In PowerShell, you can compare dates using simple comparison operators to check if one date is earlier, later, or equal to another date.
$date1 = Get-Date "2023-01-01"
$date2 = Get-Date "2023-12-31"
if ($date1 -lt $date2) {
Write-Host "Date1 is earlier than Date2"
} elseif ($date1 -gt $date2) {
Write-Host "Date1 is later than Date2"
} else {
Write-Host "Date1 is equal to Date2"
}
Understanding Dates in PowerShell
What is a Date Object?
In PowerShell, a date is represented as a DateTime object, which allows for extensive manipulation and comparison of dates and times. This object not only holds various date and time values but also provides methods to perform operations involving these values.
Creating Date Objects
Creating date objects in PowerShell can be accomplished in several ways, each suited for different use cases.
-
Using `Get-Date`: This cmdlet fetches the current date and time based on your system's time.
$currentDate = Get-Date
This creates a DateTime object storing the current date and time.
-
Specifying Custom Dates: You can create date objects for specific dates using `[datetime]::Parse()`.
$specificDate = [datetime]::Parse("2023-10-15")
This flexibility allows for easy manipulation and retrieval of date data throughout your scripts.
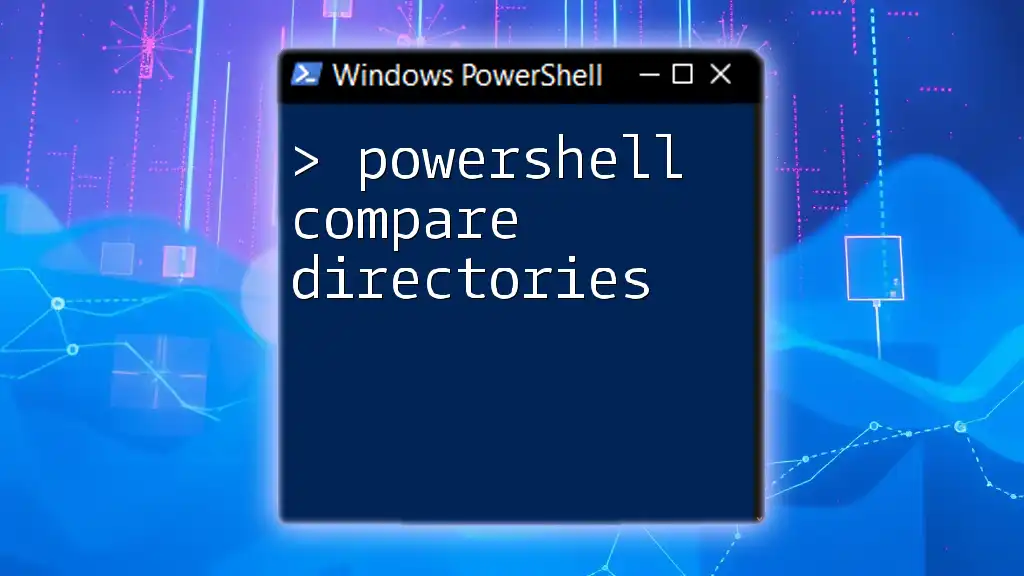
Basic Comparison Techniques
Comparing Date Objects Directly
PowerShell enables you to compare date objects using basic comparison operators like `-eq`, `-gt`, and `-lt`. This allows you to assess whether one date is equal to, greater than, or less than another date.
$date1 = Get-Date "2023-10-01"
$date2 = Get-Date "2023-10-15"
if ($date1 -lt $date2) {
Write-Host "Date1 is earlier than Date2"
}
In this example, if `date1` is less than `date2`, the statement will execute, displaying a message confirming that comparison.
Using `Where-Object` for Filtering Dates
The `Where-Object` cmdlet is particularly useful for filtering collections of date objects based on specific conditions.
