To upload a file using PowerShell, you can use the `Invoke-WebRequest` cmdlet to send the file to a specified URL.
Invoke-WebRequest -Uri 'http://example.com/upload' -Method Post -InFile 'C:\path\to\your\file.txt'
Understanding PowerShell File Upload Methods
What is PowerShell?
PowerShell is a versatile automation and scripting framework built on the .NET framework, designed to help IT professionals automate tasks and manage configurations. Unlike traditional command-line interfaces, PowerShell operates with a rich object model and supports a plethora of commands, known as cmdlets, which allow for intricate management of systems.
Common Scenarios for File Uploading
In the realm of file management, the need to upload files can arise in various contexts, such as:
- Uploading to remote servers for data sharing or backup.
- Uploading to cloud storage services like OneDrive or AWS S3 for secure storage and retrieval.
- Transferring files between systems in a local network for collaboration or system maintenance.
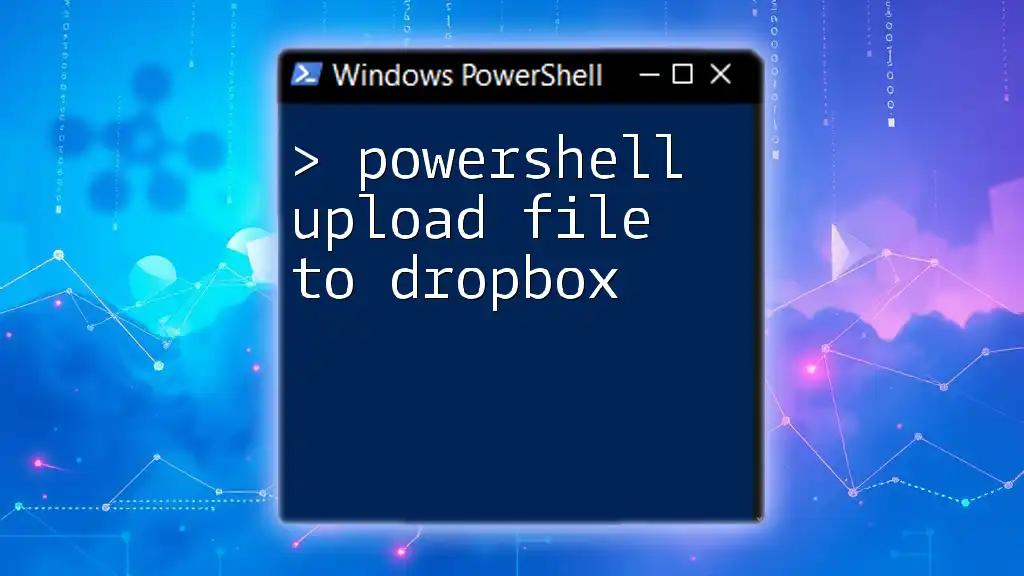
Uploading Files Using PowerShell
Using `Copy-Item` for Local Transfers
The `Copy-Item` cmdlet is a fundamental command in PowerShell, tailored for file and directory copying within local and remote locations.
Syntax
The basic syntax of `Copy-Item` is as follows:
Copy-Item -Path <String> -Destination <String> [-Recurse] [-Force]
- `-Path` specifies the source file or directory.
- `-Destination` designates where to copy the item.
- `-Recurse` allows copying all items in subdirectories.
- `-Force` overwrites files at the destination without prompting.
Example Code Snippet:
Copy-Item -Path "C:\LocalFolder\File.txt" -Destination "\\RemoteServer\SharedFolder"
In this example, the file `File.txt` is copied from a local folder to a specified location on a remote server.
Uploading Files to Remote Servers with `SCP`
What is SCP?
Secure Copy Protocol (SCP) is used for securely transferring files between systems over SSH (Secure Shell). SCP guarantees that files are uploaded over encrypted connections, making it a secure method for file transfers.
Using PowerShell with SSH and SCP
Before utilizing SCP, ensure you have an SSH client installed. PowerShell supports SCP through command-line interfaces.
Example command:
scp C:\LocalFolder\File.txt username@remotehost:/remote/directory/
In this command:
- `C:\LocalFolder\File.txt` is the local file to upload.
- `username@remotehost:/remote/directory/` specifies the target user and destination path on the remote server.
Uploading Files to Cloud Storage with PowerShell
OneDrive Uploading
OneDrive serves as a prominent cloud storage solution, offering seamless integration with PowerShell for file management.
