The `whereis` command in PowerShell is utilized to find the location of a command executable or script within the system's paths. Here's a simple code snippet demonstrating its use:
where.exe notepad
This command returns the path to the Notepad executable if it exists in the system's environment paths.
Understanding Where-Object
What is Where-Object?
The `Where-Object` cmdlet is a powerful tool in PowerShell that allows users to filter objects based on specific criteria. It enables you to evaluate each object in a collection and return only those that meet a certain condition. This is especially useful when you need to sift through large sets of data or files and find elements that fit your requirements.
Syntax Breakdown
The basic syntax of the `Where-Object` cmdlet can be represented as follows:
Where-Object [-Property] <String> [-Value] <Object>
In this syntax:
- `-Property` refers to the property of the object you're filtering. This could be any attribute, such as `Name`, `Length`, or `Status`.
- `-Value` is the expected value of the property specified. This allows you to set conditions for what you're searching for.
Additionally, you can use parameters like `-FilterHashtable` to filter objects using a hashtable that specifies conditions for filtering.
Common Uses of Where-Object
`Where-Object` can be used in various scenarios to filter objects efficiently. Below are some common examples:
- Example 1: Filtering files by size
Get-ChildItem | Where-Object { $_.Length -gt 1MB }
In this example, you list all items in the current directory, filtering only those that are larger than 1MB.
- Example 2: Finding processes by name
Get-Process | Where-Object { $_.Name -like "*chrome*" }
Here, you're fetching all processes and filtering to include only those whose names contain "chrome".
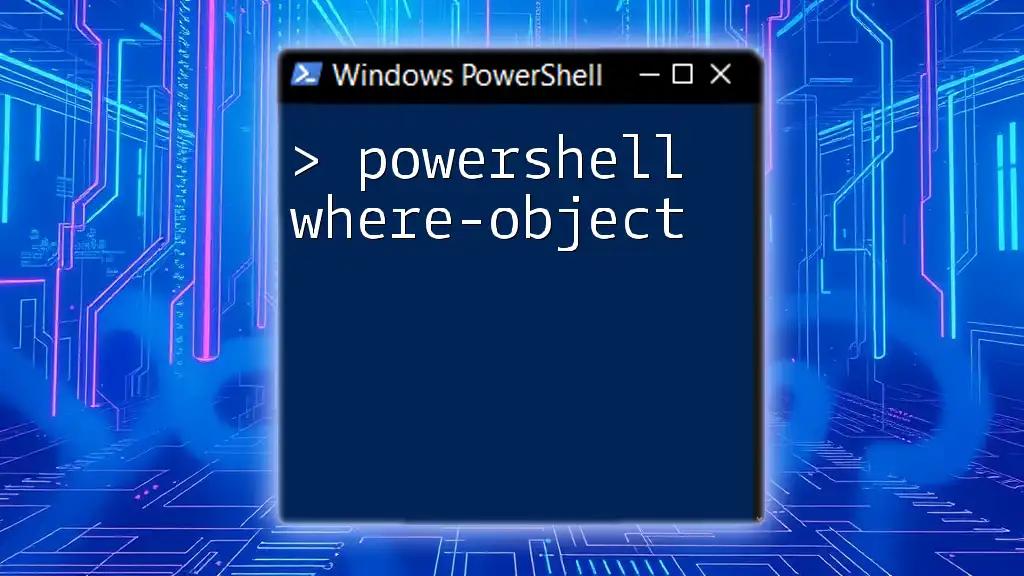
Practical Applications of Where-Object
Navigating the File System
One of the most practical applications of the `Where-Object` cmdlet is navigating the file system. It allows you to filter files and directories effectively, saving time and effort.
- Finding specific file types
Get-ChildItem -Recurse | Where-Object { $_.Extension -eq ".txt" }
This command searches through all directories recursively and returns only `.txt` files, allowing you to pinpoint text documents quickly.
Working with Services
Another key application is filtering system services using `Where-Object`. This can help you manage or monitor your services effectively.
- Listing running services
Get-Service | Where-Object { $_.Status -eq 'Running' }
In this example, you're filtering the list of all services to include only those that are currently running, helping you quickly identify services that are active and operational.
Filtering Events and Logs
`Where-Object` can also be particularly useful in filtering system events and logs. This functionality is vital for monitoring system health and troubleshooting.
- Getting specific events
Get-WinEvent -LogName System | Where-Object { $_.LevelDisplayName -eq 'Error' }
With this command, you gather event logs from the System log and filter them to display only events marked as errors, which can help in identifying issues within the system.

