In PowerShell, a heredoc is a convenient way to define multi-line strings, allowing you to include large blocks of text without needing to concatenate or escape quotes.
Here's a simple example of a heredoc in PowerShell:
$heredoc = @'
This is a heredoc.
It allows you to write multiple lines of text.
Goodbye, World!
'@
Write-Host $heredoc
What is a Here-Doc in PowerShell?
In PowerShell, a Here-Doc, often referred to as a heredoc, is a method for defining string literals that span multiple lines. This feature primarily simplifies the process of handling long strings or blocks of text without the need for line continuation characters, making scripts cleaner and more readable. The term "heredoc" originates from programming languages like Perl but has been adapted for use in PowerShell.
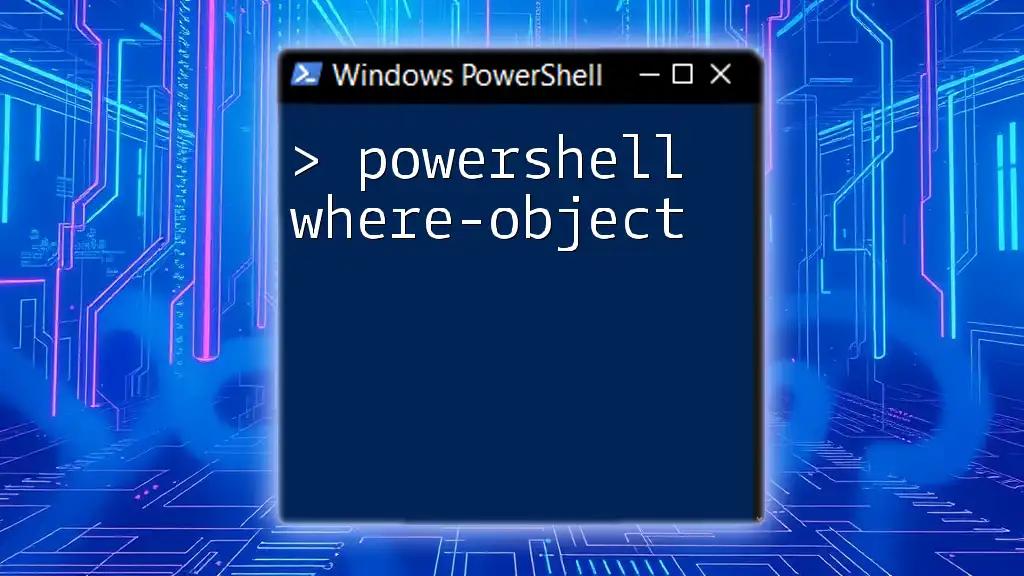
Understanding Here Strings in PowerShell
Definition of Here Strings
A Here String in PowerShell is a special type of string that allows developers to include line breaks and quotes directly in the string without requiring escape characters. This capability makes Here Strings particularly useful for creating multi-line content, such as SQL queries, HTML templates, or email bodies.
Syntax of Here Strings
There are two types of syntax for Here Strings in PowerShell: single-quoted and double-quoted Here Strings.
- Single-Quoted Here Strings:
- Defined with `@'` at the start and `'@` at the end.
- Do not evaluate variables within the string.
- Double-Quoted Here Strings:
- Defined with `@"` at the start and `@"` at the end.
- Evaluate variables and support interpolation within the string.
Syntax Examples
Single-Quoted Here String Example
Here’s an example of a single-quoted Here String that demonstrates how variables are treated:
$variableName = "PowerShell"
$singleQuotedString = @'
This is a single-quoted Here String.
It does not evaluate variables: $variableName
'@
In this case, `$singleQuotedString` would contain the literal text of `$variableName`, not its value, resulting in:
This is a single-quoted Here String.
It does not evaluate variables: $variableName
Double-Quoted Here String Example
Now, let's look at a double-quoted Here String where variables are evaluated:
$variableName = "PowerShell"
$doubleQuotedString = @"
This is a double-quoted Here String.
It evaluates variables: $variableName
"@
The output of `$doubleQuotedString` would be:
This is a double-quoted Here String.
