In PowerShell, you can compare two lists to find the differences or common elements using the `Compare-Object` cmdlet. Here's a simple example:
$List1 = 'A', 'B', 'C', 'D'
$List2 = 'B', 'C', 'E', 'F'
Compare-Object -ReferenceObject $List1 -DifferenceObject $List2
Understanding Lists in PowerShell
What Constitutes a List?
In PowerShell, a list is primarily represented through arrays and other collection types, such as hashtables. Lists can contain various elements, including strings, integers, and even complex objects. This makes lists incredibly versatile for different data management tasks.
Creating Lists in PowerShell
Creating lists in PowerShell can be done easily using arrays. Below is a simple example:
$list1 = @("Apple", "Banana", "Cherry")
$list2 = @("Banana", "Date", "Cherry")
In this snippet, `$list1` and `$list2` contain strings representing fruit names. These arrays will serve as our reference for comparison.

Methods to Compare Lists
Using the `Compare-Object` Cmdlet
One of the most effective ways to compare two lists in PowerShell is by utilizing the `Compare-Object` cmdlet. This cmdlet allows you to identify differences between two sets of data.
Here’s how it's done:
Compare-Object -ReferenceObject $list1 -DifferenceObject $list2
Parameters
- `-ReferenceObject`: This parameter specifies the first collection you want to compare (in this case, `$list1`).
- `-DifferenceObject`: This parameter specifies the second collection for comparison (in this case, `$list2`).
When you run the above command, you will see output listing items that are unique to each list, indicated by the SideIndicator (`=>` for items only in `$list2` and `<=` for items only in `$list1`).
Understanding the Output
The output from `Compare-Object` can be quite insightful. The `InputObject` property displays the differing items, while the `SideIndicator` helps in identifying where the items belong.
For example:
InputObject SideIndicator
----------- ---------------
Date =>
Apple <=
Filtering Comparisons
To focus on specific differences, you can use the `Where-Object` cmdlet in conjunction with `Compare-Object`.
Here's an example that filters for items only in `$list2`:
Compare-Object -ReferenceObject $list1 -DifferenceObject $list2 | Where-Object { $_.SideIndicator -eq '=>' }
This command will return only the items that are present in `$list2` but not in `$list1`, enabling you to quickly identify new additions.
Advanced Comparison Techniques
Comparing with Custom Properties
When dealing with more complex data, you might want to compare lists of custom objects. Here’s how you can create and compare custom objects:
$list1 = @(@{Name="John"; Age=30}, @{Name="Jane"; Age=25})
$list2 = @(@{Name="John"; Age=30}, @{Name="Bob"; Age=28})
Compare-Object -ReferenceObject $list1 -DifferenceObject $list2 -Property Name, Age
This comparison checks both the Name and Age properties, offering a granular view of differences.
