In PowerShell, a file path is a string that specifies the location of a file or directory in the file system, allowing users to easily reference and manipulate files.
Get-ChildItem 'C:\Users\YourUsername\Documents'
Understanding File Paths in PowerShell
What is a FilePath?
A file path refers to the specific location where a file or folder resides within a file system. Understanding file paths is essential when working with PowerShell commands, as it determines how you access, modify, or run operations on files and directories. There are two primary types of file paths:
- Absolute FilePath: This provides the complete path to the file, starting from the root of the file system. For instance, `C:\Users\Example\Documents\File.txt` is an absolute path.
- Relative FilePath: This describes the location of a file relative to the current working directory. For example, if your current directory is `C:\Users\Example`, the relative path to the `Documents` folder would be `Documents\File.txt`.
Structure of File Paths
A traditional file path typically consists of several components:
- Drive Letter: Indicates the storage device (e.g., C:).
- Folder Names: Represents the hierarchy of directories leading to the file (e.g., `Users\Example\Documents`).
- File Name + Extension: The actual name of the file along with its extension (e.g., `File.txt`).
Understanding this structure is crucial for effectively using PowerShell file commands.
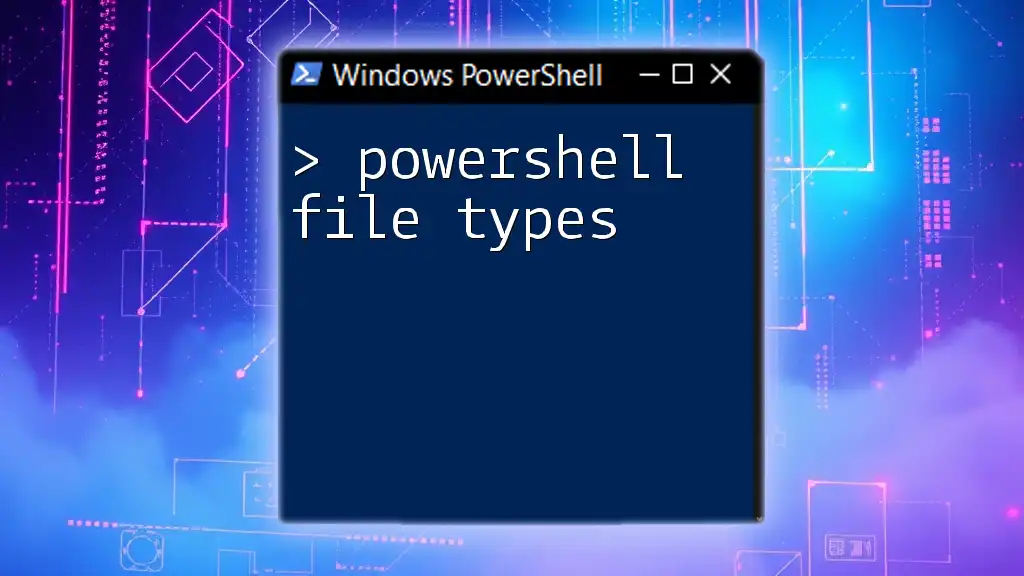
Working with FilePaths in PowerShell
Basic Commands for FilePath Management
Get-ChildItem
This command allows you to list the files and folders within a specified path. It's a versatile command useful for directory exploration.
Example usage:
Get-ChildItem -Path C:\Example
In this example, `Get-ChildItem` lists all items within the `C:\Example` directory, providing essential information such as file names and types.
Set-Location
This command is used to change the current working directory. Changing directories is a frequent task that helps streamline file management operations.
Example usage:
Set-Location -Path C:\Example
After executing this command, your working directory will change to `C:\Example`, allowing you to reference files relative to this new location.
Test-Path
This cmdlet checks whether a specified file or directory exists in the given path, which is essential for validation before performing file operations.
Example usage:
Test-Path C:\Example\File.txt
If the file exists, `Test-Path` returns `True`; otherwise, it returns `False`. This functionality prevents errors when attempting to access non-existent files.
Navigating Directories
Using CD Command
PowerShell supports the `cd` command, a familiar shorthand for changing directories.
Example usage:
cd C:\Users\Example\Downloads
This command will move the current directory to the `Downloads` folder under `C:\Users\Example`, establishing a new reference point for subsequent commands.
