The `ForEach-Object` cmdlet in PowerShell allows you to perform an operation on each item in a collection in a concise manner. Here's a simple code snippet demonstrating its use:
1..5 | ForEach-Object { Write-Host "Number: $_" }
Basics of ForEach-Object
PowerShell's ForEach-Object command, often abbreviated as `ForEach`, is one of the most versatile and essential constructs in PowerShell scripting. Understanding its syntax is crucial for effective automation. The general syntax for the `ForEach` construct is as follows:
ForEach ($item in $collection) {
# Process each item
}
In this syntax:
- `$item` represents the current element being processed.
- `$collection` is a collection of items, such as an array or a list.
When using ForEach-Object, the core syntax looks slightly different and is often used in a pipeline context. The primary difference is that it operates on objects passed through the pipeline.
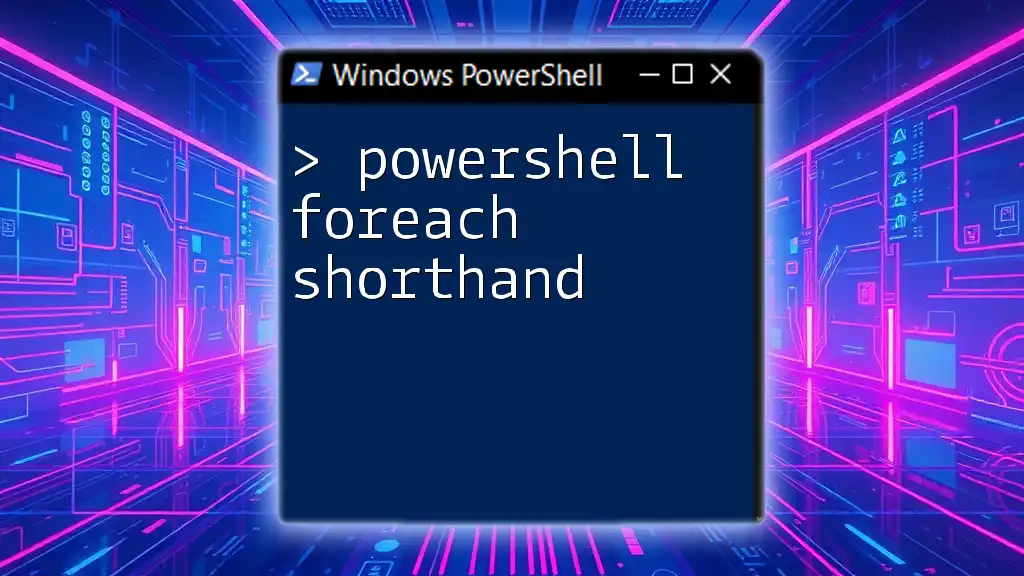
ForEach vs. ForEach-Object
It's essential to differentiate between the classic `ForEach` loop and the `ForEach-Object` cmdlet, as their usage scenarios may vary.
Differences Between ForEach and ForEach-Object
-
ForEach Loop: Utilized primarily for iterating through collections explicitly declared in your script. For example:
$fruits = "Apple", "Banana", "Cherry" ForEach ($fruit in $fruits) { Write-Host "Fruit: $fruit" }
-
ForEach-Object Cmdlet: Best utilized in conjunction with a pipeline. For example:
$fruits = "Apple", "Banana", "Cherry" $fruits | ForEach-Object { Write-Host "Fruit: $_" }
Here, `$_` is a placeholder representing the current object in the pipeline.
Performance Considerations
While both methods achieve similar outcomes, using ForEach-Object in pipelines can often yield better performance, especially with larger datasets or when processing multiple cmdlets in succession. Understanding when to leverage one over the other can make a significant difference in execution time and script efficiency.
