PowerShell recursive operations allow you to perform actions on items in a directory and its subdirectories, enabling efficient management and automation of tasks.
Get-ChildItem -Path "C:\Example\Path" -Recurse
Understanding Recursion in PowerShell
What is Recursion?
Recursion is a fundamental concept in programming where a function calls itself in order to solve a problem. It usually consists of two major components: a base case, which stops the recursive calls, and the recursive case, which continues to call the function until it reaches the base case.
A simple analogy for recursion is the process of descending a staircase: if you’re at the top step, you step down one step at a time until you reach the bottom. Each step represents a recursive call, and the bottom step is your base case.
Recursive vs. Iterative Approaches
When faced with a problem, you might be tempted to use either a recursive or an iterative approach (loops). Here are the main differences:
-
Recursive Methods:
- Elegantly express solutions that involve repetitive tasks.
- Can lead to more straightforward and manageable code.
-
Iterative Methods:
- Typically faster and more memory-efficient.
- Avoid the risk of stack overflow that can occur with deep recursive calls.
Consider using recursion when dealing with problems that are inherently hierarchical, like traversing tree structures or directory trees.
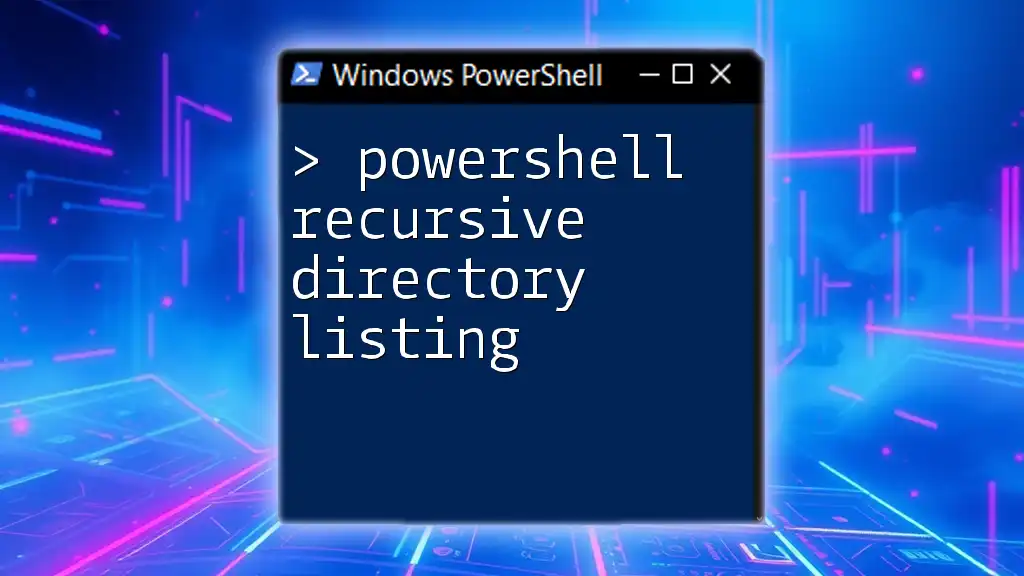
Basic PowerShell Recursive Functions
Creating a Simple Recursive Function
In PowerShell, creating a recursive function is straightforward. Below is an example of a recursive function that calculates the factorial of a number:
function Factorial($num) {
if ($num -le 1) {
return 1
} else {
return $num * (Factorial($num - 1))
}
}
In this code, if the number is less than or equal to one, the function returns 1 (the base case). For other numbers, it multiplies the number by the factorial of the number minus one (the recursive case).
Tail Recursion in PowerShell
Tail recursion is a special case where the recursive call is the last operation in the function. Its primary advantage is that it can be optimized by the compiler to prevent increasing the call stack depth.
Here’s how you could implement a tail-recursive factorial function:
function TailFactorial($num, $acc=1) {
if ($num -le 1) {
return $acc
} else {
return TailFactorial($num - 1, $num * $acc)
}
}
This approach is more memory-efficient because it reuses the same stack frame for all calls.
