PowerShell date math allows users to easily manipulate and calculate date and time values using simple commands and expressions.
Here’s a code snippet to add 30 days to the current date and display the result:
$futureDate = (Get-Date).AddDays(30)
Write-Host "The date 30 days from today is: $futureDate"
Understanding DateTime Objects in PowerShell
What is a DateTime Object?
In PowerShell, a DateTime object represents a specific point in time. It encapsulates not just the date, but also the time down to the nanosecond. This is crucial for performing various mathematical operations related to dates and times, especially when automating scripts or managing system tasks.
Creating DateTime Objects
To create a DateTime object in PowerShell, you can use the `Get-Date` cmdlet, which retrieves the current date and time. For example, you can initialize a variable with the current date as follows:
$currentDate = Get-Date
If you wish to create a DateTime object for a specific date, you can do so by passing a string representation of the date to the Get-Date cmdlet:
$specificDate = Get-Date "2023-10-01"
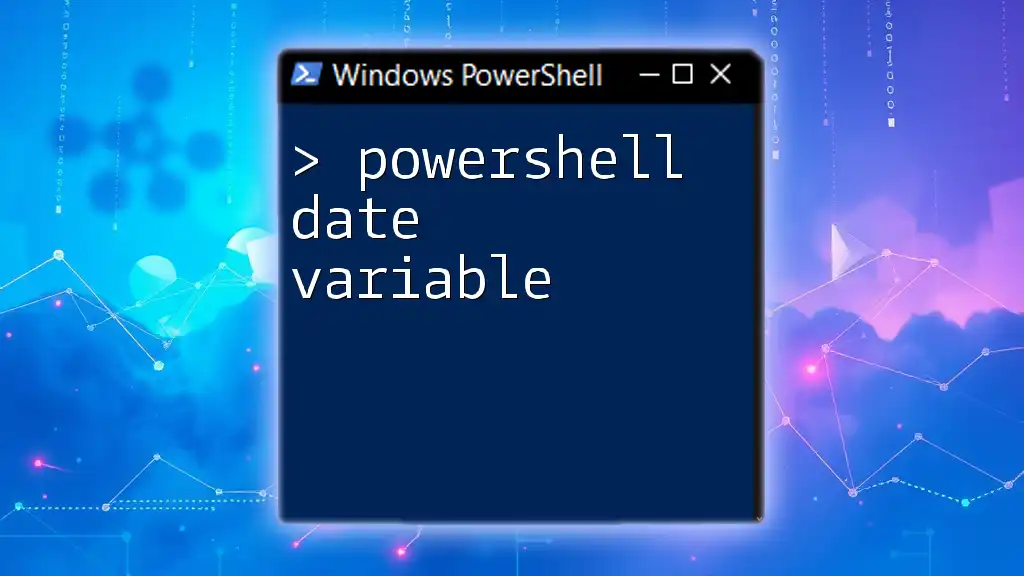
Basic Date Math Operations
Adding Days, Months, and Years
PowerShell provides several methods to perform date arithmetic easily. For instance, you can add days, months, or years to a DateTime object using the `AddDays()`, `AddMonths()`, and `AddYears()` methods. Here’s how you can do it:
$tomorrow = $currentDate.AddDays(1)
$nextMonth = $currentDate.AddMonths(1)
$nextYear = $currentDate.AddYears(1)
Each of these lines calculates a future date based on the current date. For example, `$tomorrow` will contain the date corresponding to tomorrow.
Subtracting Days, Months, and Years
Just as you can add to a date, you can also subtract using the same methods with negative values. For example:
$yesterday = $currentDate.AddDays(-1)
$lastMonth = $currentDate.AddMonths(-1)
These calculations are handy for scenarios where you need to reference past dates.
