In PowerShell, you can use the `ForEach` shorthand with the `%` symbol to iterate over a collection of items quickly and concisely.
Here’s a simple example in PowerShell:
1..5 | % { Write-Host "Number: $_" }
Understanding ForEach Loop in PowerShell
What is a ForEach Loop?
The ForEach loop in PowerShell allows you to iterate over a collection of items. This is particularly useful in scenarios where you need to process, manipulate, or display each item in a dataset. Common use cases include working with arrays, lists, and even the output from other cmdlets.
Syntax of ForEach Loop
The syntax for a traditional ForEach loop is straightforward. Here’s a basic example demonstrating its structure:
$items = @(1, 2, 3, 4, 5)
ForEach ($item in $items) {
Write-Host "Item: $item"
}
In this loop, `$items` is an array, and for each element in the array, the code inside the curly braces executes, allowing you to work with each `$item` individually.

The ForEach-Object Cmdlet
Introduction to ForEach-Object
The ForEach-Object cmdlet serves a similar purpose but works differently. It is particularly powerful in pipeline scenarios, allowing you to process each item as it flows through the pipeline, often leading to more concise code.
Syntax and Basic Usage
The syntax for ForEach-Object looks like this:
Get-Process | ForEach-Object { $_.Name }
In this example, the `Get-Process` cmdlet retrieves a list of running processes, which are then piped into ForEach-Object. The `$_` variable represents the current object in the pipeline, making it easier to refer to it without needing to specify its name explicitly.
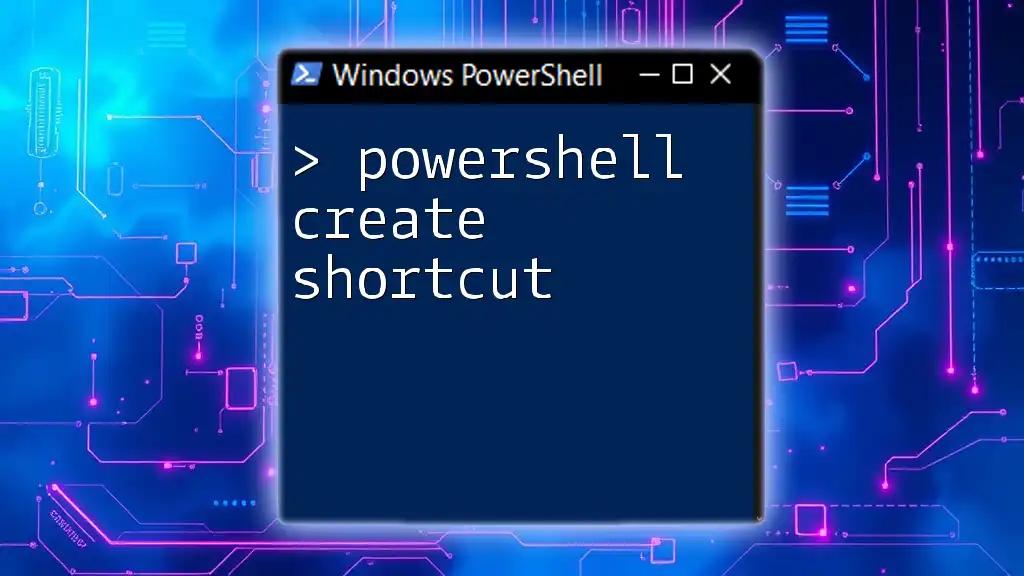
Shorthand Syntax for ForEach: The Magic of ForEach-Object
Introduction to Shorthand Usage
The ForEach-Object cmdlet is remarkably versatile and can be used in a shorthand manner that enhances code readability and efficiency. By utilizing the `$_` automatic variable, you can streamline your code significantly.
Shorthand Example 1: Basic Usage
Here’s a simple example using shorthand to get the names of processes:
Get-Process | ForEach-Object { $_.Name }
This command takes all running processes and returns just their names. The use of `$_` here simplifies reference to the current process, demonstrating how shorthand can enhance both clarity and conciseness.
Shorthand Example 2: Filtering Data
Filtering data with shorthand can also be effectively performed with a combination of Where-Object and ForEach-Object. Here's how to filter out processes that have a CPU usage greater than 100 milliseconds:
Get-Process | Where-Object { $_.CPU -gt 100 } | ForEach-Object { $_.Name }
In this command, the Where-Object cmdlet first filters processes based on CPU usage, and then ForEach-Object outputs only the names of those processes that meet the criteria. This cascaded command structure showcases the power of PowerShell shorthand, reducing complexity without sacrificing functionality.
Shorthand Example 3: Modifying Objects
You can also modify properties of objects in collection using shorthand. Here’s a practical example to stop services:
Get-Service | ForEach-Object { $_.Status = 'Stopped' }
In this code, we retrieve all services and set their status to 'Stopped'. While the example assumes you have the appropriate permissions, it illustrates how easily you can manipulate a collection of objects using shorthand.