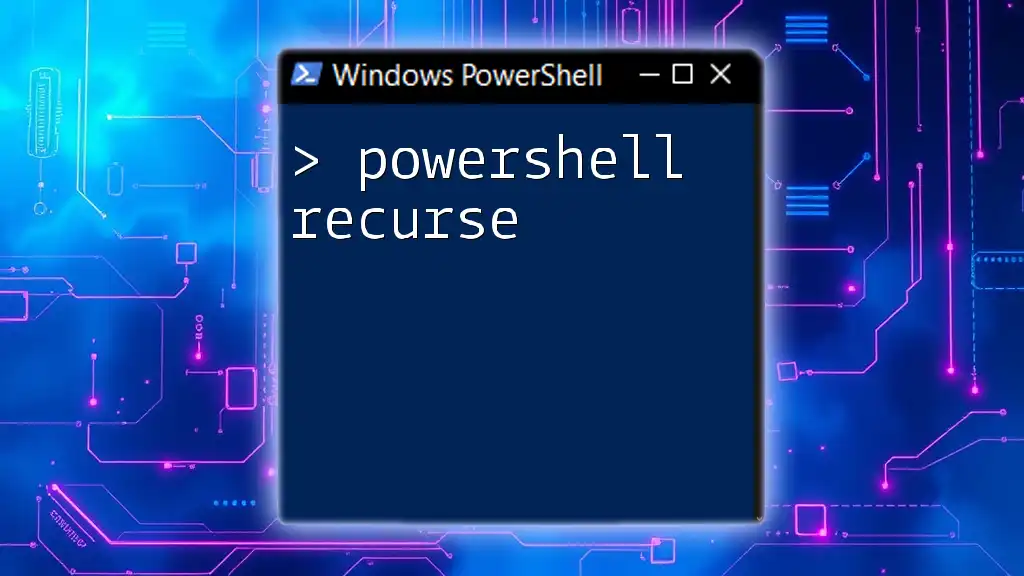
Working with File Systems: Recursive Tasks in PowerShell
Recursively Listing Files
One of the practical uses of recursion in PowerShell is traversing the filesystem. You can use the `Get-ChildItem` cmdlet with the `-Recurse` parameter to list all files and directories in a specified path:
Get-ChildItem -Path "C:\Your\Directory" -Recurse
This command retrieves all files and subfolders within the specified directory, providing a complete view of the directory structure.
Deleting Files Recursively
When managing files, recursion can be powerful but potentially risky, especially during deletion. Always proceed with caution. Below is a command that deletes all `.tmp` files recursively from a specified directory:
Get-ChildItem -Path "C:\Your\Directory" -Include *.tmp -Recurse | Remove-Item
In this command, `Get-ChildItem` selects `.tmp` files, which are then deleted by piping the output to `Remove-Item`. Be wary of using this command indiscriminately, as it may lead to the permanent loss of important files.
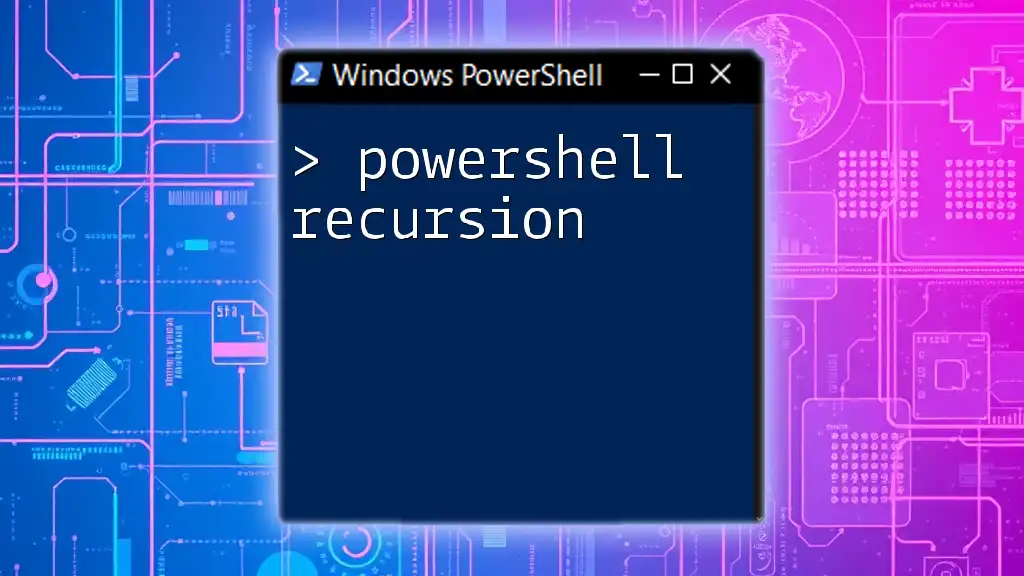
Using Recursive Functions for Complex Operations
Example: Searching for a Specific File Type
Creating a recursive function to search for files of a specific type can be highly beneficial. Here's a function that finds all `.txt` files in any nested directory:
function Find-TxtFiles {
param ($path)
Get-ChildItem -Path $path -Recurse -Filter "*.txt"
}
Here, `Get-ChildItem` provides a convenient way to search for text files while exploring the directory tree, making it quick and efficient.