Advanced Techniques with Where-Object
Using Script Blocks for Complex Queries
`Where-Object` allows for advanced filtering through the use of script blocks. Script blocks enable you to create more sophisticated conditions for filtering.
- Example of complex filtering
Get-ChildItem | Where-Object { $_.Length -gt 1MB -and $_.CreationTime -lt (Get-Date).AddMonths(-1) }
This code retrieves files larger than 1MB that were created over a month ago. The use of logical operators like `-and` provides a way to combine conditions, yielding precise results.
Combining Where-Object with Other Cmdlets
Pipelining is a foundational concept in PowerShell that allows you to pass the output of one cmdlet as the input to another. `Where-Object` excels in this area, enhancing its functionality and usability.
- Example of combining cmdlets
Get-Process | Where-Object { $_.WorkingSet -gt 100MB } | Sort-Object -Property WorkingSet -Descending
In this example, you first gather all processes, filter those with a working set greater than 100MB, and then sort the result by working set size in descending order. This approach provides a quick way to identify resource-intensive applications.

Tips for Effective Use of Where-Object
Performance Considerations
When using `Where-Object`, performance is key, especially when dealing with large datasets. While the cmdlet is powerful, it’s also crucial to use it judiciously—inefficient filtering can lead to longer processing times.
Best Practices:
- Use more specific filters first to reduce the amount of data passed through the pipeline.
- Limit the use of `Where-Object` on large collections whenever possible.
Error Handling
As with any scripting task, using `Where-Object` can lead to common mistakes. Understanding these pitfalls can save valuable time.
Common errors include:
- Misnaming properties or not using the correct casing.
- Forgetting that the `$_` variable represents the current object in the pipeline.
Debugging PowerShell scripts effectively will enable you to identify these mistakes easily.
Recommendations for Learning Resources
The PowerShell ecosystem is vast, and continuous learning is essential. Here are some recommended resources:
- Official PowerShell Documentation: Comprehensive and up to date.
- Online Courses: Platforms like Pluralsight and Udemy offer courses specifically on PowerShell.
- Community Forums: Participate in PowerShell user groups and forums for support and sharing knowledge.

Conclusion
The `Where-Object` cmdlet is an incredibly versatile asset in PowerShell that significantly enhances your ability to filter and manipulate data. By leveraging its capabilities effectively, you can streamline your workflows, making it simpler to focus on what truly matters in your PowerShell tasks. Remember to practice using `Where-Object` in various scenarios, and don’t hesitate to explore advanced filtering techniques to maximize your scripting efficiency.

FAQs
What is the difference between Where-Object and other filtering cmdlets?
While `Where-Object` is specifically designed for filtering objects based on conditions, other cmdlets like `Select-Object` are used for selecting specific properties from an object without filtering based on conditions.
Can Where-Object be used with custom PowerShell objects?
Absolutely! You can use `Where-Object` with custom PowerShell objects by filtering based on the properties of those objects. For example:
$customObjects = @(
[PSCustomObject]@{ Name = 'Item1'; Value = 100 },
[PSCustomObject]@{ Name = 'Item2'; Value = 200 }
)
$customObjects | Where-Object { $_.Value -gt 150 }
This filters out only the objects whose `Value` is greater than 150.
How do I chain multiple Where-Object filters?
Chaining multiple `Where-Object` filters is straightforward and can refine your queries. For instance:
Get-ChildItem | Where-Object { $_.Length -gt 1MB } | Where-Object { $_.Extension -eq ".txt" }
Here, you can chain filters to first get items larger than 1MB and then further filter to only include `.txt` files. This enhances the granularity of your search results.