The `-imatch` operator in PowerShell performs a case-insensitive regular expression match on strings, allowing for flexible pattern matching without worrying about text casing.
Here's a code snippet demonstrating its usage:
$inputString = "Hello, World!"
if ($inputString -imatch "hello") {
Write-Host 'Match found!'
} else {
Write-Host 'No match.'
}
Understanding Regular Expressions in PowerShell
What are Regular Expressions?
Regular expressions, often abbreviated as regex, are powerful tools used to search, match, and manipulate text based on specific patterns. They are particularly useful for system administrators and developers to automate tasks such as validating data, filtering information, and performing complex string manipulations. Understanding regular expressions is essential for maximizing the functionality of PowerShell.
The Basics of `-match` vs `-imatch`
In PowerShell, the `-match` operator enables you to perform case-sensitive matching against a specified pattern. Conversely, `-imatch` is the operator designed for case-insensitive matching. Grasping this distinction is crucial when you're working with string comparisons or validations.
Importance of Case-Sensitivity:
- When to Use `-match`: Use this operator when you want to ensure exact matches, including case.
- When to Use `-imatch`: Use `-imatch` when dealing with user input or data that may vary in capitalization. This approach ensures broader matching capabilities without worrying about letter cases.
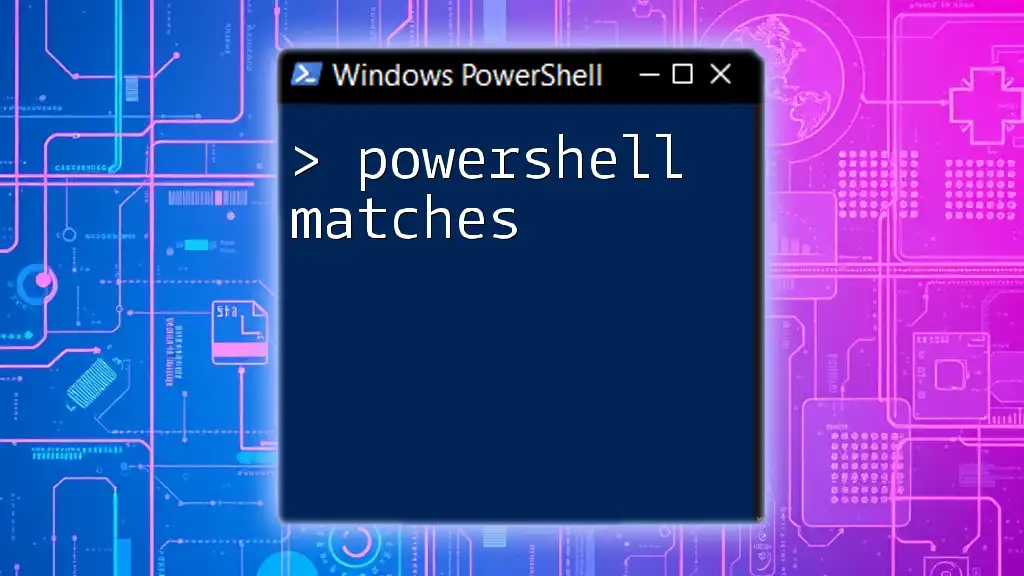
Using the `-imatch` Operator
Syntax and Parameters
The syntax for the `-imatch` operator is straightforward:
<expression> -imatch <pattern>
You can leverage this operator to search through strings, ensuring that the search is conducted without regard for letter case. Note that `<expression>` can include variables or literals, and `<pattern>` consists of a regex pattern.
Simple Examples
Example 1: Basic iMatch Usage
"Hello World" -imatch "hello"
In this example, even though "Hello" contains a capital "H," the `-imatch` operator finds a match, demonstrating the effectiveness of case insensitivity.