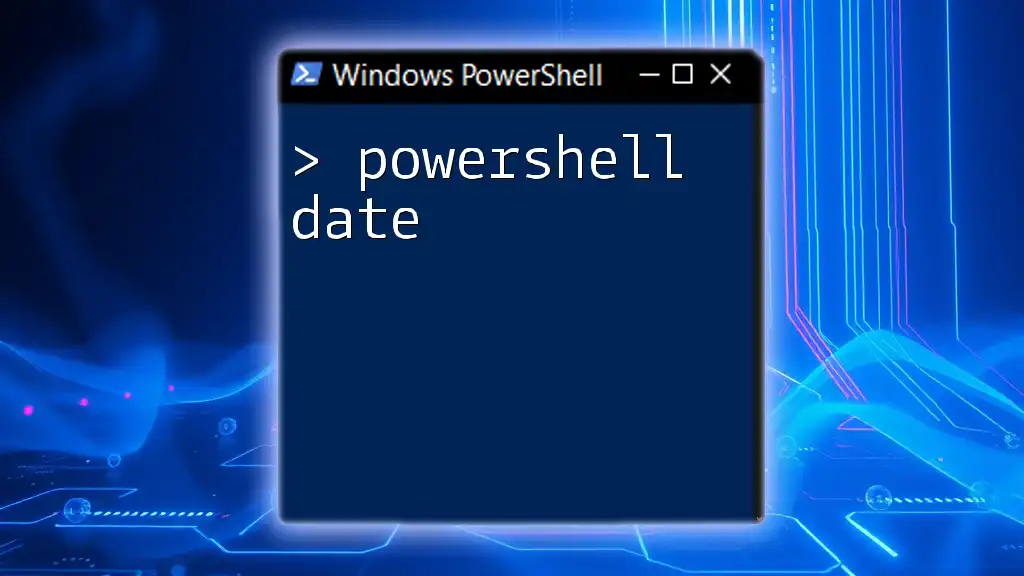
Advanced Date Calculations
Calculating Date Differences
One powerful feature of PowerShell is the ability to find the difference between two dates. When you subtract one DateTime object from another, you receive a TimeSpan object, which represents the duration between those two times:
$dateDiff = $specificDate - $currentDate
You can then access properties of the `TimeSpan` object such as `.Days`, `.Hours`, `.Minutes`, and `.Seconds` to identify how many days, hours, minutes, or seconds the difference represents.
Finding the Next Specific Day of the Week
If you need to determine the next occurrence of a specific day of the week, you can achieve that through a little bit of logic. Here’s how you can find the next Monday, for example:
$daysAhead = (([int][DayOfWeek]::Monday) - [int]$currentDate.DayOfWeek + 7) % 7
$nextMonday = $currentDate.AddDays($daysAhead)
This calculation cleverly takes into account the current day of the week and determines how many days to add to get to Monday.
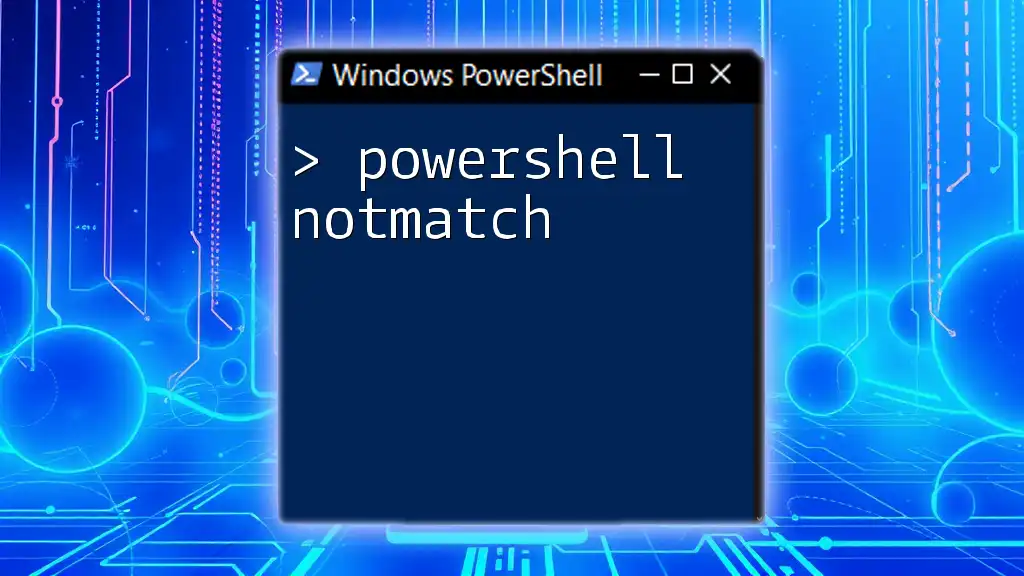
Formatting Date Outputs
Formatting Dates for Readability
Presenting dates in a readable format is crucial when displaying them in reports or user interfaces. You can use the `-Format` parameter with Get-Date to customize the output format:
$formattedDate = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
This command results in the current date formatted as "YYYY-MM-DD HH:MM:SS", which is often more suitable for logging or reporting.
