In PowerShell, you can find a substring within a string using the `-like` operator or the `.Contains()` method to check for its existence.
Here's a code snippet illustrating both methods:
# Using -like operator
$string = 'Hello, World!'
$substring = 'World'
if ($string -like "*$substring*") {
Write-Host "'$substring' found in '$string'"
}
# Using .Contains() method
if ($string.Contains($substring)) {
Write-Host "'$substring' found in '$string'"
}
Understanding Substrings in PowerShell
What is a Substring?
A substring is simply a sequence of characters within a larger string. For instance, in the string "PowerShell is powerful," the substring "Power" is a portion of the complete string. Mastering substring operations allows you to efficiently manipulate and analyze text data, which is a common requirement in scripting and automation tasks. Understanding how to find and extract substrings can significantly enhance your scripting capabilities and make your workflows more efficient.
Importance of Finding Substrings
Finding substrings in larger strings is a critical skill for PowerShell scripting. Common use cases include:
- Data Validation: Ensuring that specific criteria are met within strings.
- Log Analysis: Extracting relevant information from logs (e.g., error messages).
- Filtering Output: Narrowing down command results based on substring matching.
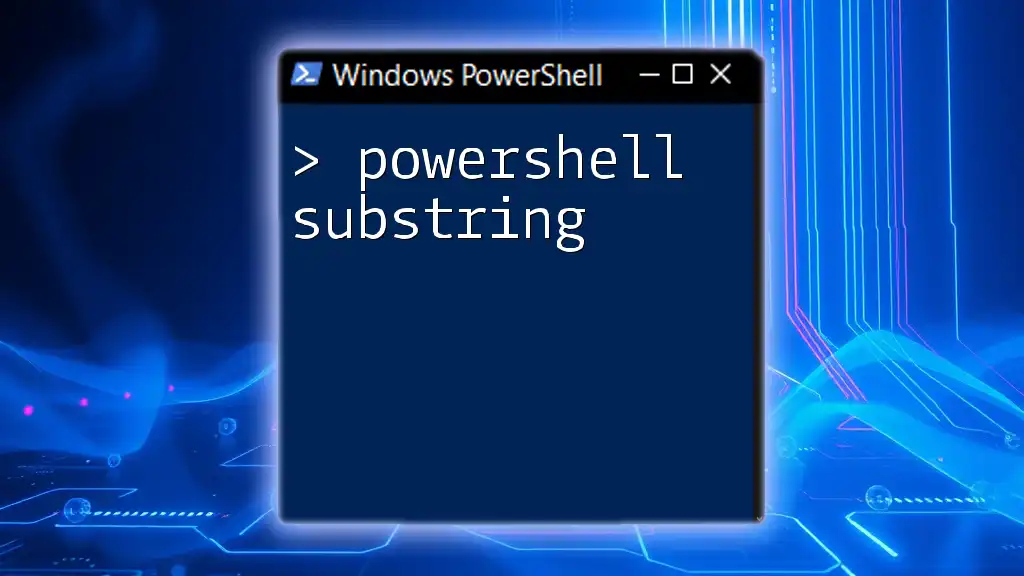
Finding Substrings in PowerShell
Using the `-like` Operator
The `-like` operator in PowerShell is used for pattern matching and allows for the incorporation of wildcard characters. This is particularly useful for finding substrings with flexible conditions.
$string = "Hello World"
if ($string -like "*World*") {
Write-Host "Substring found!"
}
In this example, the script checks if "World" exists within the string "Hello World." The wildcard `*` before and after the substring allows for any sequence of characters, confirming the substring is indeed present.
Using the `-match` Operator
The `-match` operator leverages regular expressions for pattern matching, providing a powerful tool for substring searches.
$string = "PowerShell Scripting"
if ($string -match "Scripting") {
Write-Host "Substring exists!"
}
This example illustrates how to use regex to detect whether "Scripting" exists within the string. The `-match` operator is case-sensitive, which is important to note when matching substrings.
Using the `.Contains()` Method
When you want a straightforward way to check for a substring, the `.Contains()` method is an excellent choice.
$string = "Learn PowerShell"
if ($string.Contains("PowerShell")) {
Write-Host "PowerShell found in string."
}
In this case, the script checks if "PowerShell" is part of "Learn PowerShell." If it is, it outputs a confirmation message. This method is simple and effective; however, it's important to remember that it is also case-sensitive.