Changing Drives
Switching between different drives is straightforward in PowerShell. Use the `Set-Location` cmdlet to change to a different drive.
Example usage:
Set-Location D:
This command allows you to change from the current drive to drive D: quickly, enabling operations within that drive.
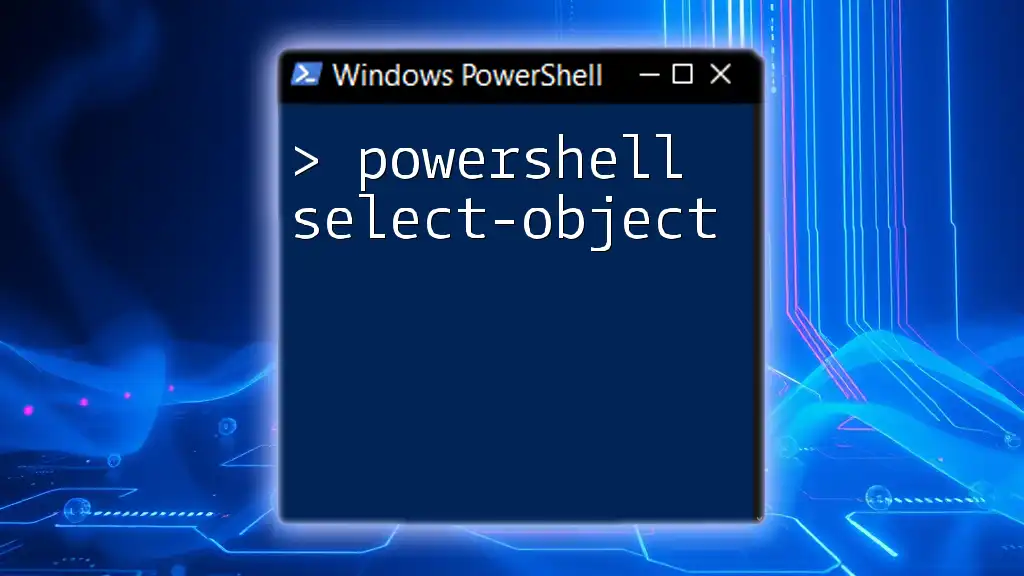
Advanced FilePath Techniques
Using Wildcards with FilePaths
What are Wildcards?
Wildcards are special characters used to represent one or more characters in search patterns. In PowerShell, the two common wildcards are `*` (which represents any number of characters) and `?` (which represents a single character).
Examples of Wildcard Usage
When managing large datasets or folders, wildcards can significantly simplify the process. One common usage is with the `Get-ChildItem` cmdlet:
Get-ChildItem -Path C:\Example\*.txt
In this example, the command retrieves all `.txt` files within the `C:\Example` directory, demonstrating how wildcards can filter results efficiently.
String Manipulation for FilePaths
Working with FilePath Strings
Creating dynamic paths is often necessary when automating tasks. PowerShell provides several string manipulation methods to simplify this process.
Example usage of `Join-Path`:
$basePath = 'C:\Users\Example'
$filePath = Join-Path -Path $basePath -ChildPath 'Documents\File.txt'
Here, `Join-Path` constructs a full file path dynamically, improving readability and reducing potential errors in manually concatenating strings.
Using Split-Path
The `Split-Path` cmdlet is beneficial for extracting specific components from a file path.
Example usage:
Split-Path -Path 'C:\Users\Example\Documents\File.txt' -Leaf
This command returns just the file name with the extension (`File.txt`), stripping away any preceding directory information, which can be particularly useful for logging or naming conventions.