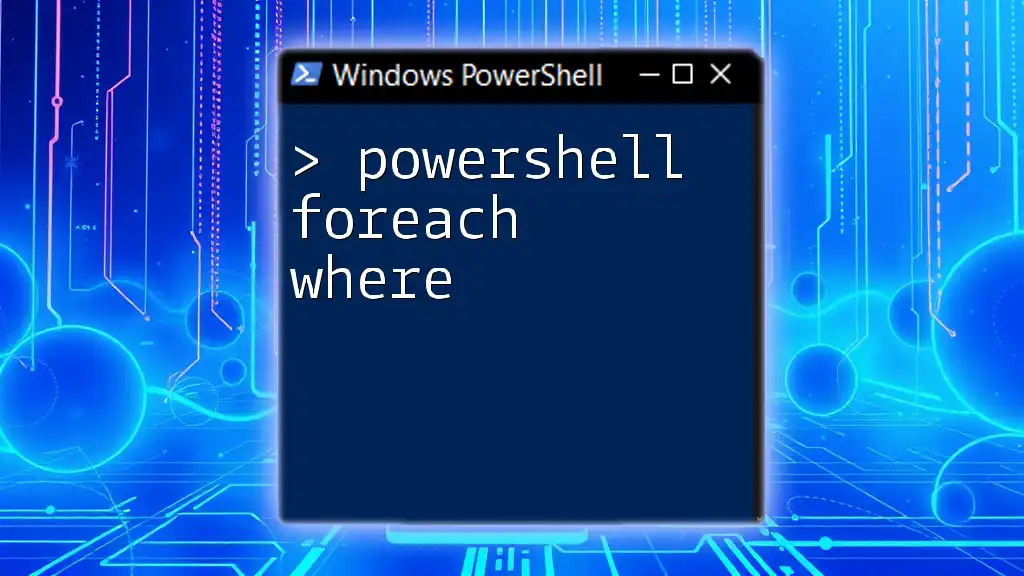
Practical Applications of ForEach-Object
Understanding practical applications can solidify your grasp of `ForEach-Object`. Here are some typical scenarios:
Looping Through Arrays
One common use case is iterating through arrays. For example, if you have an array of fruits, you might want to print each one:
$fruits = "Apple", "Banana", "Cherry"
$fruits | ForEach-Object {
Write-Host "Fruit: $_"
}
This example shows how easily you can output each fruit name simply by piping the array through ForEach-Object.
Looping Through Objects
You can also use ForEach-Object to process collections of custom objects. Consider a scenario where you have user information stored as objects:
$users = @(
[PSCustomObject]@{ Name="John"; Age=30 },
[PSCustomObject]@{ Name="Jane"; Age=25 }
)
$users | ForEach-Object {
Write-Host "$($_.Name) is $($_.Age) years old."
}
This method allows you to access properties such as `Name` and `Age` iteratively, making it an effective way to process structured data.
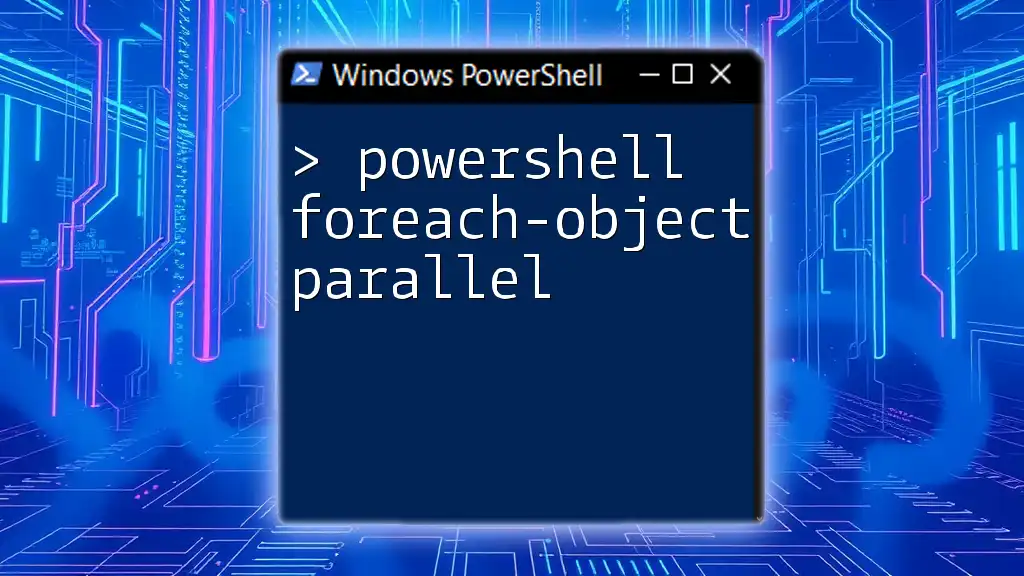
Error Handling in ForEach-Object
Error handling is a vital component of any robust script. With `ForEach-Object`, you can incorporate Try-Catch blocks for better error management.
