The `Receive-Job` cmdlet in PowerShell is used to retrieve the results of background jobs that have been started using `Start-Job`.
$job = Start-Job -ScriptBlock { Get-Process }
Receive-Job -Job $job
Understanding PowerShell Jobs
What are PowerShell Jobs?
PowerShell jobs are asynchronous tasks that allow scripts to run in the background. They can be classified into two types: background jobs and scheduled jobs. Background jobs facilitate the execution of tasks without hindering the main script’s flow, while scheduled jobs are set to run at specific times or intervals.
Typically, jobs are used in automation scenarios where tasks like file processing, system monitoring, or data retrieval are performed without requiring the user to wait for completion, enabling a more efficient scripting experience.
Importance of Jobs in PowerShell
Utilizing jobs in PowerShell enhances the performance of scripts significantly. Here are a few key benefits:
- Concurrency: Multiple jobs can run simultaneously, allowing you to leverage system resources effectively.
- Separation of tasks: Jobs help in isolating long-running tasks, enabling scripts to perform other operations concurrently.
- Real-world applications: Whether gathering system data or executing complex scripts, jobs facilitate better resource management and increase overall productivity.
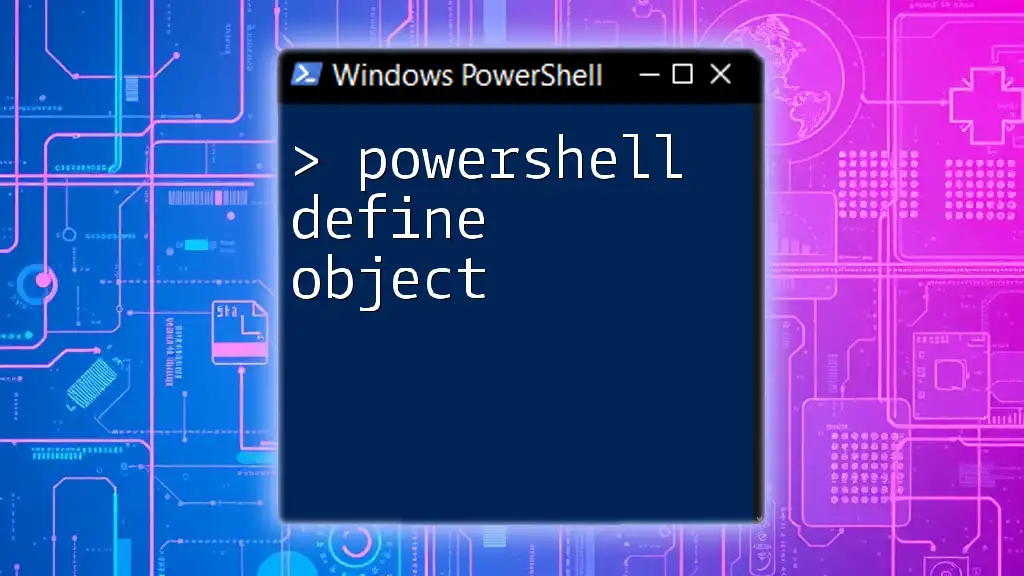
The `Receive-Job` Command
Introduction to `Receive-Job`
The `Receive-Job` command is a crucial part of managing background jobs in PowerShell. It serves the purpose of retrieving the results of a job once it has completed. Understanding how and when to use this command is vital for effective job management.
Syntax and Parameters
The syntax for `Receive-Job` is as follows:
Receive-Job [-Id] <Int32> [-Keep] [-Verbose] [-Debug] [-ErrorAction <ActionPreference>] [-ErrorVariable <String>] [-OutVariable <String>] [-OutBuffer <Int32>] [<CommonParameters>]
Here's a breakdown of some important parameters:
- `-Id`: Specifies the job ID for the job from which to receive results. Each job is assigned a unique identifier.