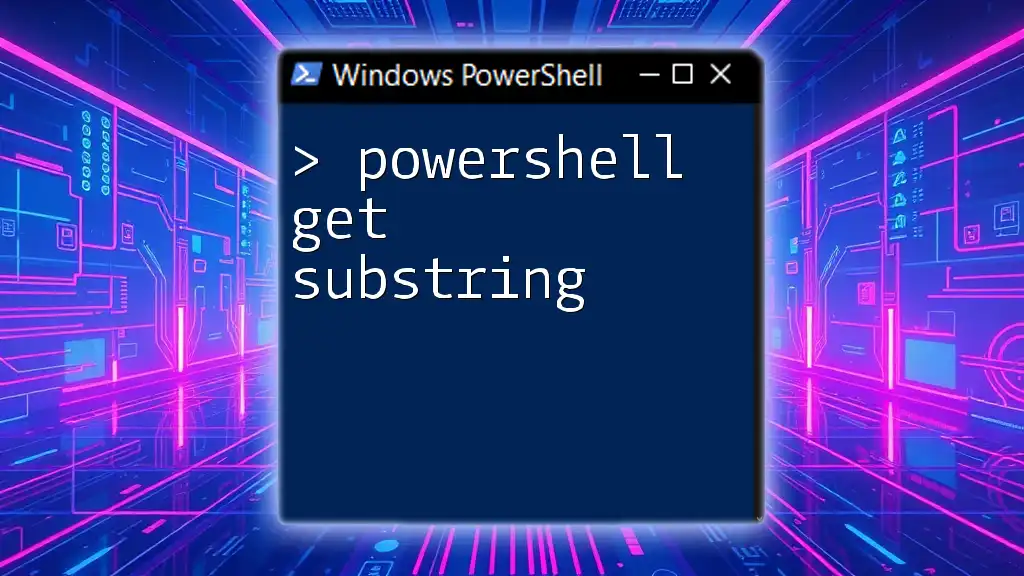
Extracting Substrings in PowerShell
Using the `Substring()` Method
The `Substring()` method is essential for extracting specific segments of a string, defined by a starting index and length.
$string = "PowerShell is great"
$substring = $string.Substring(0, 10) # Output: "PowerShell"
Write-Host $substring
Here, the `Substring()` method retrieves "PowerShell" by starting at index 0 and encompassing 10 characters. Understanding how indices work (starting from zero) is key to using this method effectively.
Using the `Split()` Method
The `Split()` method is an efficient way to break down a string into smaller parts based on a delimiter.
$string = "apples, oranges, bananas"
$fruits = $string.Split(", ")
Write-Host $fruits[1] # Output: "oranges"
In this example, the string is split by the comma and space. The output retrieves "oranges," demonstrating how `Split()` makes it easy to access specific substrings within a larger string.
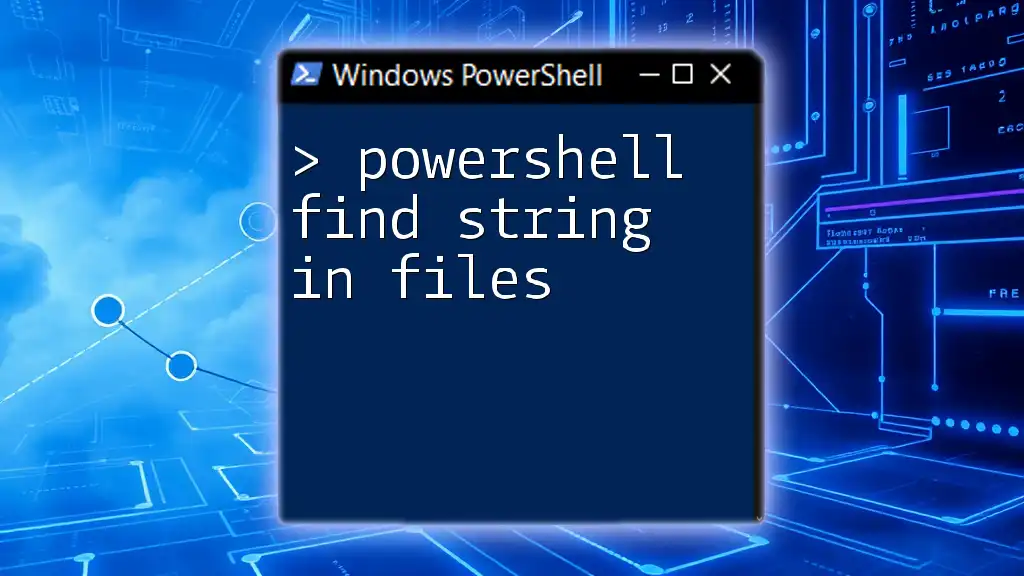
Practical Examples of PowerShell Substring Operations
Searching for Substrings in Log Files
Finding substrings can be particularly helpful when analyzing log files for specific entries, such as error or warning messages. For example, if you wanted to search through a log file for all entries that contain "Error," your script might look something like this:
Get-Content "C:\Logs\application.log" | Where-Object { $_ -like "*Error*" }
This command reads a log file and filters only the lines that contain "Error," allowing for quick and effective analysis of potential issues.
Filtering Output Using Substrings
Another common application of substring finding is within command outputs. For instance, the command below uses `Where-Object` to filter processes containing the name "Power":
Get-Process | Where-Object { $_.Name -like "*Power*" }
This lists all processes whose names include "Power," demonstrating how to effectively filter system information based on string matching.
Combining Methods for Advanced String Manipulation
By integrating various methods, you can perform more complex operations. For instance, if you want to count how many times the term "Power" appears in a string, you can do so as follows:
$string = "PowerShell is very Power"
$count = ($string.Split("Power").Count) - 1
Write-Host "The word 'Power' appears $count times."
