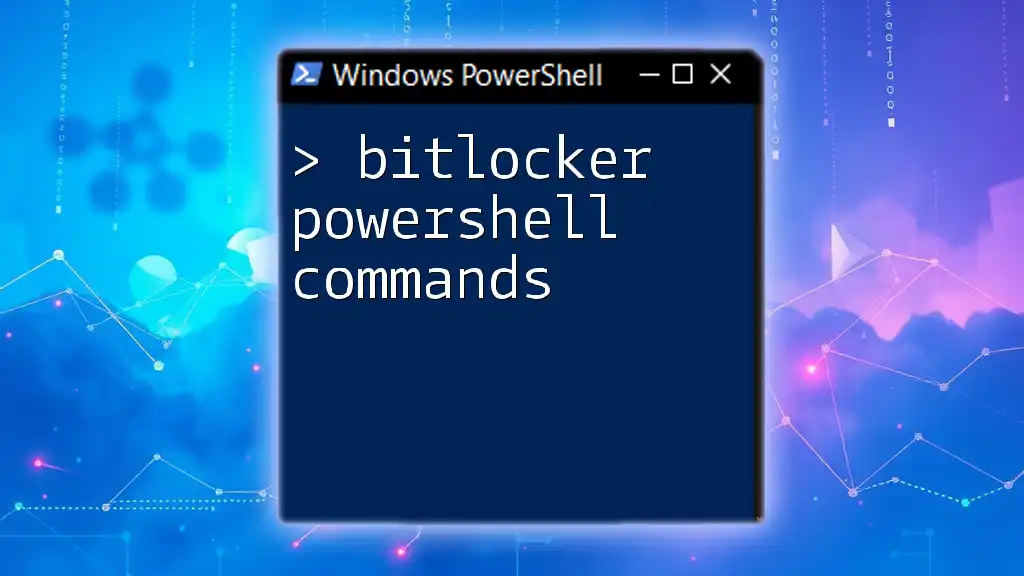
Best Practices for Using WriteErrorException
How to Write Effective Error Messages
Crafting effective error messages is vital for debugging and user experience. Here are some key points to consider:
- Clarity: Ensure your message is straightforward and easy to understand.
- Conciseness: Avoid overwhelming users with excessive detail; focus on the key issue.
Common Mistakes to Avoid:
- Using vague or generic messages that do not provide useful information.
- Overly verbose messages that may confuse rather than clarify.
Integrating WriteErrorException in Scripts
To maximize WriteErrorException's utility:
- Use It Consistently: Integrate WriteErrorException along with other structured error handling techniques.
- Standardize Error Responses: Develop a template or standard format for your error messages to ensure consistency across your scripts.
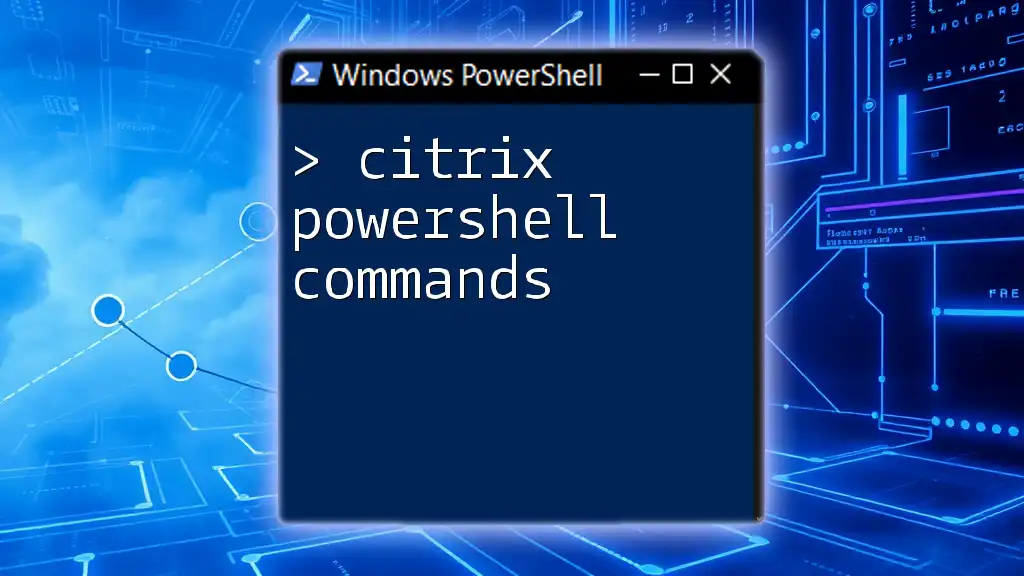
Use Cases and Scenarios
When to Use WriteErrorException
The implementation of WriteErrorException is essential in various scenarios, particularly when your script interacts with external systems, such as:
- File Operations: Handling errors when files cannot be found or accessed.
