In PowerShell, you can catch specific exceptions using a `try` block followed by a `catch` block that specifies the exception type you want to handle.
try {
# Code that may throw an exception
Get-Content "nonexistentfile.txt"
} catch [System.IO.FileNotFoundException] {
Write-Host "The file was not found!"
}
Understanding Exceptions in PowerShell
What is an Exception?
An exception is an unexpected event that occurs during the execution of a program, altering its normal flow. Exceptions can arise due to various reasons, such as coding errors, invalid user input, or issues specific to the environment. It's essential to differentiate between exceptions and errors: while errors indicate a serious problem that prevents the program from running, exceptions can be anticipated and caught.
Types of Exceptions
In PowerShell, exceptions can be broadly categorized as system exceptions and application exceptions. System exceptions are built into the .NET Framework and include classes like `System.IO.IOException` (related to input/output issues) and `System.NullReferenceException` (when trying to access an object reference that is null). Application exceptions represent issues unique to specific applications and may be defined by the developer.
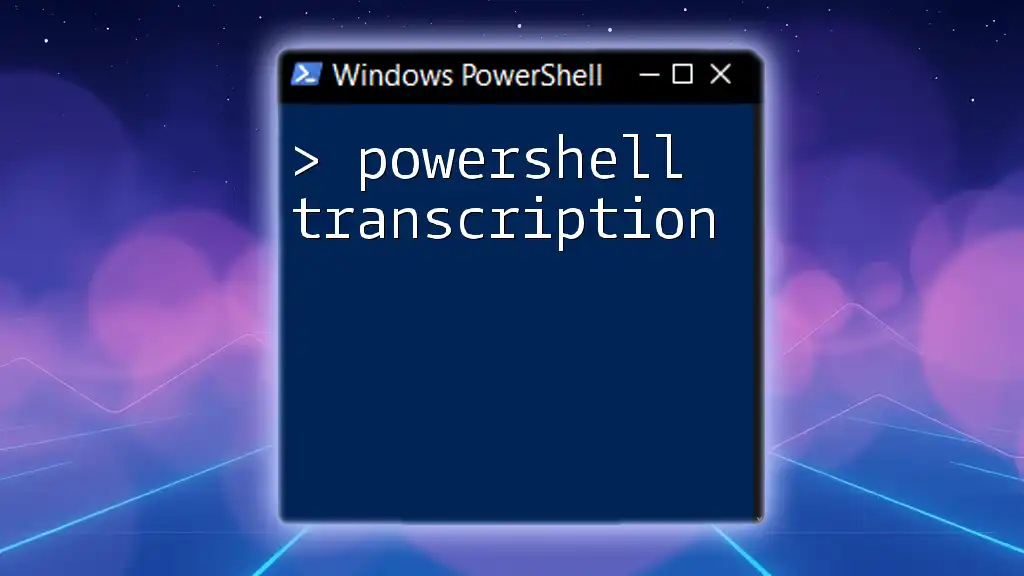
The Try-Catch Block
Basic Syntax of Try-Catch
PowerShell provides a structured way to handle exceptions using the `try-catch` block. The basic syntax involves encasing code that may throw an exception in a `try` block and defining how to handle it in a `catch` block. Here’s a simple example:
try {
# Code that may throw an exception
Get-Item "C:\NonExistentFile.txt"
} catch {
# Code to handle the error
Write-Host "An error occurred: $_"
}
This snippet attempts to access a non-existent file and will catch the resulting exception, allowing the script to manage the error gracefully without crashing.
