In PowerShell, you can extract a specific substring from a string using the `Substring` method, which takes the starting index and an optional length as parameters.
Here's a code snippet that demonstrates how to get a substring:
# Extracting a substring from a string
$string = "Hello, World!"
$substring = $string.Substring(7, 5) # Extracts 'World'
Write-Host $substring
Understanding Substrings in PowerShell
What is a Substring?
A substring is a sequence of characters that exists within a larger string. The concept of substrings is fundamental in programming as it allows developers to manipulate and extract specific portions of strings for various uses, such as displaying data, logging information, or formatting outputs.
Why Use Substrings in PowerShell?
Mastering substring manipulation in PowerShell opens a variety of possibilities. Substrings are commonly used to:
- Process and analyze data: Strings often contain structured information, and breaking them down into manageable pieces enhances clarity.
- Output and formatting: Presenting data in a human-readable format is crucial, and substrings allow you to extract and format pieces of information as required.
- Script optimization: Efficient use of substrings can lead to more readable, maintainable, and performant PowerShell scripts.
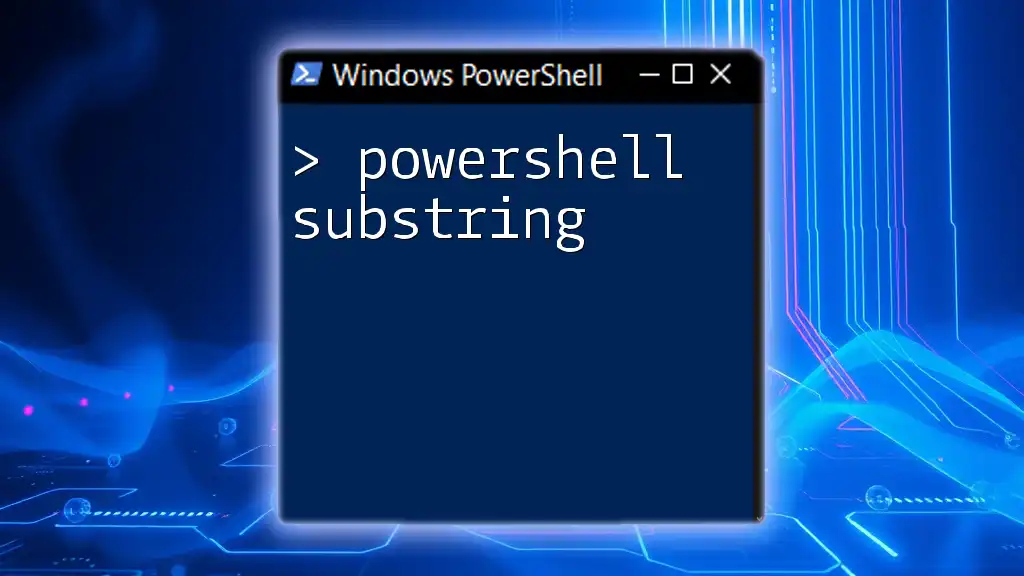
PowerShell Get Substring Command
Syntax of the Get Substring Method
In PowerShell, the primary method to retrieve a substring from a string is the `Substring()` method. Its basic syntax looks like this:
String.Substring(startIndex, length)
- startIndex: This is the zero-based index where the substring begins.
- length: This indicates how many characters are included in the substring.
Basic Example of Using Get Substring
Here's a simple example to illustrate the basic usage of the `Substring()` method:
$exampleString = "Hello, PowerShell!"
$substring = $exampleString.Substring(7, 10)
Write-Output $substring # Output: PowerShell
In this example, we start extracting from index 7 (the "P" in "PowerShell") and take 10 characters, which gives us "PowerShell".