Merging Comparisons
After you’ve compared lists, combining the results can be beneficial for a comprehensive overview. Here’s a way to merge output:
$mergedResults = Compare-Object $list1 $list2
$mergedResults | Format-Table -Property SideIndicator, InputObject
This approach will present your comparison in a tabular format, making it easier to digest.
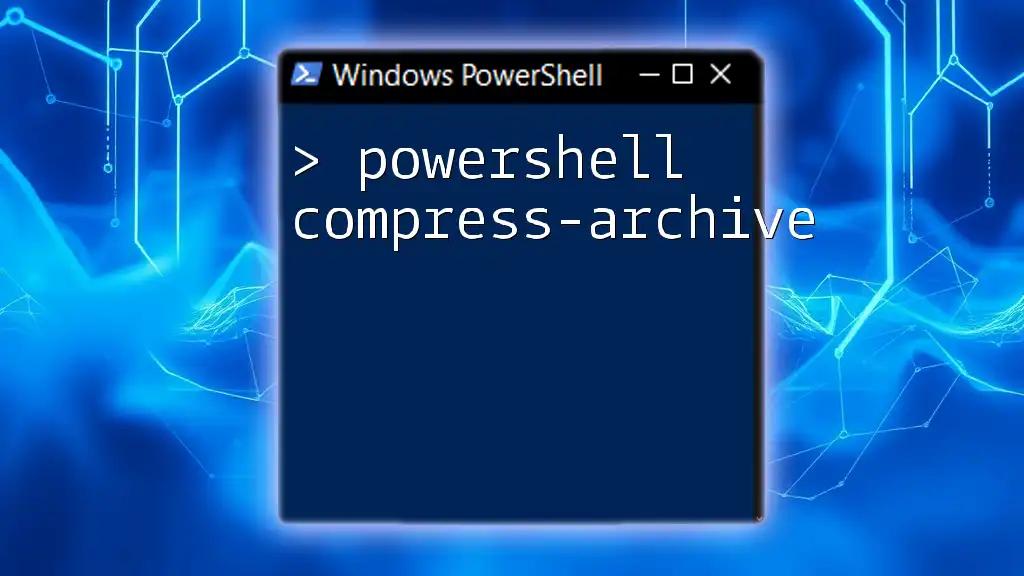
Practical Use Cases
Real-world Applications of List Comparison
Understanding how to compare two lists is invaluable in several domains:
- IT Management: Checking asset inventories to ensure accuracy and completeness.
- Quality Assurance: Verifying lists of test cases to confirm that all scenarios are covered.
- Data Migration: Ensuring that records are accurately transferred with no omissions.
Example Scenarios
Scenario 1: Identifying Duplicates in User Lists
If you have lists of users and want to find duplicates, you can compare the lists directly:
Compare-Object -ReferenceObject $list1 -DifferenceObject $list2 | Where-Object { $_.SideIndicator -eq '==' }
Scenario 2: Verifying Migration Success between Databases
After migrating data, comparing old and new data sets can help identify discrepancies or missing records.
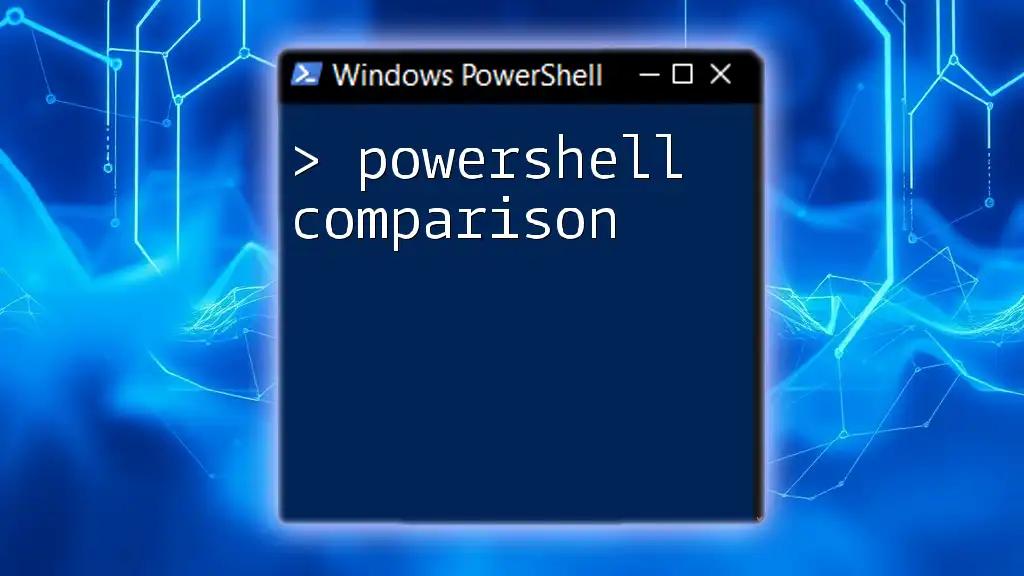
Troubleshooting Common Issues
Handling Null Values
It’s essential to check for null values before performing comparisons to prevent errors:
if ($null -ne $list1 -and $null -ne $list2) {
Compare-Object -ReferenceObject $list1 -DifferenceObject $list2
}
This simple check can save you from unexpected issues.
Skipping Duplicates
If your lists contain duplicates and you wish to ignore them, you can filter them out prior to comparison:
$uniqueList1 = $list1 | Select-Object -Unique
$uniqueList2 = $list2 | Select-Object -Unique
Compare-Object -ReferenceObject $uniqueList1 -DifferenceObject $uniqueList2
By using `Select-Object -Unique`, you'll ensure that your comparison is clean and focused only on unique entries.

Summary
In this guide, we explored various methods to compare two lists in PowerShell, from utilizing the `Compare-Object` cmdlet to understanding and filtering outputs. The flexibility of PowerShell, alongside its powerful list comparison capabilities, provides a solid foundation for data management tasks. Practicing these techniques in different scenarios will improve your proficiency and ensure you can tackle real-world data challenges effectively. If you have any questions or would like further insights, don't hesitate to reach out!

Additional Resources
For more in-depth understanding, refer to the [official Microsoft documentation on PowerShell](https://docs.microsoft.com/en-us/powershell/scripting). You might also explore recommended books or online courses for further learning, and consider joining community forums where PowerShell enthusiasts share knowledge and support each other on their scripting journeys.