A PowerShell log file is a record that captures events and actions executed by PowerShell scripts or commands, providing valuable insights for debugging and monitoring.
Here's a simple code snippet to create a log file in PowerShell:
# Create a log file and write a message with a timestamp
$logFile = "C:\Logs\PowerShell_Log.txt"
$message = "$(Get-Date -Format 'yyyy-MM-dd HH:mm:ss') - Script executed successfully."
Add-Content -Path $logFile -Value $message
What is PowerShell Logging?
Definition of Logging in PowerShell
PowerShell logging refers to the process of capturing and storing information about actions executed in PowerShell scripts or commands. Logs serve as a record of events, errors, and transactions that happen during the execution of scripts, making it easier to troubleshoot issues, track changes, and audit usage.
Importance of Logging
Using log files in PowerShell is essential for several reasons:
- Troubleshooting: If a script fails or behaves unexpectedly, logs provide information about what occurred leading up to the failure.
- Auditing: Logs can be used to track who performed actions and what they did, which is crucial for security compliance.
- Monitoring: Continuous monitoring of logs helps in identifying trends, bottlenecks, or issues before they escalate.
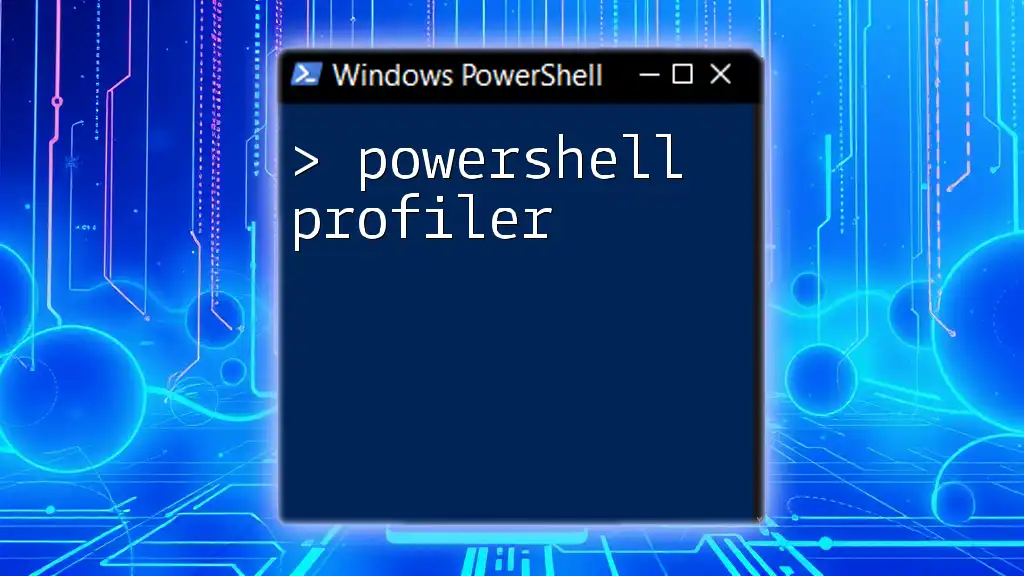
Creating Log Files in PowerShell
Basic Approach to Creating a Log File
Before recording any logs, you must ensure you have the necessary file system permissions to create log files. You can use the `New-Item` cmdlet to create a log file in your desired directory:
New-Item -Path "C:\Logs\mylog.txt" -ItemType File
This command creates an empty file named `mylog.txt` in the `C:\Logs` directory. Make sure the directory exists, or you'll encounter an error.
Writing to Log Files
Using Out-File Cmdlet
To write output to a log file, use the `Out-File` cmdlet. This cmdlet is efficient for logging messages to text files. For instance, you can append a new log entry as follows:
"Log entry at $(Get-Date)" | Out-File -FilePath "C:\Logs\mylog.txt" -Append
This command writes a new entry with the current timestamp to `mylog.txt`, adding it to the end of the file.
Using Redirection Operators
Another simple way to log messages is by using redirection operators. The `>>` operator appends output to a file:
"Log entry at $(Get-Date)" >> "C:\Logs\mylog.txt"
This approach is straightforward and requires less typing while achieving the same result.
Logging Script Events
When developing PowerShell scripts, incorporating logging directly within your script is beneficial. You can create a logging function, such as:
Function Log-Event {
param (
[string]$message
)
"$message - $(Get-Date)" >> "C:\Logs\mylog.txt"
}
# Call the function
Log-Event -message "Script started."