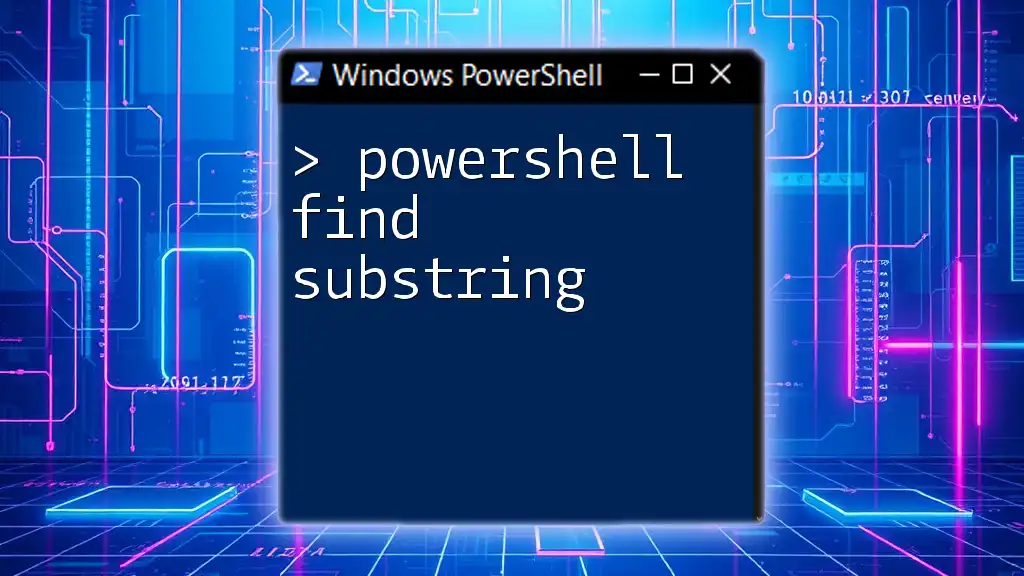
Powershell Left Substring
What is Left Substring?
The left substring refers to the portion of a string that is taken from the beginning up to a specified length. This concept is often used in cases where you need the first part of a string without concern for what comes after it.
Using Left Substring in PowerShell
Here's how you can implement a left substring in PowerShell:
$exampleString = "PowerShell Programming"
$leftSubstring = $exampleString.Substring(0, 10)
Write-Output $leftSubstring # Output: PowerShell
In this code snippet, the `Substring()` method starts at the beginning of the string (index 0) and takes the first 10 characters, resulting in "PowerShell".
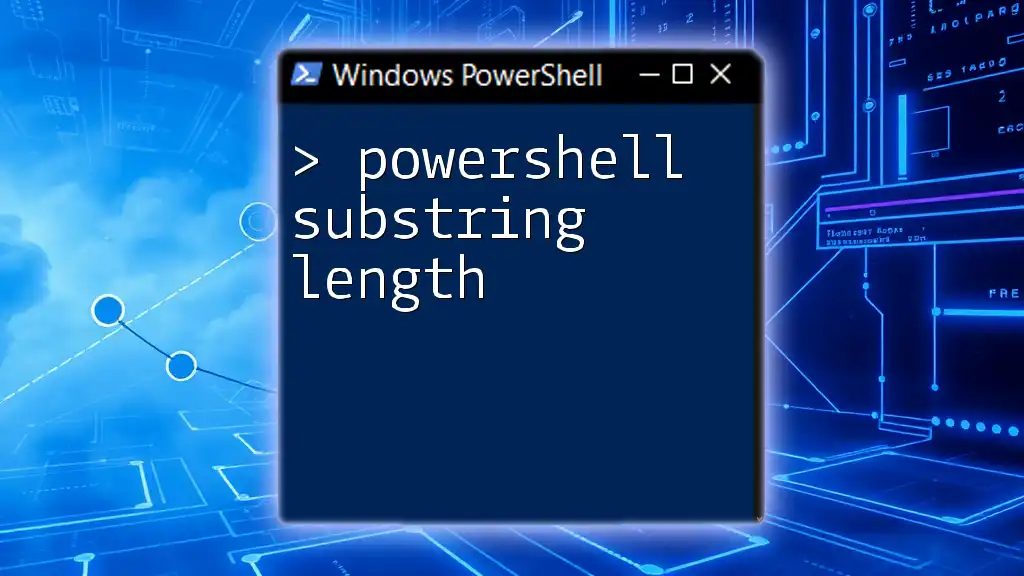
Powershell Extract Substring
How to Extract Substrings in PowerShell
PowerShell provides multiple ways to extract substrings. The `Substring()` method allows for specific locations and lengths, but sometimes you might need to retrieve parts of a string based on varying indices. Regular expressions can also be an alternative for more complex extraction tasks.