Using Try-Catch Blocks
Handling potential errors is streamlined with the usage of Try-Catch blocks. Here's how you could implement this in a numeric processing scenario:
$numbers = 1..5
$numbers | ForEach-Object {
Try {
if ($_ -eq 3) { throw "Error at 3" }
Write-Host "Processing $_"
}
Catch {
Write-Host "Caught an error: $_"
}
}
In this example, when the number `3` is encountered, the script throws an error which is then caught and displayed, allowing for graceful error handling without breaking execution flow.
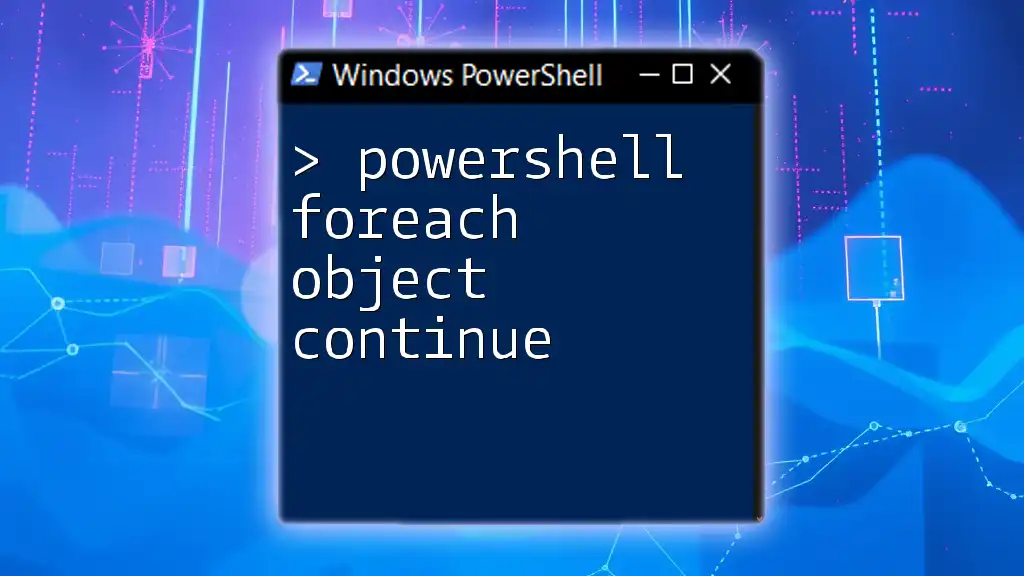
Working with Pipelines
One of the strengths of ForEach-Object lies in its ability to seamlessly integrate with other cmdlets via the pipeline.
Integration with Other Cmdlets
For example, consider you want to list all running processes and their memory usage. You can achieve this efficiently with ForEach-Object:
Get-Process | ForEach-Object {
Write-Host "$($_.Name) is using $($_.WorkingSet / 1MB) MB"
}
This demonstrates how you can easily process each object returned by the `Get-Process` cmdlet, providing meaningful output about memory usage.
