PowerShell comparison involves evaluating expressions to determine their truth value, allowing users to make decisions based on conditions; for example, you can compare two numbers using the `-eq` operator to check for equality.
$number1 = 5
$number2 = 10
if ($number1 -eq $number2) {
Write-Host 'The numbers are equal.'
} else {
Write-Host 'The numbers are not equal.'
}
Understanding Comparison Operators in PowerShell
What are Comparison Operators?
Comparison operators in PowerShell serve a crucial role in scripting by allowing you to evaluate expressions. They help determine the relationship between two values, enabling conditional logic that can control the flow of your scripts. Knowing how to use these operators effectively is essential for any PowerShell practitioner.
Types of Comparison Operators
PowerShell offers several types of comparison operators that can be broadly categorized into equality operators and relational operators.
-
Equality Operators: These operators check if two values are the same or different.
- `-eq`: Used to check if two values are equal.
- `-ne`: Used to check if two values are not equal.
-
Relational Operators: These operators evaluate numerical relationships.
- `-gt`: Greater than.
- `-lt`: Less than.
- `-ge`: Greater than or equal to.
- `-le`: Less than or equal to.
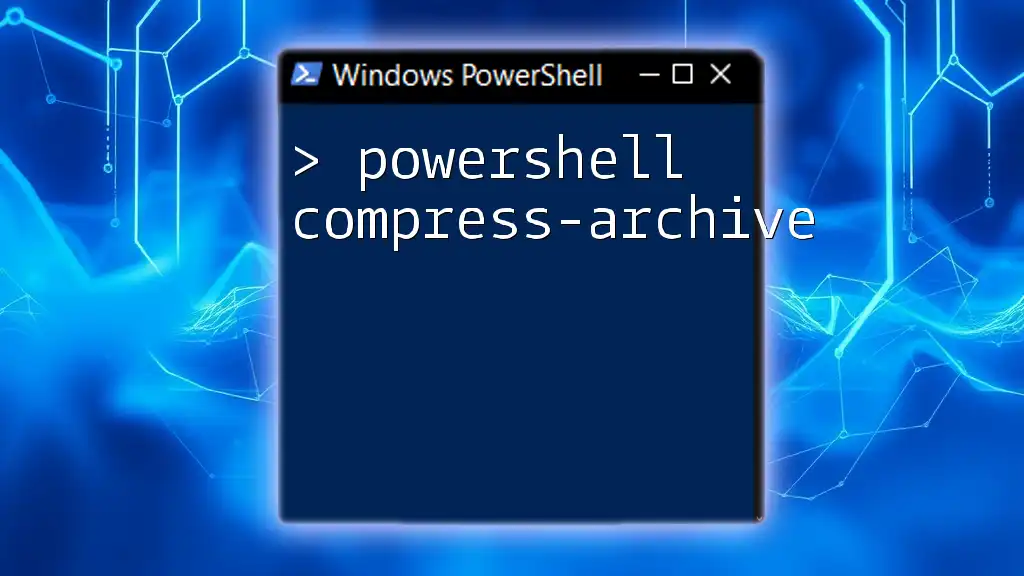
PowerShell Comparisons: How to Use Them
Syntax of Comparison Operators
The syntax of comparison operators is straightforward, yet their proper usage is key to successful script execution. Here’s a basic example of using the `-eq` operator:
$a = 5
$b = 5
if ($a -eq $b) {
"They are equal"
}
This script will print "They are equal" because the values of `$a` and `$b` are indeed equal.