Code Examples for Extracting Substrings
Example 1: Extracting a substring from a file path
Imagine you have a file path from which you want to extract the filename:
$file = "C:\Users\Owner\Documents\Report.txt"
$fileName = $file.Substring($file.LastIndexOf('\') + 1)
Write-Output $fileName # Output: Report.txt
In this example, the `LastIndexOf()` method finds the position of the last backslash, and `Substring()` retrieves everything after it, effectively isolating the file name.
Example 2: Extracting variable parts from a string
You can also extract dynamic portions based on some criteria. For instance, if you have a mixed string like:
$fullString = "ProductID:12345|ProductName:Widget"
$productID = $fullString.Substring(10, 5) # Output: 12345
Write-Output $productID
Here, `Substring(10, 5)` extracts the digits corresponding to the product ID, starting from the position right after "ProductID:".
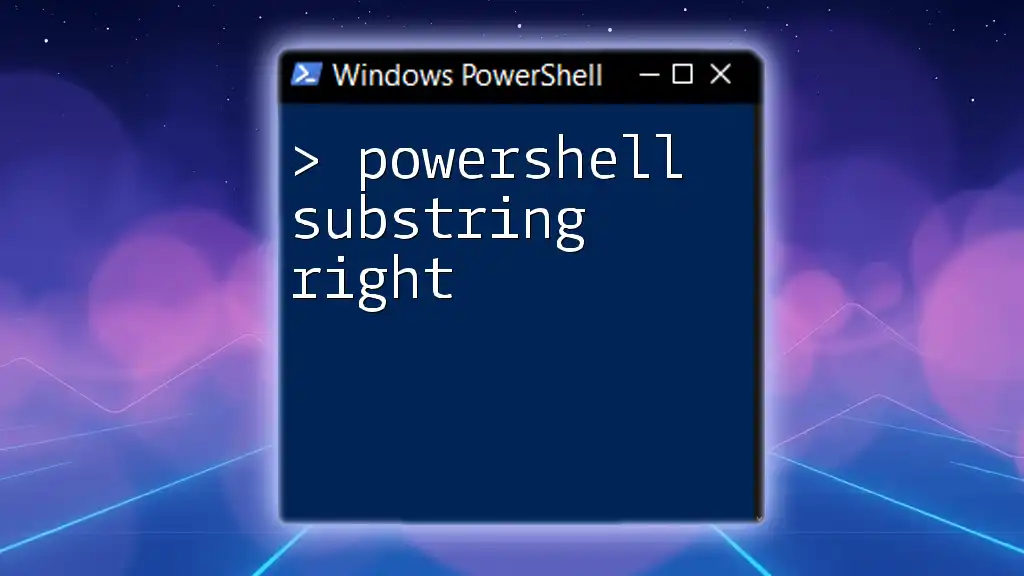
Powershell Substring Left: An Alternative Approach
Utilizing the `-replace` Operator
Sometimes, using the `-replace` operator can be a more flexible approach to extract the left portion of a string. This operator is used for string replacement but can easily adapt for extracting substrings.
Example of Substring Left with `-replace`
$exampleString = "FirstName:LastName"
$leftPart = $exampleString -replace ":.*", ""
Write-Output $leftPart # Output: FirstName
In this snippet, the `-replace` operator is used to remove everything following the colon, including the colon itself. This effectively gives you the first name.