Chaining Cmdlets
The flexibility of piping allows for chaining multiple cmdlets in succession. Leveraging this can create powerful one-liners for complex tasks. For example, if you want to find text files in a directory and output their names:
Get-ChildItem | Where-Object { $_.Extension -eq ".txt" } | ForEach-Object {
Write-Host "Text file found: $($_.Name)"
}
This chain filters the files based on an extension and then uses `ForEach-Object` to act on the results.
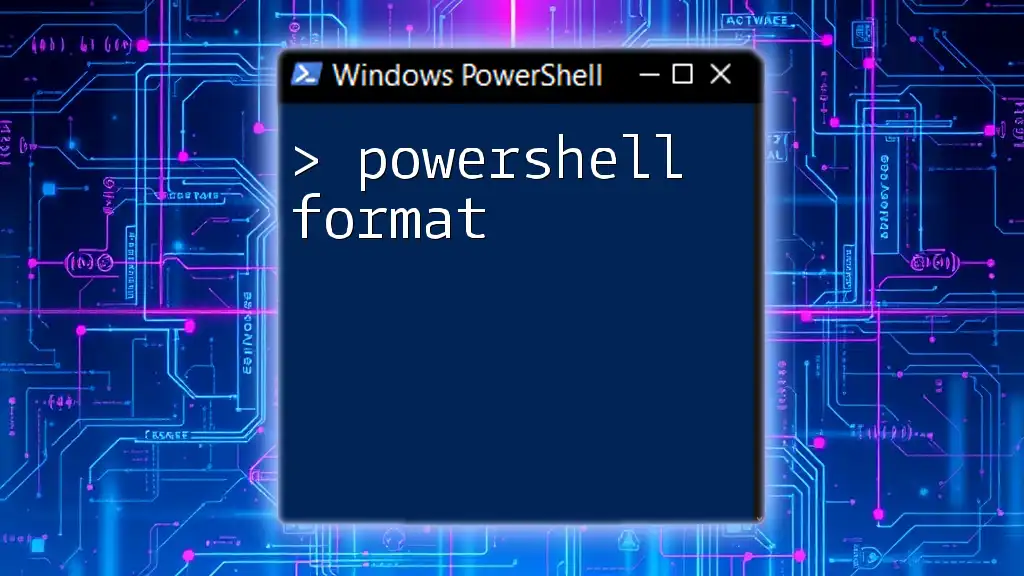
Advanced Techniques
Once you've mastered the basics, you might wish to explore advanced techniques involving ForEach-Object.
Using ForEach-Object with Parameters
You can pass parameters into the ForEach-Object script block, which allows for flexible script designs. For instance, if you want to specify a search pattern for files:
$files = Get-ChildItem
$pattern = '*.txt'
$files | ForEach-Object -Process {
if ($_.Name -like $pattern) {
Write-Host "Text file found: $_"
}
}
In this example, files are filtered by the specified pattern, demonstrating the power of parameterization in your scripts.
Choosing Between ForEach and ForEach-Object for Complex Tasks
For complex tasks involving multiple iterations and internal logic, you may choose between `ForEach` and `ForEach-Object`. Generally, ForEach is better for smaller collections and when you have already defined the data, while ForEach-Object shines in contexts requiring data from piped input.
