To efficiently locate and manage duplicate files using PowerShell, you can utilize the following command snippet that identifies and lists files sharing the same hash value.
Get-ChildItem "C:\Path\To\Directory" -Recurse | Group-Object -Property Length, LastWriteTime | Where-Object { $_.Count -gt 1 } | Select-Object Name, Count
Understanding Duplicate Files
What are Duplicate Files?
Duplicate files are exactly what they sound like—multiple copies of the same file stored on your system. These can occur for a variety of reasons, including accidental copies made during file management, system backups that store identical files more than once, or software applications duplicating files during their operation.
Why You Should Remove Duplicate Files
Managing duplicate files is essential for several reasons:
- Storage Space Considerations: Duplicate files consume valuable hard drive space, potentially leading to performance degradation over time as storage fills up.
- Improved Organization and Efficiency: Having multiple copies of files can clutter your system and disrupt your workflows. Organizing your files and removing duplicates can lead to a cleaner and more efficient digital filing system.
- Avoidance of Confusion and Errors: Multiple copies can cause confusion, resulting in the mishandling of files. This may lead to using outdated versions or missing critical updates.
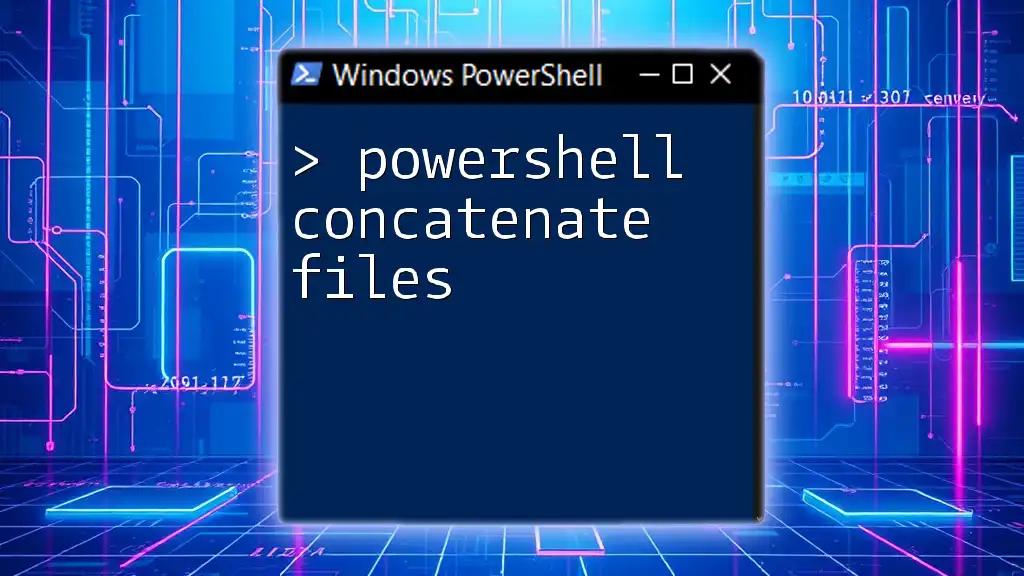
Getting Started with PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and shell designed specifically for task automation and configuration management. Unlike the traditional Command Prompt, PowerShell is built on the .NET framework and can interact with various components of the operating system, providing a more robust solution for file management.
Setting Up Your PowerShell Environment
Accessing PowerShell on Windows is straightforward. You can find it by searching for "PowerShell" in the Start menu. For tasks that require elevated permissions, such as modifying system files, run PowerShell as an Administrator by right-clicking on its icon and selecting "Run as Administrator."