$dates = @(
Get-Date "2023-10-01",
Get-Date "2023-10-15",
Get-Date "2023-11-01"
)
$filteredDates = $dates | Where-Object { $_ -gt (Get-Date "2023-10-10") }
In this snippet, the `Where-Object` filters any date from the array that is greater than October 10, 2023, allowing you to focus on relevant dates.
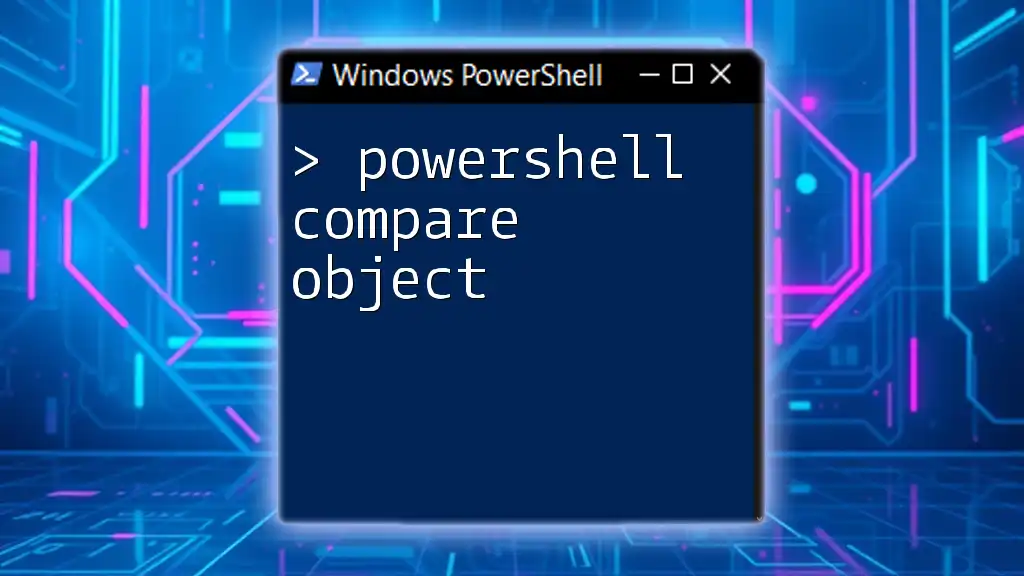
Advanced Date Comparison Methods
Calculating the Difference Between Dates
One of the major advantages of working with date objects is the ability to calculate the time difference between two dates. This can be done using the `Subtract()` method.
$date1 = Get-Date "2023-10-01"
$date2 = Get-Date "2023-10-15"
$diff = $date2.Subtract($date1)
Write-Host "The difference is $($diff.Days) days."
This script calculates the difference in days between the two dates and displays it. This technique can be useful in a variety of applications, such as calculating age or the duration of a project.
Comparing Dates with Time Components
When comparing dates, it is essential to include the time component, especially if your script involves timestamps.
$dateTime1 = Get-Date "2023-10-15 10:00 AM"
$dateTime2 = Get-Date "2023-10-15 2:00 PM"
if ($dateTime1 -lt $dateTime2) {
Write-Host "DateTime1 is earlier than DateTime2"
}
In this example, if `dateTime1` occurs before `dateTime2`, the script confirms the comparison, further showcasing PowerShell's ability to handle complex date comparisons that involve time.
Date Range Comparisons
Sometimes you may need to check if a specific date falls within a defined date range. This can be done using conditional statements.
$startDate = Get-Date "2023-10-01"
$endDate = Get-Date "2023-10-31"
$checkDate = Get-Date "2023-10-15"
if ($checkDate -ge $startDate -and $checkDate -le $endDate) {
Write-Host "CheckDate is within the range."
}
This conditional check confirms whether `checkDate` falls between `startDate` and `endDate`, making your script versatile for date validation tasks.
