The `ForEach-Object` cmdlet in PowerShell can be combined with the `Where-Object` cmdlet to iterate over a collection and filter elements based on a specified condition. Here's an example:
Get-Process | Where-Object { $_.CPU -gt 100 } | ForEach-Object { Write-Host $_.Name }
Understanding PowerShell Scripting
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administration and automation. Originally developed by Microsoft, it has evolved into a robust tool that allows users to manage and automate the administration of both local and remote Windows systems.
PowerShell integrates command-line scripting with the .NET Framework, enabling users to manipulate objects rather than just plain text. This object-based pipeline facilitates efficient data handling and complex task automation.
The Scripting Basics
Every PowerShell command is referred to as a cmdlet, which combines a verb and a noun, such as `Get-Service` or `Set-Item`. PowerShell scripts consist of commands that can accept parameters and return output in the form of objects. Understanding the structure of a PowerShell command is crucial for writing effective scripts.
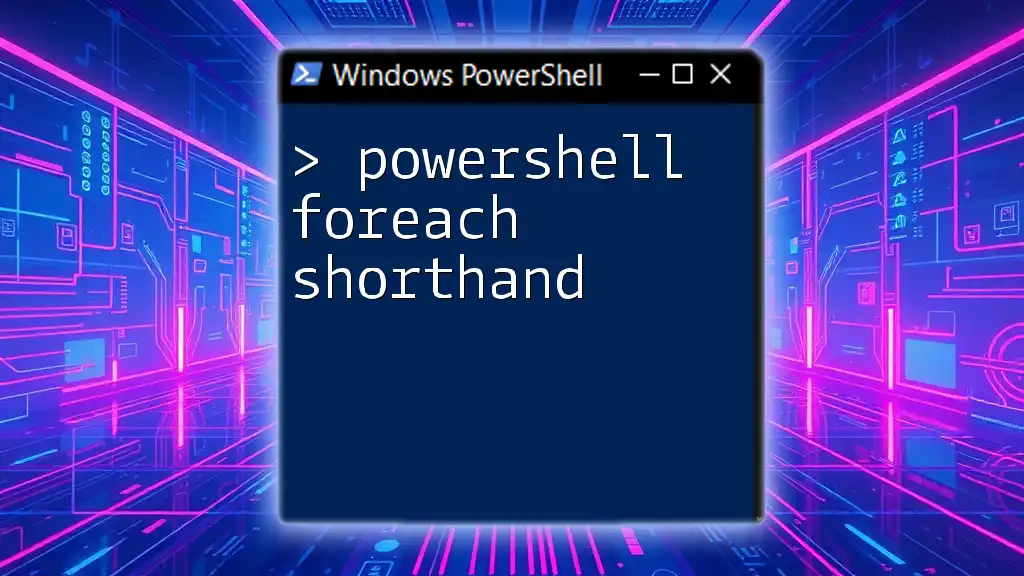
The Foreach Command
What is Foreach?
The `Foreach` command in PowerShell is designed to iterate over a collection of items, allowing users to perform actions on each item within that collection. It enables users to automate repetitive tasks efficiently.
Syntax of the Foreach Command
The basic syntax of the `Foreach` statement is straightforward:
foreach ($item in $collection) {
# code to execute
}
In this structure, `$item` represents the current item in the iteration, and `$collection` is the array or collection being iterated through.
Use Cases for Foreach
The `Foreach` command is versatile and can be used in various contexts:
- Iterating through arrays: When you have a list of items, you can easily perform operations on each item within that list.
- Working with collections of objects: `Foreach` is particularly useful when dealing with objects returned from cmdlets.
For example, to retrieve and display the names of all running services, you can use:
Get-Service | ForEach-Object { $_.DisplayName }
In this snippet, `ForEach-Object` iterates over each service and outputs its display name.