- Service Calls: Managing exceptions when dealing with external APIs or services.
In modern development practices, particularly within CI/CD pipelines, implementing WriteErrorException can preserve logs and alert development teams to critical failures without halting the process entirely. This proactive approach enables better monitoring and quicker resolution of issues.
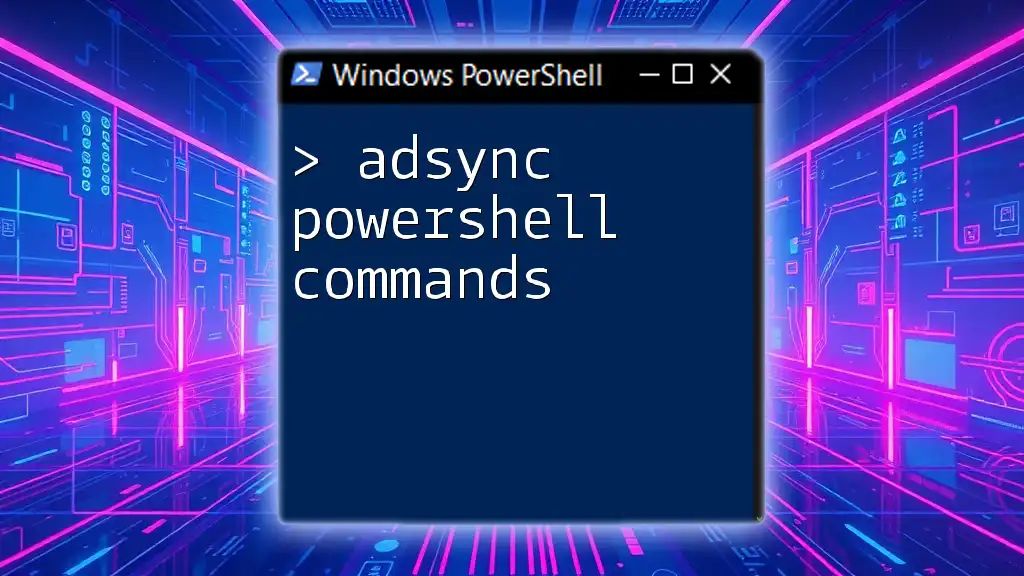
Conclusion
Utilizing `microsoft.powershell.commands.writeerrorexception` is a powerful method for managing error responses within PowerShell scripts. By following the best practices outlined in this guide and applying the demonstrated examples, you can enhance the reliability of your scripts and create a more user-friendly environment for anyone running your code.
Consider integrating WriteErrorException in your PowerShell workflows to ensure clearer communication of issues, benefiting developers and users alike.