General Structure of Try-Catch
A `try` block can contain any number of statements that you suspect might throw exceptions. If an exception occurs, the control is transferred to the corresponding `catch` block. Additionally, you can use a `finally` clause, which will always execute regardless of whether an exception occurred, making it ideal for cleanup operations.
try {
# Code that may throw an exception
} catch {
# Code to handle the error
} finally {
# Code that will always execute
Write-Host "Cleanup tasks"
}
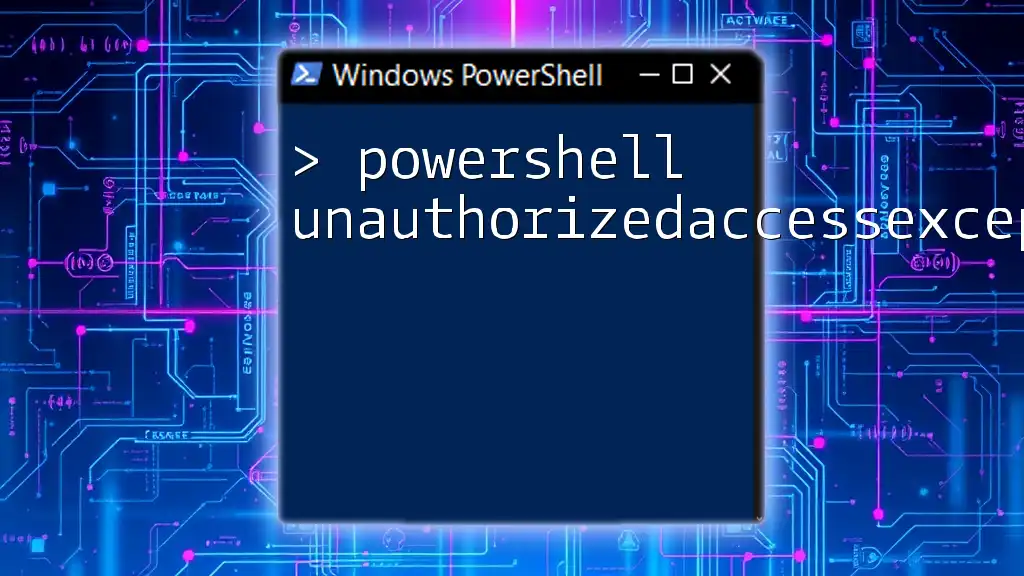
Why Catch Specific Exceptions?
Importance of Specificity
Catching specific exceptions rather than generic ones greatly enhances the robustness of your scripts. When you catch a specific exception, you tailor your error handling to the nature of the error, which can lead to more meaningful error messages and better debugging experiences. For example, if you are working with file operations, catching a `System.IO.IOException` allows you to provide precise feedback related to file access issues.
Performance Considerations
Using specific error handling can also lead to performance benefits, as it allows your script to avoid unnecessary work by addressing issues directly. Instead of funneling all exceptions through a single handler, targeted catches enable your code to efficiently direct the flow and resources where they are genuinely needed.
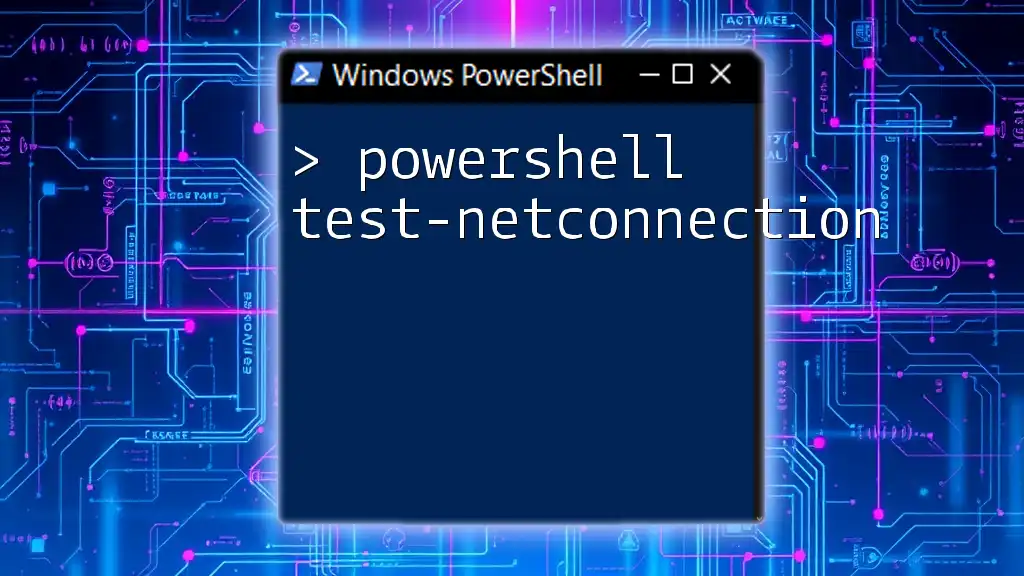
Implementing Specific Exception Handling
The Syntax for Catching Specific Exceptions
To catch a specific type of exception in PowerShell, you can specify the exception type in your `catch` block. This approach not only provides clarity but also enhances your control over the error-handling process. Here’s how it looks:
try {
# Code that may throw a specific exception
Get-Item "C:\NonExistentFile.txt"
} catch [System.IO.IOException] {
Write-Host "Caught an IO Exception!"