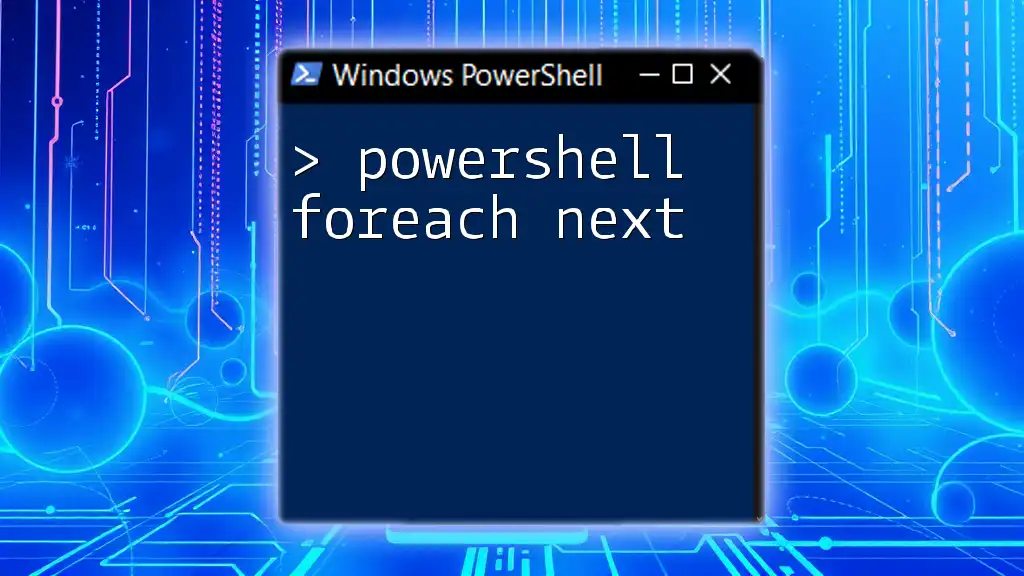
The Where Command
What is Where?
The `Where` command, specifically `Where-Object`, allows users to filter collections based on specified conditions. It enables you to pinpoint exactly what you need from a larger dataset.
Syntax of the Where Command
The syntax for `Where-Object` is as follows:
$collection | Where-Object { condition }
In this syntax, `condition` is a script block that defines the filtering criteria.
Use Cases for Where
`Where-Object` is invaluable when needing to filter out specific items from a dataset:
- Filtering arrays and collections: Easily extract items that meet certain criteria.
A practical example is finding processes that use more than 50 CPU resources:
Get-Process | Where-Object { $_.CPU -gt 50 }
Here, `Where-Object` filters through all processes and returns only those with a CPU usage greater than 50.
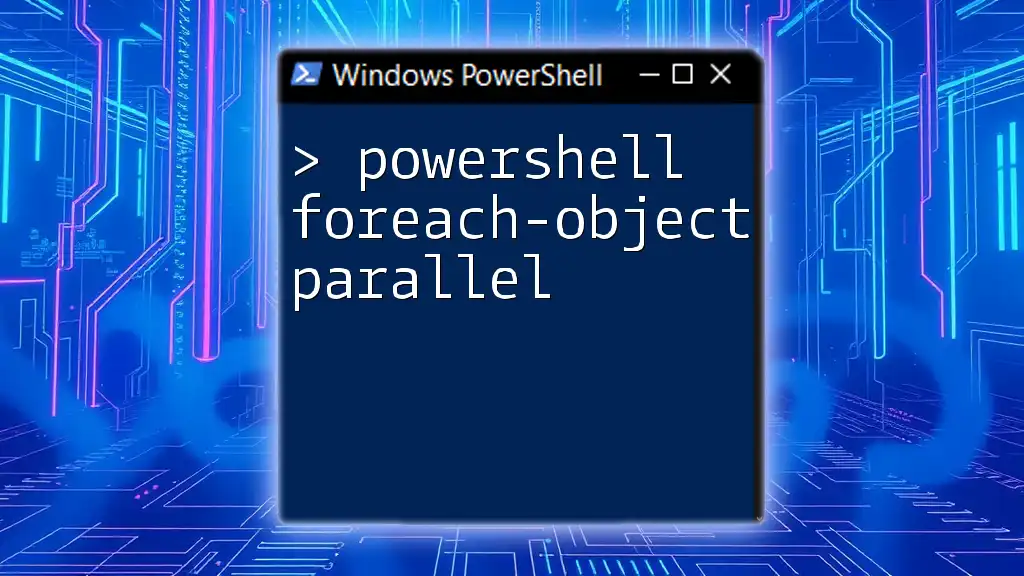
Combining Foreach and Where
The Power of Combining Foreach and Where
Combining `Foreach` and `Where` commands creates powerful scripts that enable both filtering and iterative processing. This synergy allows for more complex data manipulation and efficiency in automation tasks.