In this example, the `Log-Event` function takes a string message, appends the current date and time, and writes it to the log file. You can call this function throughout your script to keep a detailed log of its execution.
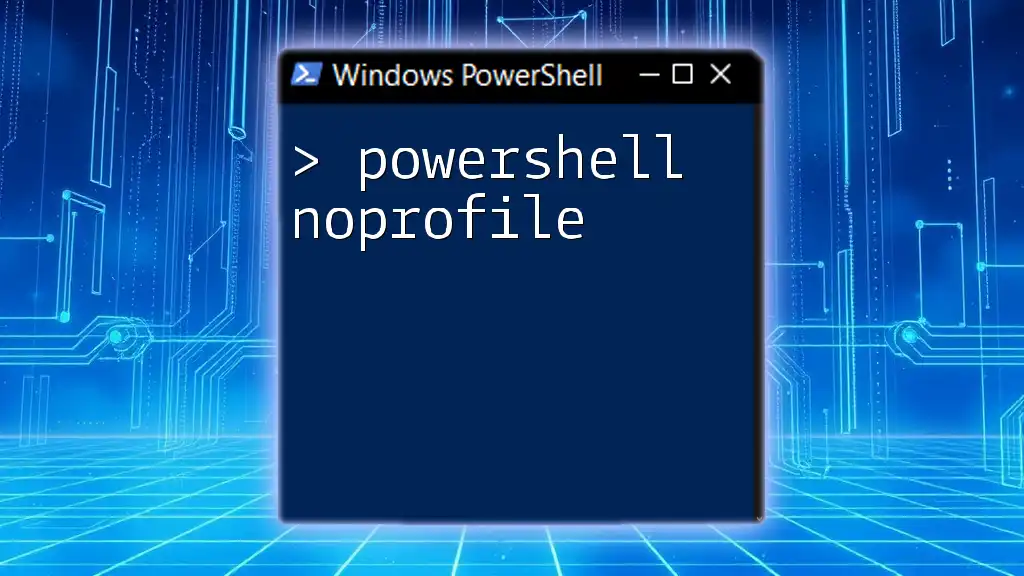
Managing Log Files
Viewing Log Files
To view the contents of your log files, use the `Get-Content` cmdlet, which reads the file line by line:
Get-Content -Path "C:\Logs\mylog.txt"
This command displays the entire contents of `mylog.txt` in the terminal, allowing you to review the logs.
Monitoring Log Files in Real-Time
PowerShell enables real-time log monitoring using the same `Get-Content` cmdlet with the `-Wait` parameter:
Get-Content -Path "C:\Logs\mylog.txt" -Wait
This command opens the log file and continuously outputs new entries as they are added, providing an immediate view of the log's activity.
Log File Size Management
Over time, log files can grow significantly in size. It’s crucial to implement size limits and manage log file retention. You can check a log file's size and rotate it if it exceeds a certain threshold:
$logPath = "C:\Logs\mylog.txt"
if ((Get-Item $logPath).length -gt 10MB) {
# Rotate log file or truncate
Move-Item -Path $logPath -Destination "C:\Logs\mylog_$(Get-Date -Format 'yyyyMMddHHmmss').txt"
New-Item -Path $logPath -ItemType File
}
In this code snippet, if the log file exceeds 10MB, it gets renamed with a timestamp, and a new log file is created for further logging.
