In PowerShell, you can easily replace a substring within a string using the `-replace` operator, as shown in the following example:
$originalString = "Hello, World!"
$modifiedString = $originalString -replace "World", "PowerShell"
Write-Host $modifiedString # Output: Hello, PowerShell!
Understanding PowerShell String Manipulation
The Role of Strings in PowerShell
Strings are fundamentally important in PowerShell, serving as a crucial data type for storing and manipulating text. PowerShell extensively uses strings for input and output processes, configuring settings, and formatting data. Understanding how to manipulate strings effectively can greatly enhance your scripting capabilities and improve the automation of repetitive tasks.
Importance of Replacing Substrings
In many automation scenarios, especially when dealing with configuration files or user inputs, the need to replace certain substrings becomes paramount. For example, you may want to modify messages, replace default values, or sanitize data before processing it. Replacing substrings helps in managing and customizing the output, facilitating a more tailored user experience and data management.
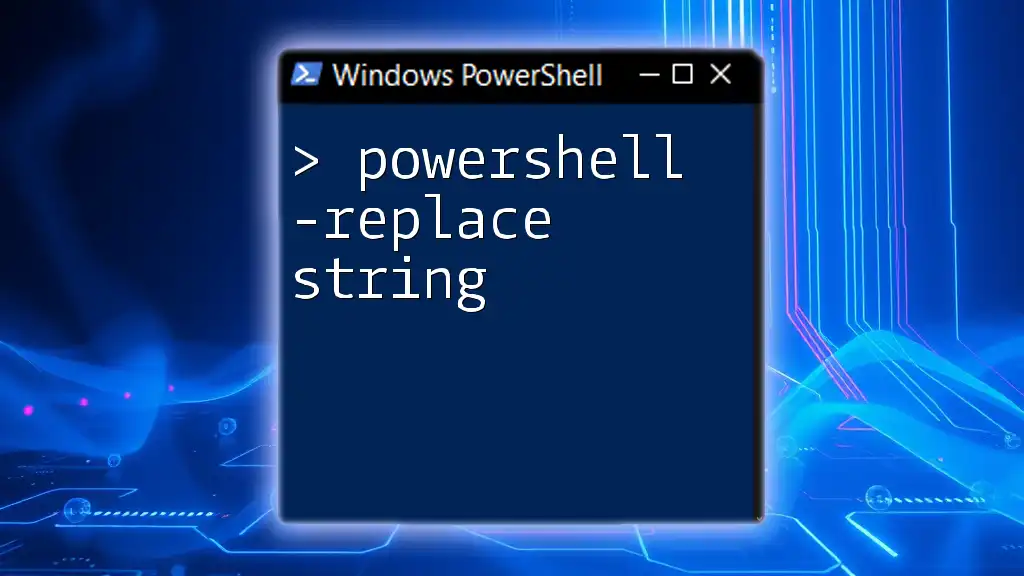
How to Use `-replace` Operator
What is the `-replace` Operator?
The `-replace` operator in PowerShell provides a simple yet powerful way to replace substrings. It employs regular expressions, allowing for flexible pattern matching. The syntax generally looks like this:
$newString = $originalString -replace 'pattern', 'replacement'
Key Features:
- It handles case sensitivity by default.
- It supports regex patterns, making it adept for complex replacements.
Basic Example of Using `-replace`
Here's a straightforward example demonstrating the `-replace` operator:
$message = "Hello, World!"
$updatedMessage = $message -replace "World", "PowerShell"
Write-Output $updatedMessage # Output: Hello, PowerShell!
In this example, "World" is replaced with "PowerShell", showcasing how easy it is to modify strings using this operator.
