Certainly! To search for a specific string within files using PowerShell, you can utilize the `Select-String` cmdlet, which scans through files and returns the lines containing the desired text.
Here's a code snippet for that:
Select-String -Path "C:\path\to\your\files\*.txt" -Pattern "yourSearchString"
Understanding PowerShell String Search
What is a String in the Context of PowerShell?
In PowerShell, a string is a sequence of characters used to represent text data. Strings are fundamental in scripting as they enable you to work with text in various forms, such as file names, text content, command parameters, and more. For instance, the string `"Hello, World!"` is a simple representation of a greeting.
Benefits of Using PowerShell for File Search
Using PowerShell to search for strings in files offers several advantages:
- Speed: PowerShell is optimized for efficient file operations, allowing quick searches across multiple files.
- Flexibility: You can tailor your search commands and patterns according to specific needs, including searching multiple file types or using regular expressions.
- Integration: PowerShell commands can be combined with other cmdlets and scripts, enhancing functionality and easing automation tasks.

Basic PowerShell Commands for Searching Strings
Get-Content
The `Get-Content` cmdlet is used to read content from files. Its syntax is straightforward:
Get-Content -Path "filepath"
For example, to read the contents of a text file located at `C:\example\file.txt`, you would use:
Get-Content -Path "C:\example\file.txt"
This command outputs the entire content of the specified file, line by line.
Select-String
The `Select-String` cmdlet is specifically designed for searching through file content. Its syntax is:
Select-String -Path "filepath" -Pattern "search_string"
For instance, to search for the term `"error"` in all `.txt` files in `C:\example`, use:
Select-String -Path "C:\example\*.txt" -Pattern "error"
The output will display the filename, line number, and the line itself where the string was found, making it easy to identify occurrences quickly.

Advanced Searching Techniques
Searching Multiple Files
PowerShell offers the ability to search multiple files using wildcards. You can specify different file types or a directory. For example, to find the word `"warning"` in all `.log` files, you can run:
Select-String -Path "C:\example\*.log" -Pattern "warning"
To include subdirectories in your search, utilize the `-Recurse` parameter:
Select-String -Path "C:\example\*.*" -Pattern "urgent" -Recurse
This command will search through all files in the `C:\example` directory and its subdirectories for the string `"urgent"`.
Case Sensitivity and Regular Expressions
PowerShell allows you to control case sensitivity in your searches. For example, if you want to search for the exact casing of `"Error"`, use:
Select-String -Path "C:\example\file.txt" -Pattern "Error" -CaseSensitive
You can also leverage regular expressions to create more complex search patterns. For instance, if you want to find variations of the word "failure," such as `"failed"` or `"failure"`, you can use:
Select-String -Path "C:\example\*.txt" -Pattern "fail(ed|ure)"
This flexibility lets you create powerful search commands tailored to your specific needs.