Example 2: Using iMatch with Variables
$greeting = "Welcome to PowerShell"
$pattern = "welcome"
$greeting -imatch $pattern
Here, the variable `$greeting` contains a string, and the pattern "welcome" is matched regardless of the initial capital "W." This showcases the flexibility of `-imatch` in real-world scenarios.
Practical Use Cases
Filtering Arrays
One of the most practical applications of `-imatch` is filtering data within arrays. For instance, consider you have an array of fruits and you want to find all fruits that contain the letter "a":
$fruits = @("Apple", "banana", "Cherry", "date")
$fruits | Where-Object { $_ -imatch "a" }
This command results in a list of fruits that have "a" in their names, showcasing how `-imatch` simplifies filtering conditions.
Validating User Input
Validating user inputs is another critical area where `-imatch` excels. Below is an example of how you can use it to validate an email format:
$input = Read-Host "Enter your email"
if ($input -imatch "^[^@\s]+@[^@\s]+\.[^@\s]+$") {
"Valid Email"
} else {
"Invalid Email"
}
In this snippet, the regex ensures that the string entered conforms to a typical email pattern while ignoring case, improving user experience during data input.
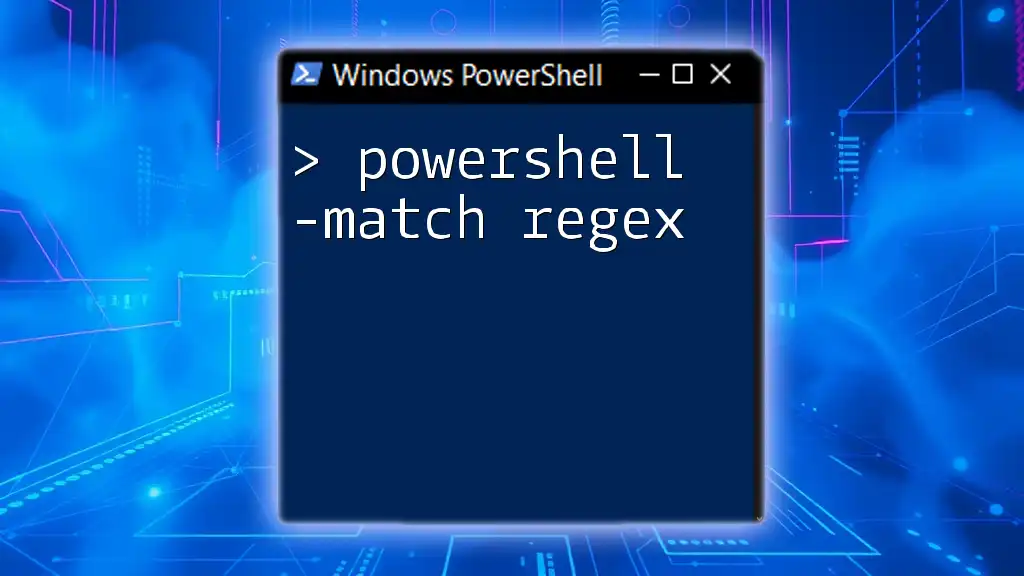
Advanced Techniques with `-imatch`
Using iMatch with Complex Patterns
The ability to utilize complex regular expression patterns with `-imatch` cannot be understated. For example, if you want to check if a password meets certain criteria, you can use:
"Password@123" -imatch "^[A-Za-z@0-9]+$"
This regex validates that the password starts with letters or numbers and may include the "@" character, providing a way to enforce password policies effectively.
Error Handling with `-imatch`
Error handling is essential when using any operator in scripting. With `-imatch`, ensure you wrap your matching code with try/catch blocks where appropriate. This can help you handle unexpected outcomes gracefully and maintain script robustness.