Custom Date Formats
In addition to the predefined formats, you can create custom date formats using the `.ToString()` method on a DateTime object. For example:
$customFormat = $currentDate.ToString("dd/MM/yyyy")
This outputs the date in the "DD/MM/YYYY" format, which is useful in various locales.
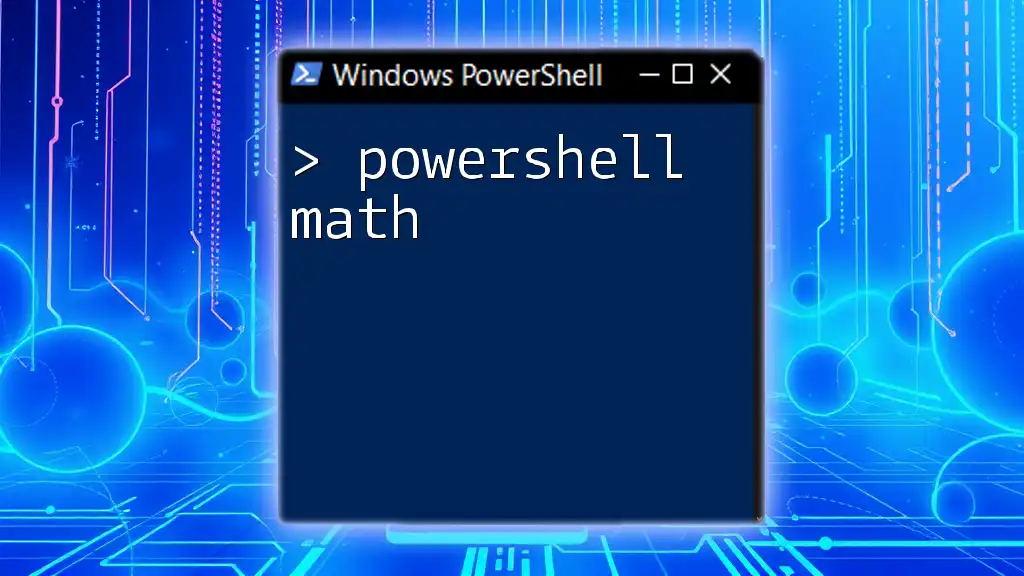
Practical Examples
Example 1: Scheduling Reminders
PowerShell date math can be particularly useful in scheduling reminders. You can easily calculate a future date to set a reminder:
$reminderDate = $currentDate.AddDays(3)
Write-Host "Your reminder is set for: " $reminderDate
This simple script informs the user that a reminder is set three days from the current date.
Example 2: Log File Retention Policy
Understanding how to manipulate dates can significantly aid in system maintenance tasks, such as cleaning up log files. You can check the age of log files in a specified directory and delete those older than 30 days with a script like this:
$logFiles = Get-ChildItem "C:\Logs"
foreach ($file in $logFiles) {
if ((Get-Date) - $file.CreationTime -gt (New-TimeSpan -Days 30)) {
Remove-Item $file.FullName
}
}
This script streamlines a regular maintenance task, ensuring that log files are managed effectively.
