The `UnauthorizedAccessException` in PowerShell occurs when a script or command attempts to access a resource (like a file or directory) without the necessary permissions, leading to an error.
Here's a code snippet that demonstrates catching this exception:
try {
Get-Content 'C:\ProtectedFolder\secret.txt'
} catch [System.UnauthorizedAccessException] {
Write-Host 'Access denied. You do not have permission to view this file.'
}
Common Scenarios for UnauthorizedAccessException
PowerShell `UnauthorizedAccessException` typically arises during operations that require specific permissions. Understanding these scenarios is crucial for effective scripting.
File System Operations
One of the most prevalent causes of `UnauthorizedAccessException` occurs during file system access. For example, attempting to read or modify a file that is protected or requires elevated privileges can trigger this exception.
Registry Access
Accessing or modifying the Windows Registry can lead to similar issues, especially when trying to read keys that require administrative permissions. For instance, if a PowerShell user attempts to modify a system-level registry key without appropriate rights, they will encounter an `UnauthorizedAccessException`.
Network Operations
When accessing network resources (like shared folders or files), permission issues can arise. Trying to read a file in a network share without adequate permissions will also result in this exception.
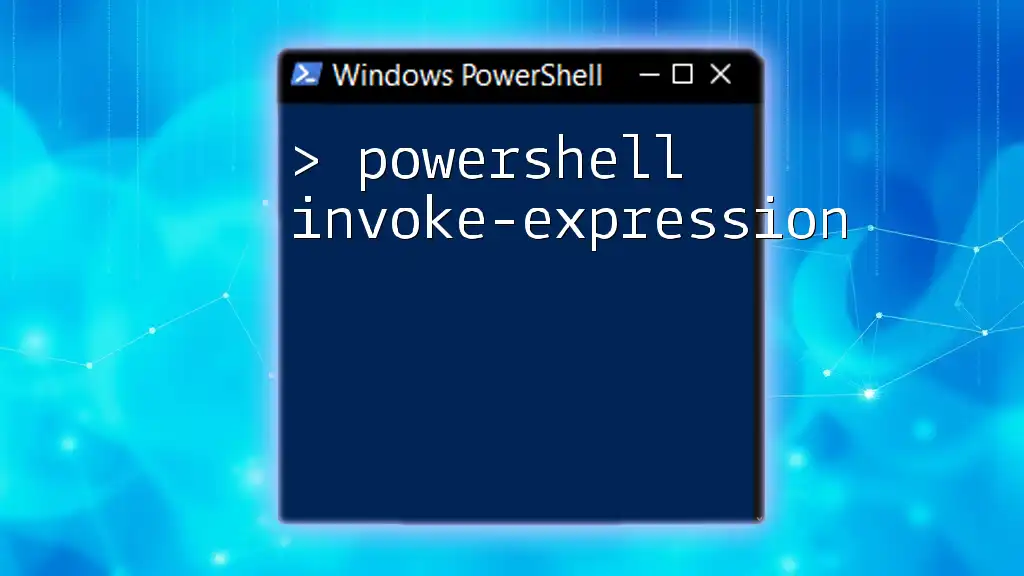
Understanding the Exception's Structure
Description of the Exception
The `UnauthorizedAccessException` is a specific type of exception in .NET that signals that an attempt to access a resource was denied, typically due to insufficient permissions. Common properties of this exception include:
- Message: Describes the issue in detail.
- Source: Indicates where the exception occurred.
- StackTrace: Provides context for where in the code the error happened.
Example of an UnauthorizedAccessException
Consider the following code snippet, where an attempt is made to retrieve the content of a protected file:
Get-Content "C:\ProtectedFile.txt"
If you do not have permission to access the file, the result will be an `UnauthorizedAccessException`, informing you that access is denied.