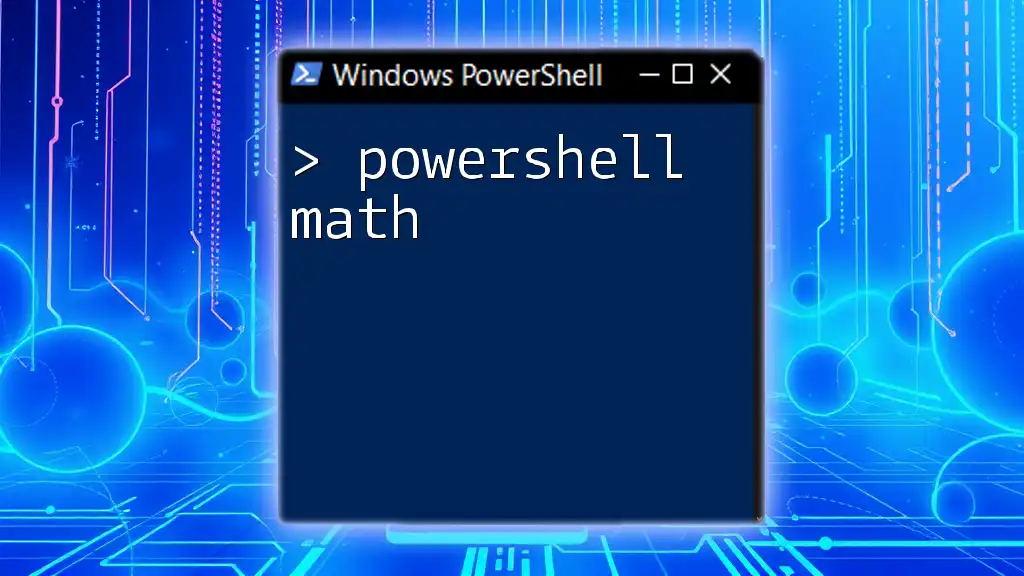
Debugging and Testing Regular Expressions
Using the `Select-String` Cmdlet
The `Select-String` cmdlet serves as a powerful tool for searching through strings or files using regex patterns. When paired with the `-imatch` operator, it provides efficient text searching capabilities.
"Sample text with a pattern" | Select-String -Pattern "pattern" -CaseSensitive:$false
This command searches for "pattern" within a string and utilizes the case-insensitive feature, making it easy to locate text without worrying about casing differences.
Tips for Building and Testing Regular Expressions
Building and testing regex patterns can be tricky. Utilize online regex testers which allow you to input both your pattern and sample text to visualize matches. Regular expressions are complex; leveraging such tools can save a considerable amount of time.
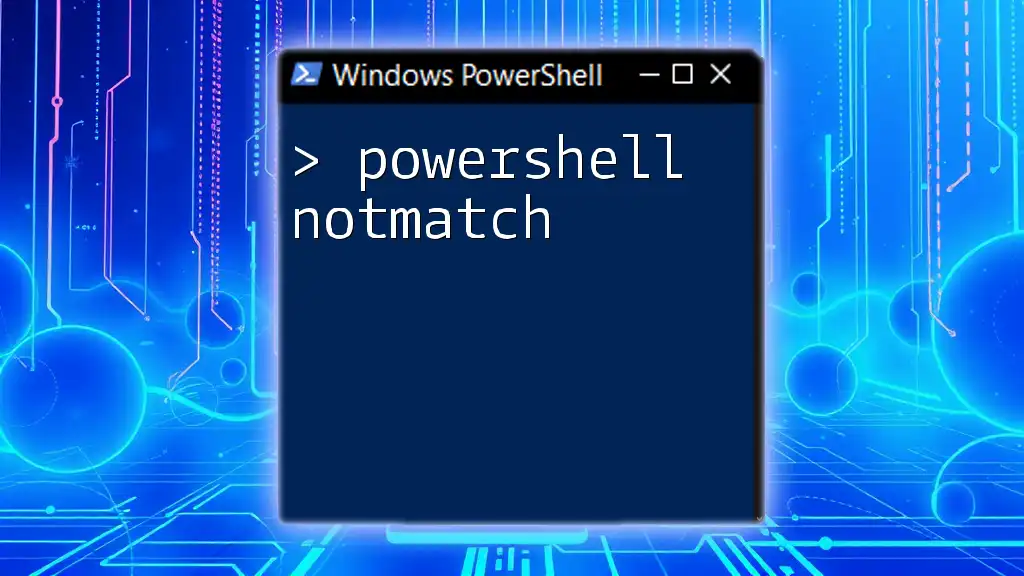
Common Mistakes to Avoid
Understanding Case-Sensitivity
Common Pitfalls: A frequent mistake is assuming that the regex used in `-match` works the same as with `-imatch`. Remember, if you omit the "i" in `-imatch`, your matches may fail unexpectedly due to case.
