In PowerShell, a wildcard is a special character used to represent one or more characters in a string, allowing for flexible pattern matching when searching for files or running commands.
Here's an example of using a wildcard to list all text files in a directory:
Get-ChildItem *.txt
Understanding PowerShell Wildcard Characters
What are PowerShell Wildcard Characters?
Wildcard characters are special symbols used in PowerShell to represent one or more characters in strings. They are essential for efficiently searching for files, filtering processes, or querying other object properties without needing the exact names or formats. Their most common symbols in PowerShell include the asterisk (`*`) and the question mark (`?`).
Common Wildcard Characters
-
Asterisk (`*`): Represents zero or more characters. It is the most flexible wildcard since it can match any sequence of characters, including none.
-
Question Mark (`?`): Represents a single character. It can be utilized when you know some characters but not all.
Examples:
Get-ChildItem *.txt # Retrieves all text files.
Get-ChildItem file?.doc # Retrieves files like file1.doc, fileA.doc, etc.
These examples illustrate how wildcards simplify searching and filtering in PowerShell. Instead of explicitly stating each file name, you can use these characters to represent groups of files.
Special Cases and Considerations
Wildcards can behave differently when used in various contexts. For instance, using wildcards with commands that do not inherently support them may lead to unexpected results. It's crucial to understand the command you are working with so that your wildcard usage aligns with its expected syntax and functionality.
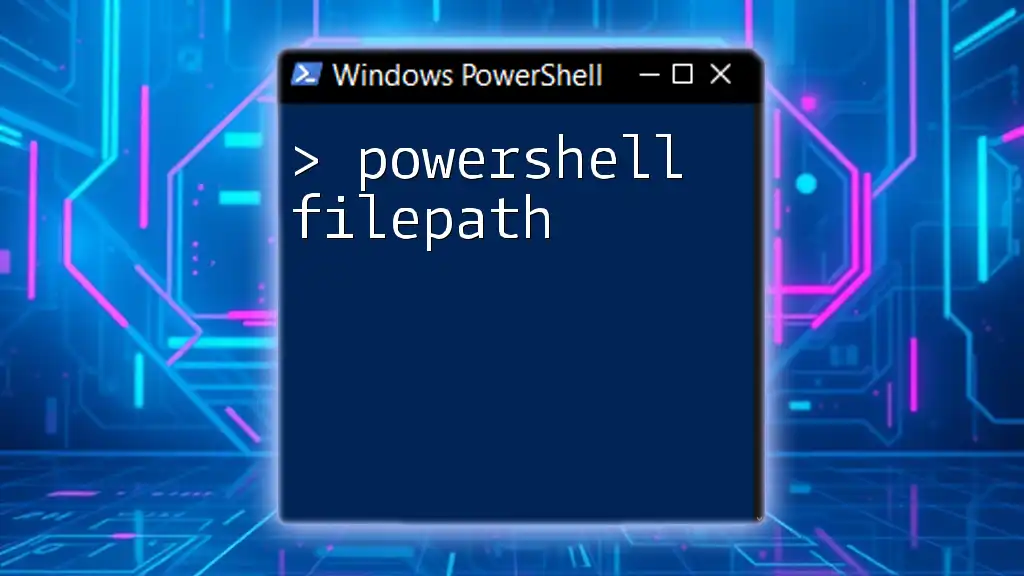
Using Wildcards in PowerShell Commands
Filtering Objects with Wildcards
Wildcards can be particularly handy when you're dealing with large sets of data. For example, when inspecting running processes, if you're looking for all instances of a specific word or substring in the process name, you can harness wildcards effectively:
Get-Process *word* # Retrieves all processes with 'word' in their name.
This command pulls up relevant processes, allowing you to pinpoint those that match your criteria quickly.
Working with Files and Directories
When dealing with files, `Get-ChildItem` combines well with wildcards. You can filter results based on file extensions or specific naming patterns. Here's how you would retrieve all Excel files in a directory:
Get-ChildItem C:\Reports\*.xlsx # Retrieves all Excel files in the Reports directory.
This approach is particularly useful for maintaining and organizing files within a directory structure.