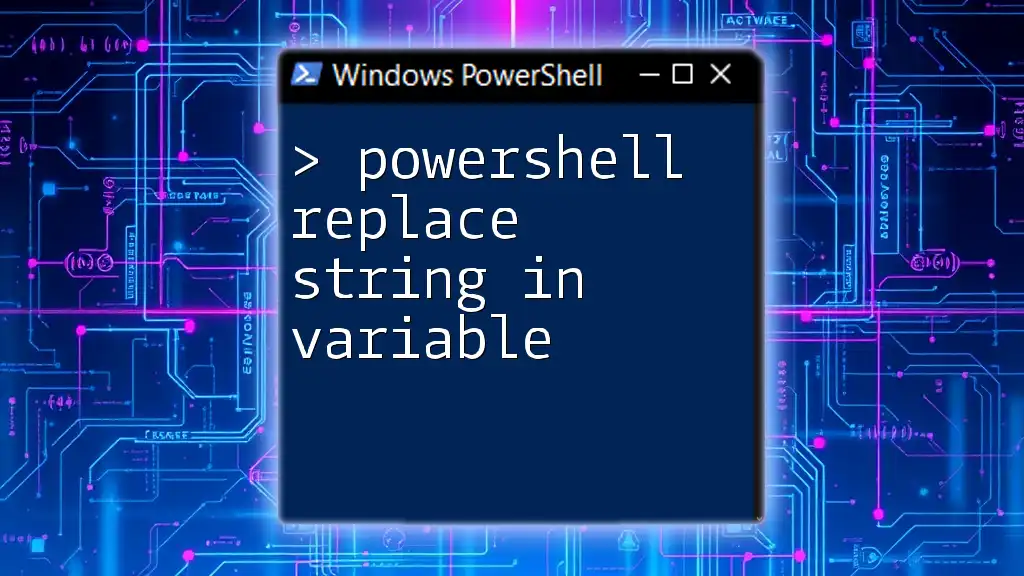
Exploring the `.Replace()` Method
Understanding the `.Replace()` Method
While `-replace` is fantastic for its regex functionality, the `.Replace()` method is useful when you need to replace static substrings without the complexity of regular expressions. This method is called on string objects and follows this syntax:
$newString = $originalString.Replace('oldValue', 'newValue')
Example of Using `.Replace()`
Here’s how you can utilize the `.Replace()` method:
$message = "Hello, World!"
$updatedMessage = $message.Replace("World", "PowerShell")
Write-Output $updatedMessage # Output: Hello, PowerShell!
In this scenario, `.Replace()` functions similarly to `-replace`, emphasizing its ease of use for straightforward substring replacements.
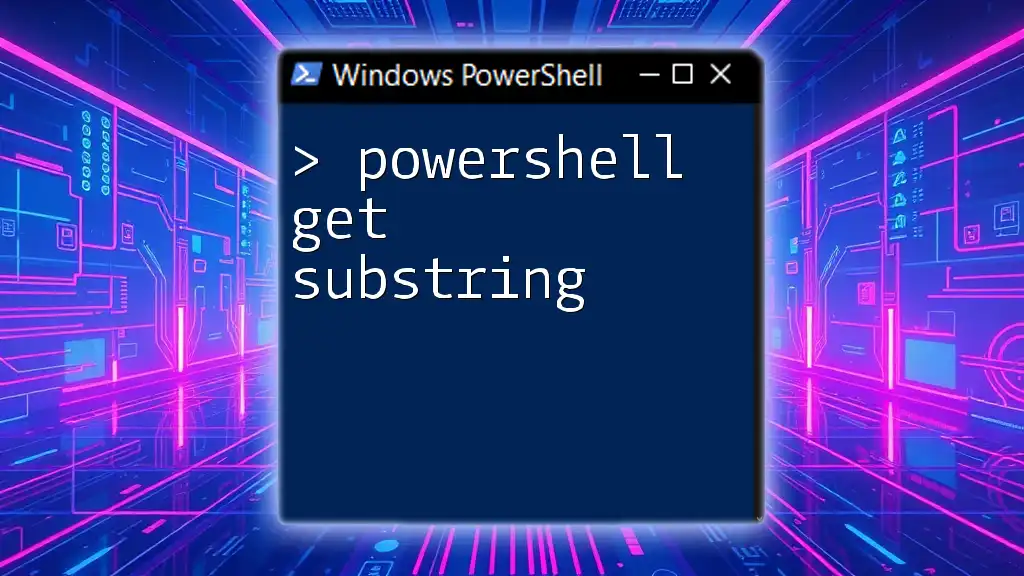
Advanced String Replacement Techniques
Using Regular Expressions with `-replace`
Delving deeper into string manipulation, using regular expressions with the `-replace` operator unlocks advanced capabilities such as wildcard matching. This approach is particularly beneficial when you don't know the exact substring but have a pattern in mind.