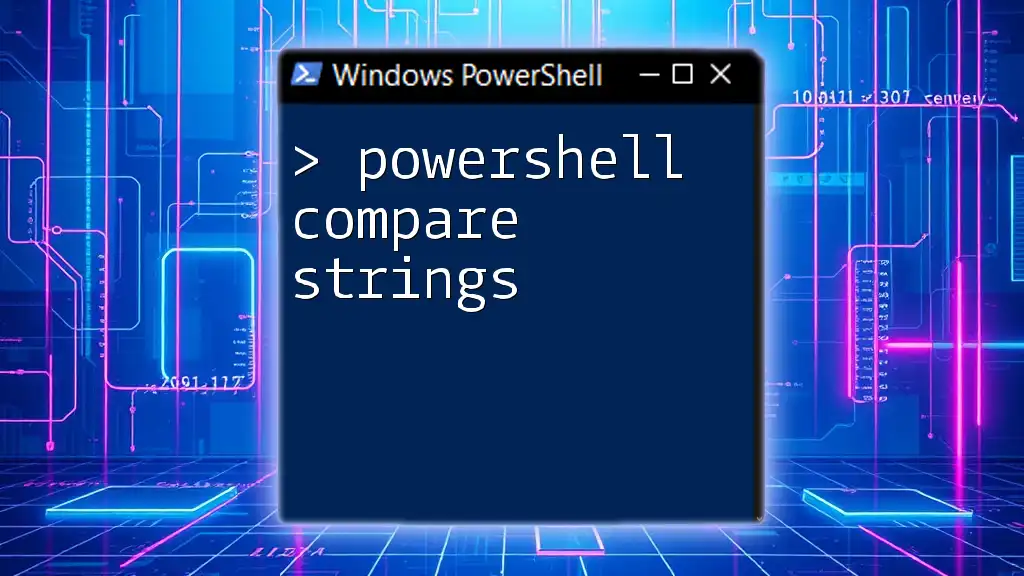
Real-World Use Cases for Date Comparison in PowerShell
Automating Report Generations Based on Date Comparison
Date comparisons play a crucial role in operations such as report generation. By assessing the last modified dates of files, you can automate the retrieval of up-to-date reports.
$files = Get-ChildItem -Path "C:\Reports" | Where-Object { $_.LastWriteTime -gt (Get-Date).AddDays(-7) }
This script collects files modified in the last week, ensuring that your reports are always recent.
Managing Logs and Events Using Dates
You can use PowerShell to filter logs based on dates, making it easier to manage and analyze log files.
$logs = Get-Content "C:\Logs\system.log" | Where-Object { $_ -match (Get-Date -Format "yyyy-MM-dd") }
This snippet extracts logs from the current day by matching the date format, allowing you to focus on real-time events.
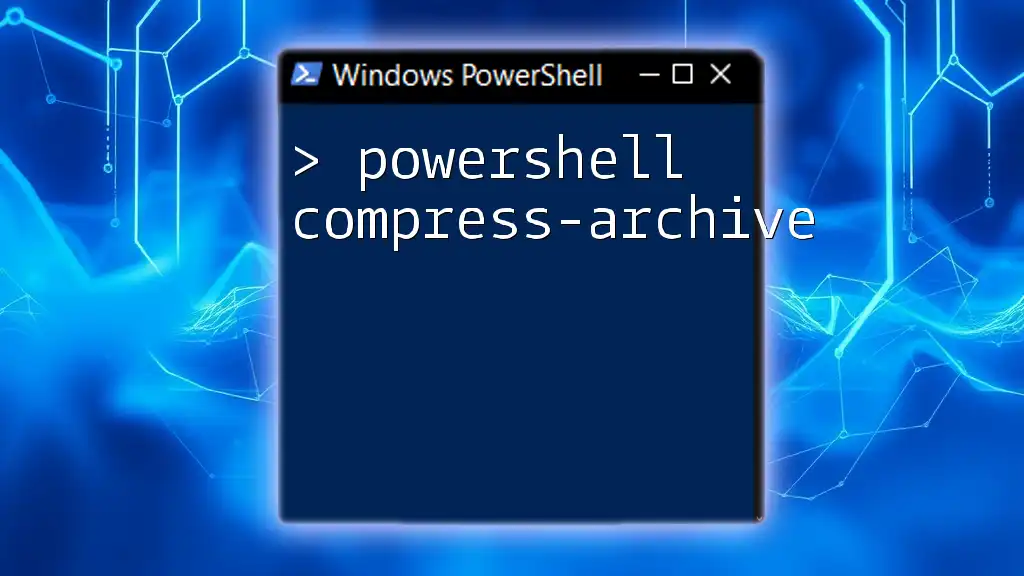
Troubleshooting Common Date Comparison Issues
Timezone Issues
When comparing dates across different time zones, it's crucial to account for this disparity. PowerShell's `Get-Date` cmdlet allows you to specify time zones, ensuring accurate comparisons.