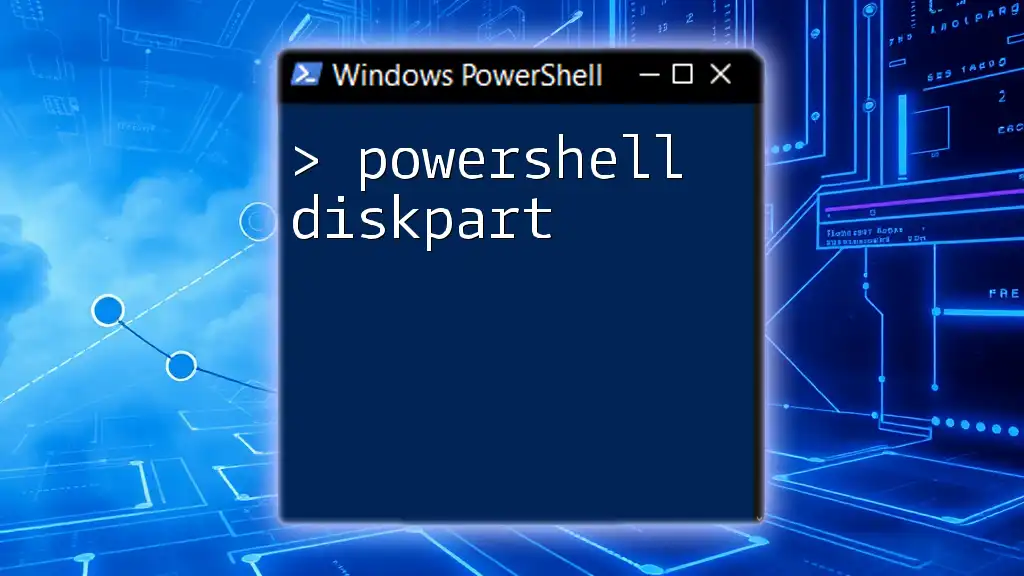
PowerShell Where Wildcard Clause
What is the Where Clause?
The `Where-Object` cmdlet allows you to filter objects in a pipeline based on specified criteria. This feature becomes powerful when combined with wildcards, as it enables you to filter more complex data sets.
Using Wildcards within Where Clauses
You can extend your wildcard capabilities with the `Where-Object` cmdlet. Here’s an example using file names to filter results dynamically:
Get-ChildItem | Where-Object { $_.Name -like '*report*' }
In this command, `$_` represents the current object in the pipeline. The `-like` operator allows you to utilize the wildcard to filter file names containing the term "report," providing you a versatile way to refine your search results.
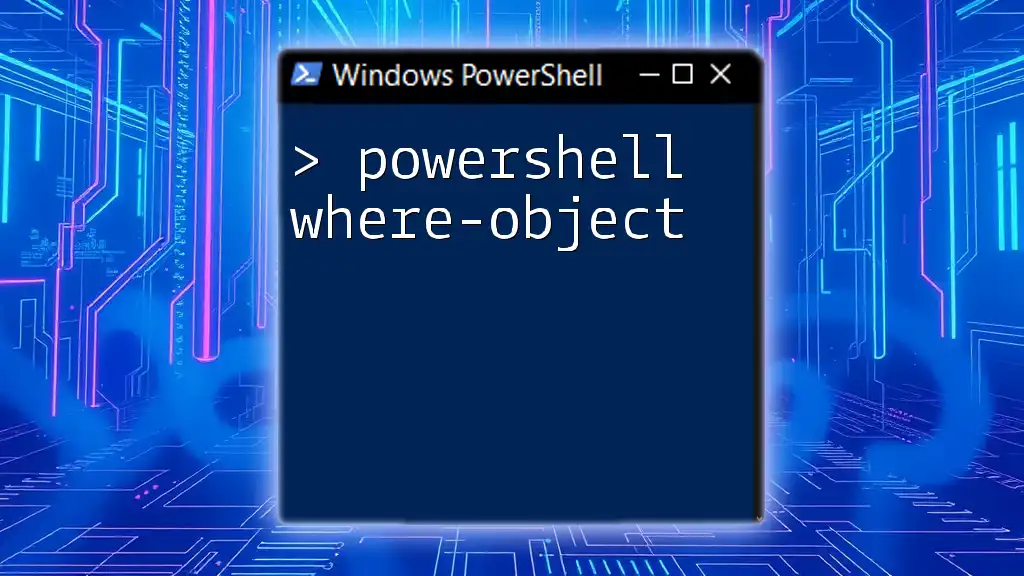
Advanced Wildcard Usage Scenarios
Combining Wildcards with Other Parameters
The real power of wildcards becomes apparent when combined with additional parameters and options. For instance, when you want to get files while traversing through subdirectories, you can harness `Get-ChildItem` along with wildcards and the `-Recurse` flag:
Get-ChildItem -Path C:\* -Recurse -Filter '*.log'
This command retrieves all log files not only from the specified directory but also from its subdirectories, showcasing the robustness of wildcards.
Wildcard Usage with Script Blocks
For more advanced scenarios, wildcards can be utilized within script blocks for intricate filtering:
$files = Get-ChildItem
$files | Where-Object { $_.Name -like '*.txt' -or $_.Name -like '*.log' }
Here, both `.txt` and `.log` patterns are used to capture files of either extension, allowing for flexible querying of the files collected.
