The `Get-Date` cmdlet in PowerShell allows you to convert a string representation of a date and time into a DateTime object, facilitating easier date manipulations and formatting.
Here’s a code snippet demonstrating its usage:
$dateString = "2023-10-01 14:30"
$datetime = Get-Date $dateString
Write-Host $datetime
Understanding DateTime Objects in PowerShell
What is a DateTime Object?
A DateTime object in PowerShell represents an instant in time, typically expressed as a date and time of day. This object is essential for manipulating and managing time-related data in your scripts. In PowerShell, DateTime objects allow you to perform various operations such as comparisons, formatting, and arithmetic. They are indispensable when automating tasks that require precise timing, such as scheduling or logging activities.
Common Use Cases for DateTime Objects
DateTime objects can be leveraged in a wide array of scenarios, including:
- File Timestamp Management: Automatically updating file timestamps when data is modified or created can enhance organization and enable effective data management.
- Logging Events: Capturing the exact time an event occurs in logs helps in tracking system performance, debugging, and auditing.
- Scheduling Tasks: Automating scheduled tasks based on specific time frames is streamlined with the utility of DateTime information.
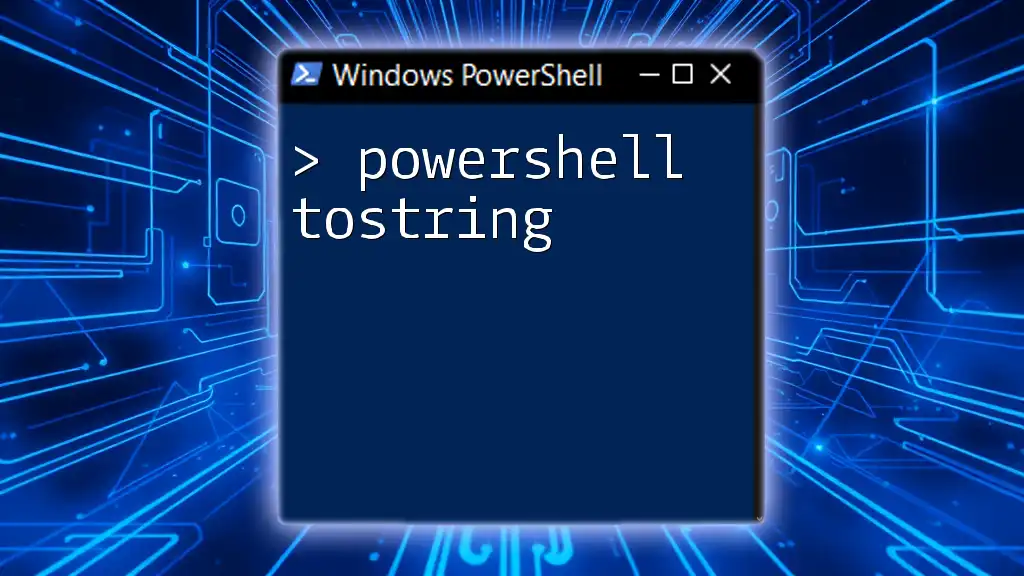
The ToDateTime Method in PowerShell
Overview of the ToDateTime Method
The ToDateTime method is a powerful feature in PowerShell that allows you to convert various formats of date and time strings into DateTime objects. This is critical for ensuring that dates are treated correctly in scripts and operations.
Syntax and Parameters
The basic syntax of the ToDateTime method is straightforward. You can use it as follows:
[datetime]::Parse("date_string")
The `Parse` method takes a date string and converts it to a DateTime object. You may also encounter the `ParseExact` method, which offers greater control by specifying the exact format of the input string.