Example Situations
Consider this scenario where you want to get the names of all running services:
Get-Service | Where-Object { $_.Status -eq 'Running' } | ForEach-Object { $_.DisplayName }
This command first filters the services to include only those that are currently running, and then it lists their display names.
More Complex Examples
In another example, you may want to extract the usernames of all enabled Active Directory user accounts:
Get-ADUser -Filter * | Where-Object { $_.Enabled -eq $true } | ForEach-Object { $_.SamAccountName }
This combines getting all AD users, filtering only those that are enabled, and then outputting their usernames.
As a practical task, you can clean up files larger than 1MB in a specific directory:
Get-ChildItem -Path C:\Temp | Where-Object { $_.Length -gt 1MB } | ForEach-Object { Remove-Item $_.FullName }
In this case, files in the `C:\Temp` directory that exceed 1MB in size are identified and removed.
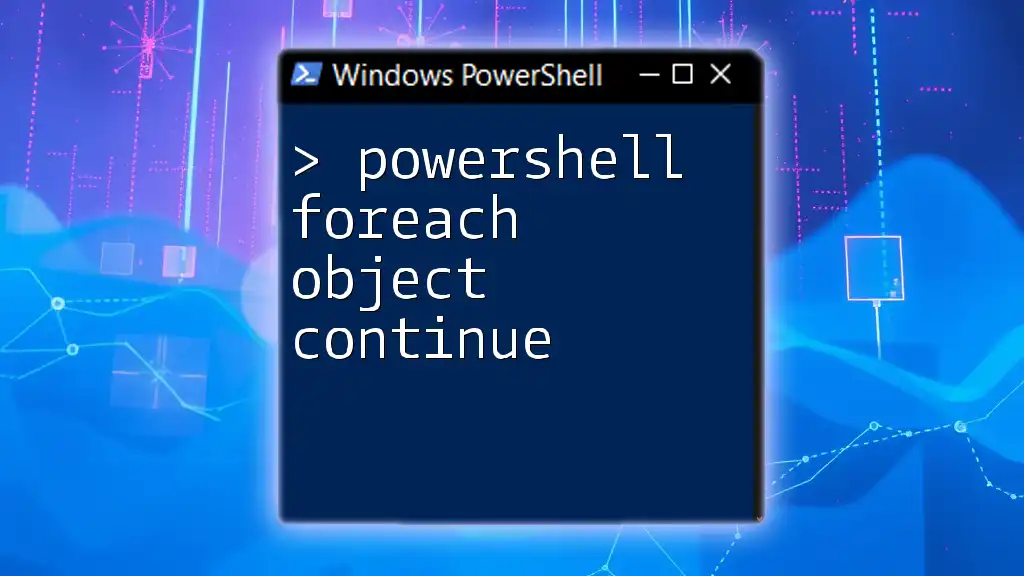
Best Practices
Writing Efficient Scripts
When crafting PowerShell scripts, efficiency is key. Here are a few best practices to keep in mind:
- Minimize usage of pipelines when unnecessary: While pipelines can streamline code, overusing them can slow performance.
- Use the `Select-Object` cmdlet to limit the properties returned, reducing memory overhead.
Readability and Maintainability
Always prioritize readability in your scripts. Utilizing meaningful variable names, incorporating comments, and spacing out code properly enhances maintainability and reduces the learning curve for others reviewing your scripts.