In this example, the `Split()` method divides the string by "Power," counting the segments to determine occurrences. The subtraction adjusts for the split resulting in one extra count.
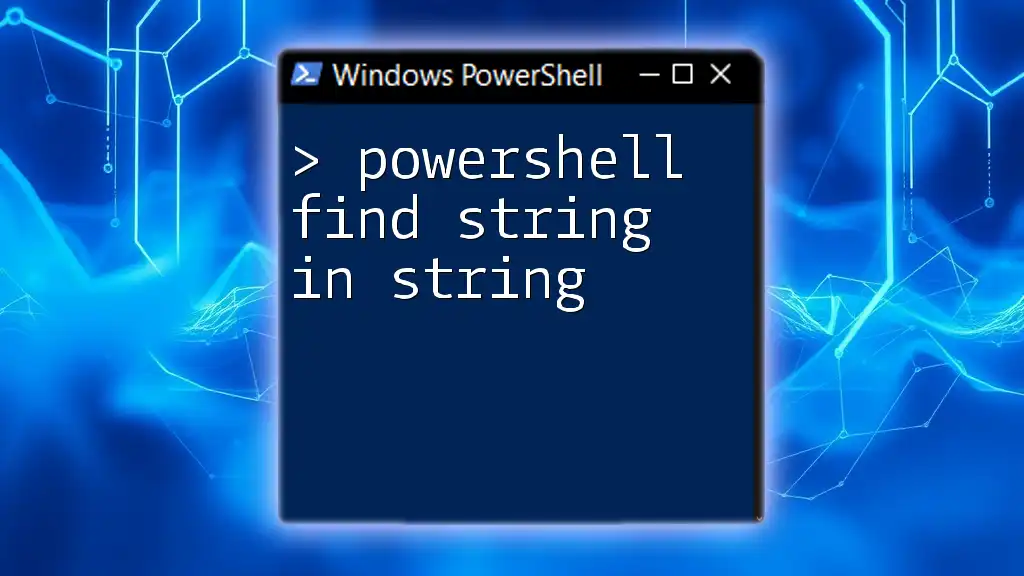
Common Pitfalls to Avoid
Case Sensitivity Issues
PowerShell string operations are case-sensitive by default. For instance, if you use the `-like` operator and search for "power," it will not match "Power." To make the search case-insensitive, use the `-ilike` operator instead:
if ($string -ilike "*power*") {
Write-Host "Substring found ignoring case!"
}
Misunderstanding String Length
When using the `Substring()` method, it's crucial to comprehend how string lengths are calculated. An incorrect understanding can lead to exceptions. For example, attempting to extract a substring that goes beyond the actual string length will result in an error.
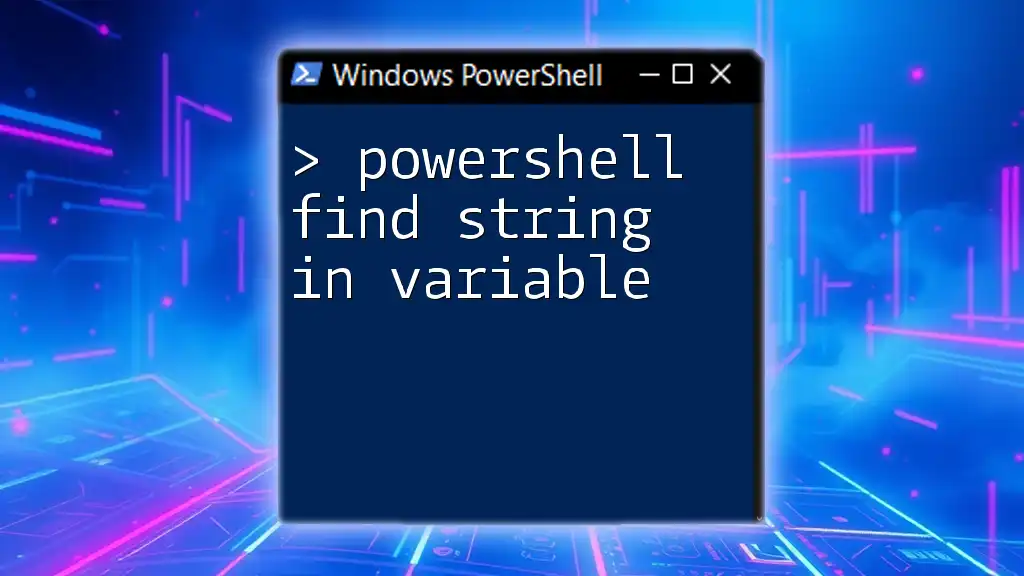
Conclusion
Mastering how to find substrings in PowerShell is essential for effective string manipulation and automation. From basic searches using `-like` and `-match` to advanced substring extraction methods, being proficient in these techniques can significantly enhance your scripting efficiency. Regular practice with real-world examples, such as log file analysis and output filtering, will further solidify your skills in working with PowerShell substrings.