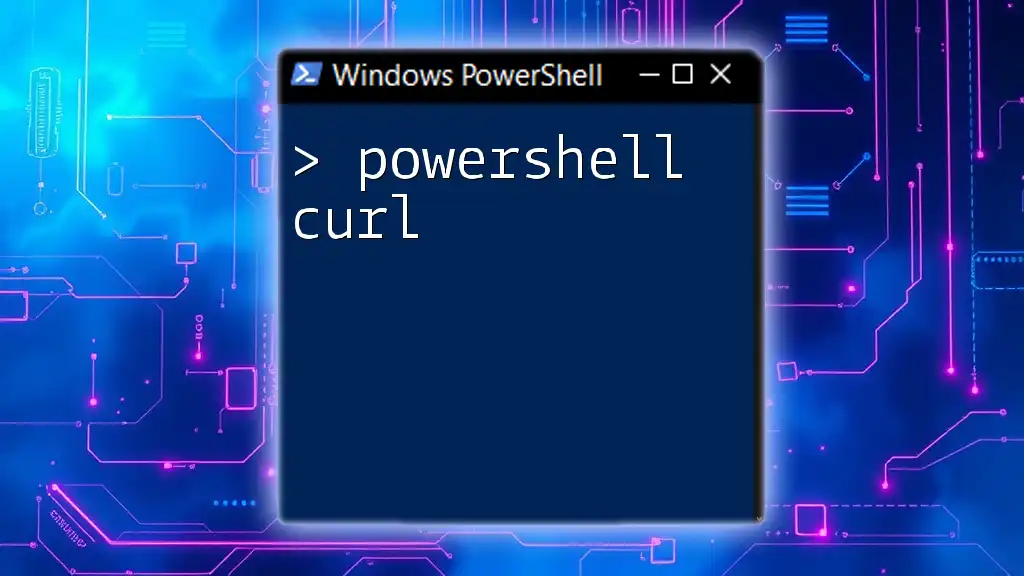
Best Practices for Using Wildcards in PowerShell
Efficiency Tips
While wildcards enhance search capabilities, overusing or improperly applying them can hinder performance. Avoid excessive wildcards, especially in large data sets, as this can lead to longer execution times. Practicing specificity where you can will generally yield better results and better performance.
Readability and Maintainability
Always prioritize writing clear code. Using too many wildcards or convoluted patterns can make scripts difficult to read and maintain. Strive for simplicity and clarity to ensure that your code remains user-friendly over time.
Testing Wildcard Patterns
Before deploying commands in environments with significant data, consider testing your wildcard patterns using `Where-Object`. Validate results in smaller batches to ensure that the command returns the desired set of objects.
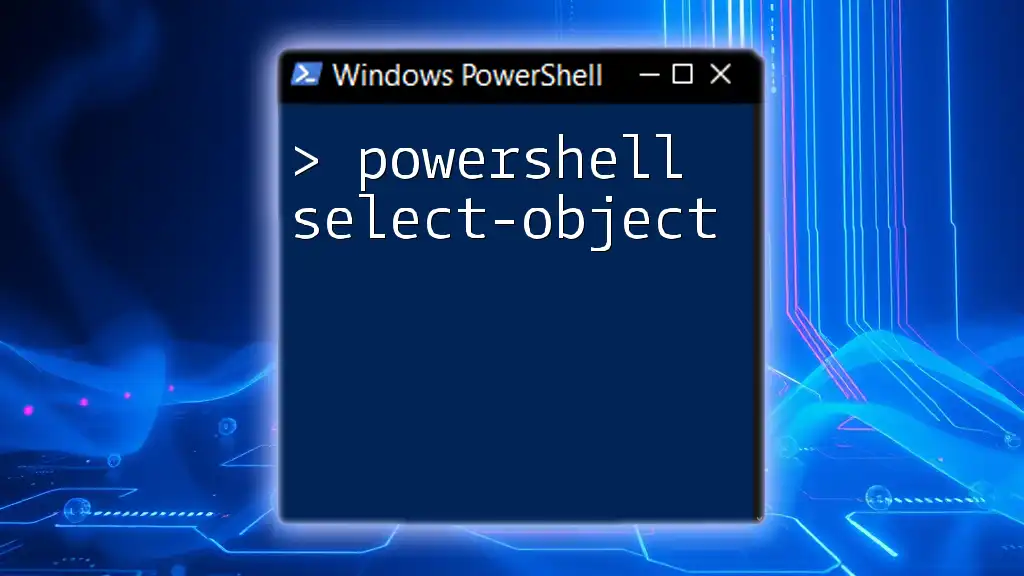
Troubleshooting Common Wildcard Issues
Understanding Wildcard Limitations
While wildcards are powerful, they do have limitations, particularly in complex file systems or with certain cmdlets. For example, when wildcards are used in improperly configured environments or in conjunction with non-compatible cmdlets, they may not yield expected results.