Forgetting to Escape Characters
When crafting regular expressions, forgetting to escape special characters can lead to incorrect matches. Consider the following example of incorrect usage:
"Can you match a dot?" -imatch "."
This will always return `True`, as the dot in regex represents any character. Conversely, using:
"Can you match a dot?" -imatch "\."
Correctly targets the period and ensures accurate matches.
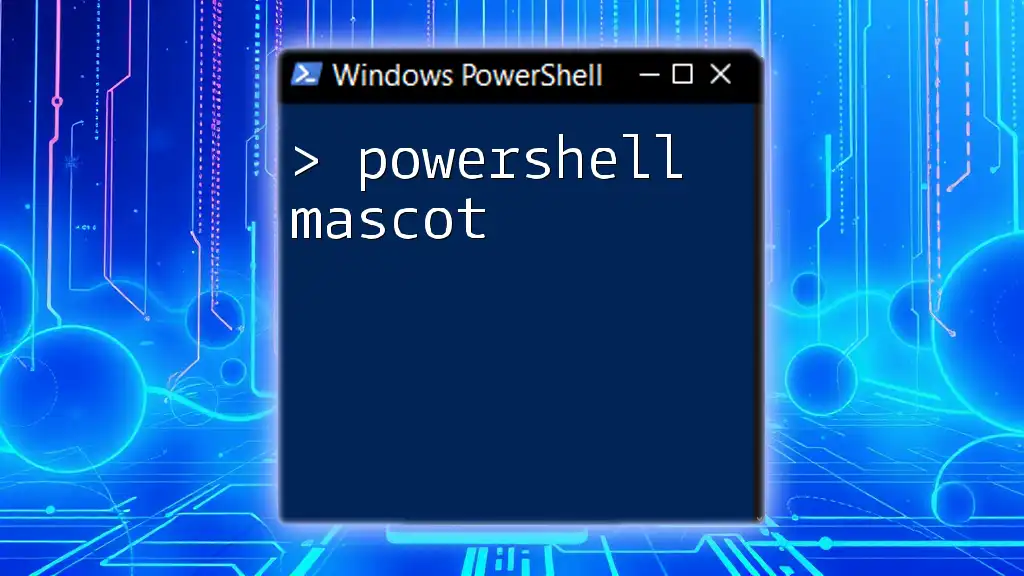
Conclusion
In conclusion, the `-imatch` operator is an invaluable tool in the PowerShell arsenal, providing flexibility for case-insensitive string comparison and powerful regular expression capabilities. By understanding its use cases, potential pitfalls, and integrating it into your daily tasks, you can enhance your scripting efficiency and accuracy. Remember to practice regularly to adapt and make the most of this feature.