Using Microsoft Graph API
To upload files to OneDrive, Microsoft Graph API is commonly utilized. This API enables programmatic access to OneDrive files and folders, requiring an authentication token for access.
Example Code Snippet:
Invoke-RestMethod -Uri "https://graph.microsoft.com/v1.0/me/drive/root:/File.txt:/content" `
-Method PUT -Headers @{Authorization = "Bearer $token"} `
-InFile "C:\LocalFolder\File.txt"
In this example:
- The API call uploads `File.txt` from the local directory to the root of the OneDrive.
- Ensure that the `Bearer $token` is acquired through a secure authentication process.
Uploading Files with WebDAV
What is WebDAV?
WebDAV (Web Distributed Authoring and Versioning) is an extension of HTTP that facilitates the collaborative management of files on remote web servers.
Uploading a File using `WebClient`
PowerShell allows interaction with WebDAV using the .NET `WebClient` class, which simplifies the upload process.
Example Code Snippet:
$webClient = New-Object System.Net.WebClient
$webClient.Credentials = New-Object System.Net.NetworkCredential("username", "password")
$webClient.UploadFile("http://webdavserver/path/File.txt", "PUT", "C:\LocalFolder\File.txt")
In this example,
- Credentials are specified to authenticate access to the WebDAV server.
- The command uploads the specified file to the desired directory.
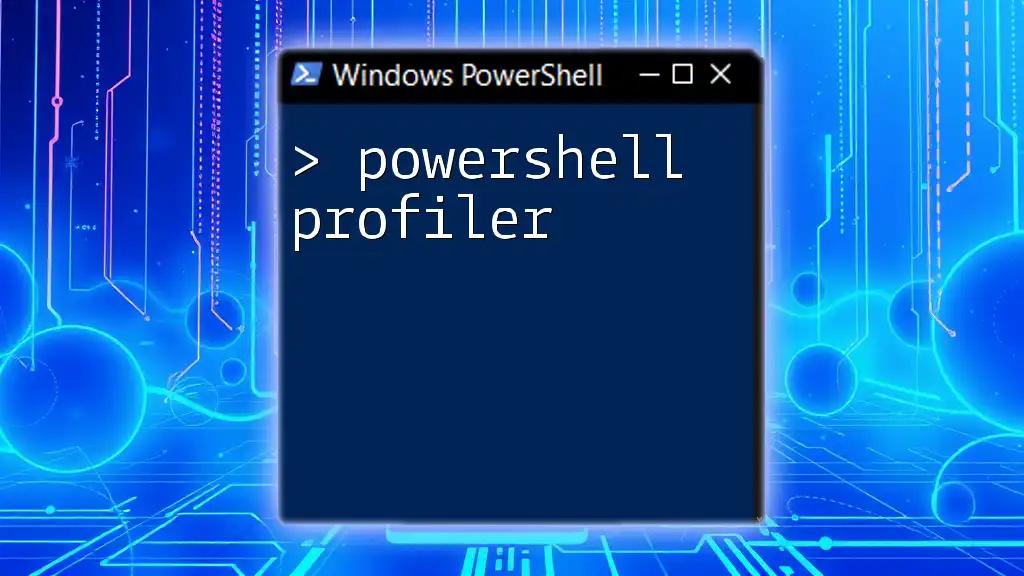
Troubleshooting Common Upload Issues
Common Errors and Their Solutions
When performing PowerShell upload file operations, users may encounter several common errors, including:
- "Access Denied": Often occurs due to insufficient permissions. Verify user privileges on the remote server or directory.
- Network-related issues: Make sure the remote server is accessible and ensure that firewall settings allow the connection.
Logging and Monitoring File Uploads
Maintaining logs can ease troubleshooting. Capturing errors and successes is vital for effective file management.
Sample Code for Logging:
try {
# PowerShell file upload operation
} catch {
# Log error to a file
$_ | Out-File "C:\Logs\UploadErrors.log" -Append
}
This code snippet captures errors during file upload operations, redirecting them to a log file for review.