Common Errors and Solutions
Typical mistakes can arise from typos in wildcard patterns or misunderstanding character behaviors. For example, using `*` where a `?` is more appropriate may lead to a broader result set than intended.
To resolve these issues, always double-check if the wildcard symbolism matches your intended query. Documentation and examples can often clarify usage and prevent common pitfalls.
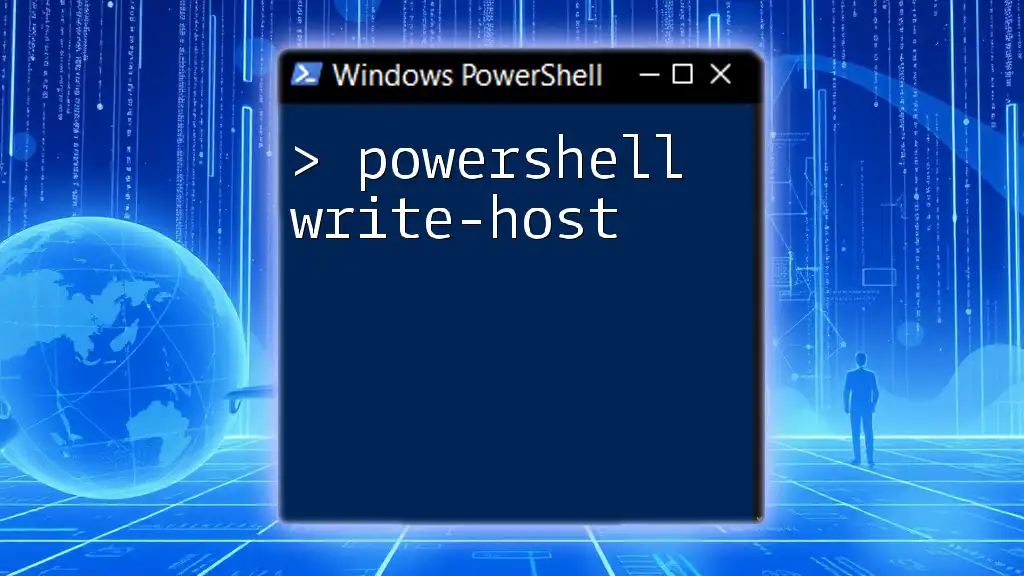
Conclusion
The proficiency of using PowerShell wildcards not only streamlines your command-line experience but also significantly enhances data retrieval capabilities. With proper understanding and practice, wildcards become a fundamental part of your PowerShell toolkit, enabling more effective and efficient management of files and processes.
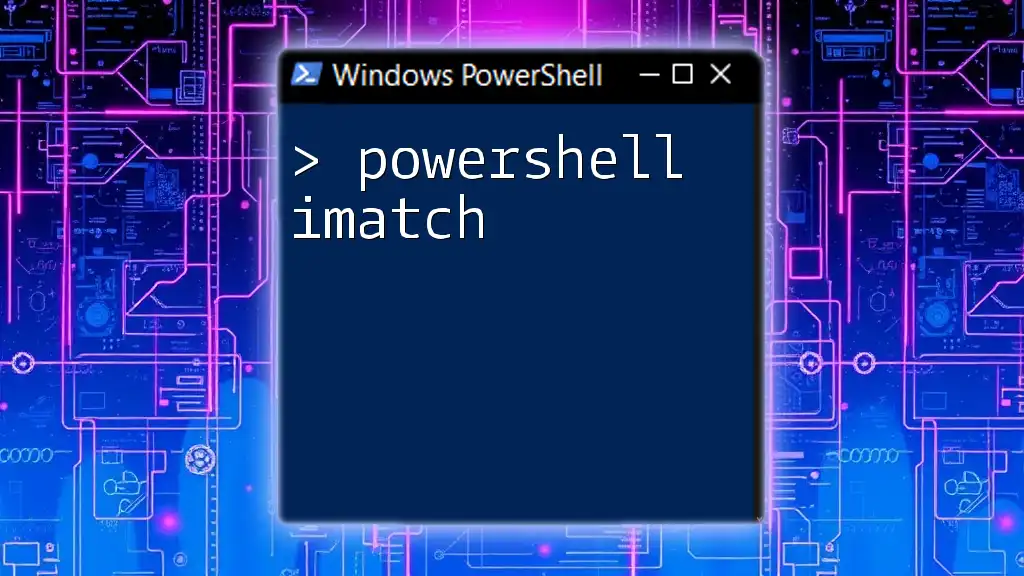
Additional Resources
For further learning, consider exploring the official Microsoft PowerShell documentation, which offers extensive details on wildcards and their usage. Engaging with online forums and communities can also provide invaluable tips and experiences from PowerShell enthusiasts and experts.