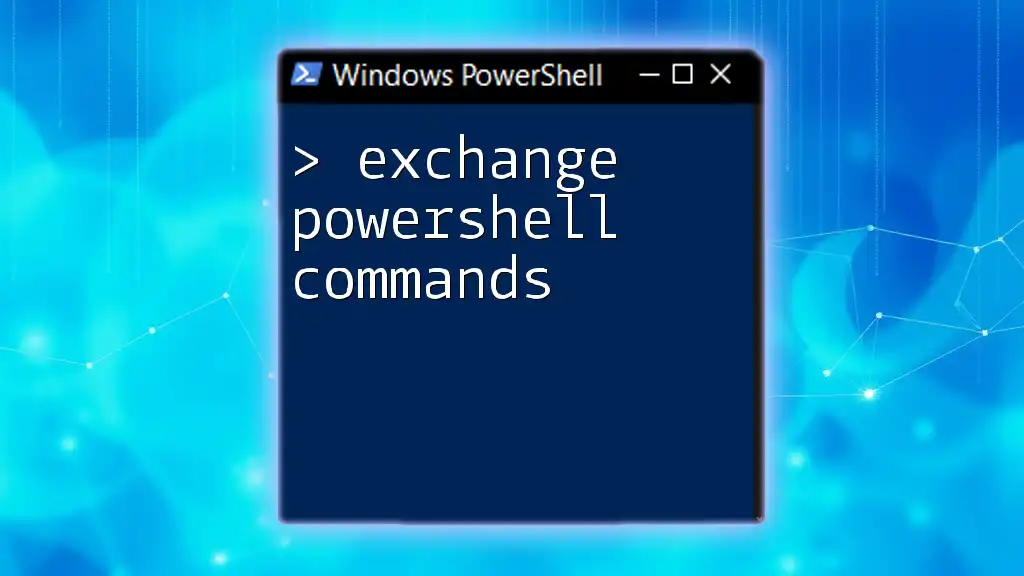
Additional Resources
For further learning, check the official Microsoft documentation on WriteErrorException and explore recommended books and tutorials to enhance your PowerShell skills.
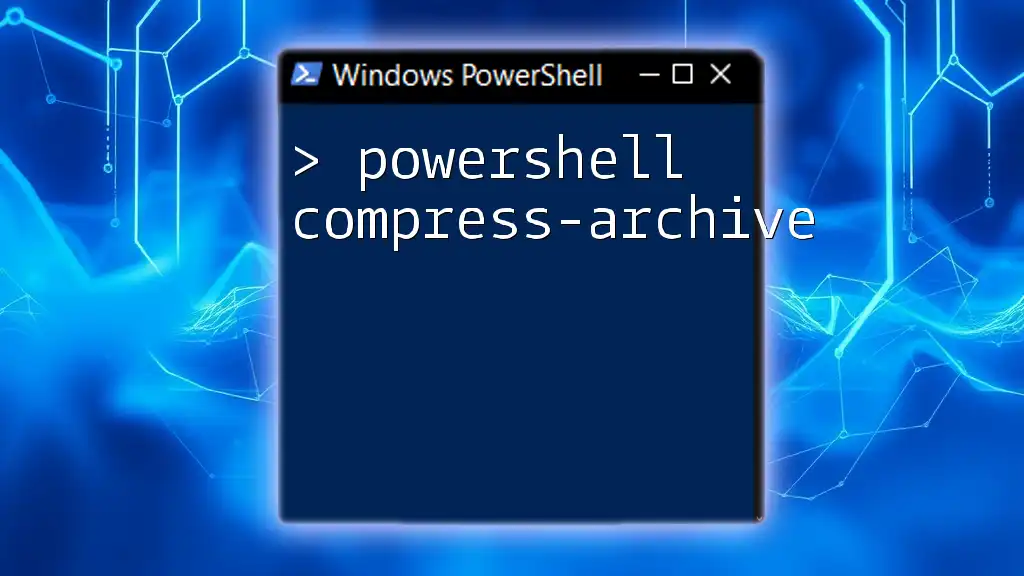
Call to Action
We would love to hear your experiences with WriteErrorException! Share your thoughts and questions in the comments below. If you’re interested in mastering more PowerShell commands, check out our services for expert training and resources.