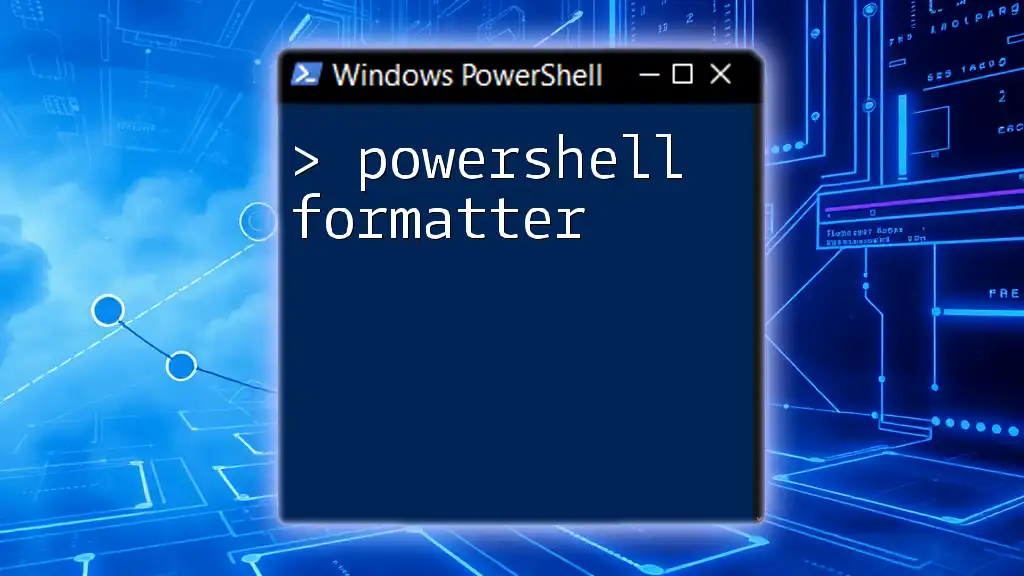
Common Pitfalls
Finally, while ForEach-Object is a powerful tool, there are some common pitfalls users often encounter.
Common Mistakes with ForEach-Object
A frequent mistake is neglecting to use the `$_` variable correctly. This can lead to confusion or errors, especially when trying to access object properties.
Performance Issues to Avoid
To optimize script performance, avoid nesting multiple `ForEach-Object` cmdlets unnecessarily. Each iteration incurs overhead, which can slow down performance.
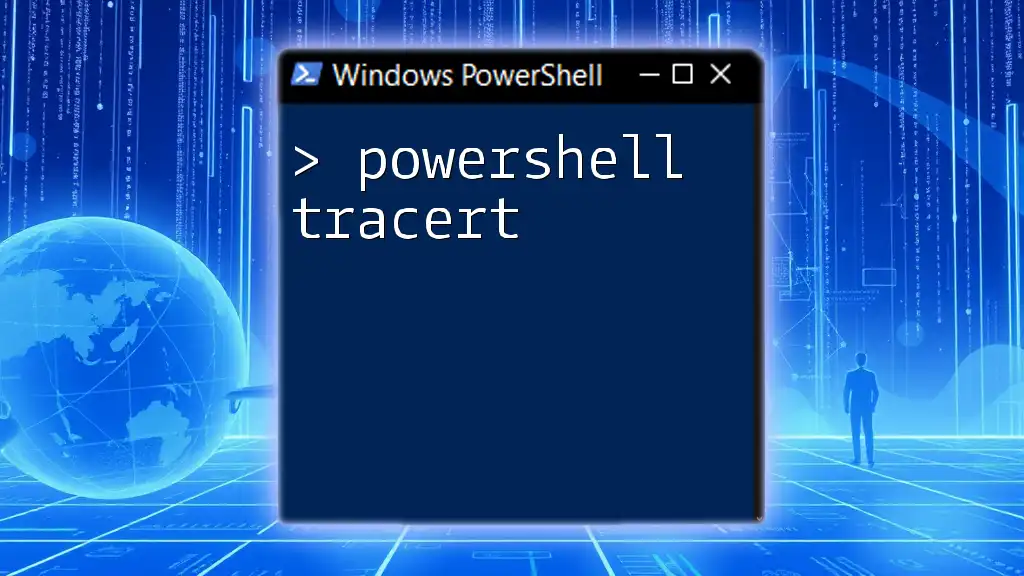
Conclusion
Mastering ForEach-Object is pivotal for anyone looking to enhance their PowerShell scripting skills. It unlocks powerful capabilities for data processing and automation, making your scripts more efficient and effective. Whether you're working with arrays, objects, or pipelines, understanding the nuances of `ForEach-Object` can greatly improve your automation tasks.