Example of Regex with `-replace`
$message = "Hello, World! Welcome to World!"
$updatedMessage = $message -replace "World", "PowerShell"
Write-Output $updatedMessage # Output: Hello, PowerShell! Welcome to PowerShell!
This example replaces all instances of "World" with "PowerShell", demonstrating the ease of applying regex with `-replace`.
Replacing Multiple Substrings
Using an Array with `-replace`
When dealing with multiple substrings, you can organize your replacements in a hashtable and loop through it:
$message = "Hello World, the World is beautiful"
$substitutions = @{"World"="PowerShell"; "beautiful"="awesome"}
foreach ($key in $substitutions.Keys) {
$message = $message -replace $key, $substitutions[$key]
}
Write-Output $message # Output: Hello PowerShell, the PowerShell is awesome
In this code, multiple substrings are seamlessly replaced, showcasing the efficiency of using a structured approach.
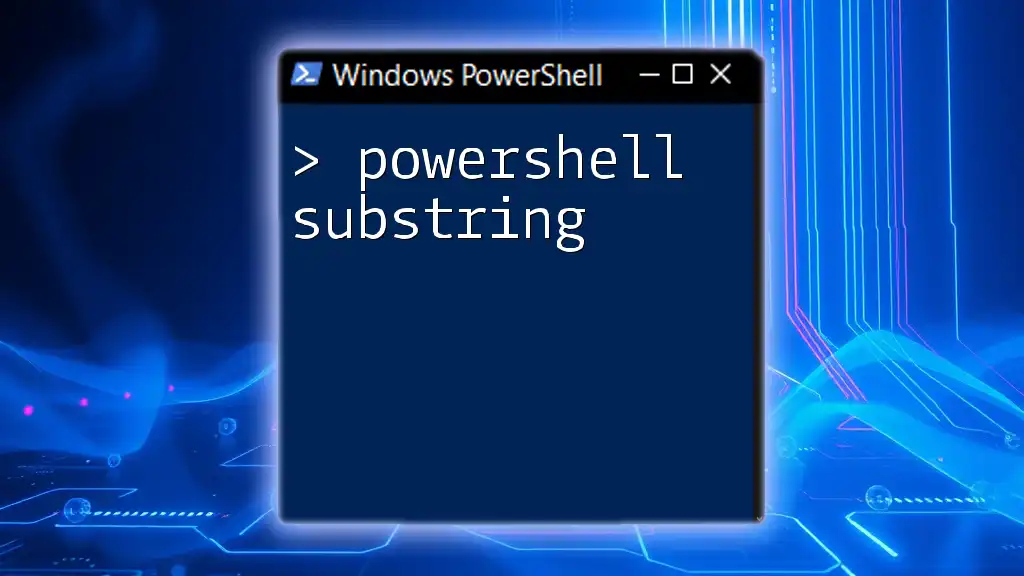
Handling Edge Cases
PowerShell String Replacement and Case Sensitivity
It's crucial to understand how PowerShell handles case sensitivity in replacements. By default, `-replace` is case sensitive, while `.Replace()` is not. This difference can significantly affect the outcome of your string manipulations.
Example of Case Insensitive Replacement
For instance, if you want to replace a substring without considering case sensitivity, `-replace` can be employed as follows:
$message = "Hello, world!"
$updatedMessage = $message -replace "world", "PowerShell", "IgnoreCase"
Write-Output $updatedMessage # Output: Hello, PowerShell!
In this case, "world", regardless of its casing, gets replaced with "PowerShell".