Example: Calculating the Size of a Directory
Another practical application of recursion is calculating the total size of a directory by summing up the sizes of all files within it. Below is a PowerShell function that accomplishes this task:
function Get-DirectorySize {
param ($path)
$size = 0
Get-ChildItem -Path $path -Recurse | ForEach-Object {
$size += $_.Length
}
return $size
}
This function uses `Get-ChildItem` to retrieve all files recursively. It then iterates through each file with `ForEach-Object` and sums their sizes. The total size is returned at the end of the function.
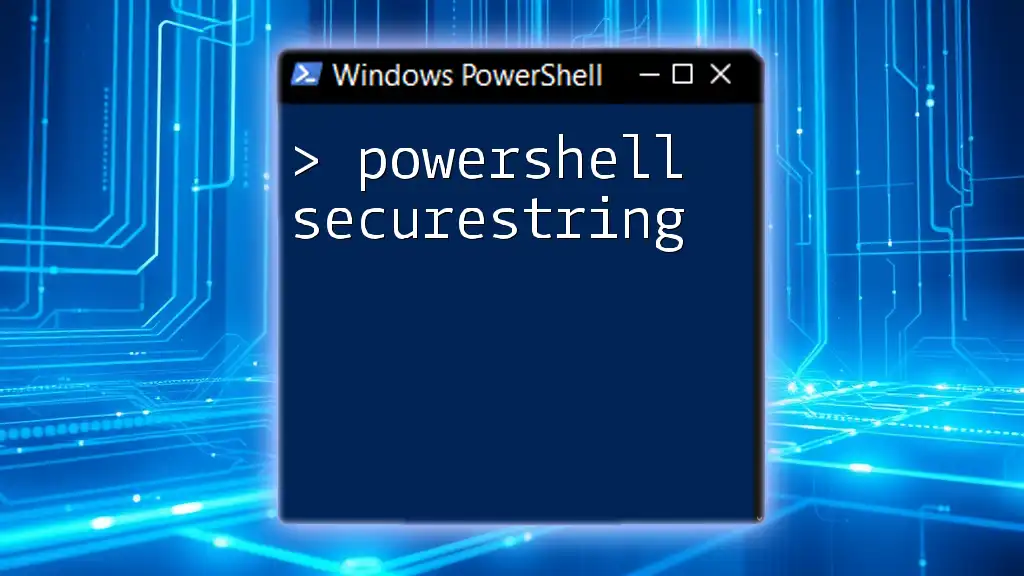
Best Practices for Writing Recursive PowerShell Scripts
Managing Stack Overflow Risks
One of the critical issues with recursion is stack overflow, which occurs when there are too many nested calls. To manage this risk:
- Limit Recursion Depth: Implement checks to ensure the function does not call itself too many times.
- Use Tail Recursion: Where possible, utilize tail recursion to minimize the call stack depth.
Commenting and Documentation
Writing well-commented code is essential, especially for recursive functions. Since recursion can be less straightforward than iterative code, clear documentation helps others (and your future self) understand the flow and logic.