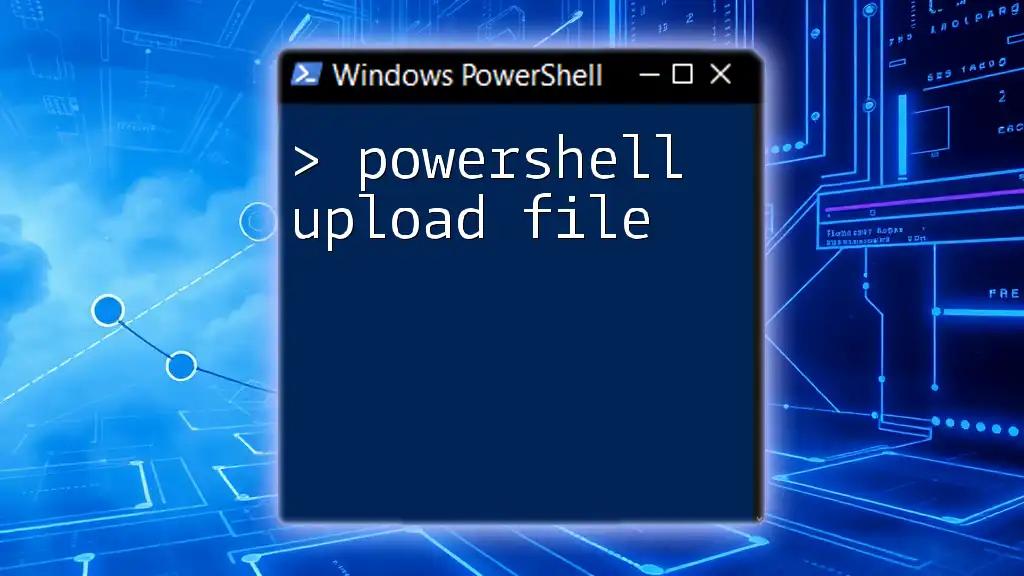
Finding Duplicate Files Using PowerShell
Basic Command for Finding Duplicates
To begin the process of finding duplicate files, you can leverage the `Get-ChildItem` cmdlet, which retrieves a list of files from a specified directory. This command is an essential building block in your search for duplicate files.
Example:
Get-ChildItem -Path "C:\Your\Directory" -Recurse
In this command, replace `"C:\Your\Directory"` with the path of the directory you want to search. The `-Recurse` parameter allows PowerShell to look through all subdirectories.
Grouping Files by Hash
Understanding File Hashes
File hashes are unique identifiers generated by hash functions to represent file contents. These hashes allow for quick comparison, making it possible to identify duplicate files effectively. Common hash algorithms include MD5 and SHA256, with SHA256 being the preferred choice due to its robustness.
Using `Get-FileHash` to Find Duplicates
To utilize file hashes for identifying duplicates, you can use the `Get-FileHash` cmdlet. By hashing files and storing their hashes in a collection, you can then quickly identify duplicates by checking for repeated hashes.
Example:
$files = Get-ChildItem -Path "C:\Your\Directory" -Recurse
$hashes = @{}
foreach ($file in $files) {
$hash = Get-FileHash -Path $file.FullName -Algorithm SHA256
if ($hashes.ContainsKey($hash.Hash)) {
$hashes[$hash.Hash] += [string]$file.FullName
} else {
$hashes[$hash.Hash] = [string]$file.FullName
}
}
In this script, we retrieve all files in the specified directory and iterate through them to generate a hash for each file. The results are stored in a dictionary, where the key is the hash value and the value is the associated file path.
Filtering and Displaying Duplicate Files
Once all files are hashed, you can filter the hash collection to find duplicates easily.
Example:
$hashes.GetEnumerator() | Where-Object { $_.Value.Count -gt 1 }
This command outputs only those hashes that have more than one associated file, effectively displaying the duplicates.