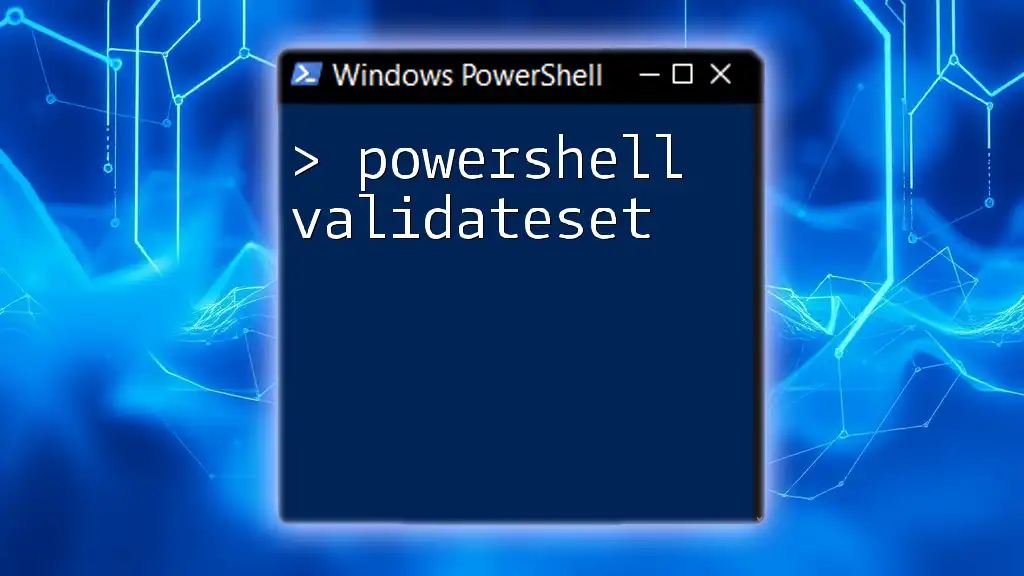
Handling UnauthorizedAccessException in PowerShell
Using Try-Catch to Manage Exceptions
Using a `try-catch` block is an effective way to manage exceptions in PowerShell. It allows you to catch specific exceptions and handle them gracefully. For example:
try {
Get-Content "C:\ProtectedFile.txt"
}
catch [System.UnauthorizedAccessException] {
Write-Host "Access Denied: You do not have permission to access this file."
}
In this case, if the access is denied, the script will print a friendly message instead of terminating abruptly.
Logging and Debugging Exception Handling
Effective logging helps in understanding what went wrong during script execution. This step is particularly useful in larger scripts where tracking multiple exceptions becomes necessary. You might implement a simple logging mechanism like this:
catch {
$errorDetails = $_.Exception.Message
Add-Content -Path "C:\Logs\error.log" -Value $errorDetails
}
In this example, any errors encountered will be logged to a specified file, aiding in future debugging efforts.
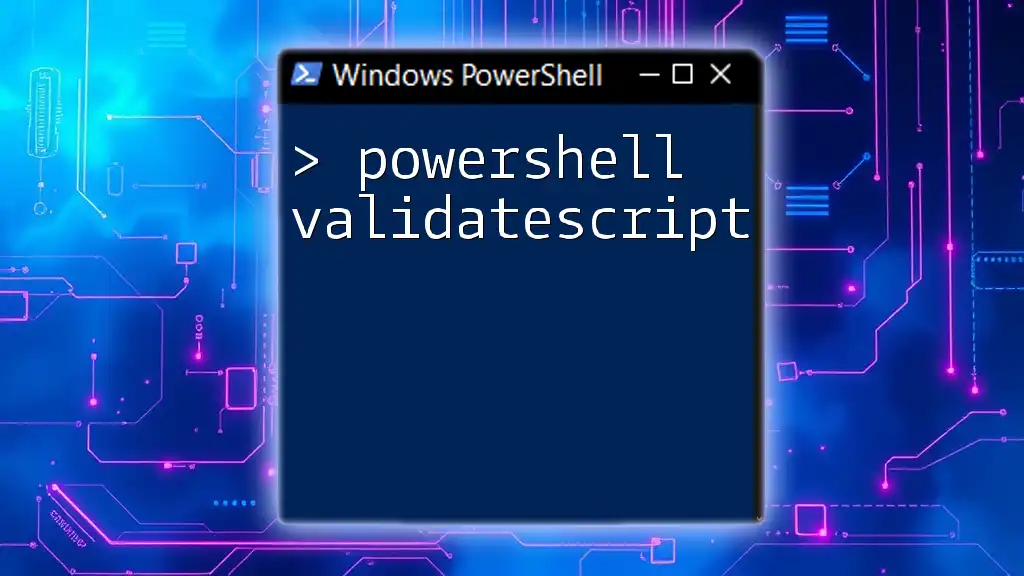
Best Practices to Avoid UnauthorizedAccessException
Check Permissions Before Performing Operations
One of the simplest ways to prevent encountering `UnauthorizedAccessException` is to check if you have permissions before trying to perform file operations. You can use the following example to verify if a file is accessible:
if (Test-Path "C:\ProtectedFile.txt" -PathType Leaf) {
# Perform actions
} else {
Write-Host "File does not exist or is not accessible."
}
In this snippet, the script checks the existence and accessibility of the file before attempting to access its content.
Use the -ErrorAction Parameter
Employing the `-ErrorAction` parameter can help manage errors more effectively within your commands. This parameter allows you to specify how the command should respond to a non-terminating error. You can use it like this:
Get-Content "C:\ProtectedFile.txt" -ErrorAction Stop
By using `-ErrorAction Stop`, you ensure that the script will halt upon encountering an unauthorized access issue, allowing for immediate error handling.