Practical Examples of Comparison Operators
- Using `-eq` for Equality: In practical use, you might compare user input or values retrieved from databases. Consider the following example:
$userInput = "admin"
$expectedInput = "admin"
if ($userInput -eq $expectedInput) {
"Access Granted"
}
- Using `-ne` for Inequality: This operator is useful for validating conditions that must not match. For example:
$password = "secret"
if ($password -ne "1234") {
"Password is valid"
}
- Greater and Less Than Comparisons: The relational operators allow you to compare numerical values directly. Here’s a useful snippet:
$x = 8
$y = 10
if ($x -lt $y) {
"x is less than y"
}
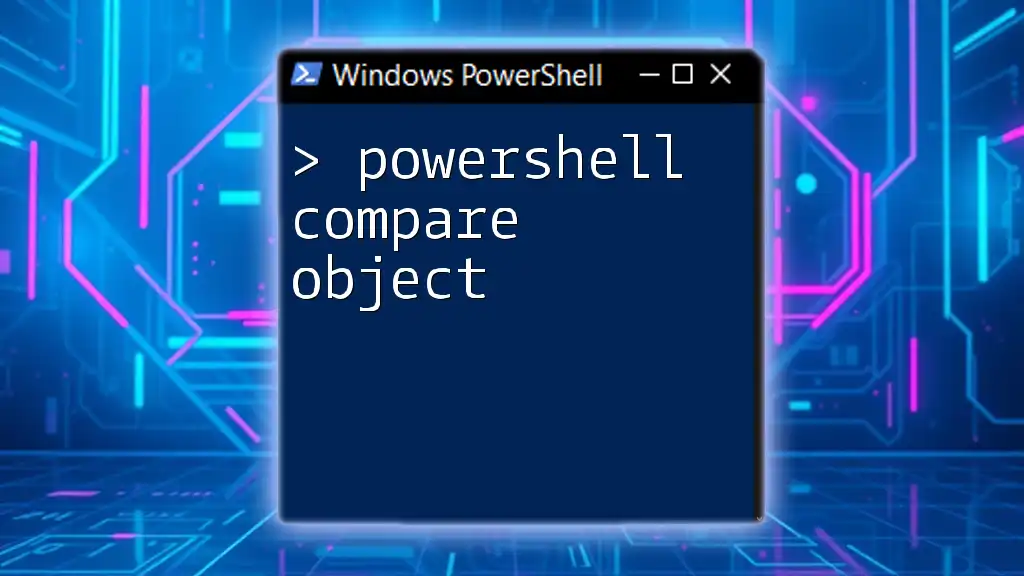
Advanced Comparison Techniques
Using Comparison Operators with Arrays
PowerShell makes it easy to perform comparisons within arrays. For instance, if you have an array of numbers and want to filter out values greater than a specific threshold, you can do the following:
$array = 1, 2, 3, 4, 5
$result = $array | Where-Object { $_ -gt 3 }
This example will leave you with an array containing the values 4 and 5.
Combining Comparison Operators
More complex logical evaluations can be performed by combining comparison operators with logical operators like `-and` and `-or`. Here’s how you might use them:
$a = 5
$b = 7
if ($a -gt 0 -and $b -lt 10) {
"Both conditions are true"
}
In this case, the script confirms that both conditions hold true before executing the enclosed code.
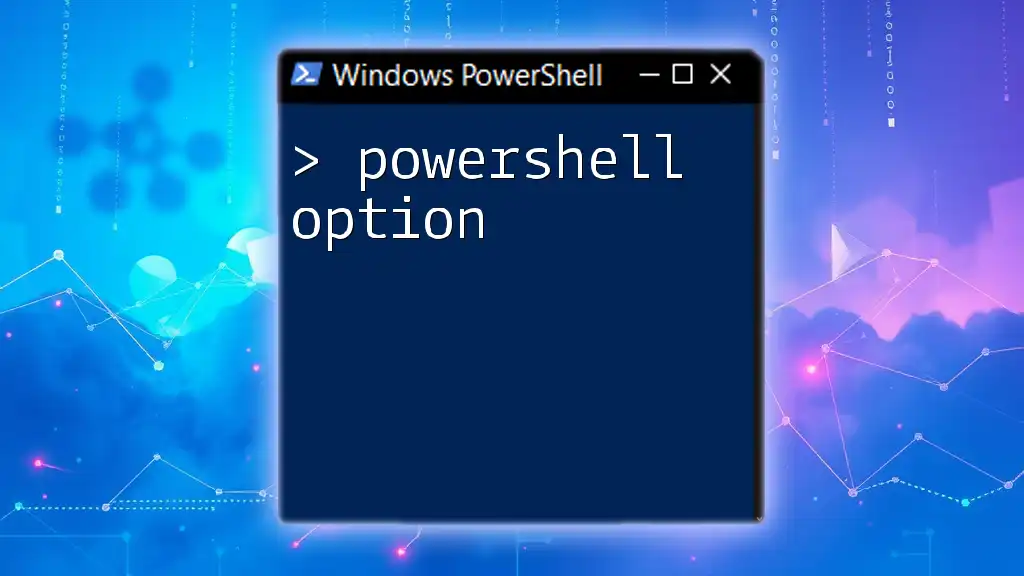
PowerShell Equality: A Deeper Dive
Understanding Type Comparison
A crucial aspect of PowerShell comparisons is how different data types behave. PowerShell is often flexible with data types, but this can lead to unexpected results. For instance, comparing a string to an integer can yield fascinating results:
$stringNumber = "5"
$intNumber = 5
if ($stringNumber -eq $intNumber) {
"Type conversion happens"
}
Here, the comparison returns true, demonstrating PowerShell's ability to automatically convert the string to an integer.