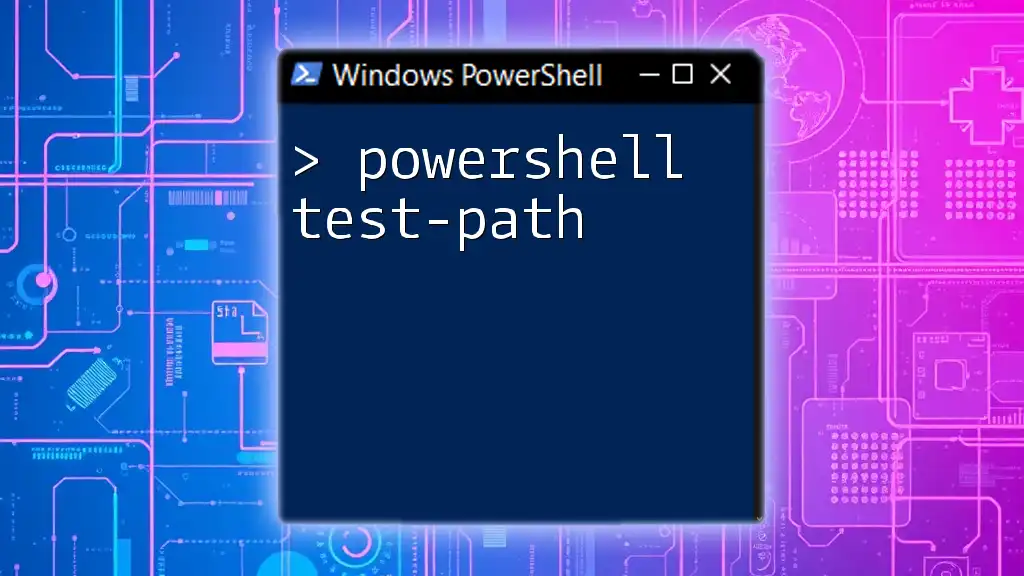
Common Errors and Troubleshooting
Common FilePath Issues
While working with file paths in PowerShell, users may encounter several common errors, such as:
- Path Not Found Errors: This occurs when the specified path does not exist. It can be due to a typo or incorrect directory structure.
- Access Denied Errors: These arise when a file or folder is restricted, preventing the script from accessing it.
Troubleshooting Techniques
To troubleshoot these issues efficiently, employing the `Test-Path` cmdlet is instrumental. Ensuring that a path exists before attempting any operations can save time and resources. Regularly checking permissions on folders or files using Windows Explorer can also provide insight into access-related problems.
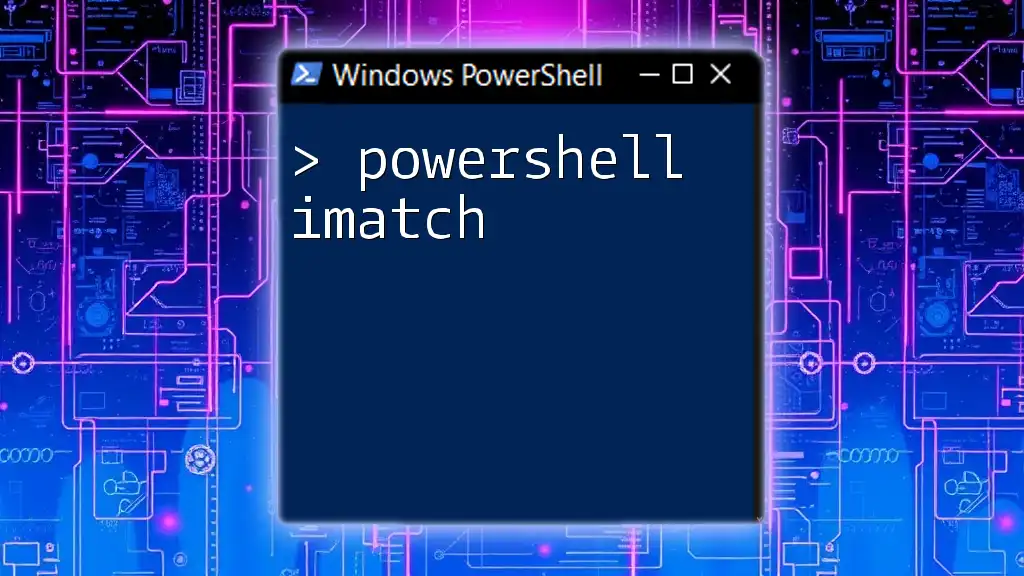
Conclusion
Mastering the concept of file paths in PowerShell is fundamental to navigating and managing files effectively. With the commands and techniques outlined in this guide, you are equipped to execute file operations confidently. As you practice, you'll find that your understanding of file paths will not only enhance your scripting skills but also lead to more efficient workflows.