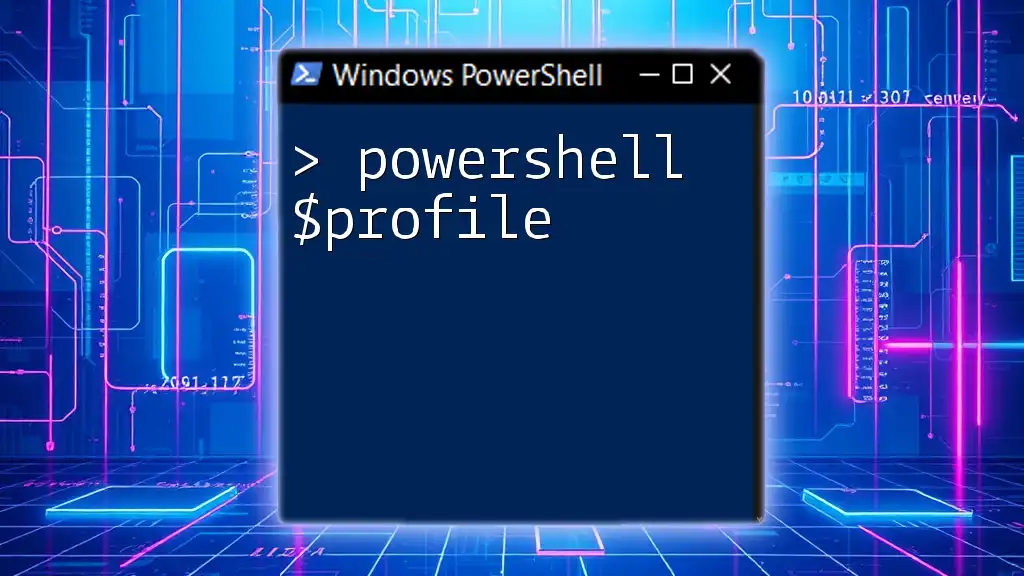
Analyzing Log Files
Searching through Log Files
When working with extensive log files, it's often necessary to search for specific entries. The `Select-String` cmdlet facilitates this:
Select-String -Path "C:\Logs\mylog.txt" -Pattern "Error"
This command searches for the term “Error” within `mylog.txt` and returns all lines containing that term, making it easier to identify issues.
Filtering and Reporting
You can filter logs to create a summary report based on specific criteria. For example:
$summary = Get-Content -Path "C:\Logs\mylog.txt" | Where-Object { $_ -like "*Error*" }
$summary
This command retrieves all lines that include the word “Error” and stores them in the `$summary` variable, which can then be displayed or processed further.
Exporting Log Data
For analytical purposes, you may want to export filtered log data to a CSV file for easy access and reporting:
$filteredLogs | Export-Csv -Path "C:\Logs\FilteredLogs.csv" -NoTypeInformation
This command exports the filtered logs to a CSV format, allowing more straightforward manipulation and integration with other tools.
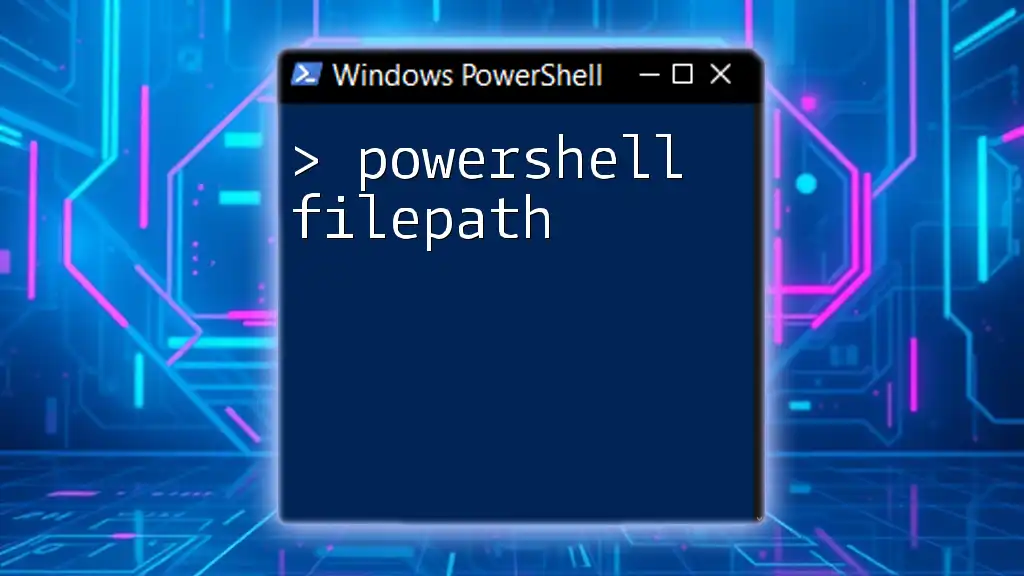
Best Practices for PowerShell Logging
Consistent Logging Format
Adopting a consistent format for your log entries is crucial for readability. Consider including essential elements such as timestamps and severity levels (e.g., INFO, ERROR) to enhance clarity and facilitate quick identification of issues.
Error Handling in Logging
When implementing logging, always consider error handling. Ensure your logging mechanism is robust enough to capture and log failures in your logging process without producing unnecessary errors or feedback.
Regular Maintenance
Logs, while invaluable, can consume significant disk space over time. It's critical to set a schedule for reviewing and maintaining log files, ensuring they do not grow indefinitely and remain manageable.