Output Options for Search Results
Displaying Output in Different Formats
The default output format of `Select-String` provides essential information, but you might prefer to save the results to a file for further examination. To save search results to a text file named `results.txt`, use:
Select-String -Path "C:\example\*.txt" -Pattern "failed" | Out-File -FilePath "C:\example\results.txt"
This command not only executes the search but also redirects the results into a new file, enabling easier review.
Filtering and Formatting Results
To enhance the readability of your output, you can use formatting commands like `Format-Table` or `Format-List`. For example, to filter and display the results with specific attributes such as filename, line number, and the line content, you could run:
Select-String -Path "C:\example\*.txt" -Pattern "not found" | Format-Table Filename, LineNumber, Line
This makes it easier to interpret the output at a glance, streamlining your workflow.
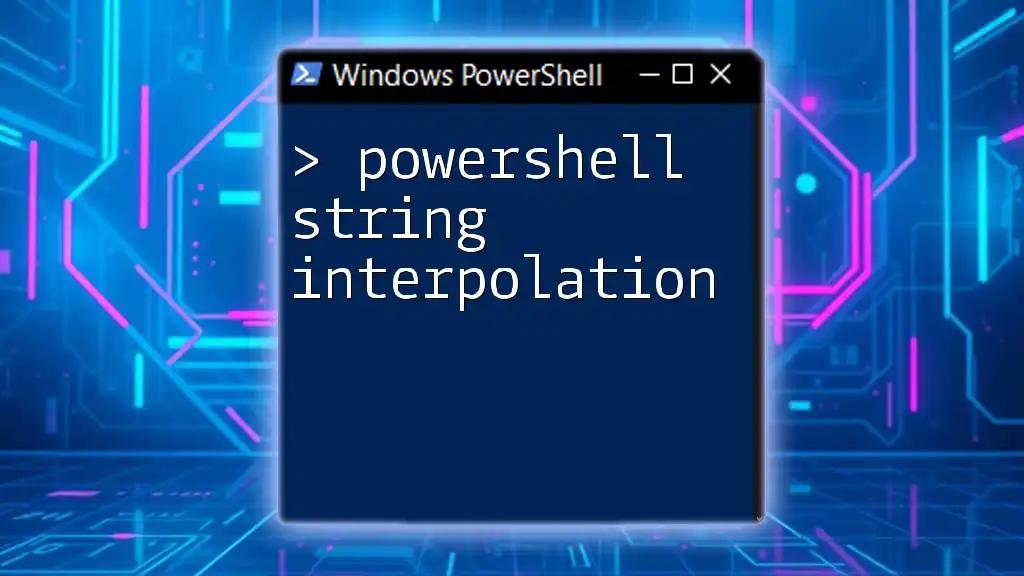
Practical Use Cases of Finding Strings in Files
Troubleshooting and Debugging
Searching for strings within files is an essential skill for troubleshooting and debugging. For example, system administrators often need to identify and analyze error messages in log files. By leveraging PowerShell, you can quickly pinpoint issues in large log files without manual inspection, making problem-solving much more efficient.
Automation Scripts
Integrating string searches in automated scripts can enhance monitoring and alerting processes. For instance, if you want to be notified when a specific failure occurs in a log file, you could implement a script that executes the string search and triggers an email alert upon detection. Here's a sample snippet demonstrating this:
if (Select-String -Path "C:\example\*.log" -Pattern "failure") {
Send-MailMessage -To "admin@example.com" -Subject "Alert: Failure Detected" -Body "Check log files."
}
This script continuously monitors log files for the specified string and sends an email alert to the system administrator if a match is found, thus automating the monitoring process.

Conclusion
In summary, mastering the command to PowerShell find string in files empowers users to unlock the full potential of PowerShell in file management. With its speed, flexibility, and integrating capabilities, PowerShell becomes an invaluable tool for searching and automating tasks. Practicing these techniques can enhance your skills and efficiency, making file operations and problem-solving faster and more effective. By continuing to explore and experiment with these commands, you'll become increasingly proficient in the realm of PowerShell scripting.
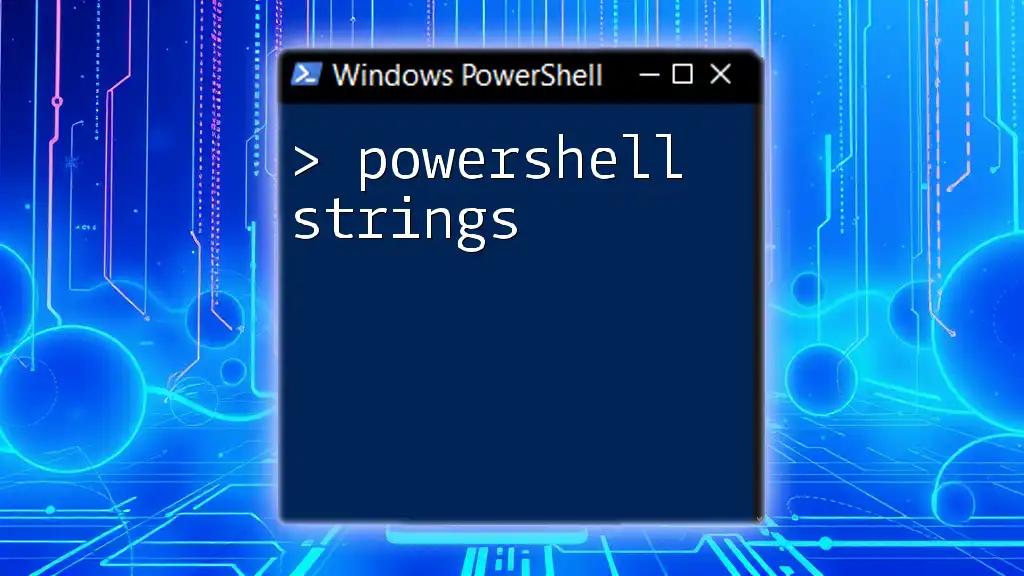
Additional Resources
For further reading, consider exploring the official PowerShell documentation, which provides in-depth guidance on advanced commands and scripting techniques. I encourage you to subscribe for future articles and tutorials to continue developing your PowerShell expertise.