$dateUTC = Get-Date -AsUTC
This retrieves the current date in Coordinated Universal Time (UTC), which helps standardize your comparisons.
Format Mismatches
Different date formats can lead to errors during comparisons. Always validate that date formats are consistent throughout your scripts. You can convert dates to a uniform format using the `ToString()` method.
$formattedDate = $specificDate.ToString("yyyy-MM-dd")
By ensuring a consistent format, you can minimize errors and improve reliability in your date comparisons.
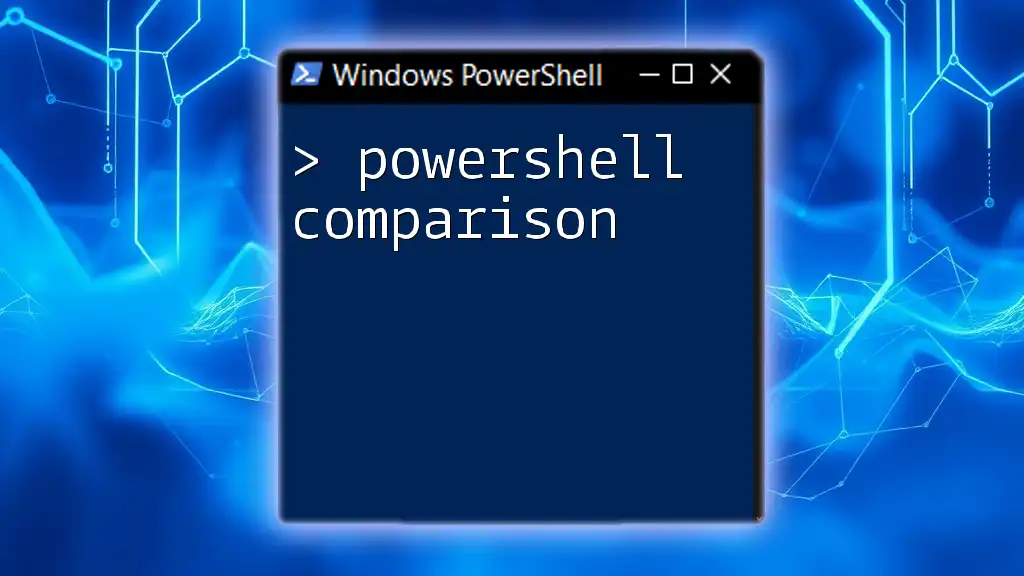
Summary
In this guide on PowerShell compare dates, we covered the essential techniques for creating, comparing, and managing date objects in PowerShell. From basic comparison methods to advanced calculations and filtering, PowerShell provides robust tools for handling date manipulations effectively. By applying these concepts, you can improve your scripting automation and enhance your workflows.