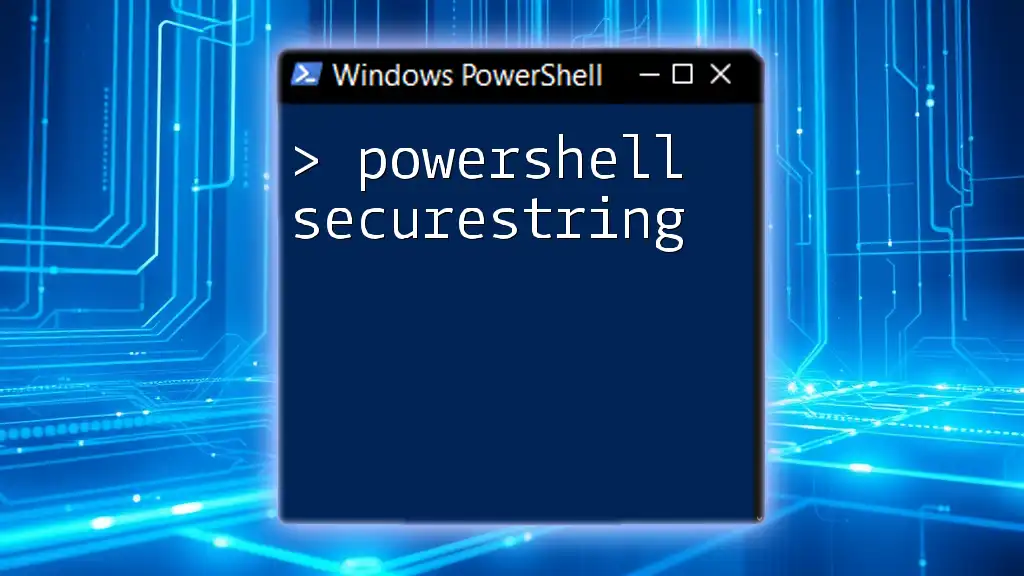
Performance Considerations
When to Use `-replace` vs. `.Replace()`
Choosing between `-replace` and `.Replace()` often depends on the complexity of the replacement. If your task involves simple, non-regex replacements, `.Replace()` tends to be faster and more readable. However, if you need the flexibility of regex for pattern matching, opt for the `-replace` operator.
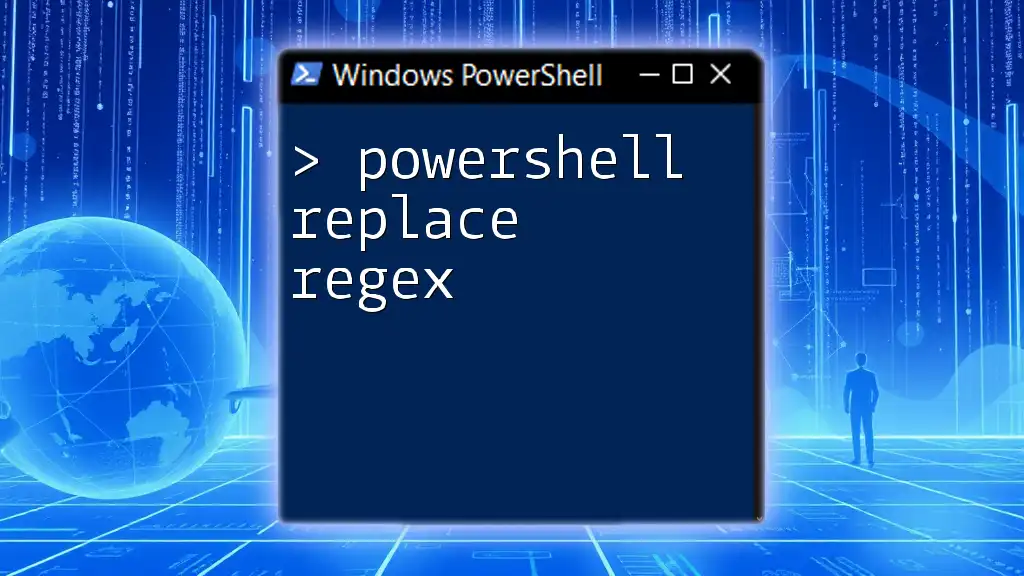
Practical Applications
Replacing Substrings in Configuration Files
In practical applications, replacing substrings can be particularly valuable when working with configuration files. For example, if you have configurations that need updates (like API keys), you can use substring replacement to automate modifications rather than manually editing files.
Automating Text Reports
String replacement also plays a significant role in automating text report generation. For example, you can use `-replace` or `.Replace()` to substitute placeholders with real data dynamically. Here’s a snippet that showcases this:
$template = "Hello {Name}, your balance is {Balance}."
$report = $template.Replace("{Name}", "John").Replace("{Balance}", "$1000")
Write-Output $report # Output: Hello John, your balance is $1000.
This method allows for quick and dynamic report generation based on user data.