} catch [System.Exception] {
Write-Host "Caught a general exception!"
}
This example catches an `IOException` specifically, allowing you to handle file-related issues distinctly from other types of exceptions.
Using Multiple Catch Blocks
PowerShell enables you to define multiple `catch` blocks, allowing you to handle various exceptions in unique ways. Here’s an example:
try {
# Code that could throw different exceptions
Get-Content "C:\NonExistentFile.txt"
} catch [System.IO.IOException] {
Write-Host "Caught an IO Exception!"
} catch [System.UnauthorizedAccessException] {
Write-Host "Caught an Unauthorized Access Exception!"
} catch {
Write-Host "Caught a general exception!"
}
In this code, different exceptions are processed independently based on their types, enabling more nuanced error handling.
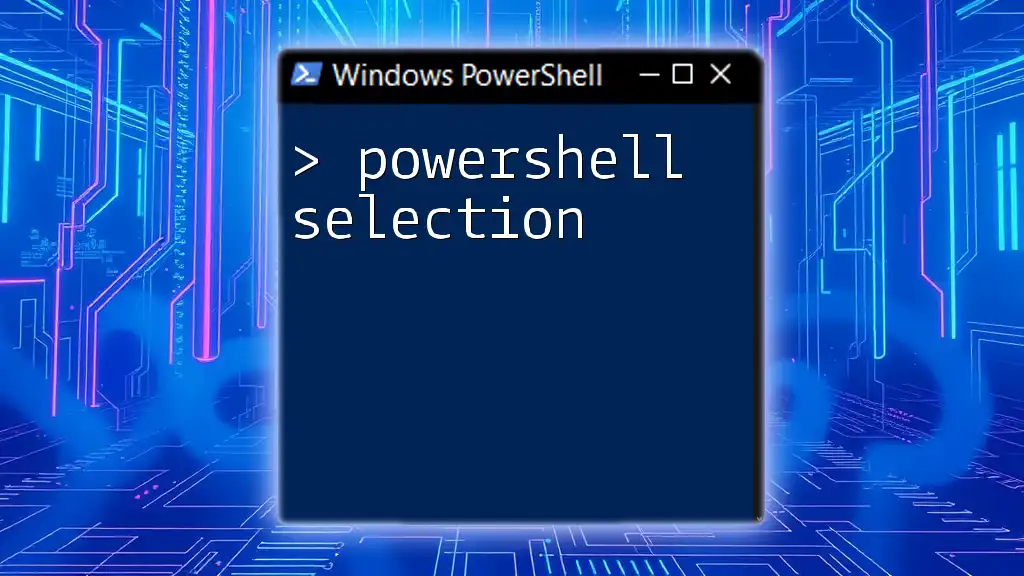
Best Practices for Catching Exceptions
Define and Document Your Error Handling Logic
A well-structured error-handling logic is crucial for maintaining clean and understandable code. Documenting your strategy allows you and your colleagues to comprehend the rationale behind your exception handling choices. Always ensure that error messages are clear and helpful for debugging.