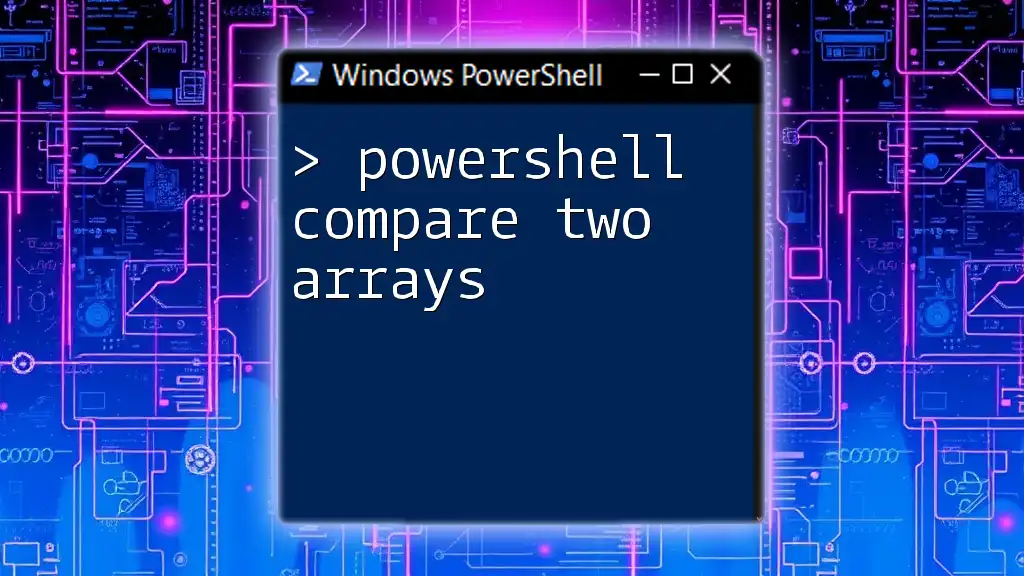
Additional Resources
For further study, refer to the official Microsoft documentation on [datetime objects in PowerShell](https://learn.microsoft.com/en-us/powershell/scripting/developer/cmdlet/how-to-use-datetime-objects). Additionally, exploring popular scripts and tools can expand your knowledge of date management in PowerShell.
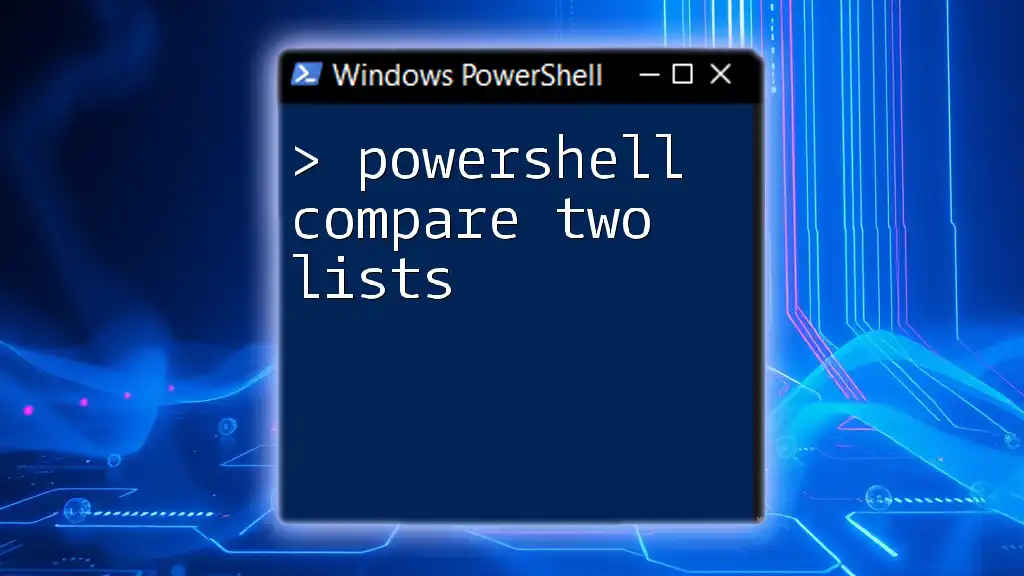
Call to Action
If you found this article helpful, subscribe for more expert tips and tricks on PowerShell. Share your own date comparison challenges or success stories in the comments, and let's continue the conversation!