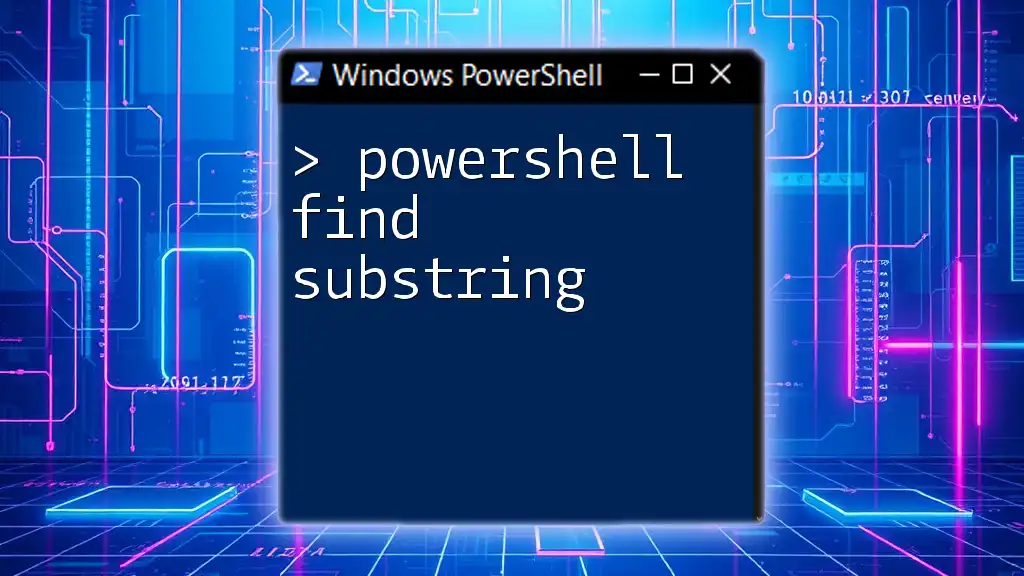
Conclusion
Through effective use of the `-replace` operator and the `.Replace()` method, you can significantly enhance your PowerShell scripting capabilities. Understanding their functionalities paves the way for better string manipulation techniques, making your scripts more robust and flexible. As you explore these string replacement tools further, consider practicing their applications across different scenarios to strengthen your command over PowerShell.
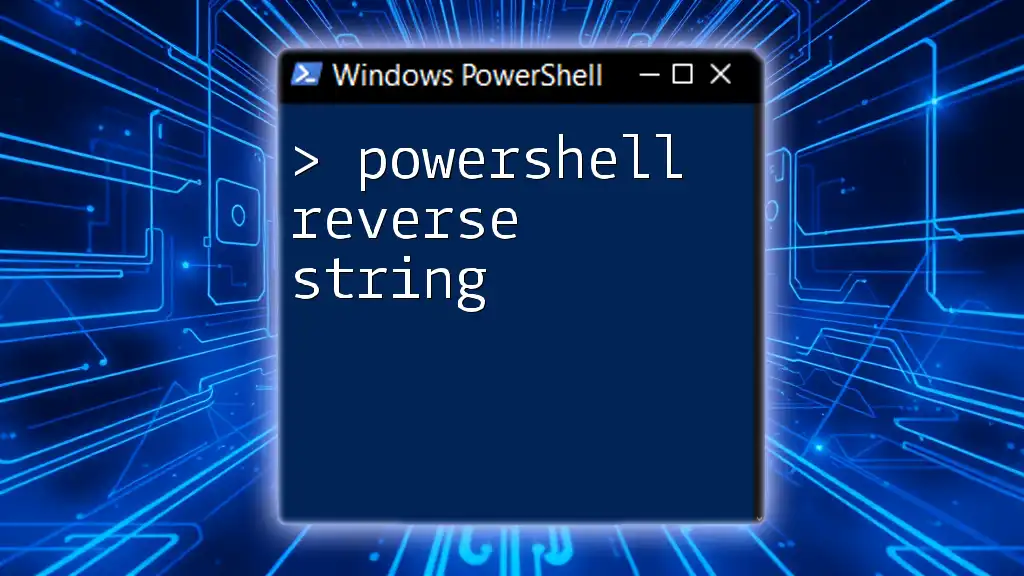
Call to Action
If you're eager to elevate your PowerShell skills, consider signing up for our courses or tutorials. Dive deeper with related articles and resources, and take your automation capabilities to the next level!