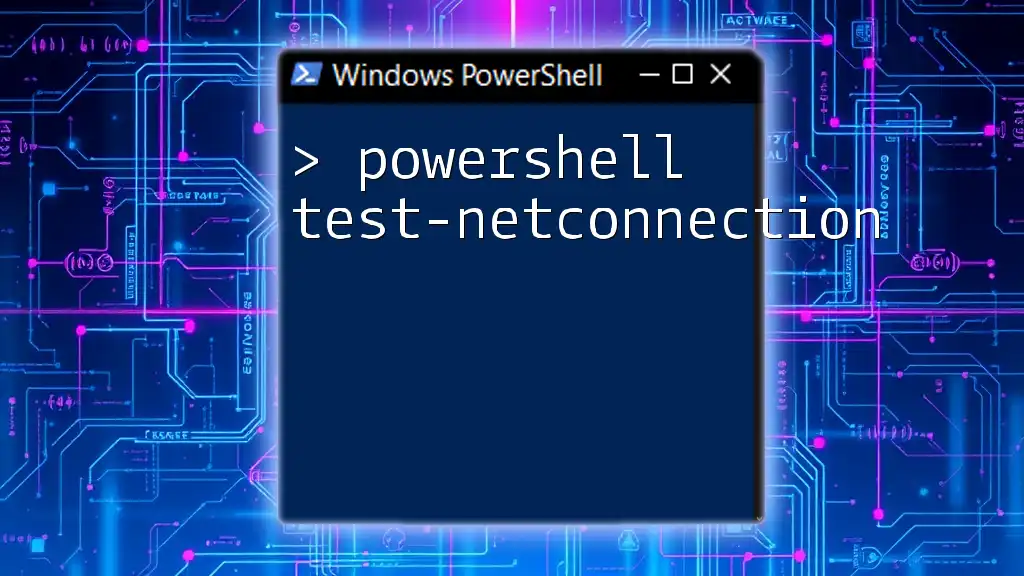
Advanced Techniques for Managing UnauthorizedAccessException
Custom Error Messages
Crafting user-friendly error messages can enhance the user experience, providing clearer insight into what went wrong. Modify your catch block to include a custom message that adds context:
catch {
Write-Host "An error occurred: $($_.Exception.Message). Please check your permissions."
}
This approach helps the user understand the nature of the problem and what steps to take next.
Creating a Function to Handle Exceptions
Creating reusable functions is another advanced technique. This method maintains the cleanliness of your scripts while providing robust exception handling. Here’s an example:
function Get-FileContentSafely {
param (
[string]$filePath
)
try {
Get-Content $filePath
}
catch [System.UnauthorizedAccessException] {
Write-Host "Access Denied. Check your permissions."
}
}
This function not only attempts to retrieve file content but also handles access issues gracefully within its structure.
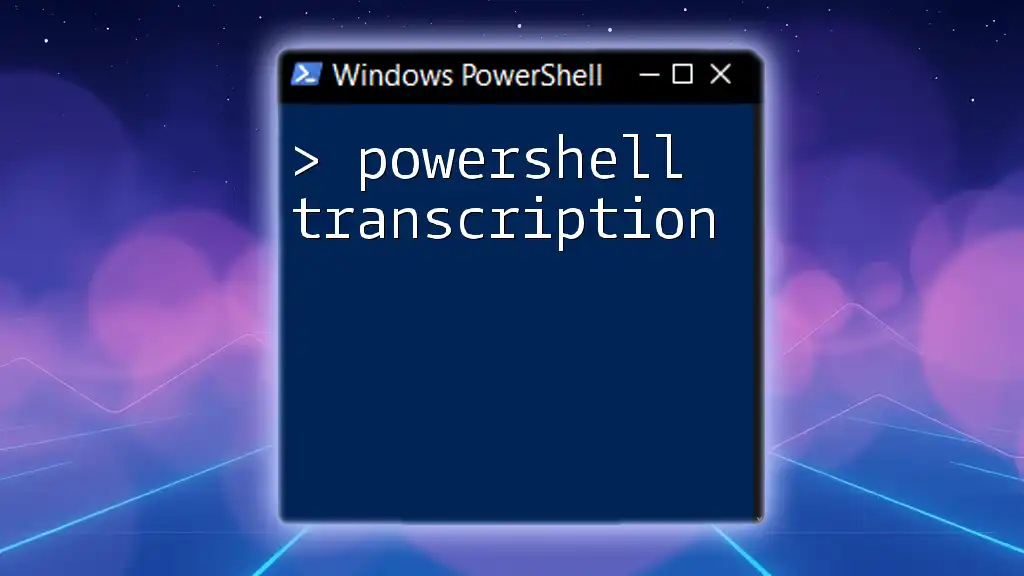
Conclusion
Understanding and managing the PowerShell UnauthorizedAccessException is vital for any script developer or system administrator. By recognizing common scenarios where this exception occurs, implementing effective error handling techniques, and adopting best practices, you can enhance your PowerShell scripting efficiency and minimize disruptions caused by permissions-related issues. Apply the knowledge and techniques from this guide to streamline your scripting process and ensure smoother interactions with system resources.