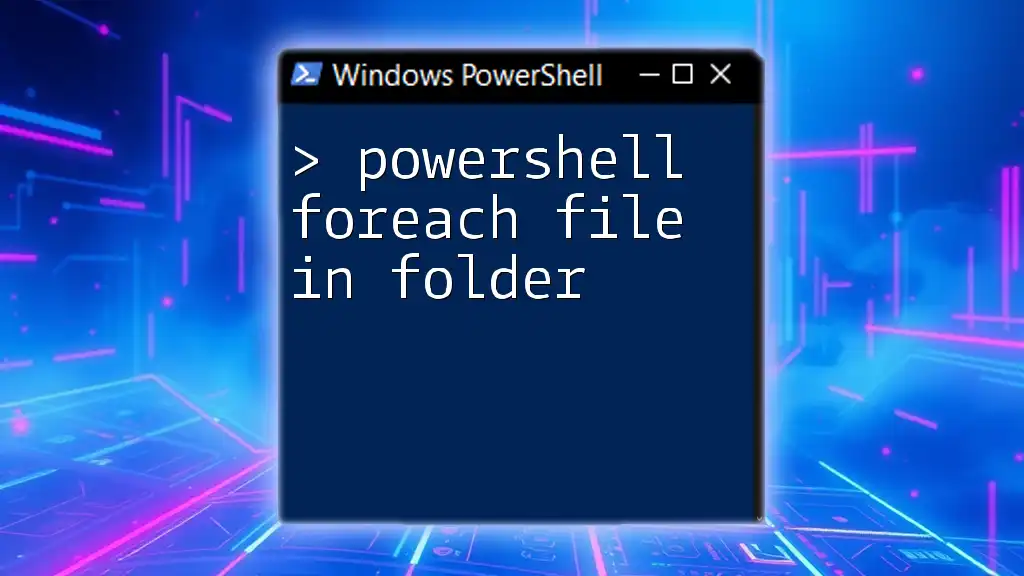
Additional Resources
For those eager to deepen their knowledge, the official PowerShell documentation is an excellent starting point. Exploring tutorials and blogs dedicated to PowerShell scripting will further enhance your learning experience.
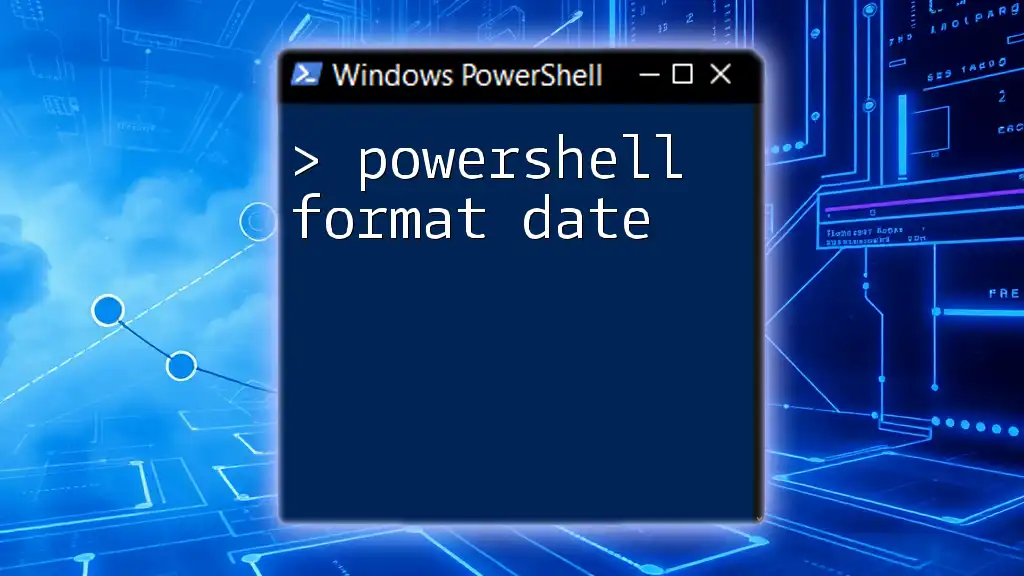
Call to Action
Join our community to stay updated and embark on your journey towards mastering PowerShell!