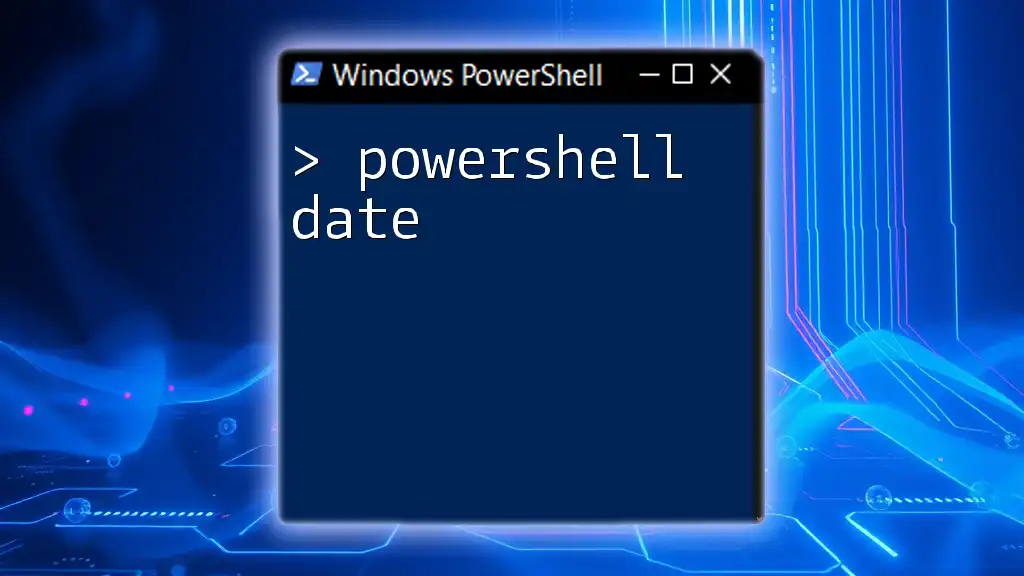
PowerShell String to Date Conversion
How to Convert Strings to DateTime
In PowerShell, there are several string formats that can be converted into DateTime objects. Familiarizing yourself with these formats is essential for effective scripting. Common formats include:
- `MM/dd/yyyy` for dates like 12/25/2023
- `dd-MM-yyyy` for dates like 25-12-2023
- `yyyy/MM/dd` for dates like 2023/12/25
Using ToDateTime for Conversion
Simple Date Conversion
To perform a basic conversion from a string to a DateTime object, you can use the following example:
$dateString = "12/31/2023"
$dateTime = [datetime]::Parse($dateString)
Write-Output $dateTime
In this case, the output will be `12/31/2023 00:00:00`, signifying midnight of that date.
Handling Different Date Formats
When dealing with various string formats, using `ParseExact` can be particularly beneficial. Here's how to convert a date string in a specific format:
$dateString = "31-Dec-2023"
$dateTime = [datetime]::ParseExact($dateString, "dd-MMM-yyyy", $null)
Write-Output $dateTime
This will convert the string into a DateTime object based precisely on the provided format.
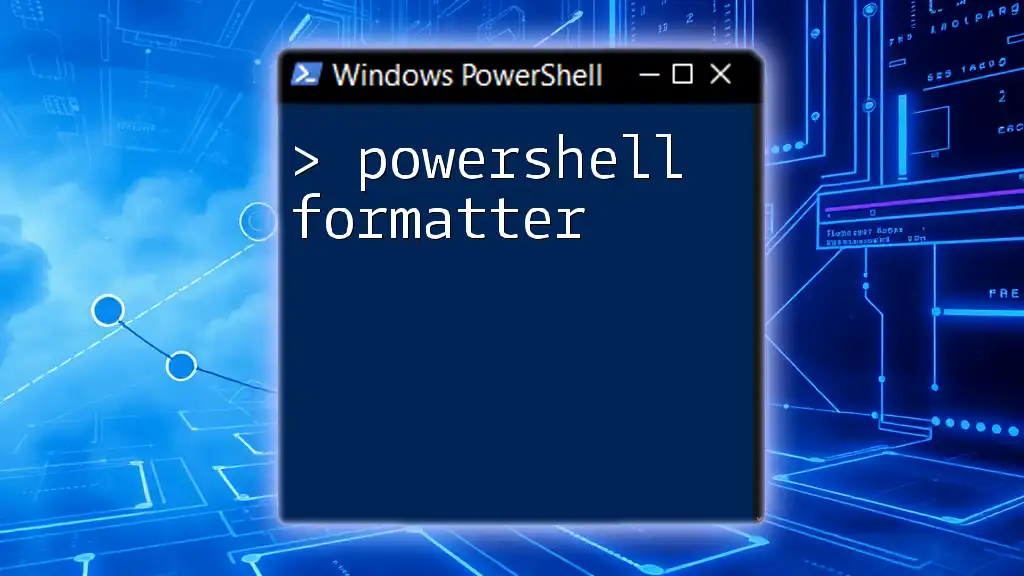
Dealing with Errors in DateTime Conversion
Common Conversion Errors
While converting strings to DateTime objects, you may encounter common errors, such as FormatException, which often occurs when the string does not conform to a recognized date format. It is essential to handle these issues explicitly to avoid script failures.
Using `Try-Catch` for Error Handling
To improve your script's resilience, you can employ a `try-catch` block for error handling. This enables your script to catch and manage exceptions gracefully. Here's an example:
try {
[datetime]::Parse("Invalid Date String")
} catch {
Write-Error "Date conversion failed: $_"
}
By implementing error handling, you can log the errors or take alternative actions without halting your script entirely.