Avoiding Overly Broad Catch Blocks
While a generic `catch` block can catch any exception, over-reliance on it can lead to unmanageable code. For instance, using only a general exception catch could mask underlying issues, making it difficult to trace the source of problems. Consider this:
try {
# Risky operation
} catch {
Write-Host "An error occurred"
}
This approach is ineffective if you aim to identify specific issues because it does not provide useful information on the nature of the error.
Logging Exceptions
Logging exceptions is another best practice, allowing you to maintain a record of errors encountered during script execution. A simple way to log exceptions is by redirecting error information to a file:
try {
# Code that may throw an exception
Get-Content "C:\NonExistentFile.txt"
} catch [System.IO.IOException] {
Add-Content "error_log.txt" "IO Exception occurred on $(Get-Date): $_"
}
This way, you can review the logs later to analyze recurring issues or potentially fix bugs.
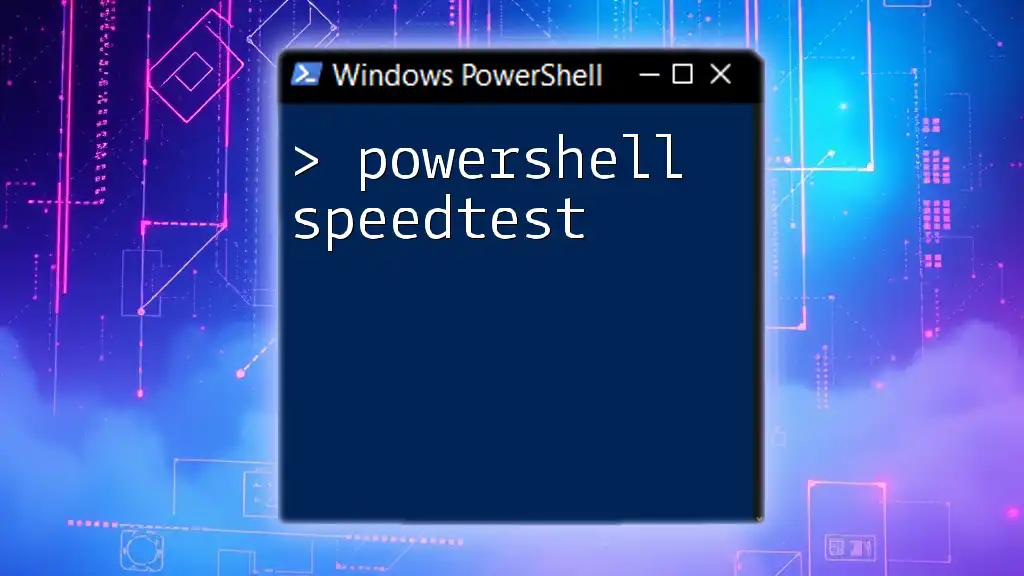
Testing Exception Handling
Unit Testing for Exception Handling
Unit testing is an effective way to ensure that your exception-handling logic works as intended. By utilizing Pester, a popular testing framework for PowerShell, you can create tests to validate that specific exceptions are properly caught.
Practical Testing Scenarios
Consider an example where you wish to verify that a specific exception is thrown and caught:
Describe "File Operations" {
It "Should throw IO Exception for nonexistent file" {
{ Get-Content "C:\NonExistentFile.txt" } | Should -Throw [System.IO.IOException]
}
}
In this unit test, we are asserting that trying to read a non-existent file should throw an `IOException`.