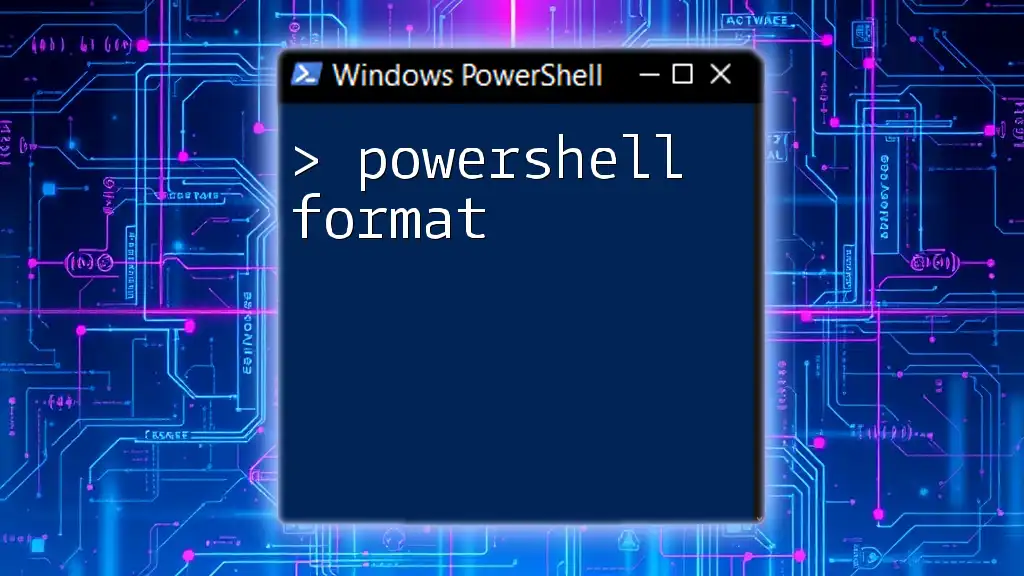
Additional Resources
For further exploration, consider consulting the official Microsoft documentation on PowerShell commands. Participating in community forums, such as those on Reddit or PowerShell.org, can also offer valuable insights and support from fellow PowerShell enthusiasts.
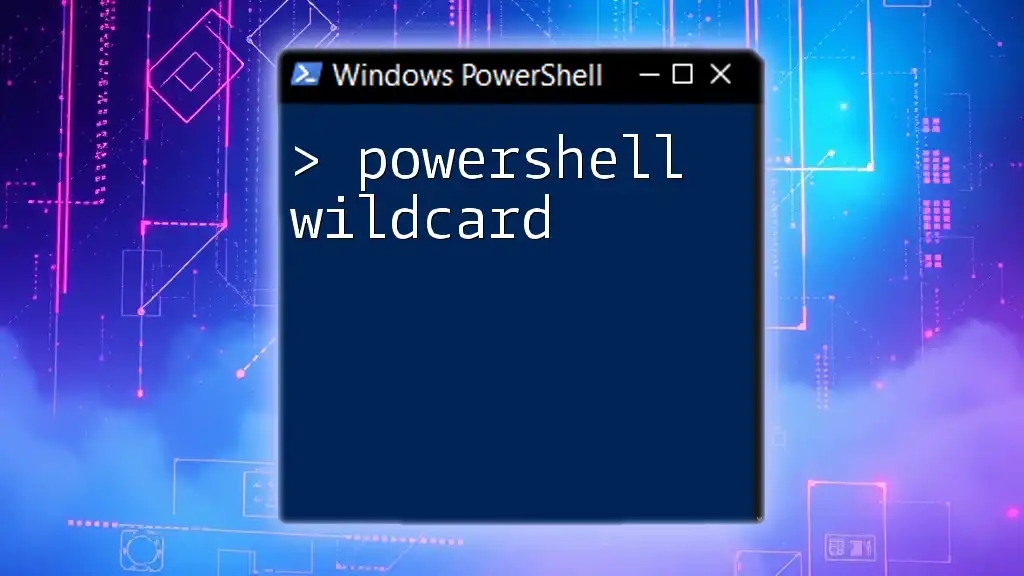
Call to Action
We encourage you to take the next step by signing up for more tutorials and insights on PowerShell usage. Feel free to share this guide with others who may benefit from a concise introduction to PowerShell file paths.