- `-Keep`: This parameter is useful when you want to retain the job in memory after receiving the results. This can help in further analysis or debugging later.
- `-Verbose`: When added, this parameter provides detailed information during execution, which can be beneficial for troubleshooting.
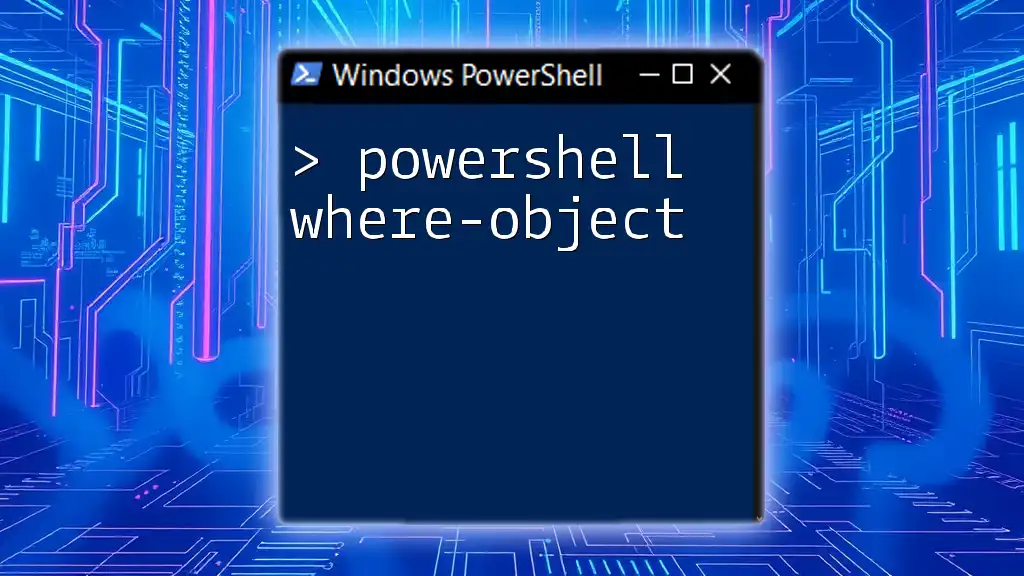
Practical Examples of Using `Receive-Job`
Basic Example: Simple Job Creation and Reception
To illustrate how to use `Receive-Job`, consider the following example where we create a new job that retrieves the current list of active processes on the system.
# Create a new job
$job = Start-Job { Get-Process }
# Receive the job results
$results = Receive-Job -Id $job.Id
$results
In this example, a background job is initiated to execute the `Get-Process` cmdlet, which retrieves all current processes. Once the job has completed, `Receive-Job` is used to capture the output.
Advanced Example: Using Jobs with Complex Scripts
Script to Retrieve System Information
This snippet demonstrates starting a job to gather system information:
# Start a job to gather system information
$systemJob = Start-Job { Get-ComputerInfo }
# Retrieve and display the job results
$systemInfo = Receive-Job -Id $systemJob.Id -Keep
$systemInfo
In this example, the `Get-ComputerInfo` cmdlet collects detailed information about the local computer. By using the `-Keep` parameter, the job remains accessible after receiving its results, providing flexibility to retrieve the same information later if needed.
Error Handling and Job Management
When working with jobs, error handling is essential. You can use `try/catch` blocks to manage potential issues:
# Example of error handling with Receive-Job
try {
$output = Receive-Job -Id $job.Id
} catch {
Write-Host "Error occurred: $_"
}
This approach ensures that your scripts can handle exceptions gracefully, providing feedback on any problems encountered while receiving job results.