It evaluates variables: PowerShell
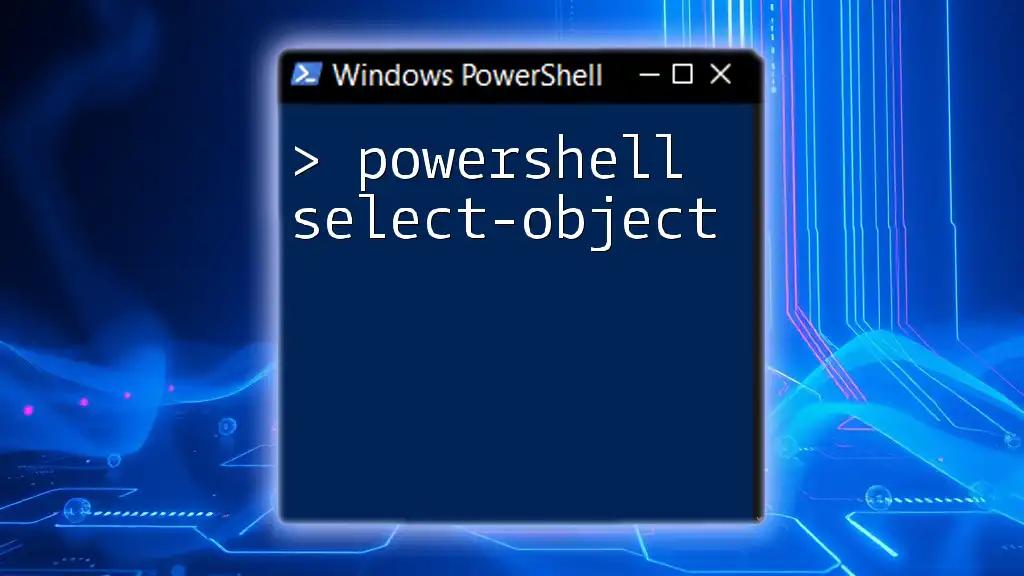
Key Advantages of Using Here Strings
Multi-line String Handling
One of the most significant advantages of using Here Strings is the ability to manage multi-line strings effortlessly. Instead of appending strings with concatenation or using `"` and `"` for line breaks, Here Strings allow you to nest entire blocks of text neatly.
Readability and Maintenance
Here Strings drastically improve code readability. When dealing with large blocks of text, especially in configurations or scripts, Here Strings provide a clear visual structure. This leads to easier maintenance as changes can be made in an organized way without affecting surrounding code or breaking the flow.
Embedding Code and Scripts
Another powerful use case for Here Strings is wrapping large code snippets or scripts within a main script. This is particularly useful for scenarios like defining a body of HTML or a SQL command where maintaining syntax clarity is crucial.
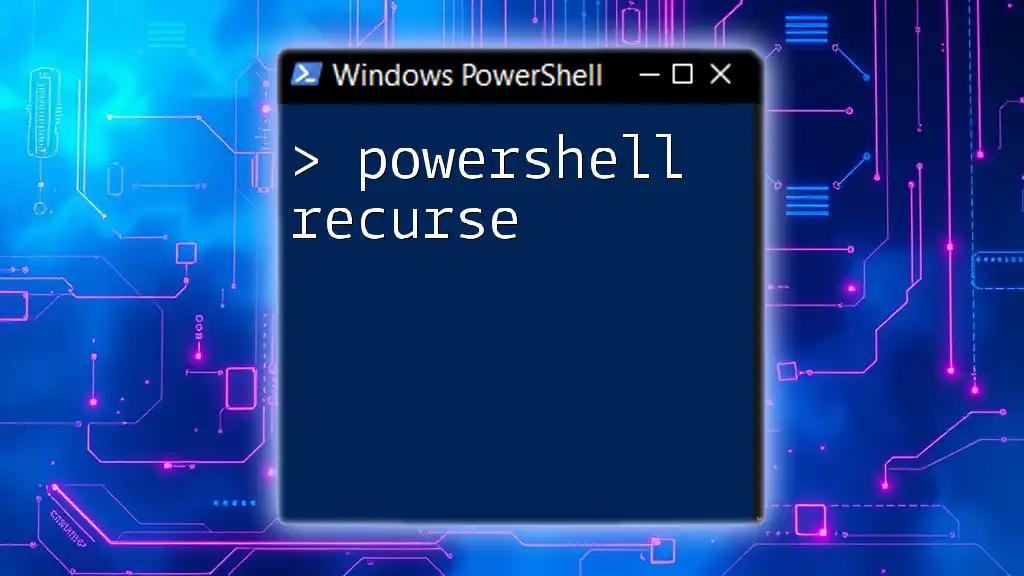
Practical Use Cases for Here Strings
Script Blocks and Documentation
Here Strings are highly effective in script blocks, allowing developers to encapsulate lengthy pieces of text or comments. This usage benefits not just the current script but also provides context when revisiting or sharing the script with others.
Sending Emails with PowerShell
You can create formatted email content using Here Strings. Here's an example of how to use a Here String for the body of an email:
$EmailBody = @"
Hello Team,
This is to inform you about the upcoming meeting on Friday.
Best regards,
Your Name
"@
Send-MailMessage -To "team@example.com" -From "you@example.com" -Subject "Meeting Reminder" -Body $EmailBody -BodyAsHtml
In this example, `$EmailBody` is a neatly defined multi-line string, ensuring your email contains readable and formatted content.