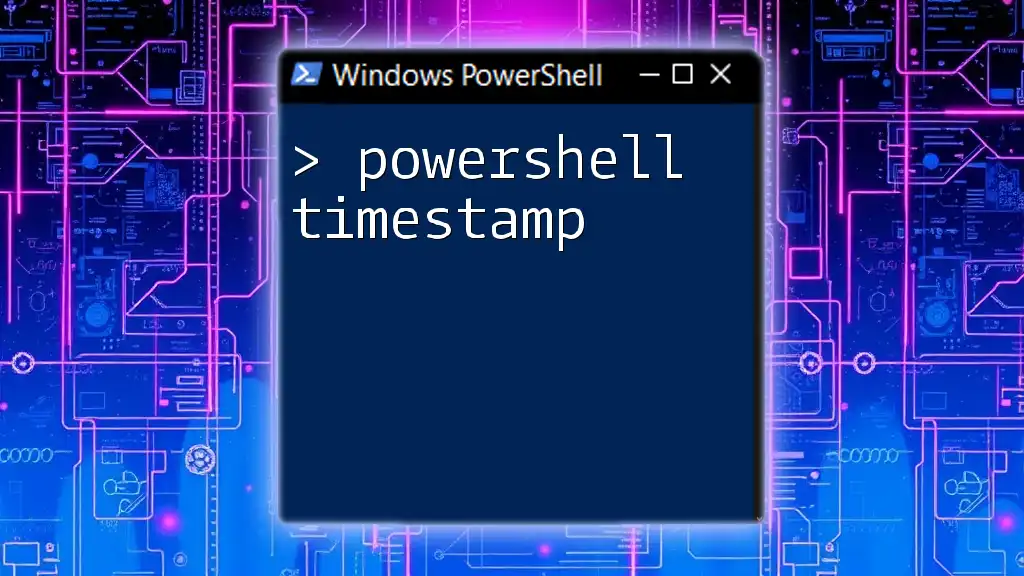
Practical Scenarios and Examples
Creating Scripts for Automated Date Handling
PowerShell scripts often perform actions based on the current date and time. For example, consider a script that logs an activity with a timestamp:
$logEntry = "Log entry at $(Get-Date)"
Add-Content -Path "C:\Logs\activity.log" -Value $logEntry
Here, `Get-Date` retrieves the current date and time, and `Add-Content` appends the log entry to a specified file. This clearly illustrates the convenience of managing DateTime in scripts.
Date Calculations using ToDateTime
Another powerful application of DateTime objects is performing calculations. For instance, you can easily add days to the current date:
$startDate = [datetime]::Now
$endDate = $startDate.AddDays(30)
In this case, `$endDate` will hold a DateTime object that represents the current date plus 30 days, which can be useful for setting up deadlines or reminders.
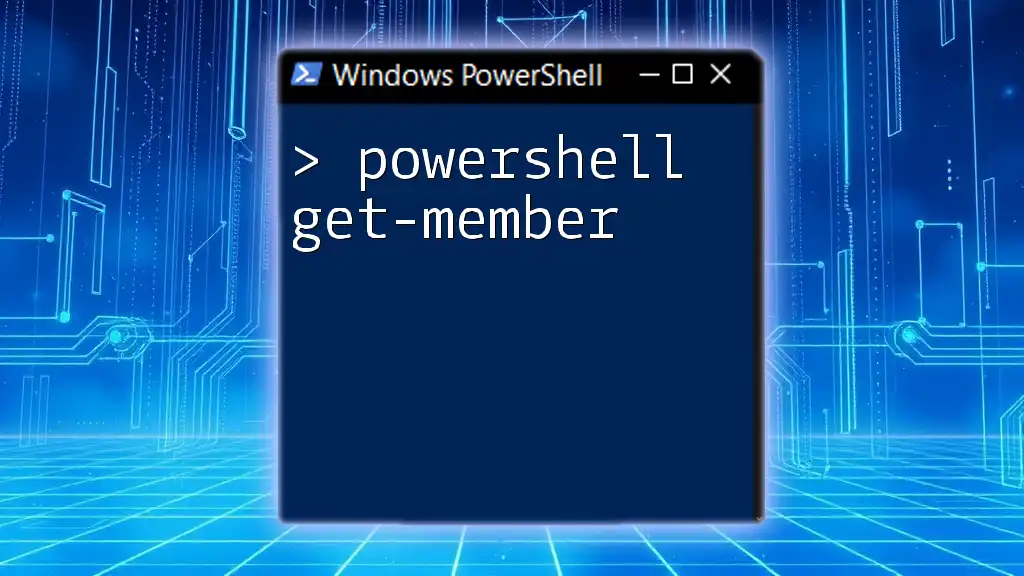
Conclusion
Mastering the ToDateTime method and understanding how to convert strings to DateTime objects are crucial skills for anyone looking to leverage PowerShell effectively. By employing various techniques like `Parse` and `ParseExact`, you can efficiently manage date and time data in your scripts.
Practice is key to confidence in using these commands: experiment with different formats, manage errors, and explore real-world scenarios to enhance your PowerShell expertise. As you continue your journey, look into additional resources and communities dedicated to PowerShell for further learning and inspiration.