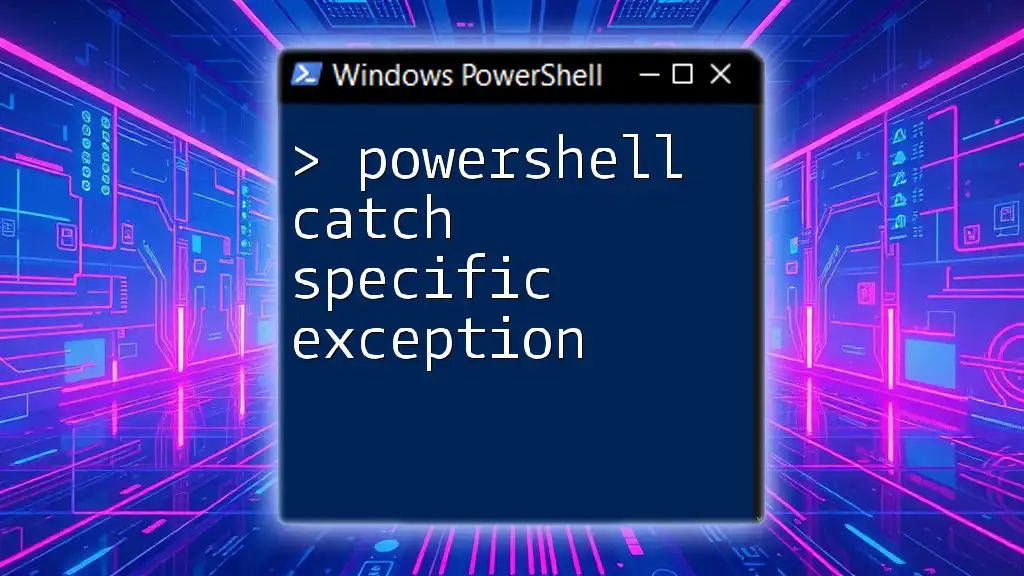
Additional Resources
For further exploration, check the official PowerShell documentation for insights into regex patterns and best practices. Continuous learning through books or online courses can also enhance your skillset, making you proficient in using PowerShell, particularly with `-imatch`.
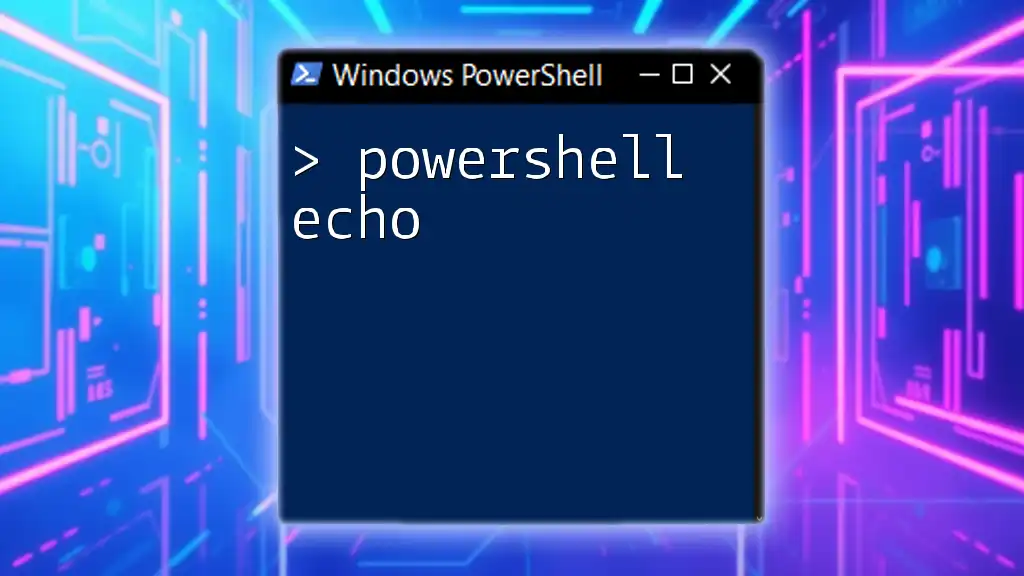
Call to Action
We encourage you to share your experiences with `-imatch` and how it has improved your PowerShell scripting. Join the conversation in the comments, and don't forget to subscribe to our newsletter for more insightful PowerShell tips and tricks!