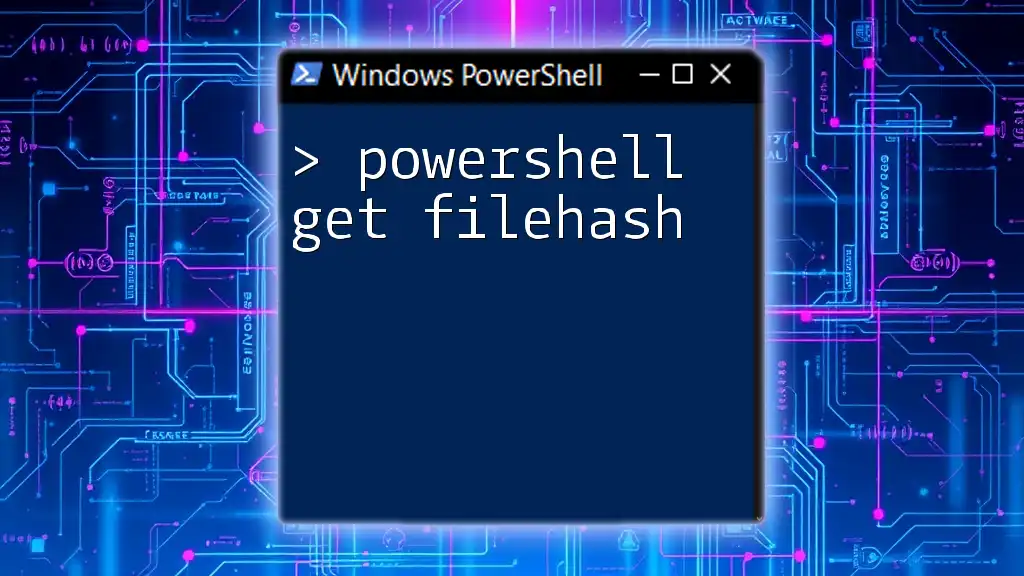
Conclusion
In summary, creating and managing PowerShell log files is an essential skill for any PowerShell user. Logging allows you to troubleshoot effectively, audit your work, and monitor system activity. Implementing logging practices not only enhances your scripts but also fortifies your overall administrative tasks. Make sure to adopt the strategies outlined in this guide to elevate your PowerShell projects.
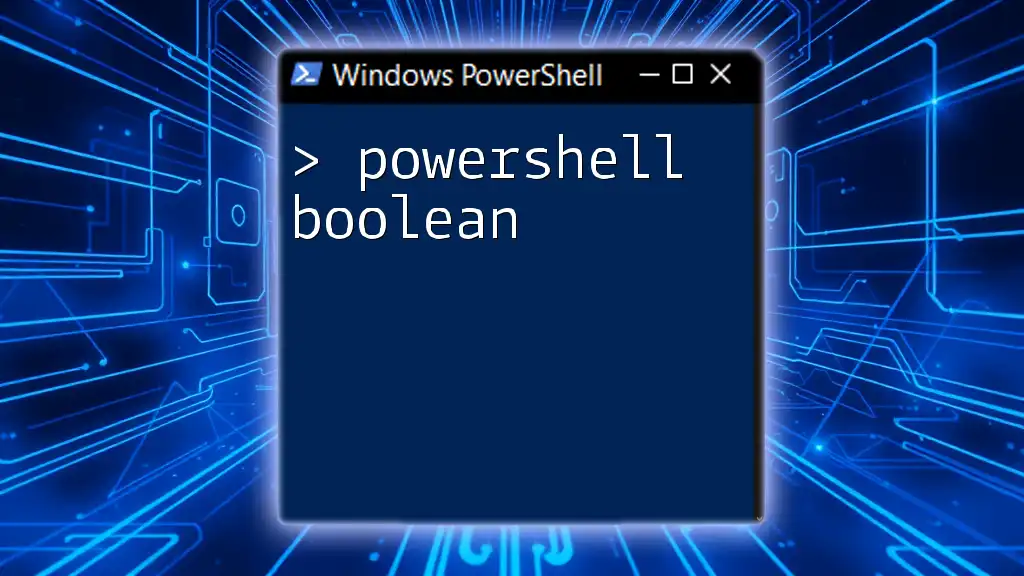
Call to Action
We encourage you to integrate logging into your PowerShell practices and share your experiences. If you have questions or comments about PowerShell log files, feel free to reach out! Additionally, subscribe to our newsletter for more tips and tricks to enhance your PowerShell expertise.