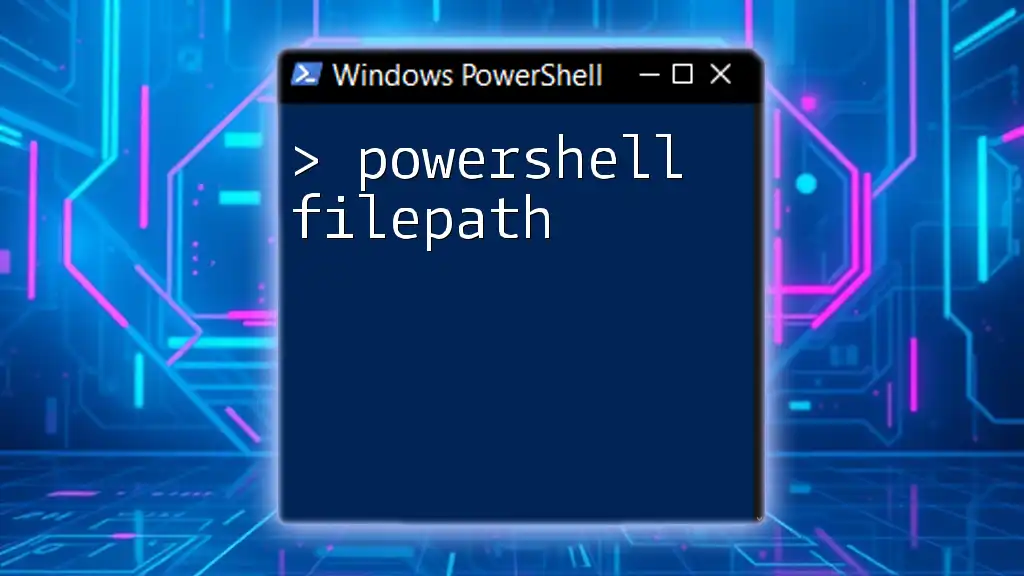
Conclusion
In this article, we explored the concept of PowerShell date math in depth, covering everything from understanding DateTime objects to performing essential calculations and formatting outputs. By leveraging these techniques, you can automate numerous tasks involving date manipulations, making your PowerShell scripting not only powerful but also efficient.
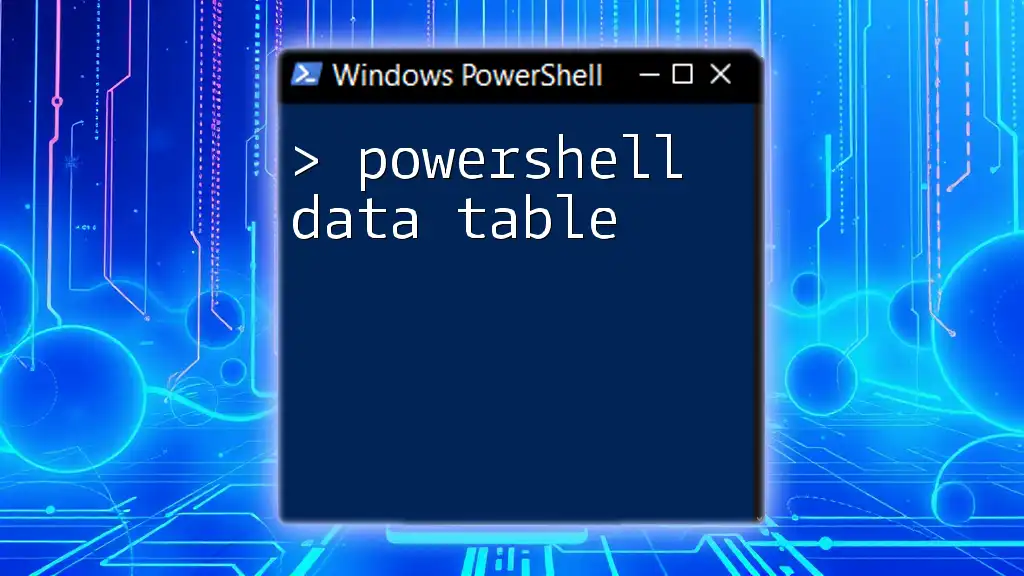
Additional Resources
For further learning, consider referring to the official PowerShell documentation. Additionally, there are various online courses and community forums dedicated to PowerShell scripting where you can continue to expand your knowledge.