Nested ForEach Usage
Understanding Nested Loops
Nested ForEach loops can be a powerful tool for iterating over more complex data structures, like arrays of arrays or collections that require multi-level processing. However, it's essential to be cautious of their complexity and potential performance implications.
Example of Nested ForEach
Here’s a practical example of nested ForEach loops that doubles the values in an array of arrays:
$arrays = @( @(1, 2), @(3, 4) )
$arrays | ForEach-Object { $_ | ForEach-Object { $_ * 2 } }
In this example, each inner array is processed separately, allowing for versatile data manipulation. This nested structure efficiently handles transformations, demonstrating how you can leverage nested loops to manage complex datasets effectively.
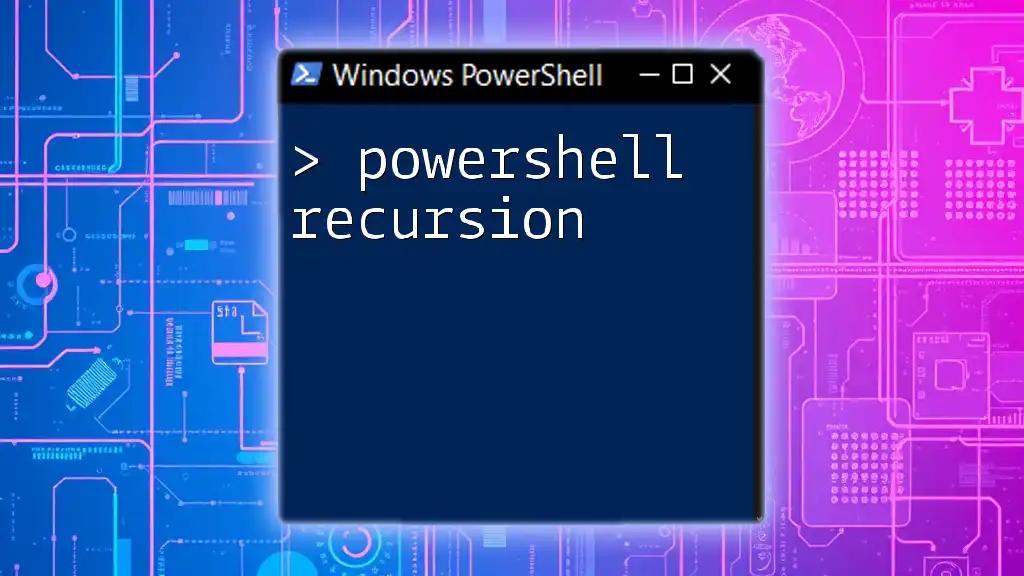
Special Use Cases for ForEach Shorthand
Array Manipulation
ForEach shorthand can be particularly useful for transforming arrays quickly. For instance, if you want to double the values in an array, you can do so succinctly:
$array = @(1, 2, 3, 4, 5)
$array | ForEach-Object { $_ * 2 }
This code returns a new array containing the doubled values, efficiently applying a transformation with minimal syntax.
File Processing
File processing can also be enhanced through ForEach-Object. Consider a scenario where you need to replace text in all .txt files in a directory:
Get-ChildItem *.txt | ForEach-Object { (Get-Content $_) -replace "old", "new" | Set-Content $_ }
Here, each text file is processed to replace the word "old" with "new". The combination of Get-ChildItem, ForEach-Object, and Set-Content showcases how you can build robust file manipulation scripts using shorthand effectively.
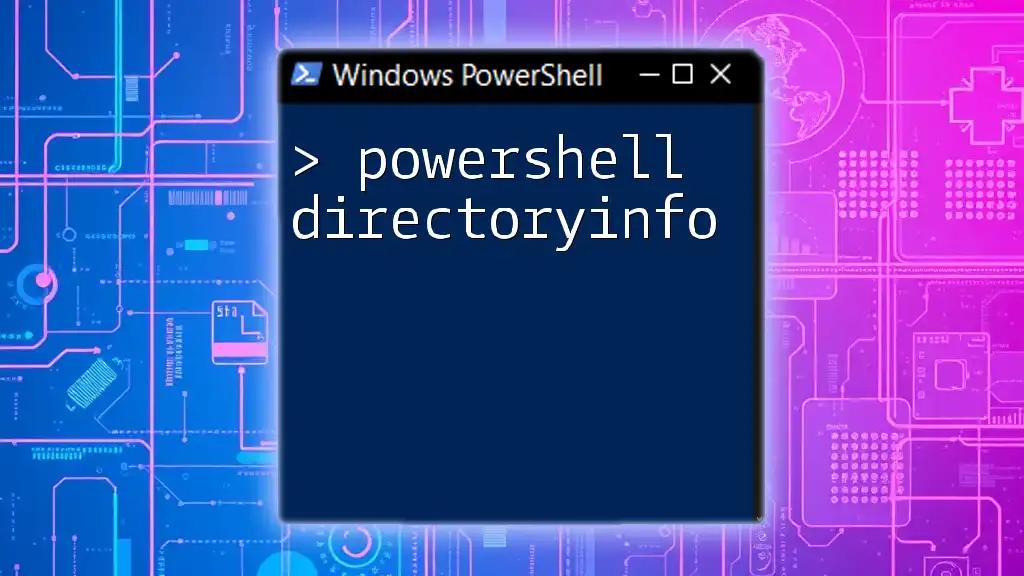
Performance Considerations
Comparing ForEach vs. ForEach-Object
When deciding between ForEach and ForEach-Object, it’s essential to consider performance. The ForEach statement can be more efficient for in-memory collections, while ForEach-Object shines in pipeline scenarios, processing items as they stream in.
Best Practices for Efficient ForEach Usage
To ensure optimal performance when using ForEach, consider the following best practices:
- Use ForEach-Object for pipeline processing.
- Limit the amount of data being processed by filtering early in the pipeline.
- Avoid modifications in place unless necessary, as they may affect the original dataset.

Conclusion
By mastering the shorthand capabilities of PowerShell's ForEach constructs, you significantly enhance your scripting efficiency and effectiveness. With the ability to streamline operations, manipulate data effortlessly, and harness the power of pipelining, PowerShell ForEach shorthand not only makes your code more concise but also elevates your overall productivity in PowerShell scripting.
Embrace these techniques in your daily scripting tasks, and your proficiency with PowerShell will surely grow. As you continue your journey, don't hesitate to explore further resources to deepen your understanding and application of these concepts.