Case Sensitivity in Comparisons
Not all comparisons are case-insensitive. PowerShell also provides case-sensitive operators like `-ceq` and `-cne`. It’s essential to choose the right operator based on your requirements. Here’s an example that illustrates this point:
$str1 = "Hello"
$str2 = "hello"
if ($str1 -ceq $str2) {
"Equal (case-sensitive)"
}
In this case, the condition evaluates to false since the case differs.
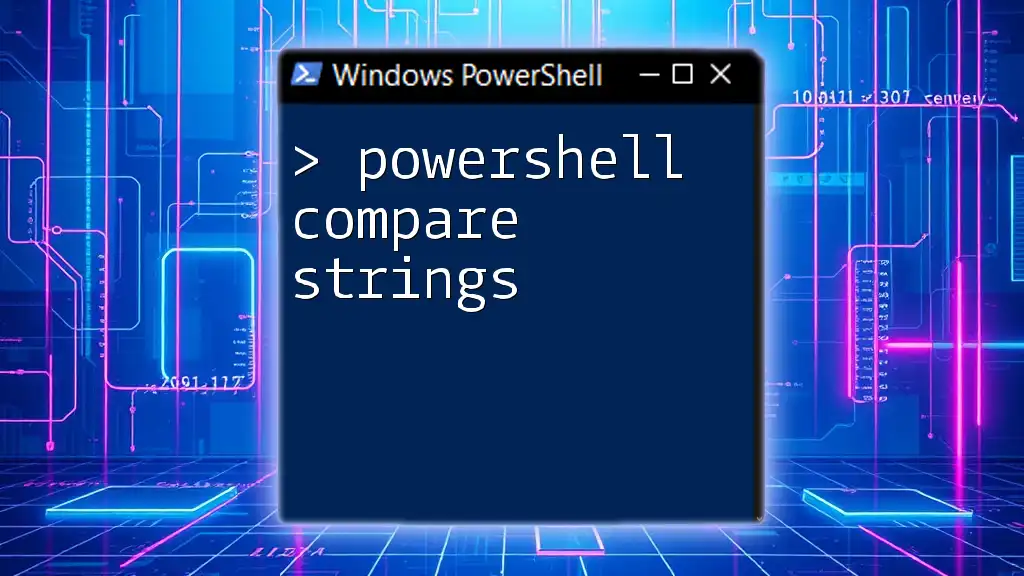
Best Practices for Using Comparison Operators
Performance Considerations
While using multiple comparison operators, be mindful of potential performance impacts. Complex comparisons may slow down your script execution. Keep your code efficient by avoiding needless complexity in your conditional checks.
Common Mistakes to Avoid
Many beginners overlook the importance of data types, leading to erroneous comparisons. Always ensure that you’re comparing like types to avoid unexpected results. Misusing relational operators can also lead to logical errors, so double-check your logic before executing your scripts.