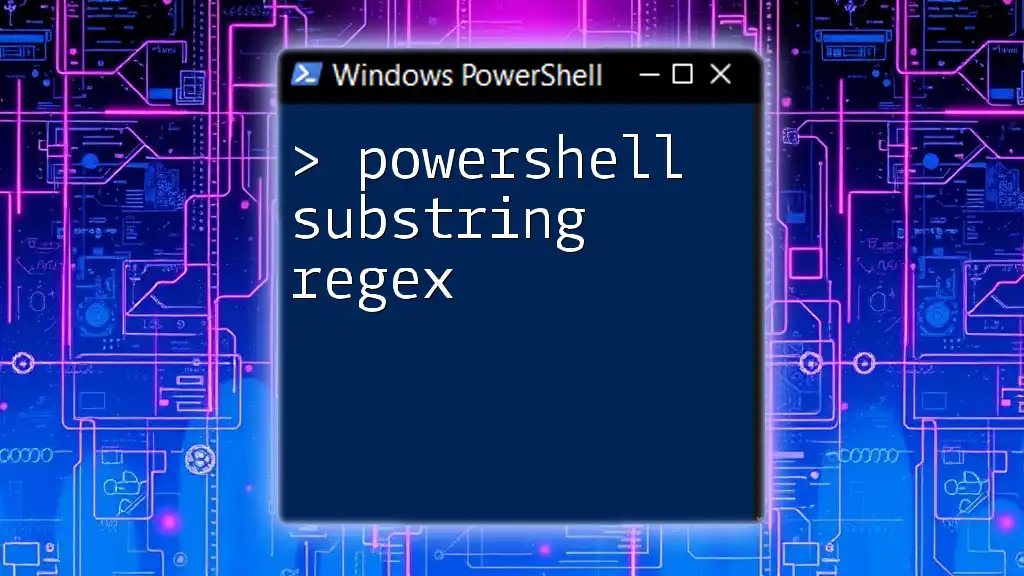
Powershell Substring Examples
Practical Use Cases for Substring Operations
-
Trimming Text: Substrings can help cleanse input by removing unwanted characters or formatting. For example, extracting only relevant information from inputs such as logs or user input.
-
Dynamic Data Processing: Substrings can be valuable when processing data that may change in length. You can use loops in combination with the substring methods to dynamically retrieve parts of each record.
-
Formatting Output: By using substrings effectively, you can construct more user-friendly outputs, such as displaying parts of an address or reformatted names.
Code Snippet Collection
Here’s a collection of various substring techniques that highlight the versatility of substrings in PowerShell:
$address = "123 Main St, Springfield, IL"
$city = $address.Substring(15, 11) # Expecting Springfield
Write-Output $city
In this example, we extract the city name from a full address by specifying the starting index and the length of the substring.
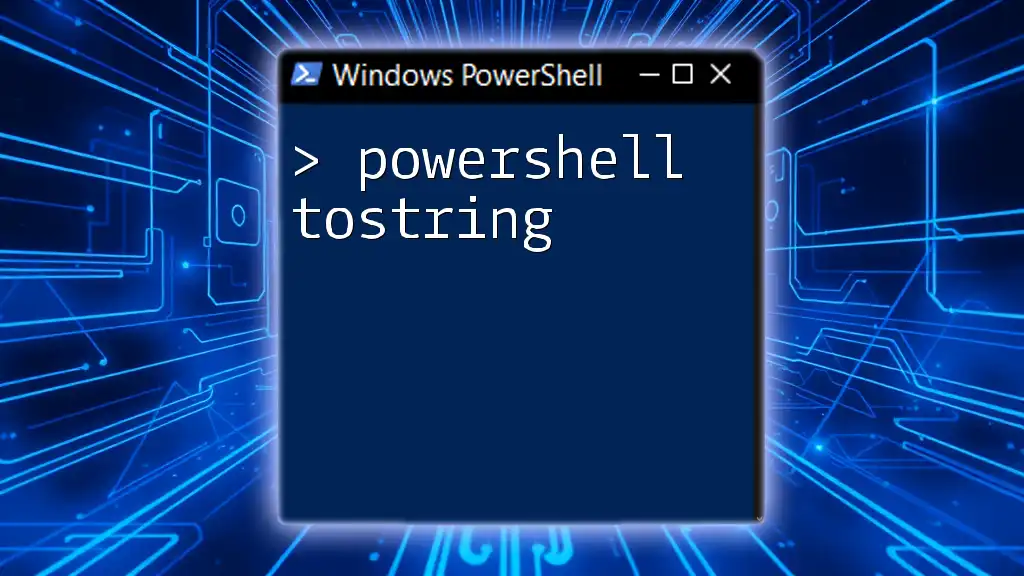
Conclusion
In this guide, we explored the powerful capabilities of the PowerShell Get Substring command, demystifying the process of extracting substrings. We began with the basics of defining substrings, and introduced the fundamental use cases, syntax, and practical examples.
Mastering substring manipulation can significantly streamline your PowerShell scripting and enhance the clarity and effectiveness of your data handling. Don't hesitate to practice these techniques and expand your PowerShell skills further!