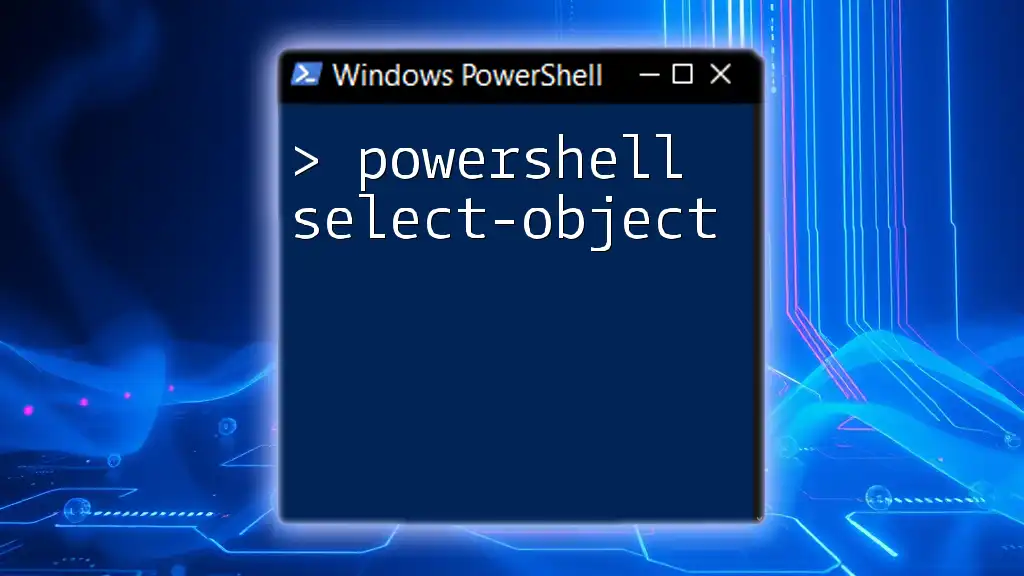
Viewing Job Status and Results
Checking Job Status Before Receiving Results
Before receiving job results, it’s often beneficial to check the status of your jobs. You can do this using the `Get-Job` cmdlet, which lists all jobs and their respective states:
Get-Job | Where-Object {$_.State -eq 'Running'}
This command filters for jobs that are currently running, helping you determine which jobs can be safely received.
Cleaning Up Jobs After Receiving Results
After using `Receive-Job`, it’s important to clean up no longer needed jobs to free up system resources. You can achieve this using the `Remove-Job` cmdlet:
# Clean up jobs after use
Remove-Job -Id $job.Id
This command ensures that you remove jobs that have served their purpose, keeping your PowerShell environment optimized.
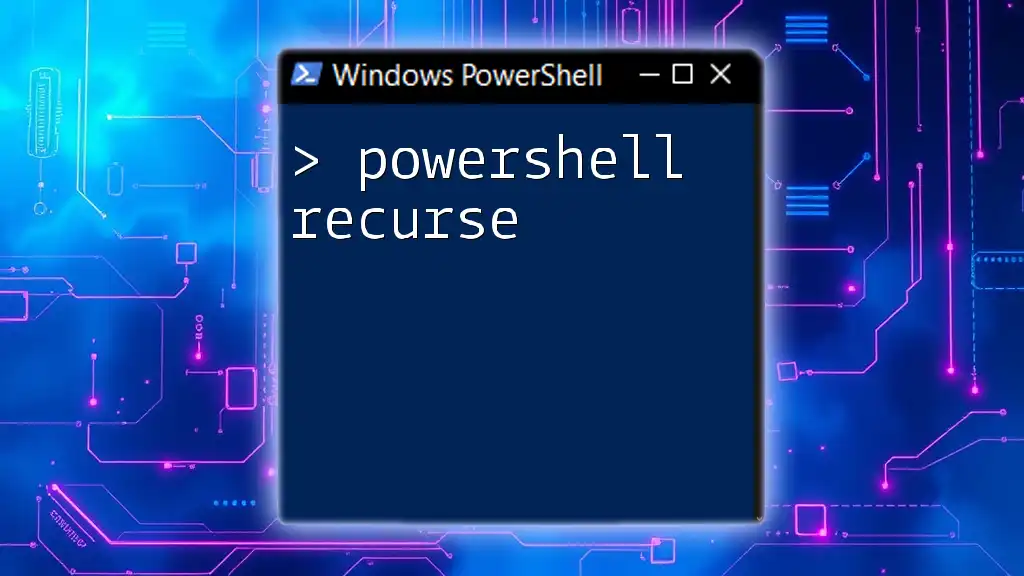
Best Practices for Using `Receive-Job`
Performance Considerations
When leveraging the `powershell receive job`, it’s crucial to optimize job usage. Here are some tips for better performance:
- Limit the number of concurrent jobs: Too many active jobs can overwhelm system resources.