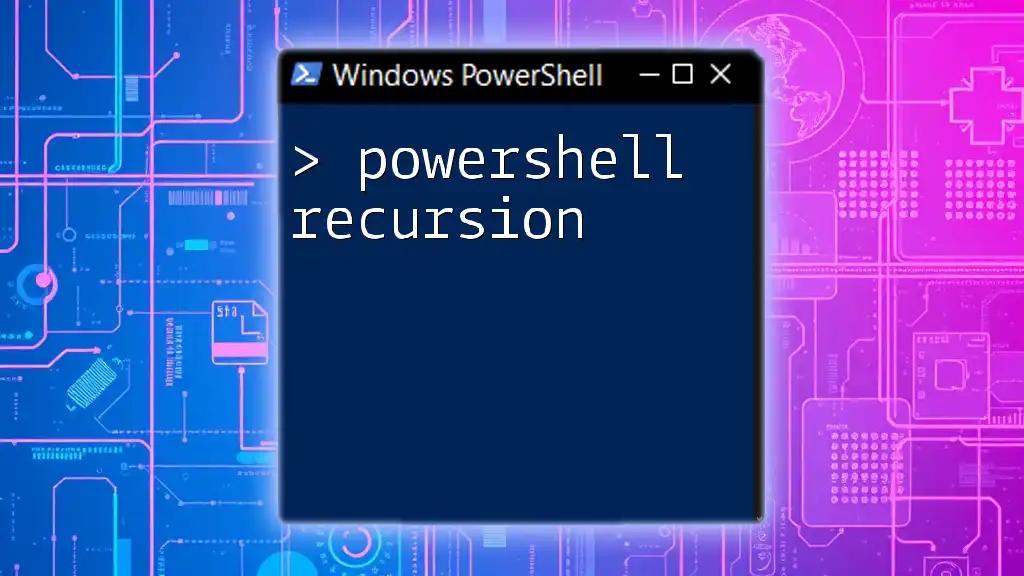
Common Mistakes and Troubleshooting
Despite their usefulness, Here Strings can trip up even seasoned developers. Here are some common mistakes:
-
Incorrect usage of quotes: Mixing up single and double quotes can lead to syntax errors. For example, attempting to define a Here String with single quotes but using a double quote at the end.
-
Forgetting to end the Here String: Make sure you close your Here String properly, or PowerShell will throw an error, leading to confusion.
-
Unexpected variable evaluation: In the case of accidentally using single quotes when you meant to use double quotes, remember that variable evaluation won't occur.
Debugging tip: Always double-check the quotes and ensure you have the correct opening and closing syntax.
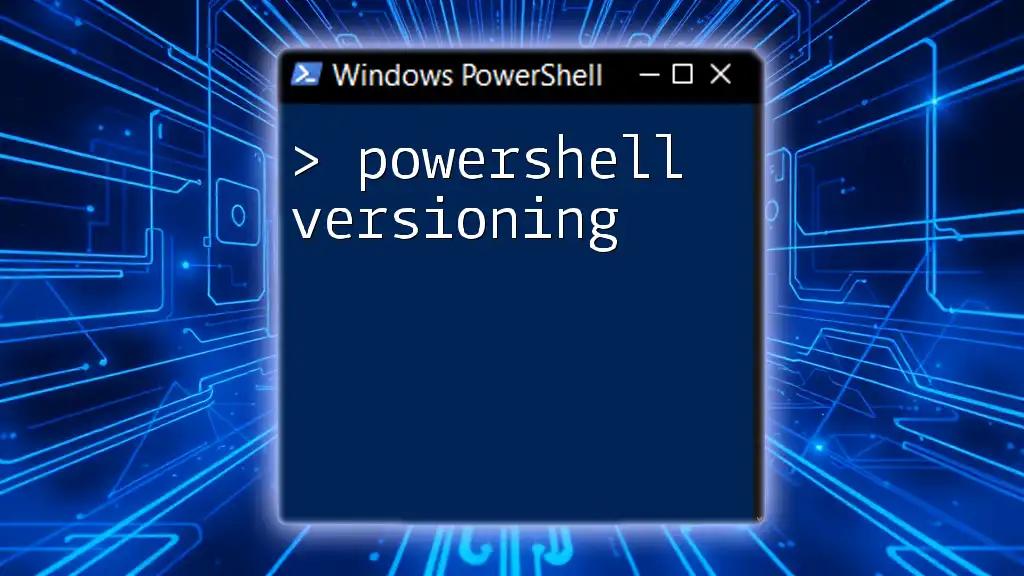
Conclusion
PowerShell Here-Docs, or Here Strings, are an essential feature for anyone looking to enhance their scripting capabilities. They simplify multi-line string handling, promote readability, and allow for variable interpolation where necessary. By mastering Here Strings, you not only streamline your scripts, but you also improve maintainability and collaboration.
Dive deeper into PowerShell features and explore how these concepts can be integrated into your daily scripting practices for effective automation and command control.