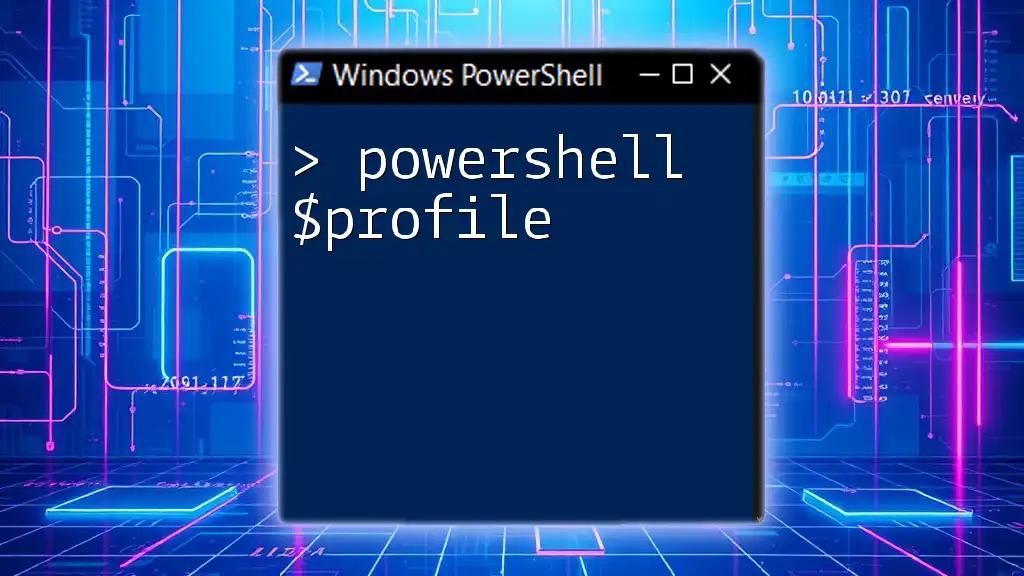
Best Practices for Uploading Files with PowerShell
Security Considerations
Security is paramount when uploading files. Always preferentially use secure methods like SCP or HTTPS when transmitting sensitive data. Additionally, manage and obfuscate credentials carefully to prevent unauthorized access.
Performance Tips
For handling large files, consider the following:
- Use compression to reduce upload times.
- Monitor bandwidth and time-out settings for efficient file transfers.

Conclusion
In this guide, we explored various methods for PowerShell upload file operations. From utilizing simple commands like `Copy-Item` to leveraging advanced APIs for cloud storage, PowerShell offers robust solutions for file management.
Experiment with these methods to enhance your automation skills and improve your overall productivity in managing files. For more PowerShell tips and tutorials, stay connected!
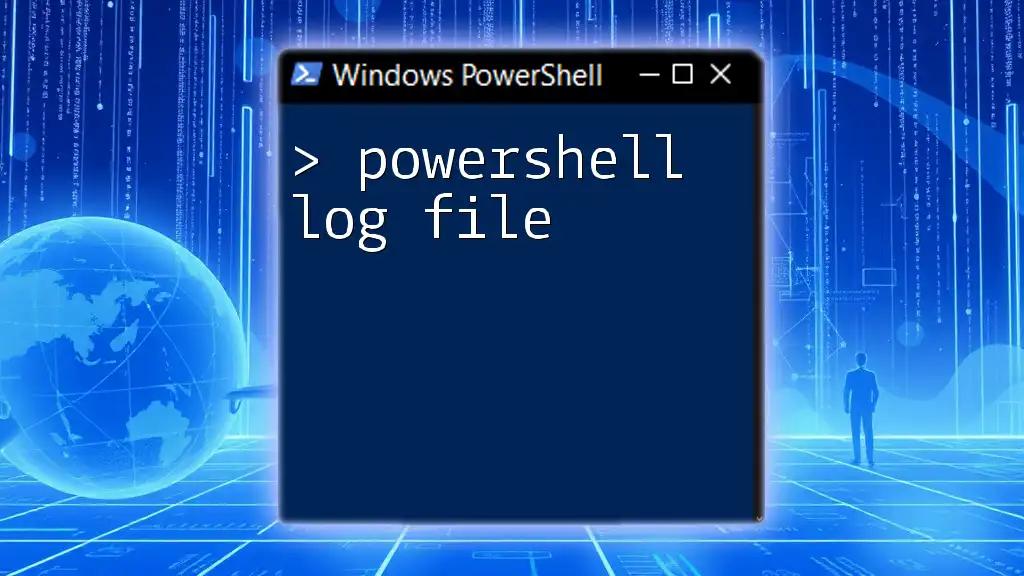
Additional Resources
For further learning, refer to official Microsoft PowerShell documentation, and consider enrolling in recommended PowerShell courses to deepen your knowledge and mastery of this powerful tool.