- Batch processing: Group tasks that share similar resources or dependencies to minimize context switching.
Workflow Considerations
Integrating jobs within larger scripts enhances functionality. Consider the following strategies:
- Use `ForEach-Object` with jobs: This enables you to process multiple items in parallel effortlessly.
- Use job results in subsequent commands: Preserve output from jobs to feed into other cmdlets, enhancing script modularity.
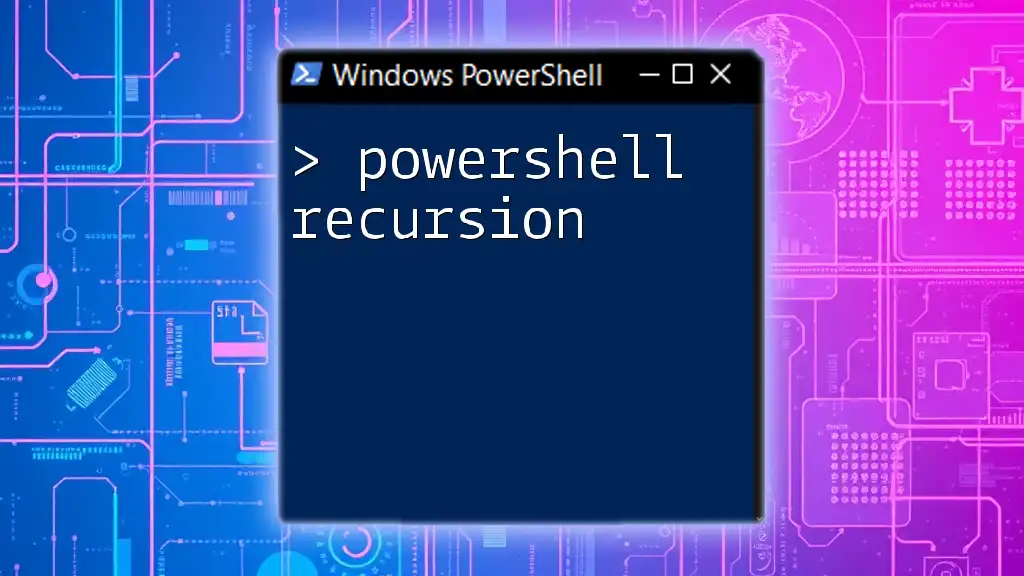
Conclusion
Understanding PowerShell receive job is essential for effective job management in PowerShell scripts. By leveraging `Receive-Job` along with the other job management cmdlets, you can create adaptable, high-performing scripts that enhance workflow automation and processing capacity.
Experimenting with background jobs can help you unlock the full potential of PowerShell scripting. Embrace the possibilities that `Receive-Job` offers and incorporate it into your everyday scripting activities.