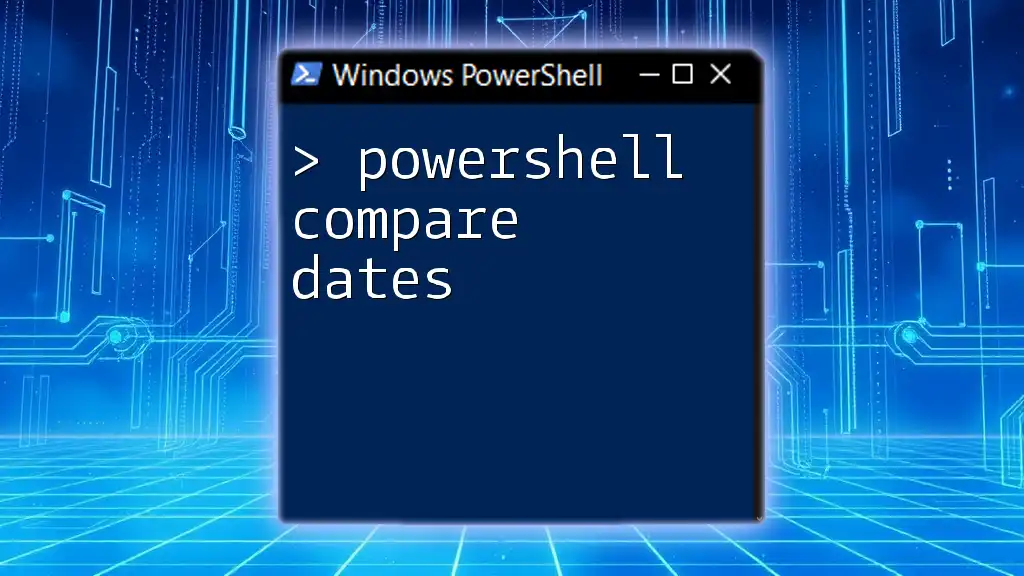
Conclusion
In the realm of PowerShell scripting, mastering PowerShell comparisons is invaluable. As you become proficient in using comparison operators effectively, you'll enhance your scripting capabilities and make your code more robust. Be sure to practice various scenarios to solidify your understanding. With time and experience, you'll harness the full power of PowerShell comparisons in your scripts.
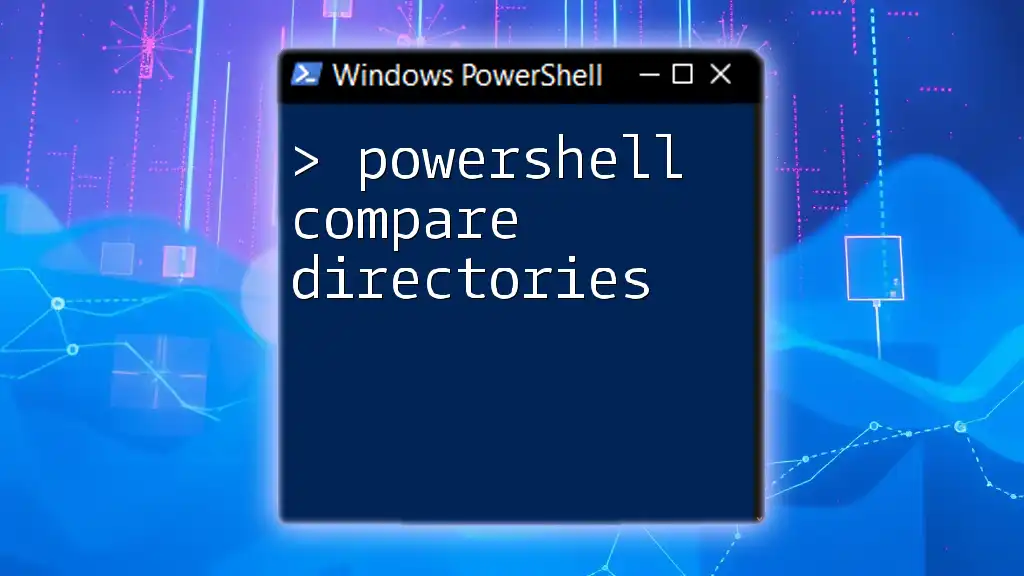
Additional Resources
For further exploration of this topic, consider diving into the official PowerShell documentation or seeking out tutorials and courses that will enrich your learning experience in PowerShell scripting.