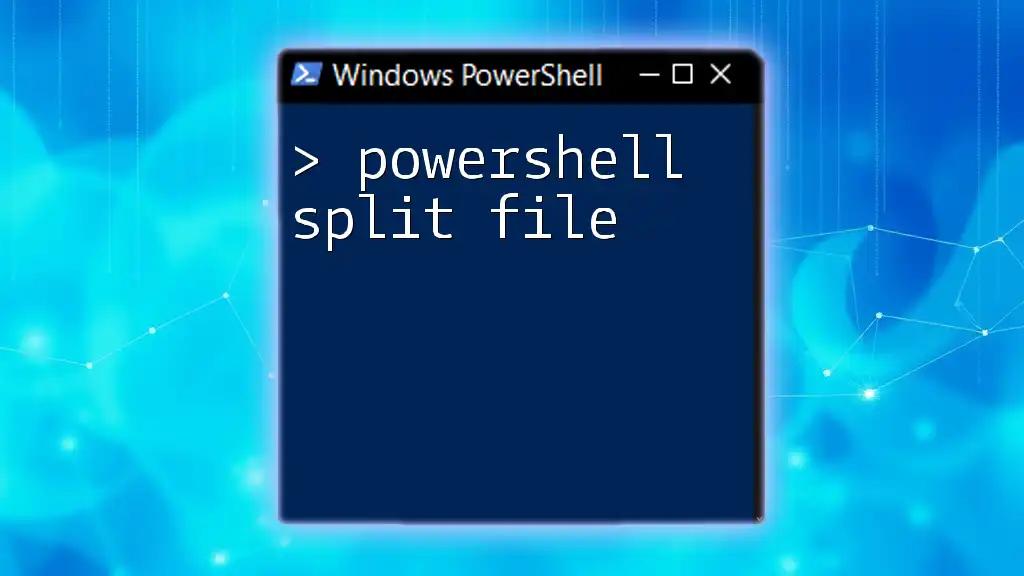
Advanced Techniques
Using PowerShell Functions
Creating a Custom Function to Find Duplicates
PowerShell allows you to encapsulate complex scripts within functions for easier reuse. By creating a function to find duplicates, you streamline the process.
Code Snippet for a Custom Function:
function Find-Duplicates {
param (
[string]$directory
)
$files = Get-ChildItem -Path $directory -Recurse
$hashes = @{}
foreach ($file in $files) {
$hash = Get-FileHash -Path $file.FullName -Algorithm SHA256
if ($hashes.ContainsKey($hash.Hash)) {
$hashes[$hash.Hash] += [string]$file.FullName
} else {
$hashes[$hash.Hash] = [string]$file.FullName
}
}
$hashes.GetEnumerator() | Where-Object { $_.Value.Count -gt 1 }
}
In this function, replace the `$directory` parameter with the desired path when calling it to find duplicate files within that directory.
Scheduled Tasks for Regular Maintenance
To avoid future buildup of duplicate files, consider automating the detection process. Using Windows Task Scheduler, you can schedule the execution of your duplicate file finder script at regular intervals. This proactive approach ensures a consistently organized file system.

Best Practices for Managing Duplicate Files
Regular Audit
To maintain a clean digital environment, perform regular audits of your files. The frequency of these audits can depend on your usage patterns, but considering a monthly review can be an effective way to stay on top of duplicate files.
Using Backup and Restore Points
Before deleting duplicate files, ensure you have a backup. Utilize restore points or external storage solutions to create backups of your critical files. This precaution offers a safety net should you inadvertently remove important files.
Tools Complementing PowerShell
Several GUI-based tools can complement your PowerShell scripts for file management. Tools like Duplicate Cleaner and CCleaner allow for visual inspection of duplicate files before deletion. Using these tools alongside PowerShell can enhance your workflow for managing duplicate files.

Conclusion
Managing duplicate files is crucial for an efficient and organized digital workspace. By utilizing PowerShell as a tool to find and eliminate duplicates, you can free up storage space and improve your system’s performance. Now that you are equipped with the knowledge to use PowerShell for finding duplicate files, you can take proactive steps in maintaining a clutter-free environment. Engage with the community, share your experiences, and explore additional resources to deepen your understanding of PowerShell.