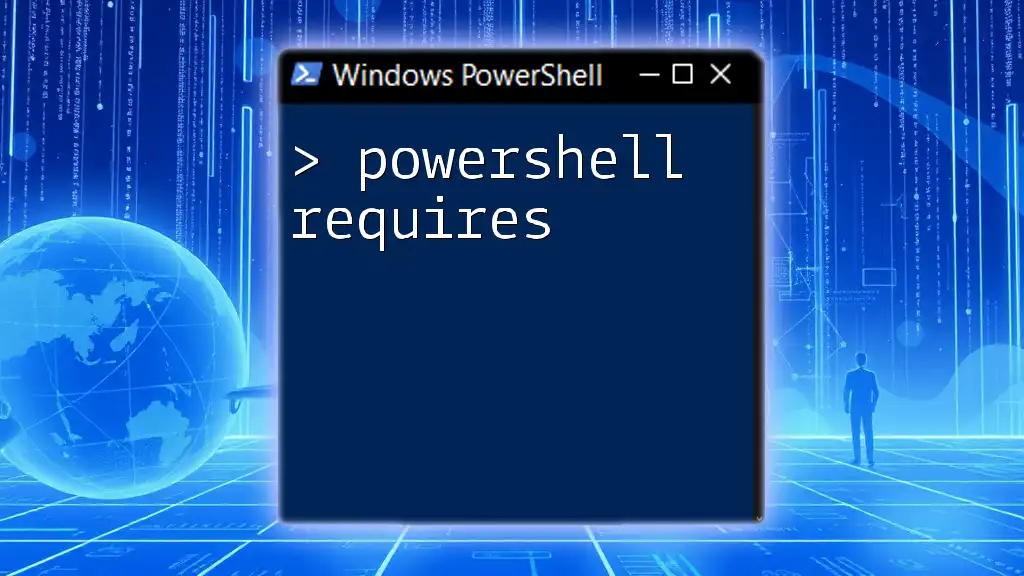
Additional Resources
To further your understanding of PowerShell jobs, consider exploring the official Microsoft documentation, joining community forums for dynamic discussions, and debugging tips, or reviewing foundational PowerShell resources like books and online courses.
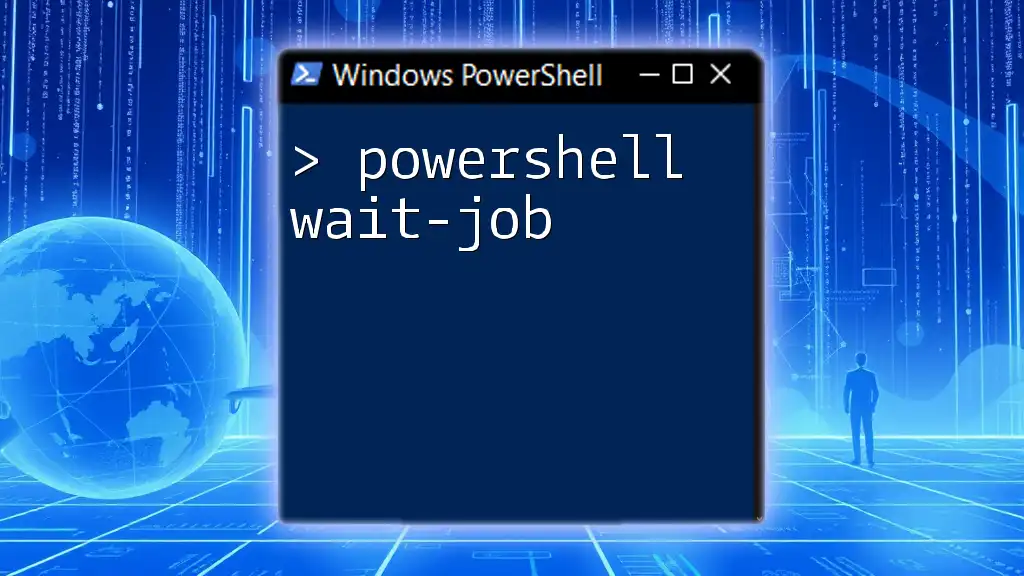
FAQs
Common questions about `Receive-Job` often relate to its application in complex scripts, error-handling techniques, and performance optimization. Addressing these key areas will prepare you for any challenges you may encounter when working with PowerShell jobs.