For example:
function Factorial {
param ($num)
# Base case: if the input number is less than or equal to 1, return 1.
if ($num -le 1) {
return 1
} else {
# Recursive case: multiply the number by the factorial of the number - 1.
return $num * (Factorial($num - 1))
}
}
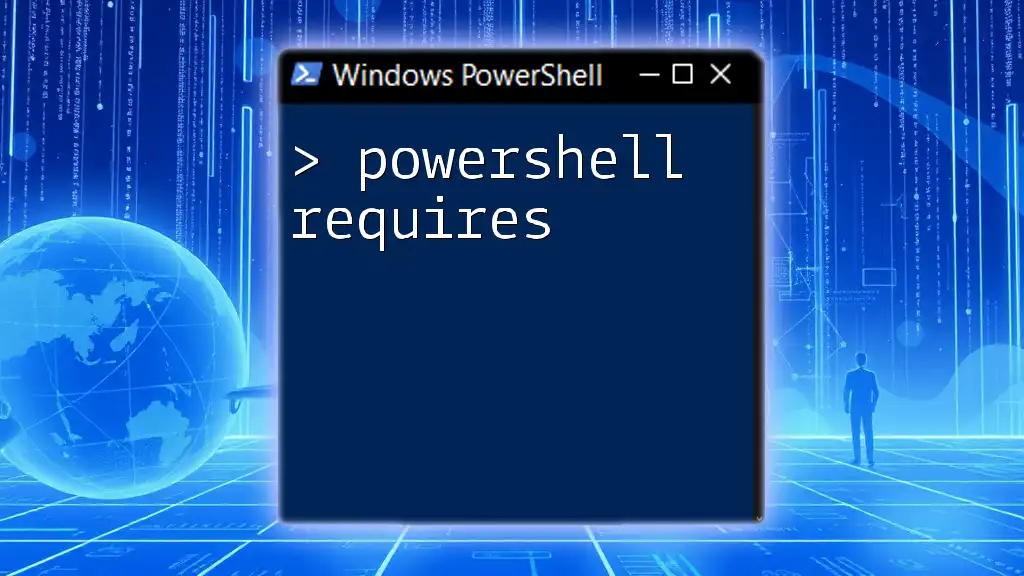
Conclusion
In summary, mastering PowerShell recursive techniques can significantly enhance your scripting capabilities. Whether you are calculating factorials, listing files, or performing more complex operations, recursion provides a powerful toolset for problem-solving.
Start experimenting with your recursive functions today, and explore more of our PowerShell tutorials for practical applications that will elevate your scripting skills!
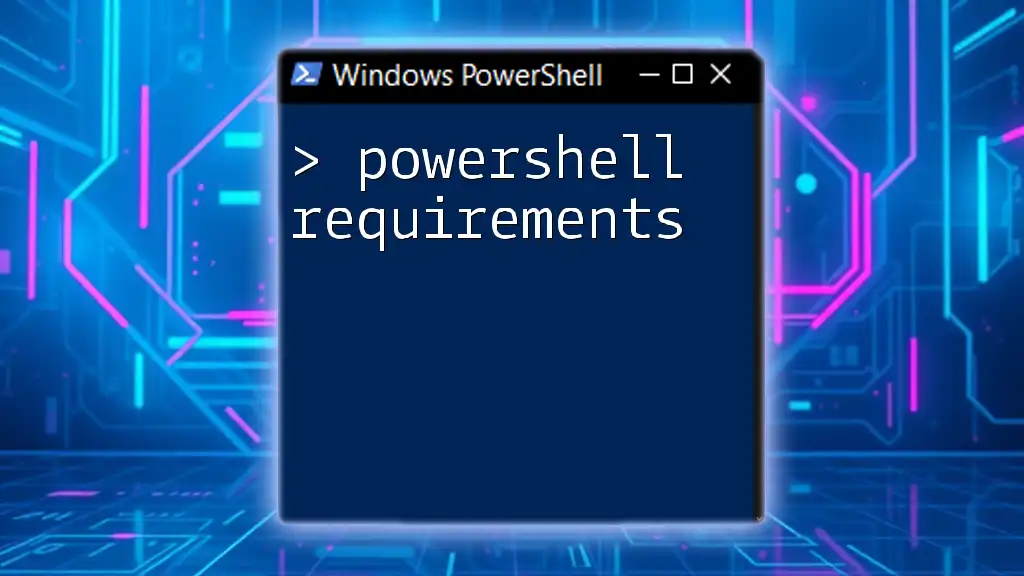
Additional Resources
For further exploration, consider visiting the official PowerShell documentation, enrolling in recommended online courses, or participating in discussion forums that cater to PowerShell users. These resources will propel your understanding of PowerShell recursive approaches to new heights.