# This script retrieves running services and displays their names
Get-Service | Where-Object { $_.Status -eq 'Running' } | ForEach-Object { $_.DisplayName }
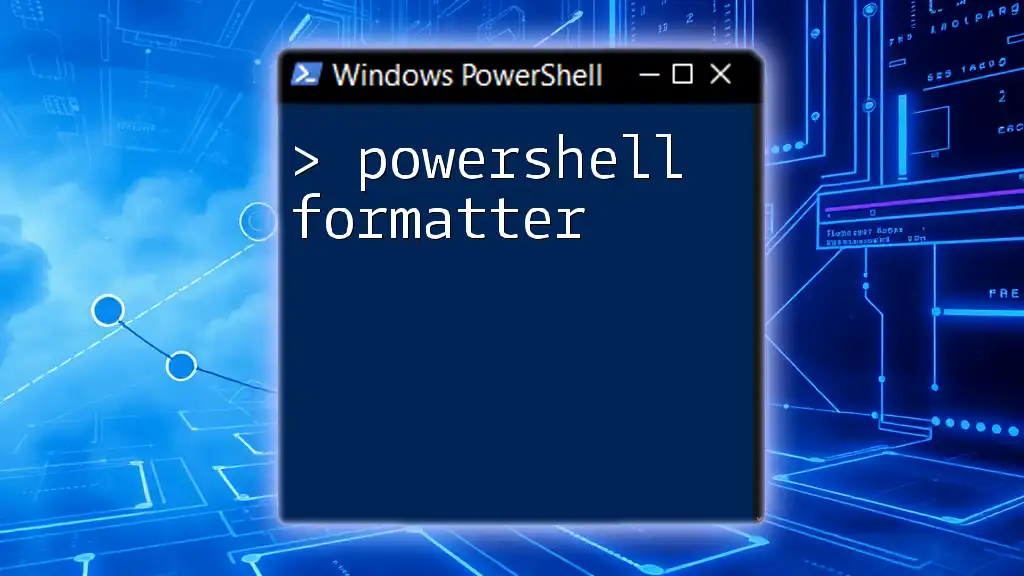
Troubleshooting Common Issues
Common Errors with Foreach and Where
When using `Foreach` and `Where`, you may encounter issues such as variable scope problems or invalid expressions in your conditions. PowerShell is sensitive to syntax; make sure to validate your expressions carefully.
Debugging Tips
Leverage the `Write-Debug` cmdlet to output intermediate results for troubleshooting. Running your script with `-Debug` will provide insights into variable contents and operations occurring at each step.
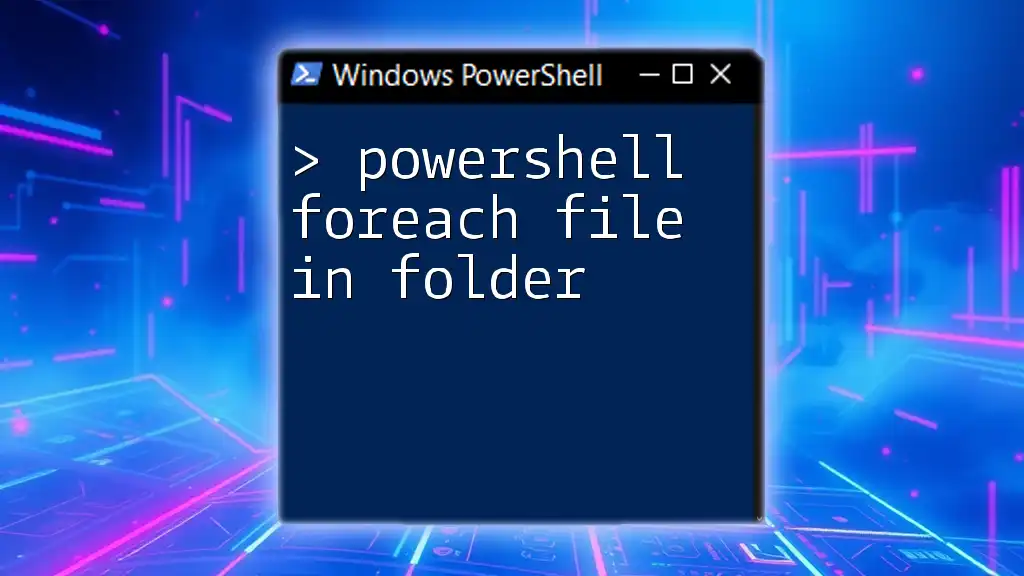
Conclusion
In this guide, we explored the essential `Foreach` and `Where` commands in PowerShell. Combining these commands effectively will enhance your scripting capabilities and streamline your workflows. Embrace practice and experimentation to fully grasp the nuances of PowerShell scripting. Ready to dive deeper? Explore more PowerShell resources and enhance your skill set for efficient automation and system management.