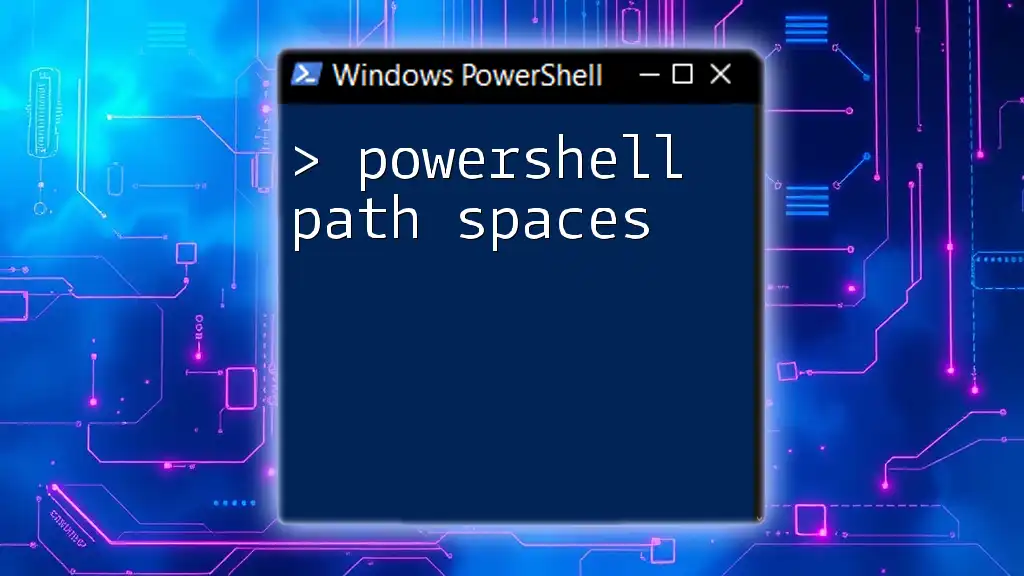
Conclusion
Catching specific exceptions in PowerShell is a powerful tool that enhances your scripts' reliability, maintainability, and performance. By structuring your error handling thoughtfully, you can improve the clarity of your code and streamline troubleshooting. Through rigorous documentation and testing, you’ll bolster your PowerShell skills and be better prepared to tackle real-world challenges in scripting. Whether you are a beginner or an experienced developer, mastering exception handling will significantly elevate the quality of your PowerShell scripts.
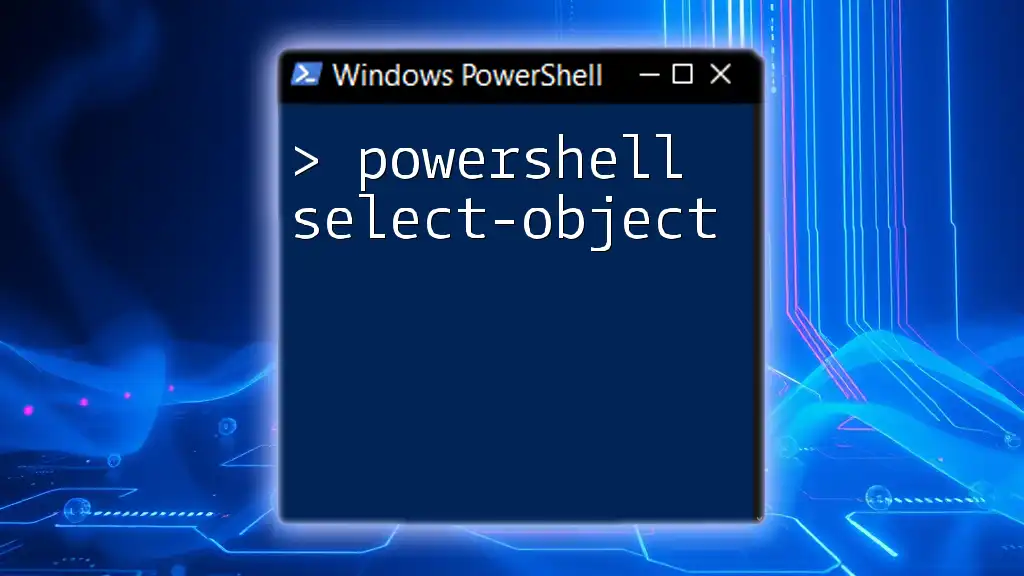
Additional Resources
Recommended Reading
To deepen your understanding of exception handling in PowerShell, consider exploring the official PowerShell documentation, online courses, and community forums that discuss best practices and advanced techniques.
Community and Support
Engaging with the PowerShell community can provide great insights and support. Online forums such as PowerShell.org and Reddit offer valuable resources and discussions for PowerShell users at all levels.